#include <stdio.h>
2 #include <unistd.h>
3 #include <string.h>
4 #include <arpa/inet.h>
5 #include <netinet/in.h>
6 #include <errno.h>
7 #include <sys/types.h>
8 #include <sys/socket.h>
9 #include <poll.h>
10 #define MAX_LISTEN_QUE 13
11
12 #define BUFF "hello client , i'm server!"
13 #define ARRAY_SIZE(x) (sizeof(x)/sizeof(x[0]))
14
15 int socket_init(void)
16 {
17 int ser_fd = -1;
18 int rv = -1;
19 int on = 1;
20 int port = 9998;
21 struct sockaddr_in ser_addr;
22
23 ser_fd = socket(AF_INET, SOCK_STREAM, 0);
24 if(ser_fd < 0)
25 {
26 printf("create socket failure:%s\n", strerror(errno));
27 return -1;
28 }
29 printf("\ncreate socket[%d]successfully!\n",ser_fd);
30
31 if((rv = setsockopt(ser_fd, SOL_SOCKET, SO_REUSEADDR, &on, sizeof(on))) < 0)
32 {
33 printf("\n设置地址重用失败:%s\n",strerror(errno));
34 return -1;
35 }
36
37 memset(&ser_addr, 0, sizeof(ser_addr));
38 ser_addr.sin_family = AF_INET;
39 ser_addr.sin_port = htons(port);
40 ser_addr.sin_addr.s_addr = htonl(INADDR_ANY);
41 rv = bind(ser_fd, (struct sockaddr *)&ser_addr, sizeof(ser_addr));
42 if(rv < 0)
43 {
44 printf("ser_fd[%d]bind port[%d]failure:%s\n", ser_fd, port, strerror(errno));
45 return -1;
46 }
47 printf("\nser_fd[%d]bind port[%d]successfully!\n", ser_fd, port);
48
49 rv = listen(ser_fd, MAX_LISTEN_QUE);
50 if(rv < 0)
51 {
52 printf("\nser_fd[%dlisten port[%d]failure:%s\n", ser_fd, port, strerror(errno));
53 return -1;
54 }
55 printf("\nser_fd[%dlisten port[%d]successfully!\n", ser_fd, port);
56
57 return ser_fd;
58 }
59
60
61
62 int main(int argc, char **argv)
63 {
64 struct pollfd fd_array[1024];
65 struct sockaddr_in cli_addr;
66 socklen_t len;
67 int maxfd;
68 int rv,i;
69 int connfd;
70 int listenfd;
71 int found;
72 char buff[1024] = {};
73
74 for(i=0; i<ARRAY_SIZE(fd_array); i++)
75 {
76 fd_array[i].fd = -1;
77 }
78
79 listenfd = socket_init();
80 fd_array[0].fd = listenfd;
81 fd_array[0].events = POLLIN;
82 maxfd = 0;
83 while(1)
84 {
85 rv = poll(fd_array, maxfd+1, -1);
86 if(rv < 0)
87 {
88 printf("socket poll failaru:%s\n",strerror(errno));
89 return -1;
90 }
91 printf("socket poll successfully\n");
92 if(fd_array[0].revents & POLLIN)
93 {
94 connfd = accept(listenfd, (struct sockaddr *)&cli_addr, &len);
95 if(connfd < 0)
96 {
97 printf("socket accpet new client failure:%s\n",strerror(errno));
98 return -1;
99 }
100 printf("accept new client[%d][%s:%d]successfully!\n", connfd, inet_ntoa(cli_addr.sin_addr),ntohs(cli_addr.sin_port));
101
102
103 found = 0;
104 for(i=1; i<ARRAY_SIZE(fd_array); i++)
105 {
106 if(fd_array[i].fd < 0)
107 {
108 fd_array[i].fd = connfd;
109 fd_array[i].events = POLLIN;
110 found = 1;
111 maxfd = i>maxfd ? i : maxfd;
112 break;
113 }
114 }
115 if(!found)
116 {
117 printf("客户端连接数量已达到最大值,所以拒绝连接\n");
118 return -1;
119 }
120 }
121 else
122 {
123 for(i=1; i<ARRAY_SIZE(fd_array); i++)
124 {
125 if(fd_array[i].fd < 0)
126 {
127 continue;
128 }
129 memset(buff, 0, sizeof(buff));
130 rv = read(fd_array[i].fd, buff, sizeof(buff));
131 if(rv < 0)
132 {
133 printf("read data from client [%d]failure:%s\n",fd_array[i].fd, strerror(errno));
134 close(fd_array[i].fd);
135 fd_array[i].fd = -1;
136 return -1;
137 }
138 else if(rv == 0)
139 {
140 printf("client get disconnection!\n");
141 close(fd_array[i].fd);
142 fd_array[i].fd = -1;
143 continue;
144 }
145 else if(rv > 0)
146 {
147 printf("read data from client[%d]successfully:%s\n", fd_array[i].fd, buff);
148 }
149 rv = write(fd_array[i].fd, BUFF, strlen(BUFF));
150 if(rv < 0)
151 {
152 printf("send data to client[%d]failure:%s\n", fd_array[i].fd, strerror(errno));
153
154 }
155 printf("send data to client[%d]successfully\n", fd_array[i].fd);
156
157 }
158 }
159 }
160 }
程序问题 在服务端运行并且连接TCP test TOOL的一个客户端之后,我再运行我自己写的客户端(一次发收数据就关闭),但是这里出现问题了,发送数据之后就卡住了,然后如果这时我使用TCP test工具的那个客户端发送数据,我自己写的那个客户端就能正常返回服务端的数据了。
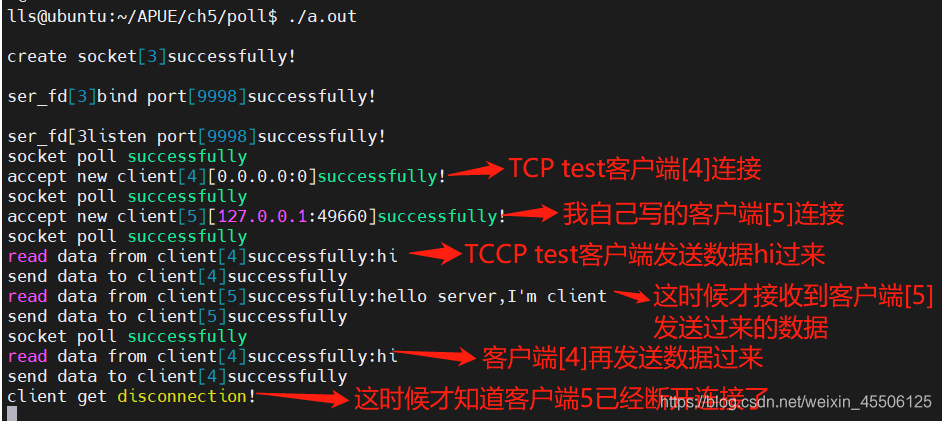
|