24.01 网络编程
A:网络模型
网络模型一般是指
OSI(Open System Interconnection开放系统互连)七层参考模型
TCP/IP四层参考模型
主机至网络层(物理层,数据链路层),网际层,传输层,应用层(
应用层,表示层,会话层)
网络模型7层概述:
1.物理层:主要定义物理设备标准,如网线的接口类型、光纤的接口
类型、各种传输介质的传输速率等。主要作用是传输比特
流
2.数据链路层:主要将从物理层接收的数据进行MAC地址(网卡的地址)
的封装与解封装。常把这一层的数据叫做帧。
3.网络层:主要将从下层接收到的数据进行IP地址的封装与解封装。
在这一层工作的设备是路由器,这一层的数据叫数据包。
4.传输层:定义了一些传输数据的协议和端口号。主要是将从下层接
收的数据进行分段和传输,到达目的地址后再进行重组。
这一层数据叫段。
5.会话层:通过传输层建立数据传输的通路。
主要在系统之间发起会话或者接受会话请求。
6.表示层:主要是进行对接的数据进行解释、加密与解密、压缩与解
压缩等。
7.应用层:主要是一些终端的应用。
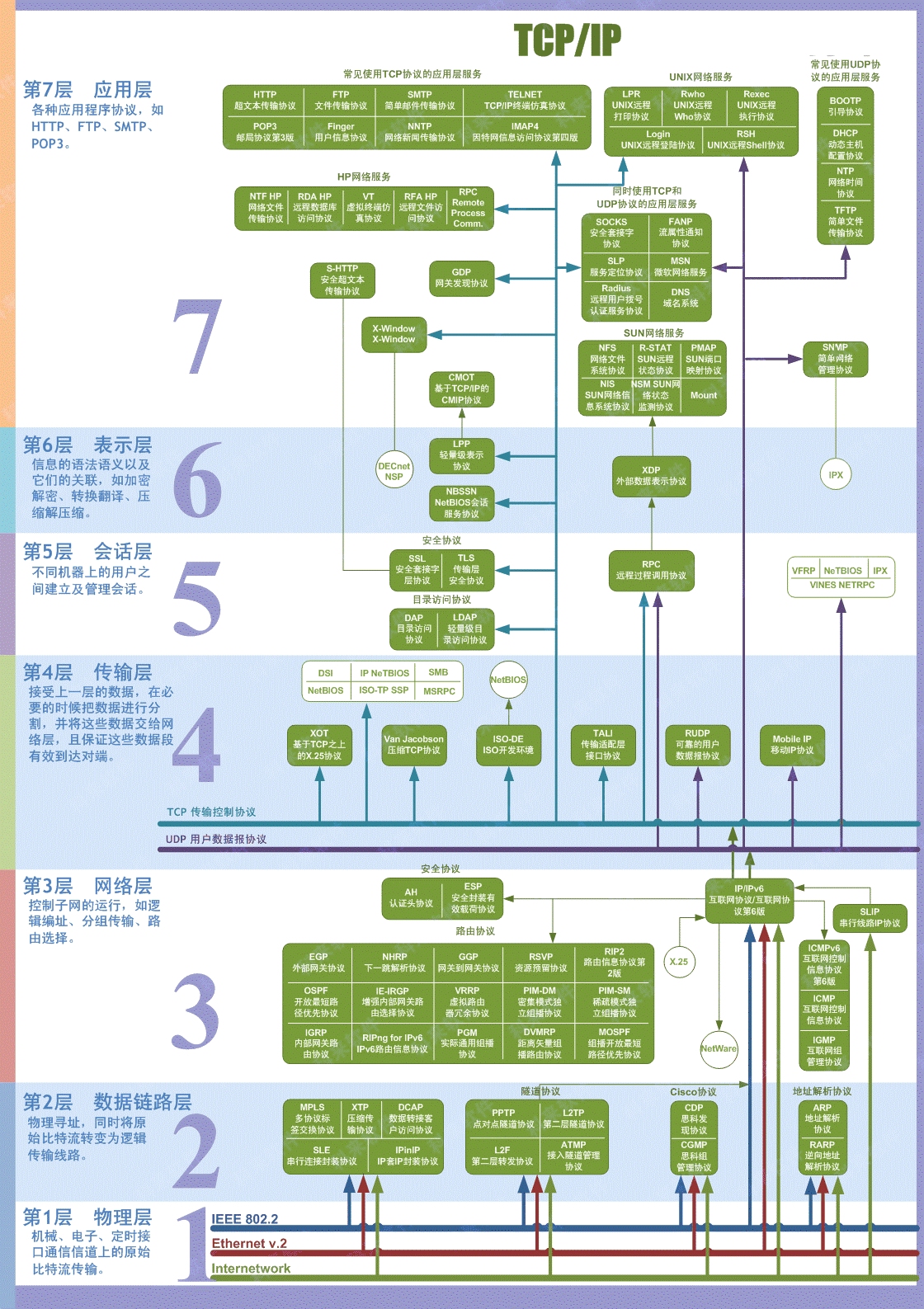
24.02 网络编程三要素
A:IP地址:InetAddress:网络中设备的标识,不易记忆,可用主机名
B:端口号:用于标识进程的逻辑地址,不同进程的标识
C:传输协议:常见TCP、UDP
24.03 InetAddress类的概述和使用
A:InetAddress类的概述
为了方便我们对IP地址的获取和操作,此类表示互联网协议(IP)地址。
B:InetAddress类的常见功能
public static InetAddress getByName(String host)
public String getHostAddress() //获取IP
public String getHostName() //获取主机名
getLocalHost()
public static void main(String[] args) throws UnknownHostException {
InetAddress address = InetAddress.getByName("LAPTOP-GM5215VR");
String hostName = address.getHostName();
String ip = address.getHostAddress();
System.out.println(hostName);
System.out.println(ip);
InetAddress localHost = address.getLocalHost();
System.out.println(localHost);
InetAddress[] allByName = InetAddress.getAllByName("LAPTOP-GM5215VR");
for (InetAddress inetAddress : allByName) {
String hostAddress = inetAddress.getHostAddress();
System.out.println(hostAddress);
}
}
24.04 Socket通信原理图解
A:Socket套接字概述:
网络上具有唯一标识的IP地址和端口号组合在一起才能构成唯一能识别的
标识符套接字。
B:Socket原理机制:
通信的两端都有Socket
网络通信其实就是Socket间的通信
数据在两个Socket间通过IO传输
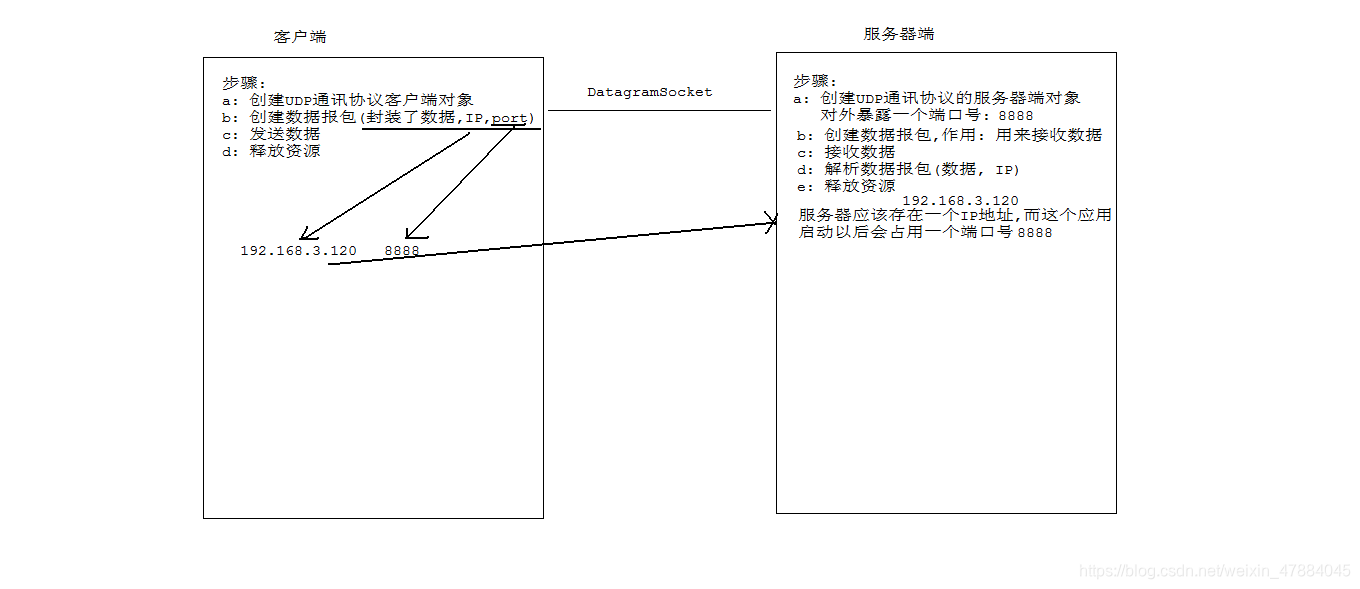 UDP通讯原理图
import java.io.IOException;
import java.net.*;
public class UDPClient {
public static void main(String[] args) throws IOException {
DatagramSocket ds = new DatagramSocket();
String msg = "你好UDP,我来了";
byte[] bytes = msg.getBytes();
DatagramPacket packet = new DatagramPacket(bytes, bytes.length, InetAddress.getByName("192.168.80.1"), 8888);
ds.send(packet);
ds.close();
}
}
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.SocketException;
public class UDPServer {
public static void main(String[] args) throws IOException {
DatagramSocket ds = new DatagramSocket(8888);
System.out.println("服务器已经开启,等待连接......");
byte[] bytes = new byte[1024];
DatagramPacket datagramPacket = new DatagramPacket(bytes,bytes.length);
ds.receive(datagramPacket);
byte[] data = datagramPacket.getData();
int length = datagramPacket.getLength();
