function tio (ws_protocol, ip, port, paramStr, param,handler,heartbeatTimeout, reconnInterval, binaryType) {
this.ip = ip
this.port = port
this.url = ws_protocol + '://' + ip + ':' + port
this.binaryType = binaryType || 'arraybuffer'
this.events = {};
this.type=null
if (paramStr) {
this.url += '?' + paramStr
this.reconnUrl = this.url + "&"
} else {
this.reconnUrl = this.url + "?"
}
this.reconnUrl += "tiows_reconnect=true";
this.param = param
this.heartbeatTimeout = heartbeatTimeout
this.reconnInterval = reconnInterval
this.lastInteractionTime = function () {
if (arguments.length == 1) {
this.lastInteractionTimeValue = arguments[0]
}
return this.lastInteractionTimeValue
}
this.heartbeatSendInterval = heartbeatTimeout / 2
this.connect = function (isReconnect) {
var _url = this.url;
if (isReconnect) {
_url = this.reconnUrl;
}
var ws = new WebSocket(_url)
this.ws = ws
ws.binaryType = this.binaryType;
var self = this
ws.onopen = function (event) {
self.fire('wsonopen')
self.lastInteractionTime(new Date().getTime())
self.pingIntervalId = setInterval(function () {
self.ping(self)
}, self.heartbeatSendInterval)
}
ws.onmessage = function (event) {
self.fire('wsonmessage',event)
self.lastInteractionTime(new Date().getTime())
}
ws.onclose = function (event) {
clearInterval(self.pingIntervalId)
try {
} catch (error) {}
console.log('1')
if(!self.type){
console.log('2')
self.reconn(event)
}
}
ws.onerror = function (event) {
}
return ws
}
this.reconn = function (event) {
var self = this
setTimeout(function () {
var ws = self.connect(true)
self.ws = ws
}, self.reconnInterval)
}
this.closeSocket = function () {
console.log('3')
this.type=true
clearInterval(this.reconnInterval)
this.ws.close()
}
this.ping = function () {
var iv = new Date().getTime() - this.lastInteractionTime();
if ((this.heartbeatSendInterval + iv) >= this.heartbeatTimeout) {
}
};
this.send = function(data){
this.ws.send(data);
};
this.on = (eventName, handler) => {
if (this.events[eventName]) {
this.events[eventName].funcs = [handler];
} else {
this.events[eventName] = {
funcs: [handler]
}
}
}
this.fire = (eventName, arg1, arg2) => {
if (!this.events) return;
if (!this.events[eventName]) return console.log('未订阅' + eventName + '事件');
let funcs = this.events[eventName].funcs || [];
funcs.forEach(func => {
if (func && typeof func === 'function') {
func(arg1, arg2);
}
});
}
this.removeEvent = (eventName) => {
if (eventName === 'all') {
this.events = null;
return false;
}
if (!this.events[eventName]) return;
this.events[eventName].funcs = [];
}
}
export default tio;
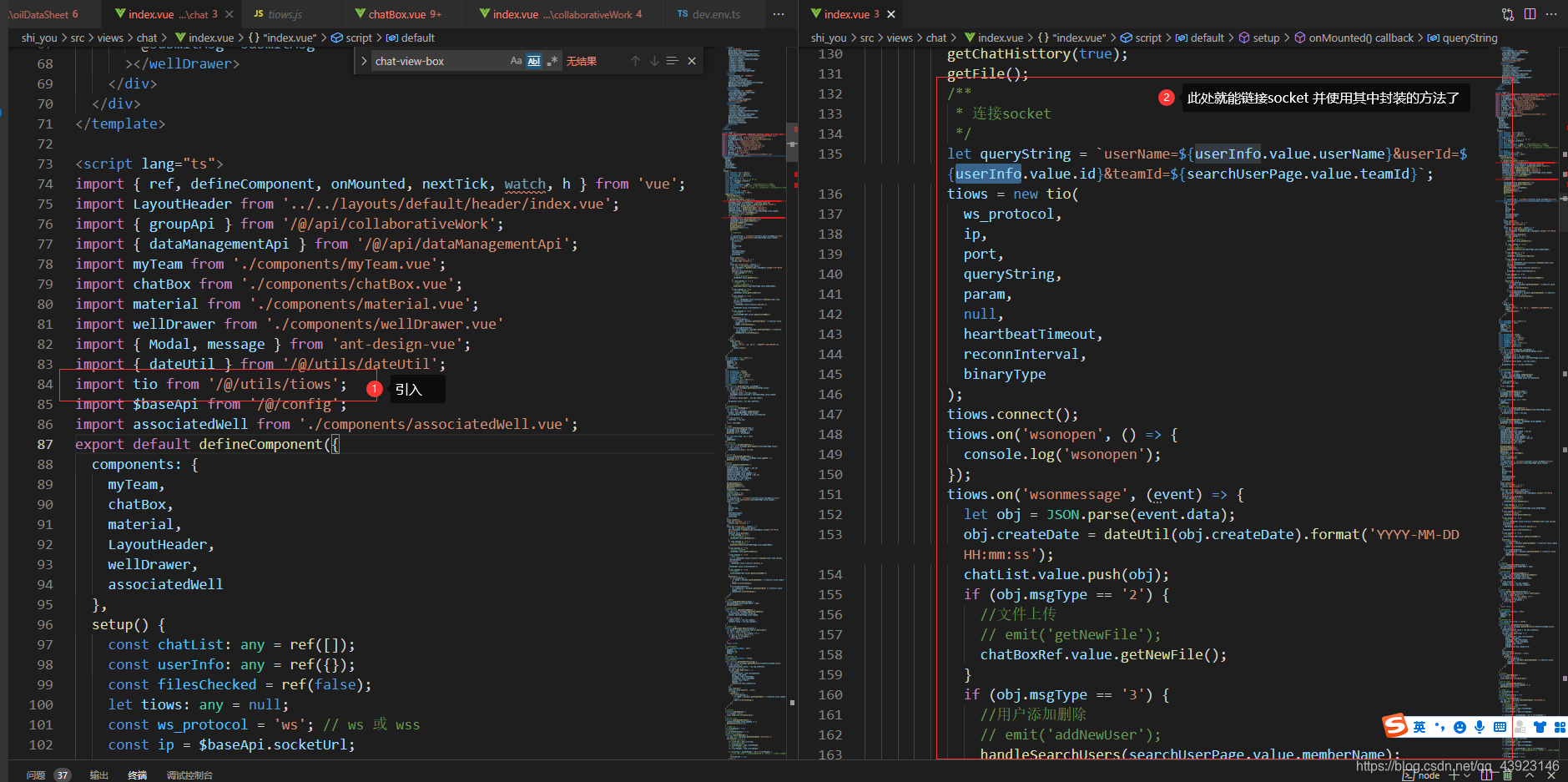
|