IP
ip地址:InetAddress 使用ipconfig查看IP地址
? 唯一定位一台网络上的计算机
? 192.168.0.6:本机的ip
? ip地址分类
? ipv4 / ipv6
? IPV4:192.168.0.6,四个字节组成。0~255,可生成42亿;30亿在北美,只有4亿在亚洲。在2011年就用尽
? IPV6:128位。8个无符号整数
? 公网(互联网)-私网(局域网)
? ABCD类地址
? A类IP地址范围1.0.0.1-126.255.255.254
? B类IP地址范围128.1.0.1-191.254.255.254
? C类IP地址范围192.0.1.1-223.255.254.254
? D类IP地址范围224.0.0.1-239.255.255.254
? 192.168.xx.xx专门给组织内部使用
? 域名:记忆问题
package com;
import java.net.InetAddress;
import java.net.UnknownHostException;
public class TestInetAddress {
public static void main(String[] args) {
try {
InetAddress inetAddress1 = InetAddress.getByName("192.168.0.6");
System.out.println(inetAddress1);
InetAddress inetAddress3 = InetAddress.getByName("localhost");
System.out.println(inetAddress3);
InetAddress inetAddress2 = InetAddress.getByName("www.baidu.com");
System.out.println(inetAddress2);
System.out.println(inetAddress2.getAddress());
System.out.println(inetAddress2.getCanonicalHostName());
System.out.println(inetAddress2.getHostAddress());
System.out.println(inetAddress2.getHostName());
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
}
端口
端口表示计算机上的一个程序的进程;
不同的进程有不同的端口号。用来区分软件
被规定0~65535
单个协议下,端口号不能冲突
端口号分类
? 公有端口号0~1023
? HTTP:80
? HTTPS:443
? FTP:21
? TELENT:23
? 程序注册端口号:1024~49151,分配给用户或程序的
? Tomcat:8080
? MySQL:3306
? Oracle:1521
? 动态,私有:49152~65535一般情况下我们不用
netstat -ano #查看所有的端口
netstat -ano|findstr "5900"#查看指定端口
tasklist|findstr "8696"#查看指定端口的进程
package com.meng.lessonip01;
import java.net.InetSocketAddress;
public class TestInetSocketAddress {
public static void main(String[] args) {
InetSocketAddress socketAddress1 = new InetSocketAddress("127.0.0.1", 8080);
InetSocketAddress socketAddress2 = new InetSocketAddress("localhost", 8080);
System.out.println(socketAddress1);
System.out.println(socketAddress2);
System.out.println(socketAddress1.getAddress());
System.out.println(socketAddress1.getHostName());
System.out.println(socketAddress1.getPort());
}
}
通信协议
协议:约定,双方进行交流的规定
网络通信协议:速率,传输码率,代码结构,传输控制。。。。
问题:非常的复杂
大事化小:分层
TCP/IP协议:实际是一种协议
重要:两者都是在传输层
? TCP:用户传输协议
? UDP:用户数据报协议
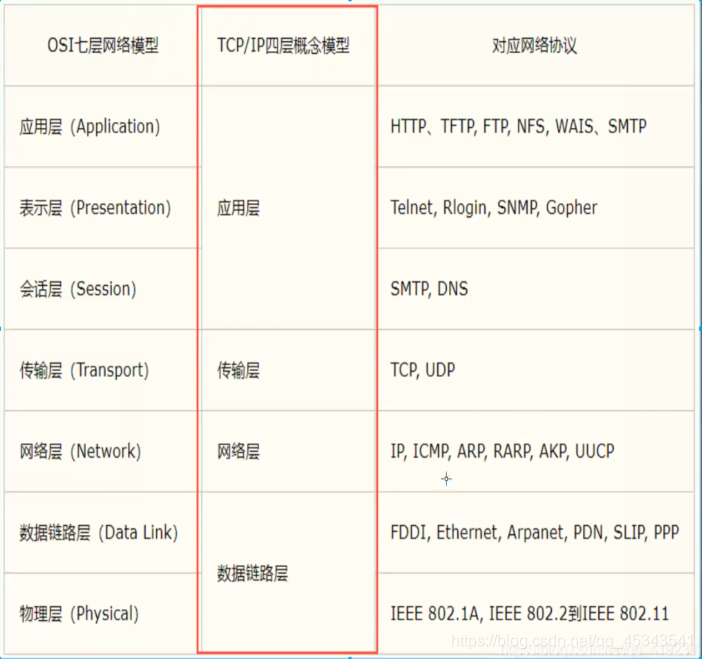
TCP UDP对比
TCP:打电话
? 连接稳定
? 三次握手(完成链接) 四次挥手(断开连接)
? 客户端 服务端
? 传输完成,释放连接,低效率
UDP:发短信
? 不连接不稳定
? 客户端服务端:没有明显的界限
? 不管有没有准备好都发给你
TCP
客户端
1.连接服务器Socket
2.发送消息
package com.meng.lesson02;
import java.io.IOException;
import java.io.OutputStream;
import java.net.InetAddress;
import java.net.Socket;
public class TcpClientDemo01 {
public static void main(String[] args) {
Socket socket =null;
OutputStream os =null;
try {
InetAddress serverIP = InetAddress.getByName("127.0.0.1");
int port = 9999;
socket = new Socket(serverIP,port);
os = socket.getOutputStream();
os.write("你好".getBytes());
} catch (Exception e) {
e.printStackTrace();
}finally {
if (os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
服务器
1.建立服务器的端口ServerSocket
2.等待用户的连接accept
3.接受用户的消息
package com.meng.lesson02;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.ServerSocket;
import java.net.Socket;
public class TcpServerDemo01 {
public static void main(String[] args) {
ServerSocket serverSocket = null;
Socket socket =null;
InputStream is =null;
ByteArrayOutputStream baos =null;
try {
serverSocket = new ServerSocket(9999);
socket = serverSocket.accept();
is = socket.getInputStream();
baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len=is.read(buffer))!=-1){
baos.write(buffer,0,len);
System.out.println(baos.toString());
}
} catch (IOException e) {
