1.计算机网络
如何实现网络通信?
通信双方地址
规则:协议通信的协议
http , ftp ,smtp , tcp , udp
TCP/IP 参考模型
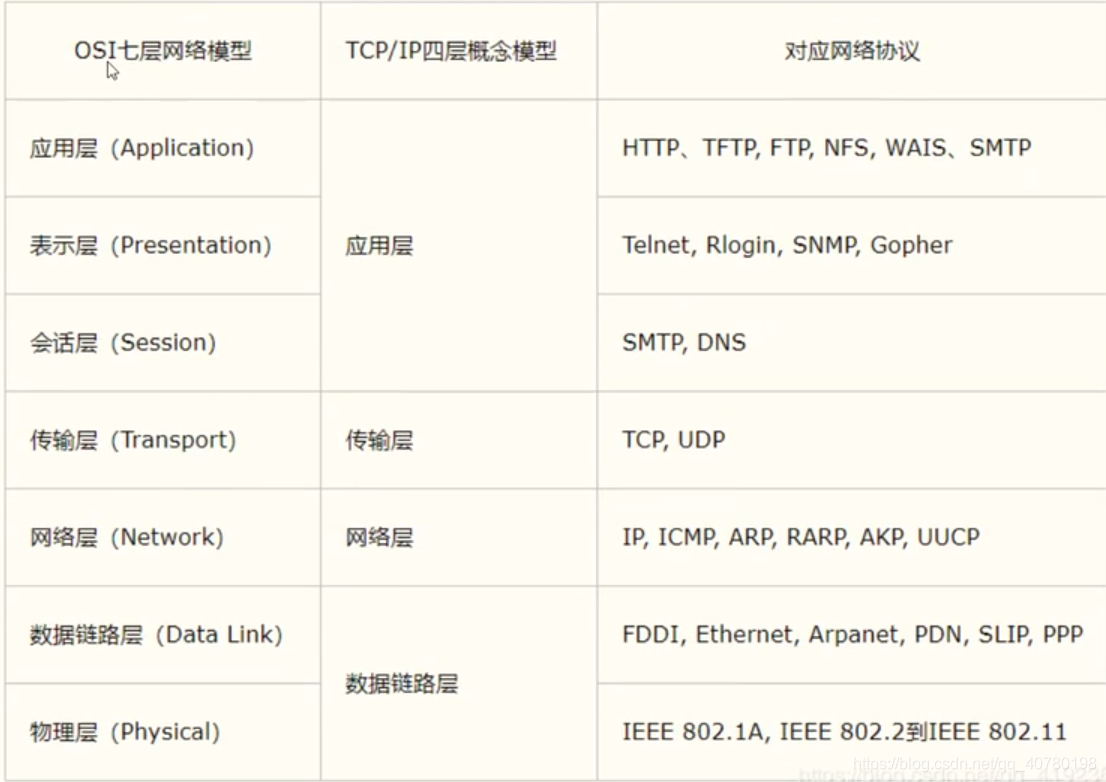
2.IP
专门表示Internet协议(IP)地址 : InetAdress.
ip地址 : InetAddress
//测试Ip
public class InetAddressDemo {
public static void main(String[] args) {
try {
//没什么用
//查询本机地址
InetAddress localHost = InetAddress.getLocalHost();
System.out.println(localHost);
//查询网络ip地址
InetAddress baiduIP = InetAddress.getByName("www.baidu.com");
System.out.println(baiduIP);
//常用方法
System.out.println("规范的名字:"+baiduIP.getCanonicalHostName()); //规范的名字
System.out.println("ip:"+baiduIP.getHostAddress()); //ip
System.out.println("域名:"+baiduIP.getHostName()); //域名
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
}
3.端口
端口表示计算机上的一个程序的进程:
public class InetSocketAddressDemo {
public static void main(String[] args) {
//没什么用
InetSocketAddress inetSocketAddress = new InetSocketAddress("127.0.0.1", 8080);
System.out.println(inetSocketAddress);
System.out.println(inetSocketAddress.getAddress());
System.out.println(inetSocketAddress.getHostName()); //host 文件
System.out.println(inetSocketAddress.getPort()); //端口
}
}
3.1 Dos命令
netstat -ano #查看所有的端口
netstat -ano|findstr "5900"
tasklist|findstr "13640" #查看指定端口的进程
4.通信协议
协议 :约定,就好比我们现在说的是普通话.
网络通信协议 : 速率,传输码率,代码结构,传输控制......