String ip = datagramPacket.getAddress().getHostAddress();
String s = new String(data, 0, length);
System.out.println(ip + "发来消息" + s);
}
}
public class UDPClient {
public static void main(String[] args) throws IOException {
DatagramSocket ds = new DatagramSocket();
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
while (true){
System.out.println("请输入要发送的消息");
String msg = reader.readLine();
byte[] bytes = msg.getBytes();
DatagramPacket packet = new DatagramPacket(bytes, bytes.length, InetAddress.getByName("192.168.80.1"), 6666);
ds.send(packet);
if ("886".equals(msg)){
break;
}
}
ds.close();
}
}
public class UDPServer {
public static void main(String[] args) throws IOException {
DatagramSocket datagramSocket = new DatagramSocket(6666);
System.out.println("服务器已经开启,等待连接.....");
while (true){
byte[] bytes = new byte[1024];
DatagramPacket dp = new DatagramPacket(bytes, bytes.length);
datagramSocket.receive(dp);
byte[] data = dp.getData();
int length = dp.getLength();
String ip = dp.getAddress().getHostAddress();
String s = new String(data, 0, length);
System.out.println(ip+" 发来消息: "+s);
if ("886".equals(s)){
break;
}
}
datagramSocket.close();
}
}
public class Demo01Thread {
public static void main(String[] args) throws SocketException {
DatagramSocket server = new DatagramSocket(6666);
DatagramSocket client = new DatagramSocket();
new UDPServerThread(server).start();
new UDPClientThread(client).start();
}
}
public class UDPClientThread extends Thread{
DatagramSocket ds = null;
public UDPClientThread(DatagramSocket ds) {
this.ds = ds;
}
@Override
public void run() {
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
while (true){
System.out.println("请输入要发送的消息");
String msg = reader.readLine();
byte[] bytes = msg.getBytes();
DatagramPacket packet = new DatagramPacket(bytes, bytes.length, InetAddress.getByName("192.168.80.1"), 6666);
ds.send(packet);
if ("886".equals(msg)){
break;
}
}
ds.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public class UDPServerThread extends Thread{
DatagramSocket datagramSocket = null;
public UDPServerThread(DatagramSocket datagramSocket) {
this.datagramSocket = datagramSocket;
}
@Override
public void run() {
System.out.println("服务器已经开启,等待连接.....");
try {
while (true){
byte[] bytes = new byte[1024];
DatagramPacket dp = new DatagramPacket(bytes, bytes.length);
datagramSocket.receive(dp);
byte[] data = dp.getData();