e.printStackTrace();
}finally {
if (baos!=null){
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (serverSocket!=null){
try {
serverSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
文件上传
服务器端
package com.meng.lesson02;
import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;
public class TcpServerDemo02 {
public static void main(String[] args) throws Exception {
ServerSocket serverSocket = new ServerSocket(9000);
Socket socket = serverSocket.accept();
InputStream is = socket.getInputStream();
FileOutputStream fos = new FileOutputStream(new File("receive.jpg"));
byte[] buffer = new byte[1024];
int len;
while ((len=is.read(buffer))!=-1){
fos.write(buffer,0,len);
}
OutputStream os = socket.getOutputStream();
os.write("我接收完了,你可以关闭了".getBytes());
fos.close();
is.close();
socket.close();
serverSocket.close();
}
}
客户端
package com.meng.lesson02;
import java.io.*;
import java.net.InetAddress;
import java.net.Socket;
public class TcpClientDemo02 {
public static void main(String[] args) throws Exception {
Socket socket = new Socket(InetAddress.getByName("127.0.0.1"), 9000);
OutputStream os = socket.getOutputStream();
FileInputStream fis = new FileInputStream(new File("mjlh.jpg"));
byte[] buffer = new byte[1024];
int len;
while ((len=fis.read(buffer))!=-1){
os.write(buffer,0,len);
}
socket.shutdownOutput();
InputStream inputStream = socket.getInputStream();
ByteArrayOutputStream bos = new ByteArrayOutputStream();
byte[] buffer2 = new byte[1024];
int len2;
while ((len2=inputStream.read(buffer2))!=-1){
bos.write(buffer2,0,len2);
}
System.out.println(bos.toString());
bos.close();
inputStream.close();
fis.close();
os.close();
socket.close();
}
}
Tomcat
服务端
? 自定义S
? Tomcat服务器S
客户端
? 自定义C
? 浏览器B
UDP
发短信:不用链接,需要知道对方地址
发送消息
发送端
package com.meng.lesson03;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
import java.net.SocketException;
public class UdpClientDemo01 {
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket();
String msg = "你好服务器";
InetAddress localhost = InetAddress.getByName("localhost");
int port=9090;
DatagramPacket packet = new DatagramPacket(msg.getBytes(), 0, msg.getBytes().length, localhost, port);
socket.send(packet);
socket.close();
}
}
接收端
package com.meng.lesson03;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.SocketException;
public class UdpServerDemo01 {
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket(9090);
byte[] buffer = new byte[1024];
DatagramPacket packet = new DatagramPacket(buffer, 0, buffer.length);
socket.receive(packet);
System.out.println(packet.getAddress().getHostAddress());
System.out.println(new String(packet.getData(),0, packet.getLength()));
socket.close();
}
}
咨询
循环发送消息
package com.meng.chat;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetSocketAddress;
public class UdpSenderDemo01 {
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket(8888);
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
while (true){
String data = reader.readLine();
byte[] datas = data.getBytes();
DatagramPacket packet = new DatagramPacket(datas, 0, data.length(), new InetSocketAddress("localhost", 6666));
socket.send(packet);
if (data.equals("bye")){
break;
}
}
socket.close();
}
}
循环接收消息
package com.meng.chat;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
public class UdpReceiveDemo01 {
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket(6666);
while (true){
byte[] container = new byte[1024];