TCP/IP 协议簇 : 实际上是一组协议
重要:
-
TCP/IP : 用户传输协议 -
UDP : 用户数据报协议
出名的协议:
TCP UDP 对比
TCP :打电话
最少需要三次,保证稳定连接
A:你愁啥?
B:瞅你咋地?
A:干一场
A:我要走了!
B:你真的要走了吗?
B:你真的真的要走了吗?
A:我真的要走了!
UDP:发短信
-
不连接,不稳定 -
客户端,服务端,没有明确的接线 -
不管有没有准备好,都可以发给你 -
DDOS:洪水攻击!(饱和攻击)
5.TCP
客户端
1.连接服务器Socket
2.发送消息
服务器
1.建立服务的端口ServerSocket
2.等待用户的连接 accept
3.接受客户端的消息
Client:
//客户端
public class TcpClient1 {
public static void main(String[] args) {
Socket socket = null;
OutputStream os = null;
try {
//1.要知道服务器的地址,端口号
InetAddress serverIP =
InetAddress.getByName("127.0.0.1");
Integer port = 9999;
//2.创建一个socket连接
socket = new Socket(serverIP,port);
//3.发送消息 IO流
os = socket.getOutputStream();
os.write("你好,欢迎学习狂神说Java".getBytes());
} catch (Exception e) {
e.printStackTrace();
}finally {
if(os != null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket != null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
Server
public class TcpServer1 {
public static void main(String[] args) {
ServerSocket serverSocket = null;
Socket socket = null;
InputStream is = null;
ByteArrayOutputStream baos = null;
try {
//1.我得有一个地址
serverSocket = new ServerSocket(9999);
while(true){
//2.等待客户端连接过来
socket = serverSocket.accept();
//3.读取客户端的消息
is = socket.getInputStream();
//管道流
baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
Integer length;
while((length = is.read(buffer)) != -1){
baos.write(buffer,0,length);
}
System.out.println(baos.toString());
}
} catch (IOException e) {
e.printStackTrace();
}finally {
if(baos != null){
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(is != null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket != null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(serverSocket != null){
try {
serverSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
5.1文件上传
Server
//服务端
public class TcpServerDemo02 {
public static void main(String[] args) {
//1.创建服务
ServerSocket serverSocket = null;
Socket socket = null;
InputStream is = null;
FileOutputStream fos = null;
try {
//1.创建服务
serverSocket = new ServerSocket(9000);
//2.监听客户端的连接
socket = serverSocket.accept();
//3.获取输入流
is = socket.getInputStream();
//4.文件输出
fos = new FileOutputStream("receive.png");
byte[] buffer = new byte[1024];
Integer len;
while((len=is.read(buffer))!=-1){
fos.write(buffer,0,len);
}
//通知客户端接收完毕
OutputStream outputStream = socket.getOutputStream();
outputStream.write("123".getBytes());
} catch (IOException e) {
e.printStackTrace();
}finally {
if(fos != null){
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(is != null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket != null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(serverSocket != null){
try {
serverSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
Client
//客户端
public class TcpClientDemo02 {
public static void main(String[] args) {
//创建一个Socket连接
InetAddress inetAddress = null;
Socket socket = new Socket();
FileInputStream fis = null;
OutputStream outputStream = null;
ByteArrayOutputStream baos = null;
InputStream inputStream = null;
try {
//1.创建一个Socket连接
inetAddress = InetAddress.getByName("127.0.0.1");
socket = new Socket(inetAddress,9000);
//2.创建一个输出流
outputStream = socket.getOutputStream();
//3.读取文件流
fis = new FileInputStream(new File("D:\\bilibiliLesson\\NetProgram\\netProject\\src\\报名ER.png"));
//4.写出文件
byte[] buffer = new byte[1024];
Integer length;
while((length=fis.read(buffer))!=-1){
outputStream.write(buffer,0,length);
}
//通知服务器已经传输完了
socket.shutdownOutput(); //我已经传输完了
//5.确定服务器接收完毕,才能断开连接
inputStream = socket.getInputStream();
baos = new ByteArrayOutputStream();
byte[] buffer2 = new byte[1024];
Integer length2;
while((length2 = inputStream.read(buffer2))!=-1){
baos.write(buffer,0,length2);
}
System.out.println(baos.toString());
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
if(baos != null){
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(inputStream != null){
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fis!=null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(outputStream!=null){
try {
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket != null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
