Java 入门学习记录(十一)
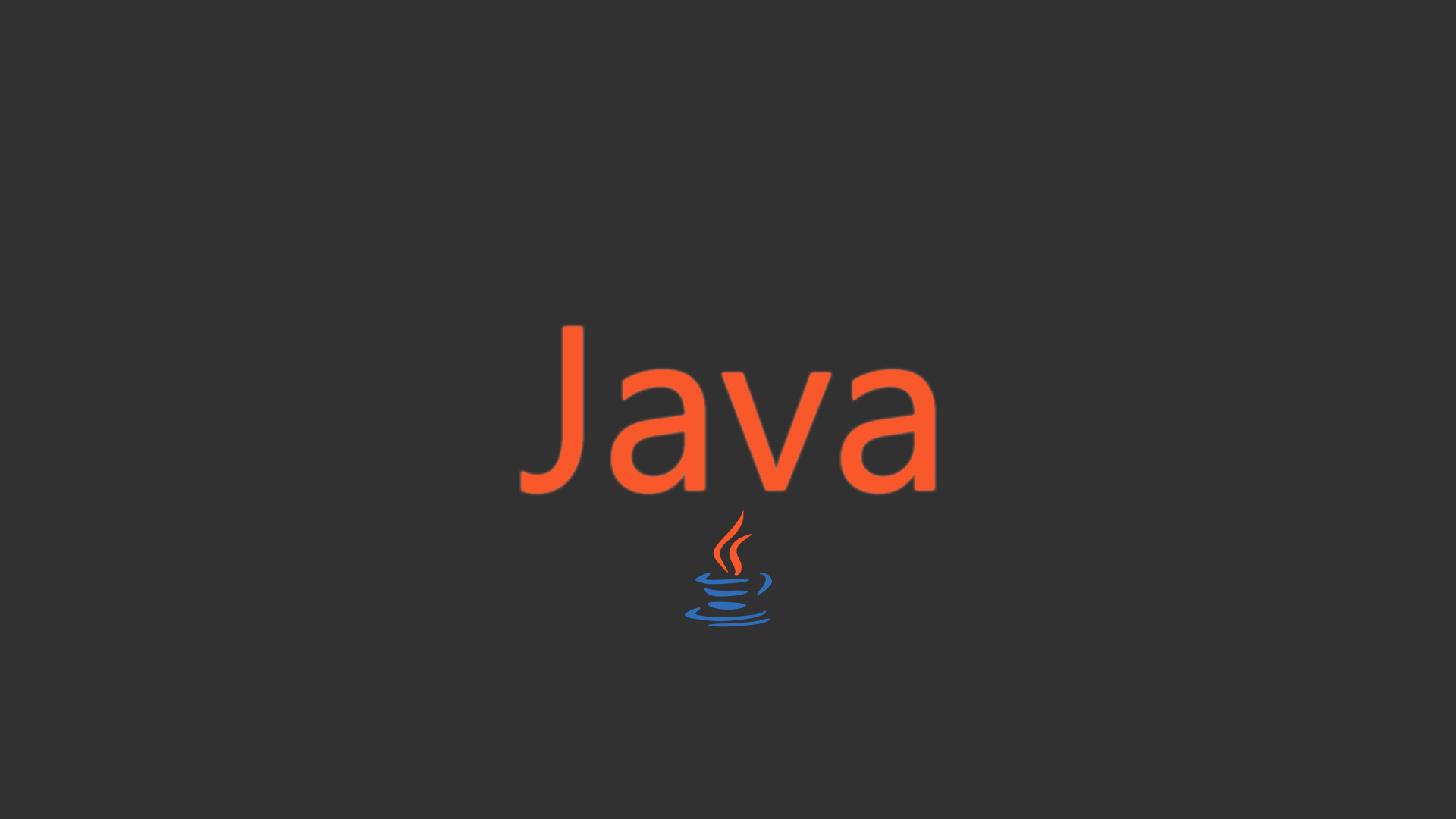
IP地址
import java.net.InetAddress;
import java.net.UnknownHostException;
public class TestInetAddress {
public static void main(String[] args) {
try {
// 查询本机地址
InetAddress byName = InetAddress.getByName("127.0.0.1");
System.out.println(byName);
InetAddress byName1 = InetAddress.getByName("localhost");
System.out.println(byName1);
InetAddress byName2 = InetAddress.getLocalHost();
System.out.println(byName2);
// 查询网站IP地址
InetAddress byName3 = InetAddress.getByName("www.baidu.com");
System.out.println(byName3);
// 常用方法
System.out.println(byName3.getAddress());
System.out.println(byName3.getCanonicalHostName());
System.out.println(byName3.getHostAddress());
System.out.println(byName3.getHostName());
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
}
端口
端口表示计算机上的一个程序的进程
- 不同进程有不同的进程 ID,用来区分软件
- 规定 0 - 65535
- TCP,UDP,IP:
- TCP:用户传输协议
- UDP:用户数据报协议
- IP:网络互联协议
OSI七层网络模型
|
TCP/IP四层概念模型
|
对应网络协议
| 应用层(Application) | 应用层 | HTTP, TFTP, FTP, NFS, WAIS, SMTP | 表示层(Presentation) | Telnet, Rlogin, SNMP, Gopher | 会话层(Session) | SMTP, DNS | 传输层(Transport) | 传输层 | TCP, UDP | 网络层(Network) | 网络层 | IP, ICMP, ARP, RARP, AKP, UUCP | 数据链路层(Data Link) | 数据链路层 | FDDI, Ethernet, Arpanet, PDN, SLIP, PPP | 物理层(Physical) | IEEE 802.1A, IEEE 802.2 - IEEE 802.11 | # TCP
创建客户端 TcpClientDemo1
import java.io.IOException;
import java.io.OutputStream;
import java.net.InetAddress;
import java.net.Socket;
import java.nio.charset.StandardCharsets;
public class TcpClientDemo1 {
public static void main(String[] args) {
Socket socket = null;
OutputStream os = null;
try {
InetAddress ServerIP = InetAddress.getByName("127.0.0.1");
int port = 9999;
socket = new Socket(ServerIP, port);
os = socket.getOutputStream();