int length = dp.getLength();
String ip = dp.getAddress().getHostAddress();
String s = new String(data, 0, length);
System.out.println(ip+" 发来消息: "+s);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
public class A {
public static void main(String[] args) throws IOException {
new Thread(){
@Override
public void run() {
try {
DatagramSocket datagramSocket = new DatagramSocket(6666);
System.out.println("A服务器已经开启,等待连接.....");
while (true){
byte[] bytes = new byte[1024];
DatagramPacket dp = new DatagramPacket(bytes, bytes.length);
datagramSocket.receive(dp);
byte[] data = dp.getData();
int length = dp.getLength();
String ip = dp.getAddress().getHostAddress();
String s = new String(data, 0, length);
System.out.println(ip+" B发来消息: "+s);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}.start();
sendMsg();
}
private static void sendMsg() throws IOException {
DatagramSocket ds = new DatagramSocket();
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
while (true){
System.out.println("请输入要发送B的消息");
String msg = reader.readLine();
byte[] bytes = msg.getBytes();
DatagramPacket packet = new DatagramPacket(bytes, bytes.length, InetAddress.getByName("192.168.80.1"), 8888);
ds.send(packet);
if ("886".equals(msg)){
break;
}
}
ds.close();
}
}
public class B {
public static void main(String[] args) throws IOException {
new Thread(){
@Override
public void run() {
try {
DatagramSocket datagramSocket = new DatagramSocket(8888);
System.out.println("B服务器已经开启,等待连接.....");
while (true){
byte[] bytes = new byte[1024];
DatagramPacket dp = new DatagramPacket(bytes, bytes.length);
datagramSocket.receive(dp);
byte[] data = dp.getData();
int length = dp.getLength();
String ip = dp.getAddress().getHostAddress();
String s = new String(data, 0, length);
System.out.println(ip+" A发来消息: "+s);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}.start();
sendMsg();
}
private static void sendMsg() throws IOException {
DatagramSocket ds = new DatagramSocket();
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
while (true){
System.out.println("请输入要发送A的消息");
String msg = reader.readLine();
byte[] bytes = msg.getBytes();
DatagramPacket packet = new DatagramPacket(bytes, bytes.length, InetAddress.getByName("192.168.80.1"), 6666);
ds.send(packet);
if ("886".equals(msg)){
break;
}
}
ds.close();
}
}
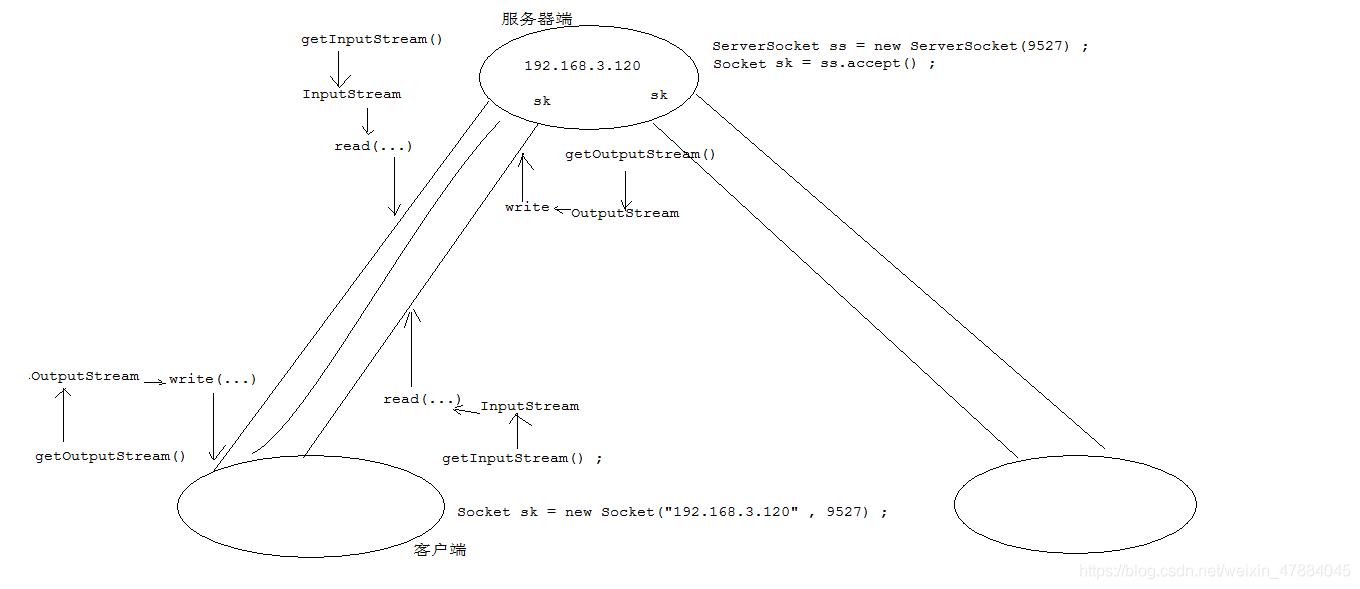
import java.io.IOException;
import java.io.OutputStream;
