1.1、概述
**地球村:**你在西安,你一个美国的朋友!
信件: 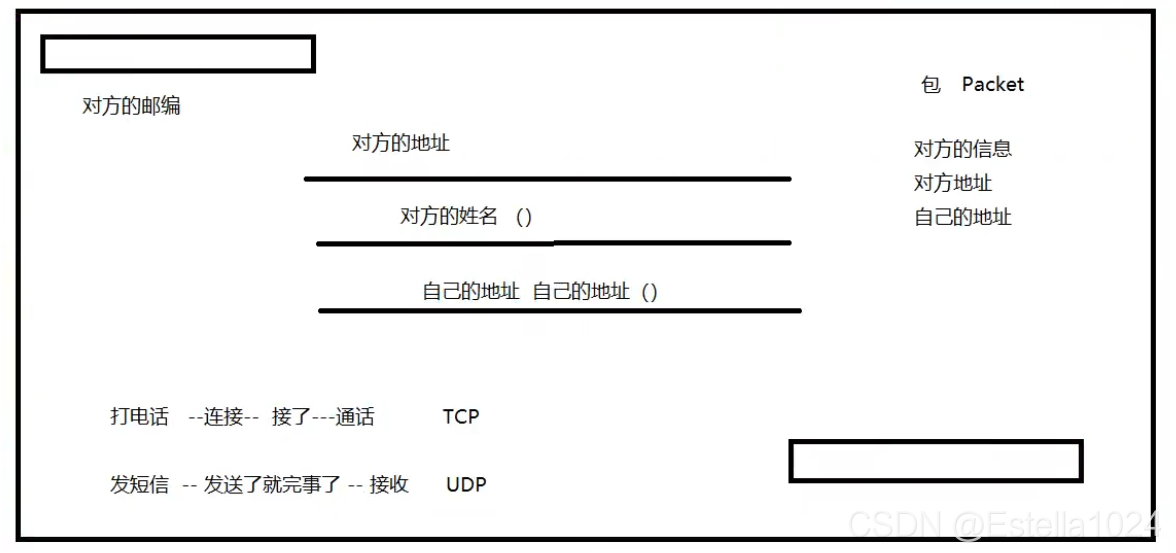
计算机网络:
计算机网络是指将地理位置不同的具有独立功能的多台计算机及其外部设备,通过通信线路连接起来,在网络操作系统,网络管理软件及网络通信协议的管理和协调下,实现资源共享和信息传递的计算机系统。
网络编程的目的:
无线电台…传播交流信息,数据交换。通信
想要达到这个效果需要什么:
- 如何准确地定位网络上的一台主机 192.168.16.124:端口,定位到这个计算机上的某个资源
- 找到了这个主机,如何传输数据呢?
javaweb:网页编程 B/S
网络编程:TCP/IP C/S
1.2、网络通信的要素
人工智能:智能汽车:工厂,人少!? 伦理:
如何实现网络的通信?
通信双方地址:
- ip
- 端口号
- 192.168.16.124:5900(可以定位到一台计算机)
规则:网络通信的协议
TCP/IP参考模型: 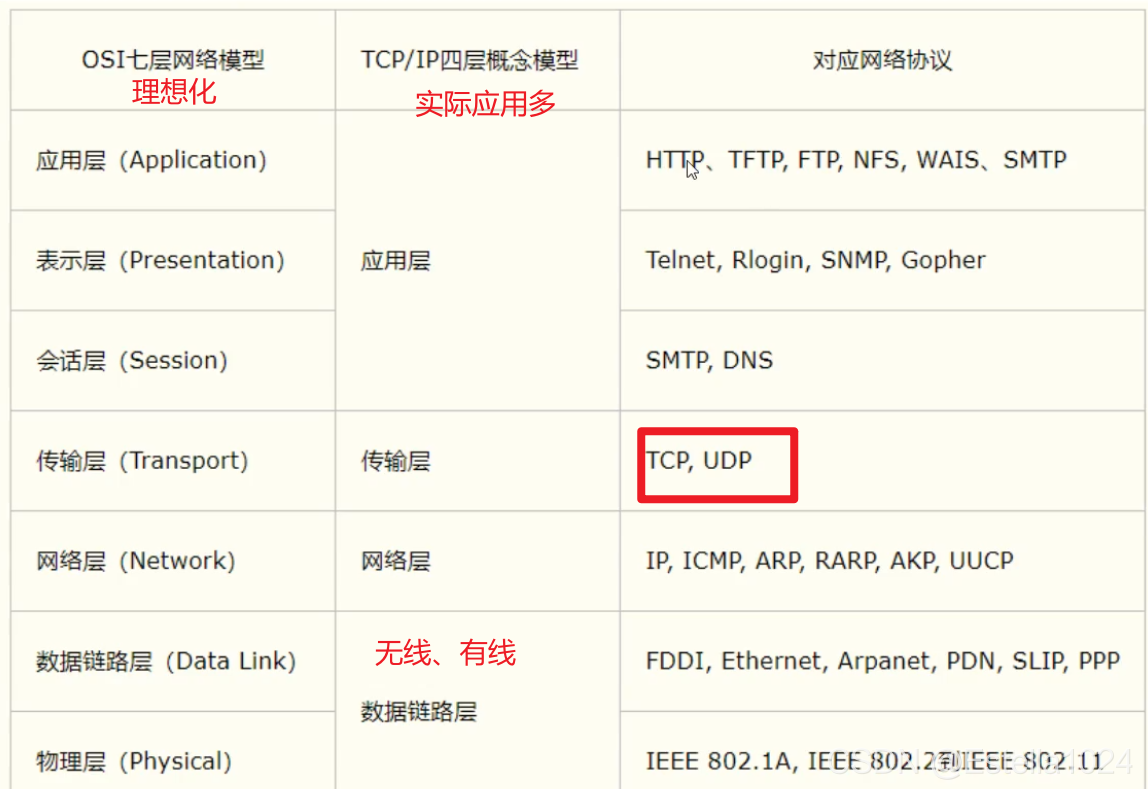
 小结:
- 网络编程中主要有两个问题
-
如何准确的定位到网络上的一台或者多台主机 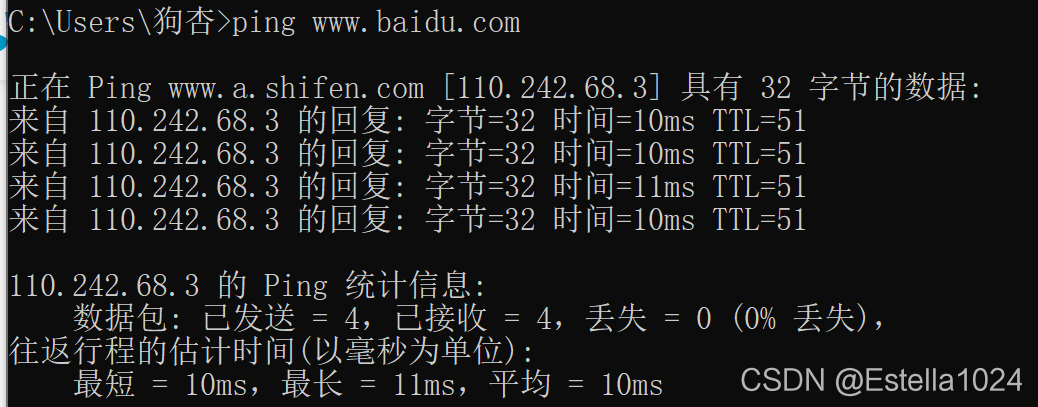 -
找到主机之后如何进行通信
- 网络编程中的要素
- 万物皆对象
1.3、IP
ip地址:inetAddress
2001:0bb2:aaaa:0015:0000:0000:1aaa:1312
- IP地址分类
- 公网(互联网 42亿)-私网(局域网)
- ABCD类地址
- 192.168.xx.xx,专门给组织内部使用的(一般是局域网)
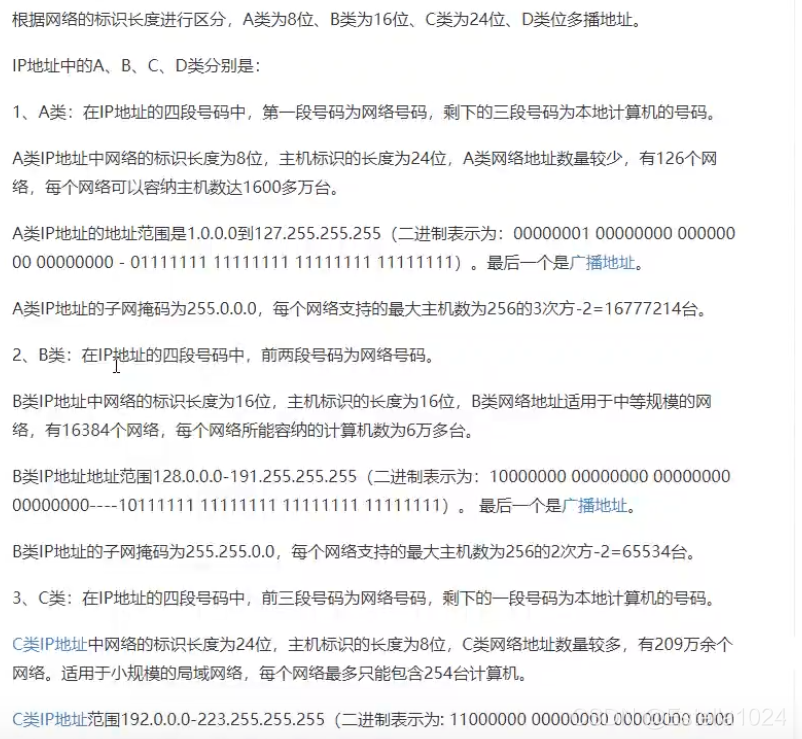 

public class TestInetAddress {
public static void main(String[] args) {