os.write("hello world! 你好世界!".getBytes(StandardCharsets.UTF_8));
} catch (Exception e) {
e.printStackTrace();
} finally {
if (os != null) {
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket != null) {
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
创建服务端 TcpServerDemo1
import java.io.ByteArrayOutputStream;
import java.io.InputStream;
import java.net.ServerSocket;
import java.net.Socket;
public class TcpServerDemo1 {
public static void main(String[] args) throws Exception{
ServerSocket serverSocket = new ServerSocket(9999);
Socket accept = serverSocket.accept();
InputStream is = accept.getInputStream();
ByteArrayOutputStream baos = new ByteArrayOutputStream();
int len;
byte[] buffer = new byte[1024];
while ((len = is.read(buffer)) != -1) {
baos.write(buffer, 0, len);
}
System.out.println(baos.toString());
baos.close();
is.close();
accept.close();
serverSocket.close();
}
}
先启动客户端,后启动服务端程序,只接收发送一次,若多次写入接收,使用循环结构监听接收即可
文件上传
客户端
import java.io.*;
import java.net.InetAddress;
import java.net.Socket;
public class TcpClientDemo2 {
public static void main(String[] args) throws Exception{
int port = 9000;
Socket socket = new Socket(InetAddress.getByName("127.0.0.1"), port);
OutputStream os = socket.getOutputStream();
FileInputStream fis = new FileInputStream(new File("Java pic001.png"));
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
os.write(buffer, 0, len);
}
socket.shutdownOutput();
InputStream is = socket.getInputStream();
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] buffer2 = new byte[1024];
int len2;
while ((len2 = is.read(buffer2)) != -1) {
baos.write(buffer2, 0, len2);
}
System.out.println(baos.toString());
fis.close();
os.close();
socket.close();
}
}
服务端
import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;
public class TcpServerDemo2 {