import java.net.Socket;
public class TCPClient {
public static void main(String[] args) throws IOException {
Socket sk = new Socket("192.168.80.1", 7777);
OutputStream out = sk.getOutputStream();
out.write("你好TCP我来了".getBytes());
sk.close();
}
}
import java.io.IOException;
import java.io.InputStream;
import java.net.ServerSocket;
import java.net.Socket;
public class TCPServer {
public static void main(String[] args) throws IOException {
ServerSocket ss = new ServerSocket(7777);
System.out.println("服务器已经开启,等待连接.....");
Socket sk = ss.accept();
InputStream in = sk.getInputStream();
byte[] bytes = new byte[1024];
int len = in.read(bytes);
String s = new String(bytes, 0, len);
System.out.println(s);
ss.close();
}
}
public class TCPClient {
public static void main(String[] args) throws IOException {
Socket socket = new Socket("192.168.80.1", 5555);
OutputStream out = socket.getOutputStream();
out.write("你好服务器,我来了".getBytes());
InputStream in = socket.getInputStream();
byte[] bytes = new byte[1024];
int len = in.read(bytes);
String msg = new String(bytes, 0, len);
System.out.println(msg);
socket.close();
}
}
public class TCPServer {
public static void main(String[] args) throws IOException {
ServerSocket ss = new ServerSocket(5555);
System.out.println("服务器已经开启,等待连接.....");
Socket sk = ss.accept();
InputStream in = sk.getInputStream();
byte[] bytes = new byte[1024];
int len = in.read(bytes);
String msg = new String(bytes, 0, len);
System.out.println(msg);
OutputStream out = sk.getOutputStream();
out.write("消息收到,放心".getBytes());
ss.close();
}
}
public class TCPClient {
public static void main(String[] args) throws IOException {
Socket socket = new Socket("192.168.80.1", 5555);
OutputStream out = socket.getOutputStream();
BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(out));
BufferedReader in = new BufferedReader(new InputStreamReader(System.in));
String line = null;
while ((line = in.readLine()) != null){
System.out.println("请输入消息:");
writer.write(line);
writer.newLine();
writer.flush();
if ("886".equals(line)){
break;
}
}
socket.close();
}
}
public class TCPServer {
public static void main(String[] args) throws IOException {
ServerSocket ss = new ServerSocket(5555);
System.out.println("服务器已经开启,等待连接.....");
Socket sk = ss.accept();
InputStream in = sk.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
String line = null;
