1.WebSocketServer
package com.south.websocket;
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class WebSocketServer {
public static void main(String[] args) throws InterruptedException {
EventLoopGroup mainGroup=new NioEventLoopGroup();
EventLoopGroup subGroup=new NioEventLoopGroup();
try{
ServerBootstrap server=new ServerBootstrap();
server.group(mainGroup,subGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new WSServerInitialzer());
ChannelFuture future = server.bind(8888).sync();
future.channel().closeFuture().sync();
}finally {
mainGroup.shutdownGracefully();
subGroup.shutdownGracefully();
}
}
}
2.WSServerInitialzer
package com.south.websocket;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.socket.SocketChannel;
import io.netty.handler.codec.http.HttpObjectAggregator;
import io.netty.handler.codec.http.HttpServerCodec;
import io.netty.handler.codec.http.websocketx.WebSocketClientProtocolHandler;
import io.netty.handler.codec.http.websocketx.WebSocketServerProtocolHandler;
import io.netty.handler.stream.ChunkedWriteHandler;
public class WSServerInitialzer extends ChannelInitializer<SocketChannel> {
protected void initChannel(SocketChannel channel) throws Exception {
ChannelPipeline pipeline = channel.pipeline();
pipeline.addLast(new HttpServerCodec());
pipeline.addLast(new ChunkedWriteHandler());
pipeline.addLast(new HttpObjectAggregator(1024*64));
pipeline.addLast(new WebSocketServerProtocolHandler("/websocket"));
pipeline.addLast(new ChatHandler());
}
}
3.ChatHandler
package com.south.websocket;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.channel.group.ChannelGroup;
import io.netty.channel.group.DefaultChannelGroup;
import io.netty.handler.codec.http.websocketx.TextWebSocketFrame;
import io.netty.util.concurrent.GlobalEventExecutor;
import java.time.LocalDateTime;
public class ChatHandler extends SimpleChannelInboundHandler<TextWebSocketFrame> {
private static ChannelGroup clients=new DefaultChannelGroup(GlobalEventExecutor.INSTANCE);
protected void channelRead0(ChannelHandlerContext ctx, TextWebSocketFrame msg) throws Exception {
String content = msg.text();
System.out.println("接收到的数据:"+content);
clients.writeAndFlush(
new TextWebSocketFrame(
"[服务器在:]"+ LocalDateTime.now()
+"接收到消息,消息内容为:"+content
)
);
}
@Override
public void handlerAdded(ChannelHandlerContext ctx) throws Exception {
clients.add(ctx.channel());
}
@Override
public void handlerRemoved(ChannelHandlerContext ctx) throws Exception {
System.out.println("客户端断开,channel对应的长ID为:"+ctx.channel().id().asLongText());
System.out.println("客户端断开,channel对应的短ID为:"+ctx.channel().id().asShortText());
}
}
1.WebSocket Api
初始化对象
var aWebSocket = new WebSocket(url [, protocols]);
生命周期
aWebSocket.onopen = function(event) {
console.log("WebSocket is open now.");
};
aWebSocket.onmessage = function(event) {
console.debug("WebSocket message received:", event);
};
WebSocket.onclose = function(event) {
console.log("WebSocket is closed now.");
};
WebSocket.onerror = function(event) {
console.error("WebSocket error observed:", event);
};
主动方法
WebSocket.send("Hello server!");
WebSocket.close();
2.具体实现
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Netty 实时通讯</title>
</head>
<script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script>
<script>
var socket;
function openSocket() {
if(typeof(WebSocket) == "undefined") {
console.log("您的浏览器不支持WebSocket");
}else{
console.log("您的浏览器支持WebSocket");
var socketUrl="http://localhost:8888/websocket";
socketUrl=socketUrl.replace("https","ws").replace("http","ws");
console.log(socketUrl)
socket = new WebSocket(socketUrl);
socket.onopen = function() {
console.log("websocket已打开");
};
socket.onmessage = function(msg) {
console.log("接收消息"+msg.data);
var receiveMsg=$("#receiveMsg");
var html=receiveMsg.html();
receiveMsg.html(html+"<br/>"+msg.data);
};
socket.onclose = function() {
console.log("websocket已关闭");
};
socket.onerror = function() {
console.log("websocket发生了错误");
}
}
}
function sendMessage() {
if(typeof(WebSocket) == "undefined") {
console.log("您的浏览器不支持WebSocket");
}else {
console.log("您的浏览器支持WebSocket");
var msgContent=$("#msgContent").val();
socket.send(msgContent);
}
}
$(function(){
openSocket();
})
</script>
<body>
发送消息:
<input type="text" id="msgContent">
<input type="button" value="发送消息" onclick="sendMessage()">
<hr>
接收消息:
<div id="receiveMsg" ></div>
</body>
</html>
三:效果图展示
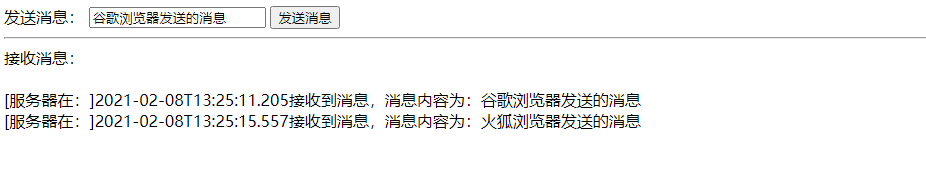
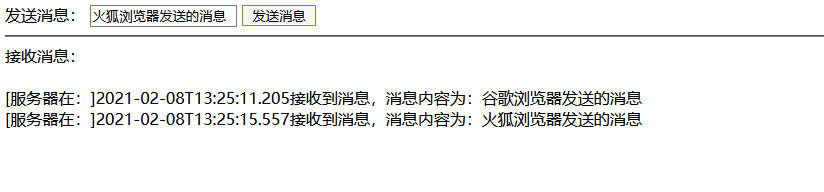