public static void main(String[] args) throws Exception {
ServerSocket serverSocket = new ServerSocket(9000);
Socket socket = serverSocket.accept();
InputStream is = socket.getInputStream();
FileOutputStream fos = new FileOutputStream(new File("receive.png"));
byte[] buffer = new byte[1024];
int len;
while ((len = is.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
OutputStream os = socket.getOutputStream();
os.write("Received and Finished!".getBytes());
fos.close();
is.close();
socket.close();
serverSocket.close();
}
}
最后会在当前目录下产生一个 receive.png 的图片
UDP
UDP 发送不需要连接,但需要知道地址,类似发短信
建一个发送客户端
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
public class UdpClientDemo1 {
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket();
String msg = "Hello Server!";
InetAddress localhost = InetAddress.getByName("localhost");
int port = 9090;
DatagramPacket packet = new DatagramPacket(msg.getBytes(), 0, msg.getBytes().length, localhost, port);
socket.send(packet);
socket.close();
}
}
建一个接收端
import java.net.DatagramPacket;
import java.net.DatagramSocket;
public class UdpReceiverDemo {
public static void main(String[] args) throws Exception{
DatagramSocket socket = new DatagramSocket(6666);
while (true) {
byte[] buffer = new byte[1024];
DatagramPacket packet = new DatagramPacket(buffer, 0, buffer.length);
socket.receive(packet);
System.out.println("buffer.length: " + buffer.length);
byte[] data = packet.getData();
String datas = new String(data, 0, packet.getLength());
System.out.println("data.length: " + data.length);
System.out.println("packet.getLength(): " + packet.getLength());
if (datas.equals("bye")) {
break;
}
System.out.println(datas);
}
socket.close();
}
}
针对接收端的这个 length 与 getLength() 的问题,可以加如上输出语句可以得到结果
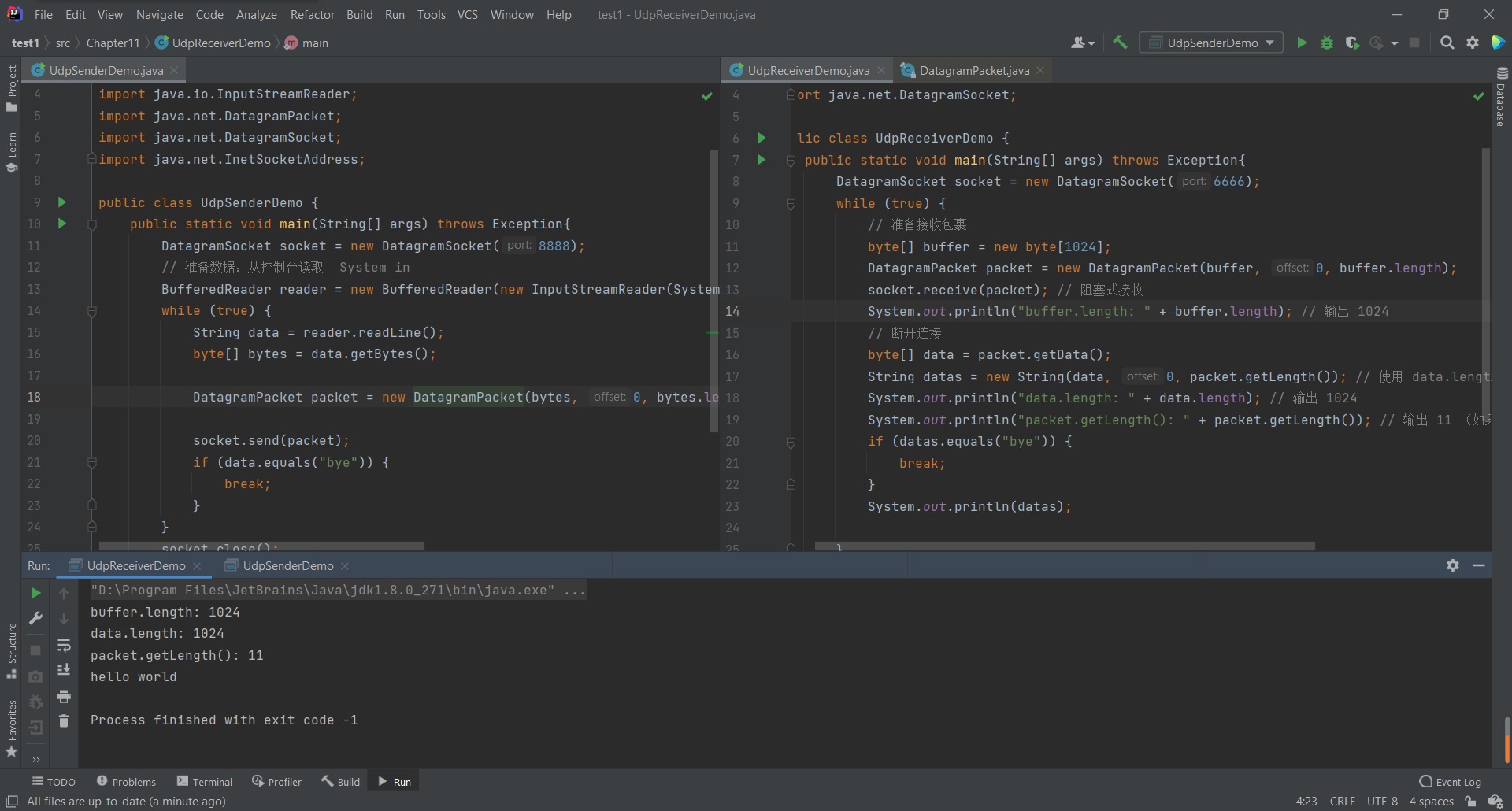
多线程聊天
创建发送程序