try {
InetAddress inetAddress1 = InetAddress.getByName("127.0.0.1");
System.out.println(inetAddress1);
InetAddress inetAddress3 = InetAddress.getByName("localhost");
System.out.println(inetAddress3);
InetAddress inetAddress4 = InetAddress.getLocalHost();
System.out.println(inetAddress4);
InetAddress inetAddress2 = InetAddress.getByName("www.bilibili.com");
System.out.println(inetAddress2);
System.out.println(inetAddress2.getCanonicalHostName());
System.out.println(inetAddress2.getHostAddress());
System.out.println(inetAddress2.getHostName());
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
}
1.4、端口
端口表示计算机上的一个程序的进程;
- 不同的进程有不同的端口号!用来区分软件!
- 被规定0 ~ 065535
- TCP,UDP:65535 * 2 ,单个协议下,端口号不能冲突(比如:tcp:80,udp:80 不行)
- 端口分类
- 公有端口0 ~ 1023(尽量不要占用)
- HTTP:80
- HTTPS:443
- FTP:21
- SSH:22 (远程连接)
- Telent:23
- 程序注册端口:1024 ~ 49151,分配给用户或者程序
- Tomcat:8080
- MySQL:3306
- Oracle:1521
- 动态、私有:49152 ~ 65535(不建议放)
netstat -ano #查看所有的端口
netstat -ano | finstr "5900" #查看指定的端口
tasklist | findstr "8696" #查看指定端口的进程
ctrl + shift + ESC #打开任务管理器
package kuangshen.socket;
import java.net.InetSocketAddress;
public class TestInetSocketAddress {
public static void main(String[] args) {
InetSocketAddress socketAddress1 = new InetSocketAddress("127.0.0.1", 8080);
System.out.println(socketAddress1);
InetSocketAddress socketAddress2 = new InetSocketAddress("localhost", 8080);
System.out.println(socketAddress2);
System.out.println(socketAddress1.getAddress());
System.out.println(socketAddress1.getHostName());
System.out.println(socketAddress1.getPort());
}
}
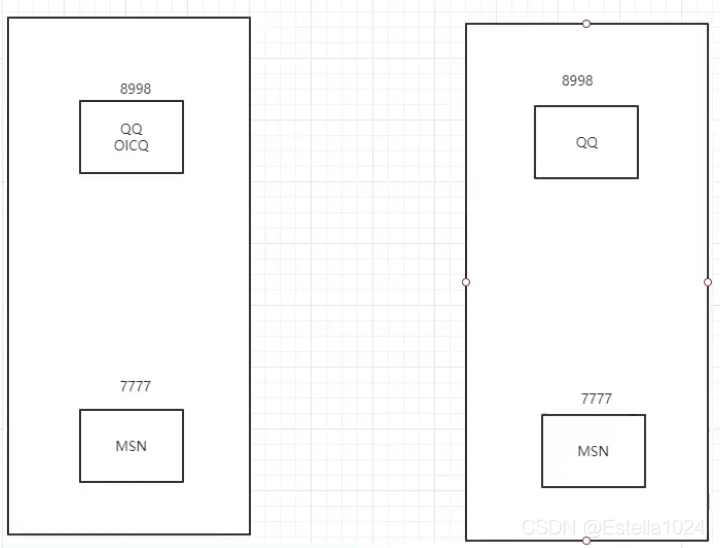
1.5、通信协议
协议:约定,就好比我们现在说的是普通话。
网络通信协议:速率,传输码率,代码结构,传输控制……
问题:非常的复杂?