BufferedWriter bw = new BufferedWriter(new FileWriter("msg.txt"));
String ip = sk.getInetAddress().getHostAddress();
while ((line = reader.readLine()) != null){
System.out.println(ip + "发来消息:" + line);
if ("886".equals(line)){
break;
}
bw.write(line);
bw.newLine();
bw.flush();
}
bw.close();
ss.close();
}
}
public class TCPClient {
public static void main(String[] args) throws IOException {
Socket socket = new Socket("192.168.80.1", 5555);
OutputStream out = socket.getOutputStream();
BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(out));
BufferedReader in = new BufferedReader(new FileReader("msg.txt"));
String line = null;
while ((line = in.readLine()) != null){
writer.write(line);
writer.newLine();
writer.flush();
}
socket.close();
}
}
public class TCPServer {
public static void main(String[] args) throws IOException {
ServerSocket ss = new ServerSocket(5555);
System.out.println("服务器已经开启,等待连接.....");
Socket sk = ss.accept();
InputStream in = sk.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
String line = null;
String ip = sk.getInetAddress().getHostAddress();
while ((line = reader.readLine()) != null){
System.out.println(ip + "发来消息:" + line);
}
ss.close();
}
}
public class TCPClient {
public static void main(String[] args) throws IOException {
Socket socket = new Socket("192.168.80.1", 5555);
OutputStream out = socket.getOutputStream();
BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(out));
BufferedReader in = new BufferedReader(new FileReader("msg.txt"));
String line = null;
while ((line = in.readLine()) != null){
writer.write(line);
writer.newLine();
writer.flush();
}
socket.shutdownOutput();
InputStream inputStream = socket.getInputStream();
byte[] bytes = new byte[1024];
int len = inputStream.read(bytes);
System.out.println(new String(bytes,0,len));
socket.close();
}
}
public class TCPServer {
public static void main(String[] args) throws IOException {
ServerSocket ss = new ServerSocket(5555);
System.out.println("服务器已经开启,等待连接.....");
Socket sk = ss.accept();
InputStream in = sk.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
String line = null;
BufferedWriter bw = new BufferedWriter(new FileWriter("msg22.txt"));
String ip = sk.getInetAddress().getHostAddress();
while ((line = reader.readLine()) != null){
bw.write(line);
bw.newLine();
bw.flush();
}
OutputStream outputStream = sk.getOutputStream();
outputStream.write("文件保存成功".getBytes());
bw.close();
ss.close();
}
}
|