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetSocketAddress;
import java.net.SocketException;
public class TalkSender implements Runnable{
DatagramSocket socket = null;
BufferedReader reader = null;
private String toIP;
private int toPort;
private int fromPort;
public TalkSender(String toIP, int toPort, int fromPort) {
this.toIP = toIP;
this.toPort = toPort;
this.fromPort = fromPort;
try {
socket = new DatagramSocket(fromPort);
} catch (SocketException e) {
e.printStackTrace();
}
reader = new BufferedReader(new InputStreamReader(System.in));
}
@Override
public void run() {
while (true) {
String data = null;
try {
data = reader.readLine();
byte[] datas = data.getBytes();
DatagramPacket packet = new DatagramPacket(datas, 0, datas.length, new InetSocketAddress(this.toIP, this.toPort));
socket.send(packet);
if ("bye".equals(data)) {
break;
}
} catch (Exception e) {
e.printStackTrace();
}
}
try {
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
socket.close();
}
}
创建接收程序
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.SocketException;
public class TalkReceiver implements Runnable{
DatagramSocket socket = null;
private int Port;
private String Identify;
public TalkReceiver(int port, String identify) {
Port = port;
Identify = identify;
try {
socket = new DatagramSocket(Port);
} catch (SocketException e) {
e.printStackTrace();
}
}
@Override
public void run() {
while (true) {
byte[] bytes = new byte[1024];
DatagramPacket packet = new DatagramPacket(bytes, 0, bytes.length);
try {
socket.receive(packet);
} catch (IOException e) {
e.printStackTrace();
}
byte[] data = packet.getData();
String datas = new String(data, 0, packet.getLength());
System.out.println(this.Identify + ": " + datas);
if ("bye".equals(datas)) {
break;
}
}
socket.close();
}
}
学生代理端
public class TalkStudent {