大事化小:分层!
重要:
- TCP:用户传输协议(用户交流数据用的)
- UDP:用户数据报协议(好比写邮件和发短信,发出去就不管了)
出名的协议:
- TCP:用户传输协议
- IP:网络互连协议
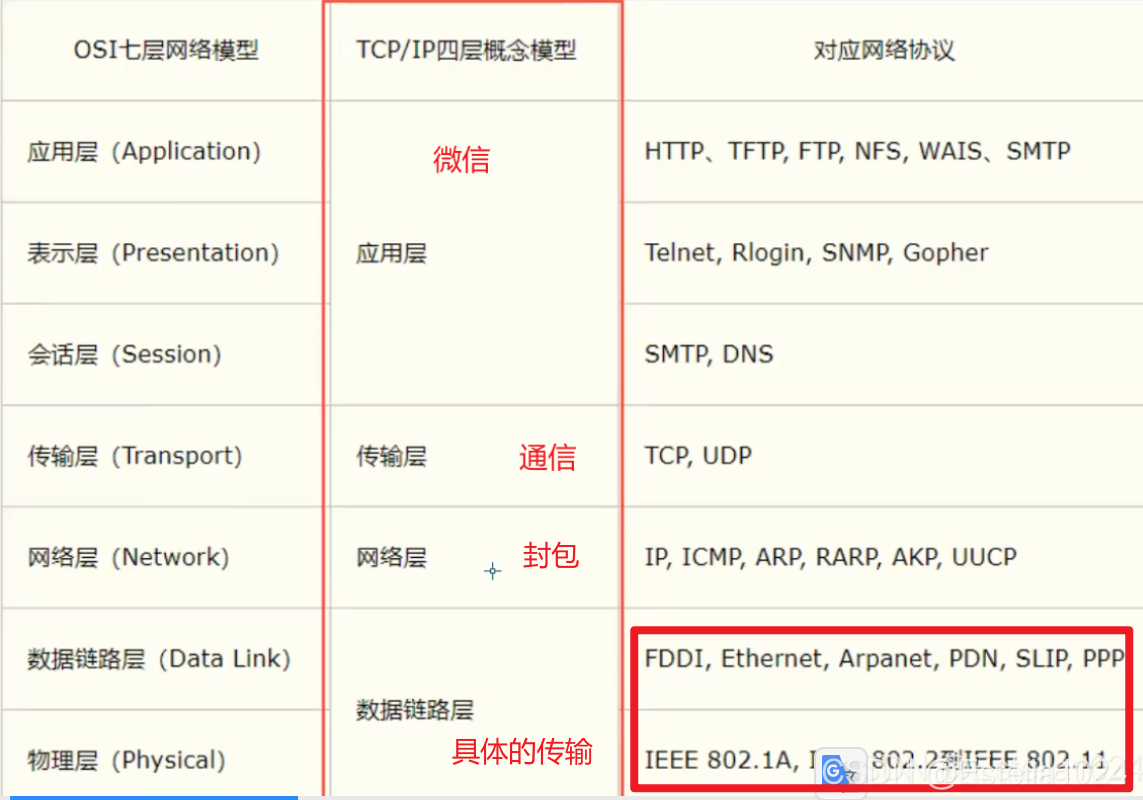
TCP UDP对比
TCP:打电话
最少需要三次,保证稳定连接!