6.UDP
6.1阻塞接收
UDP1
//不需要连接服务器
public class UdpDemo1 {
public static void main(String[] args) {
DatagramSocket socket = null;
try {
//1.建立一个Socket
socket = new DatagramSocket(8080);
//2.建一个包
String message = "hello server";
InetAddress inetAddress = InetAddress.getByName("127.0.0.1");
Integer port = 9090;
//数据,数据的长度起始,要发送给谁
DatagramPacket packet = new DatagramPacket(message.getBytes(), 0, message.getBytes().length, inetAddress, port);
//发送包
socket.send(packet);
}
catch (SocketException e) {
e.printStackTrace();
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
finally {
if(socket != null){
socket.close();
}
}
}
}
UDP2
//不需要连接服务器
public class UdpDemo1 {
public static void main(String[] args) {
DatagramSocket socket = null;
try {
//1.建立一个Socket
socket = new DatagramSocket(8080);
//2.建一个包
String message = "hello server";
InetAddress inetAddress = InetAddress.getByName("127.0.0.1");
Integer port = 9090;
//数据,数据的长度起始,要发送给谁
DatagramPacket packet = new DatagramPacket(message.getBytes(), 0, message.getBytes().length, inetAddress, port);
//发送包
socket.send(packet);
}
catch (SocketException e) {
e.printStackTrace();
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
finally {
if(socket != null){
socket.close();
}
}
}
}
UDP2
//还是要等待客户端的连接
public class UdpDemo2 {
public static void main(String[] args) {
DatagramSocket socket = null;
try {
//开放端口
socket = new DatagramSocket(9090);
//接收数据包
byte[] buffer = new byte[1024];
DatagramPacket packet = new DatagramPacket(buffer,0,buffer.length);
socket.receive(packet); //阻塞接收
System.out.println(
new String( packet.getData(),0,packet.getLength()));
} catch (SocketException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
if(socket != null){
socket.close();
}
}
}
}
6.2 UDP Chat 单方发送接收
UdpChat1
public class UdpChat1 {
public static void main(String[] args) {
DatagramSocket socket = null;
try {
//socket
socket = new DatagramSocket(8888);
//准备数据 : 控制台读取 System.in
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
while(true){
String data = reader.readLine();
byte[] s1 = data.getBytes();
//ip
InetAddress inetAddress = InetAddress.getByName("127.0.0.1");
//向谁发送什么消息
DatagramPacket packet = new DatagramPacket(s1,0,s1.length,inetAddress,9999);
//发送
socket.send(packet);
if(data.equals("bye")){
break;
}
}
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (SocketException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
if(socket != null){
socket.close();
}
}
}
}
UdpChat2
public class UdpChat2 {
public static void main(String[] args) {
try {
DatagramSocket socket = new DatagramSocket(9999);
while(true){ //循环接收
//准备接收包裹
byte[] bytes = new byte[1024];
DatagramPacket packet = new DatagramPacket(bytes,0,bytes.length);
socket.receive(packet); //阻塞接收
//断开连接 bye
byte[] data = packet.getData();
String receiveData = new String(data,0,data.length);
System.out.println(receiveData);
if(receiveData.equals("bye")){
break;
}
}
} catch (SocketException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
7 Url 统一资源定位符
统一资源定位符: 定位资源的,定位资源的,定位互联网上的某一个资源
DNS域名解析 www.baidu.com 解析成 XXX.XXX.XXX.XXX
协议://ip地址:端口/项目名/资源
资源下载
public class UrlDownload {
public static void main(String[] args) throws MalformedURLException {
//1.下载地址
URL url = new URL("https://gimg2.baidu.com/image_search/src=http%3A%2F%2Fimg12.51tietu.net%2Fpic%2F2016-080609%2F201608060952210bwjqxkdu1l68137280x180.jpg");
HttpURLConnection urlConnection = null;
InputStream inputStream = null;
FileOutputStream fos = null;
try {
//2.连接到这个资源HTTP
urlConnection = (HttpURLConnection)url.openConnection();
inputStream = urlConnection.getInputStream();
fos = new FileOutputStream("girl.jpg");
byte[] buffer = new byte[1024];
Integer length;
while((length=inputStream.read(buffer)) != -1){
fos.write(buffer, 0, length);
}
} catch (IOException e) {
e.printStackTrace();
}finally {
if(fos != null){
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(inputStream != null){
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(urlConnection != null){
try {
urlConnection.connect();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
|