目的:使用tcp完成浏览器请求并作出对应响应 浏览器输入localhost:8888/login.html 或其他html返回对应的html页面 在返回的登录页面 输入账号 密码 点击登录 url格式如下 localhost:8888/login?username=zhangsan&password=123456 根据请求 判断账号密码是否为 账号zhangsan 密码123456 返回响应 登录成功 或 登录失败
1.准备了一个简易的登录页面login.html和favicon.ico图标 注意:请求的方式必须用get方法 不然该java代码无法获取你的url从而无法判断账户和密码
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>登录页面</title>
</head>
<body>
<form action="/login" method="get">
账户:<input type="text" name="username">
<br>
密码:<input type="password" name="password">
<br>
<input type ="submit" value = "登录">
</form>
</body>
</html>
百度搜索http://www.ico51.cn/把任意一个图片转换成favicon.ico图标
2.用java写的实现代码如下
public static void main(String[] args) throws Exception {
ServerSocket ss = new ServerSocket(8888);
while (true) {
Socket s = ss.accept();
InputStream is = s.getInputStream();
InputStreamReader isr = new InputStreamReader(is);
BufferedReader br = new BufferedReader(isr);
String readLine = br.readLine();
String[] split = readLine.split(" ");
String data = split[1];
String msg = "";
OutputStream os = s.getOutputStream();
os.write("HTTP/1.1 200 OK\n".getBytes());
os.write("Content-Type: text/html ;charset=utf-8\n".getBytes());
os.write("\r\n".getBytes());
if (!data.contains("?")) {
File path = new File("D:/eclipse/workspace/JavaWeb/src/com/yunhe/day0809");
File f = new File(path, data);
FileInputStream fis = null;
try {
fis = new FileInputStream(f);
byte b[] = new byte[1024];
int len = 0;
while ((len = fis.read(b)) != -1) {
os.write(b, 0, len);
}
} catch (FileNotFoundException e) {
os.write("你请求的页面不存在".getBytes());
System.out.println(data + "文件不存在");
} finally {
fis.close();
}
os.flush();
os.close();
} else {
String[] dataArr = data.split("\\?");
System.out.println("请求的服务地址为:" + dataArr[0]);
HashMap<String, String> parseSring = parseSring(dataArr[1]);
System.out.println("请求的数据为:" + parseSring);
if (dataArr[0].equals("/login")) {
if (parseSring.get("username").equals("zhangsan") && parseSring.get("password").equals("123456")) {
msg = "登录成功";
} else {
msg = "登录失败";
}
} else {
msg = "无法处理该请求";
}
}
os.write(msg.getBytes());
}
}
public static HashMap<String, String> parseSring(String str) {
HashMap<String, String> values = new HashMap<>();
String[] split = str.split("&");
for (String string : split) {
String[] split2 = string.split("=");
values.put(split2[0], split2[1]);
}
return values;
}
3.完成以上步骤 运行java代码
①百度搜索 localhost:8888/login.html 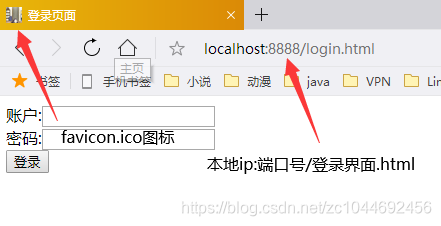 输入账户密码 账户:zhangsan 密码:123456 点击登录
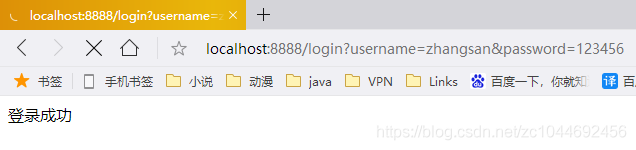 当账户或密码输入错误时 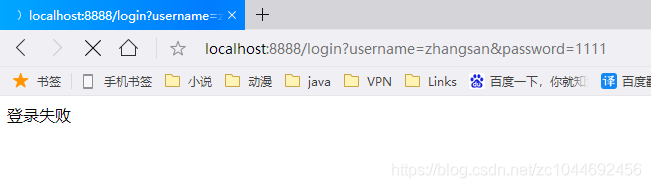 当查询不存在的logn.html文件时控制台会显示如下所示:  ②当直接查询 localhost:8888/login?username=zhangsan&password=123456 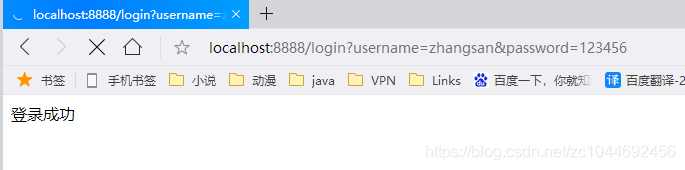
|