A:你瞅啥?
B:瞅你咋地?
A:干一场!
A:我要走了
B:你真的要走了吗?
B:你真的真的要走了吗?
A:我真的要走了
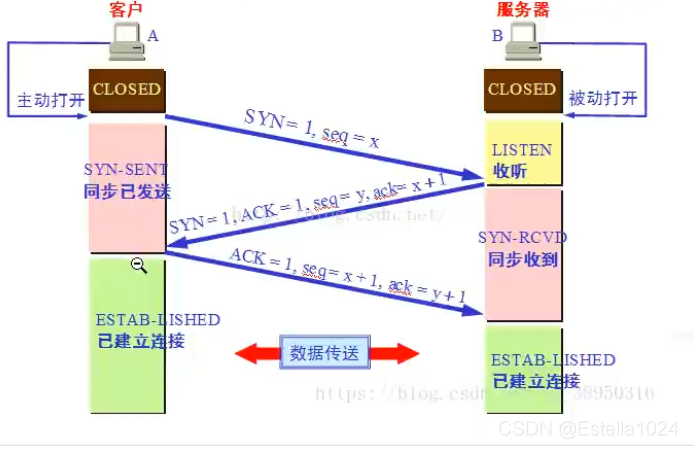
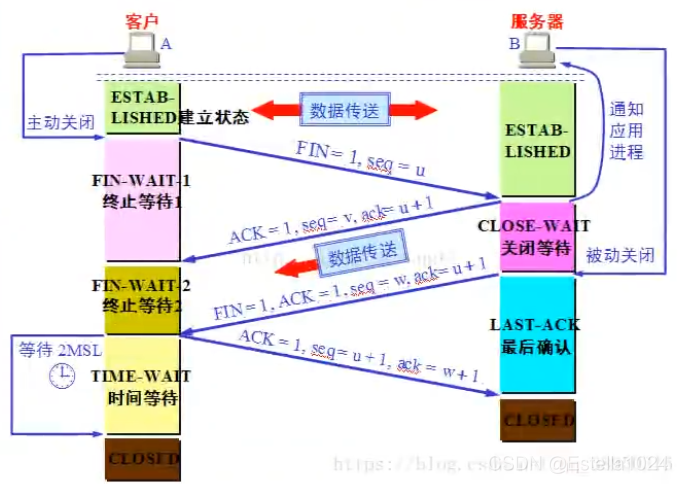
UDP:发短信
- 不连接,不稳定
- 客户端、服务端:没有明确的界限
- 不管有没有准备好,都可以发给你…
- 导弹攻击
- DDOS:洪水攻击!(饱和攻击)–>会造成端口堵塞
(DDOS:分布式拒绝服务器)
1.6、TCP
客户端
- 连接服务器Socket
- 发送消息
public class tcpClientDemo01 {
public static void main(String[] args) {
Socket socket = null;
OutputStream os = null;
try {
InetAddress serverIP = InetAddress.getByName("127.0.0.1");
int port = 9999;
socket = new Socket(serverIP, port);
os = socket.getOutputStream();
os.write("Hello World".getBytes());
} catch (Exception e) {
e.printStackTrace();
}finally {
if (socket != null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (os != null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
服务器
- 建立服务的端口ServerScoket
- 等待用户的连接accept
- 接收用户的消息
public class tcpServerDemo01 {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = null;
Socket socket = null;
InputStream is = null;
ByteArrayOutputStream baos = null;
try {
serverSocket = new ServerSocket(9999);
socket = serverSocket.accept();
is = socket.getInputStream();
baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = is.read(buffer)) != -1){
baos.write(buffer, 0, len);
}
System.out.println(baos.toString());
} catch (IOException e) {
e.printStackTrace();
}finally {
if (baos != null){
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (is != null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket != null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (serverSocket != null){
serverSocket.close();
}
}
}
}
文件上传
Tomcat
1.7、UDP
发送消息
循环发送消息
1.8、URL
|