public static void main(String[] args) {
new Thread(new TalkSender("localhost", 9999, 7777)).start();
new Thread(new TalkReceiver(8888, "Teacher")).start();
}
}
老师代理端
public class TalkTeacher {
public static void main(String[] args) {
new Thread(new TalkSender("localhost", 8888, 5555)).start();
new Thread(new TalkReceiver(9999, "Student")).start();
}
}
URL下载
URL方法介绍
import java.net.MalformedURLException;
import java.net.URL;
public class URLDemo {
public static void main(String[] args) throws MalformedURLException {
URL url = new URL("http://localhost:8080/helloworld/index.jsp?username=ShenyanWu&password=123");
System.out.println(url.getProtocol());
System.out.println(url.getHost());
System.out.println(url.getPort());
System.out.println(url.getPath());
System.out.println(url.getFile());
System.out.println(url.getQuery());
}
}
URL下载文件
利用 URL 下载,使用一个本地部署的 Tomcat 来测试
关于 Tomcat 的下载可以点击这里 : 传送门 提取码:1234
在下载文件中 bin/startup.bat 打开,然后 http://localhost:8080
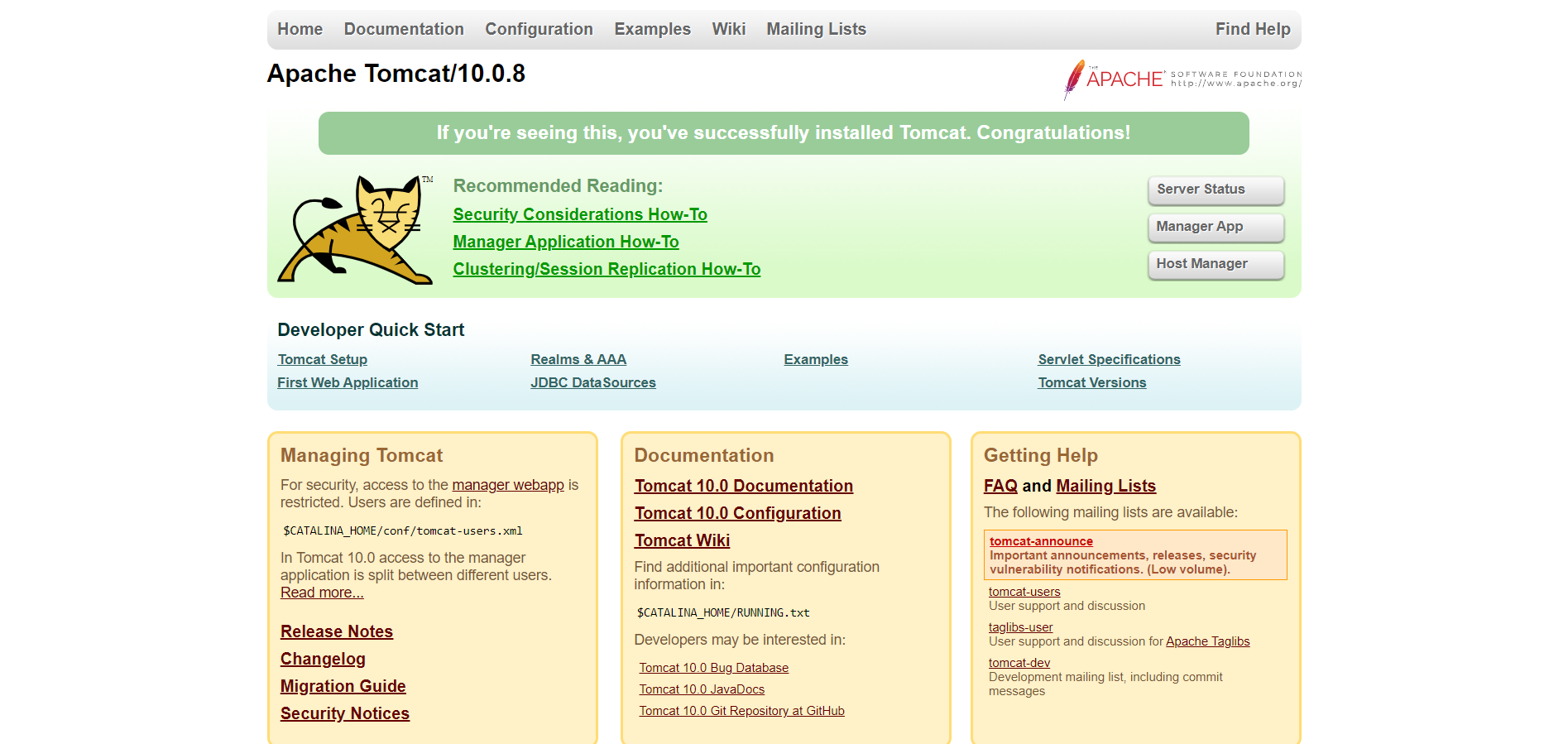
在文件中的 webapps 文件夹下创建文件夹为任意项目名称,新建一个 helloworld.txt 文件,内容随意即可
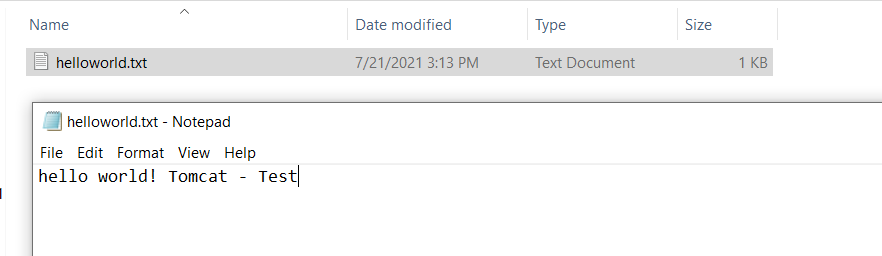
然后在 http://localhost:8080/TestJava/helloworld.txt 即可查阅文件
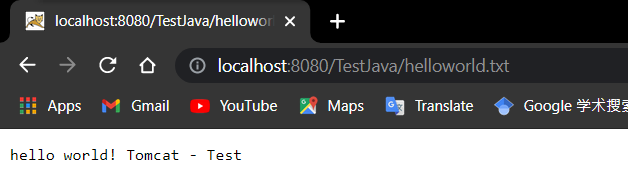
测试程序
import java.io.FileOutputStream;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class URLDownloadDemo {
public static void main(String[] args) throws Exception {
URL url = new URL("http://localhost:8080/TestJava/helloworld.txt");
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
InputStream inputStream = urlConnection.getInputStream();
FileOutputStream fos = new FileOutputStream("testDownload");
byte[] buffer = new byte[1024];
int len;
while ((len = inputStream.read()) != -1) {
fos.write(buffer, 0, len);
}
fos.close();
inputStream.close();
urlConnection.disconnect();
}
}
|