DatagramPacket packet = new DatagramPacket(container, 0, container.length);
socket.receive(packet);
byte[] data = packet.getData();
String receiverData = new String(data, 0, data.length);
System.out.println(receiverData);
if (receiverData.equals("bye")){
break;
}
}
socket.close();
}
}
在线咨询:双方都是接收方也是发送方
package com.meng.chat;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetSocketAddress;
import java.net.SocketException;
public class TalkSend implements Runnable{
DatagramSocket socket = null;
BufferedReader reader = null;
private int fromPort;
private String toIP;
private int toPort;
public TalkSend(int fromPort, String toIP, int toPort) {
this.fromPort = fromPort;
this.toIP = toIP;
this.toPort = toPort;
try {
socket = new DatagramSocket(fromPort);
reader = new BufferedReader(new InputStreamReader(System.in));
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void run() {
while (true){
try {
String data = reader.readLine();
byte[] datas = data.getBytes();
DatagramPacket packet = new DatagramPacket(datas, 0, datas.length, new InetSocketAddress(this.toIP, this.toPort));
socket.send(packet);
if (data.equals("bye")){
break;
}
} catch (Exception e) {
e.printStackTrace();
}
}
socket.close();
}
}
package com.meng.chat;
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.SocketException;
public class TalkReceive implements Runnable{
DatagramSocket socket = null;
private int port;
private String msgFrom;
public TalkReceive(int port,String msgFrom) {
this.port = port;
this.msgFrom=msgFrom;
try {
socket = new DatagramSocket(port);
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void run() {
while (true){
try {
byte[] container = new byte[1024];
DatagramPacket packet = new DatagramPacket(container, 0, container.length);
socket.receive(packet);
byte[] data = packet.getData();
String receiverData = new String(data, 0, data.length);
System.out.println(msgFrom+":"+receiverData);
if (receiverData.equals("bye")){
break;
}
} catch (Exception e) {
e.printStackTrace();
}
}
socket.close();
}
}
package com.meng.chat;
public class TalkStudent {
public static void main(String[] args) {
new Thread(new TalkSend(7777,"localhost",9999)).start();
new Thread(new TalkReceive(8888,"老师")).start();
}
}
package com.meng.chat;
public class TalkTeacher {
public static void main(String[] args) {
new Thread(new TalkSend(5555,"localhost",8888)).start();
new Thread(new TalkReceive(9999,"学生")).start();
}
}
URL
统一资源定位符:定位资源的,定位互联网上的某一个资源
DNS域名解析
协议:
package com.meng.lesson04;
import java.net.MalformedURLException;
import java.net.URL;
public class URLDemo01 {
public static void main(String[] args) throws MalformedURLException {
URL url = new URL("http://localhost:8080/helloworld/index.jsp?username=mjlh&password=111");
System.out.println(url.getProtocol());
System.out.println(url.getHost());
System.out.println(url.getPort());
System.out.println(url.getPath());
System.out.println(url.getFile());
System.out.println(url.getQuery());
}
}
网上的某一个资源
DNS域名解析
协议:
package com.meng.lesson04;
import java.net.MalformedURLException;
import java.net.URL;
public class URLDemo01 {
public static void main(String[] args) throws MalformedURLException {
URL url = new URL("http://localhost:8080/helloworld/index.jsp?username=mjlh&password=111");
System.out.println(url.getProtocol());
System.out.println(url.getHost());
System.out.println(url.getPort());
System.out.println(url.getPath());
System.out.println(url.getFile());
System.out.println(url.getQuery());
}
}
|