网络编程入门
软件结构
C/S结构:全称为Client/Server结构,是指客户端和服务端结构。常见的程序有QQ、迅雷等软件。 B/S结构:全称为Browser/Server结构,是指浏览器和服务器结构。常见的有谷歌、火狐等软件。 这两种架构各有优势,但无论哪种架构,都离不开网络的支持。
网络编程:就是在一定的协议下,实现两台计算机的通信的程序。
网络通信协议
udp:面向无连接的协议,通信的双方不用建立连接,可以直接发送数据。 好处:效率高 弊端:不安全,容易丢失数据 tcp:面向连接的协议,客户端和服务器必须经过3次握手才能建立逻辑连接,才能通信。 好处:安全 弊端:效率低
ip地址
IP地址:就相当于计算机的身份证号(唯一)
ip地址的分类
ipv4:ip地址由4个字节组成,一个字节是8位(1,0)
二进制:11001010.11000000.11001000.11111010
十进制:192.168.31.237
每个字节的范围:0-255,ip地址第一位是不能为0
数量:42亿
2^32=4294967296个
问题:随着人的增多,计算机的增多(2011年2月分配完毕),ip地址就会面临枯竭(不够),就出现了ipv6
ipv6:ipd地址由16个字节组成
数量:
2^128=3.4028236692093846346337460743177e+38
3402000000000000000000000000000000000000000000000000000000000000000000000
号称可以为地球上每一粒沙子编写一个ip地址
十六进制:fe80::a8a6:b83c:8b8b:2685%18
常用的dos命令:dos窗口
ipconfig:Windows IP 配置
无线局域网适配器 WLAN:
连接特定的 DNS 后缀 . . . . . . . :
本地链接 IPv6 地址. . . . . . . . : fe80::a8a6:b83c:8b8b:2685%18
IPv4 地址 . . . . . . . . . . . . : 192.168.31.237
子网掩码 . . . . . . . . . . . . : 255.255.255.0
默认网关. . . . . . . . . . . . . : 192.168.31.1
ping ip地址:测试你的电脑和指ip地址的电脑是否可以连通
ping 空格 IP地址
ping 220.181.57.216 ping指定的ip地址
ping www.baidu.com ping域名
ping 本机IP地址:127.0.0.1、localhost
端口号
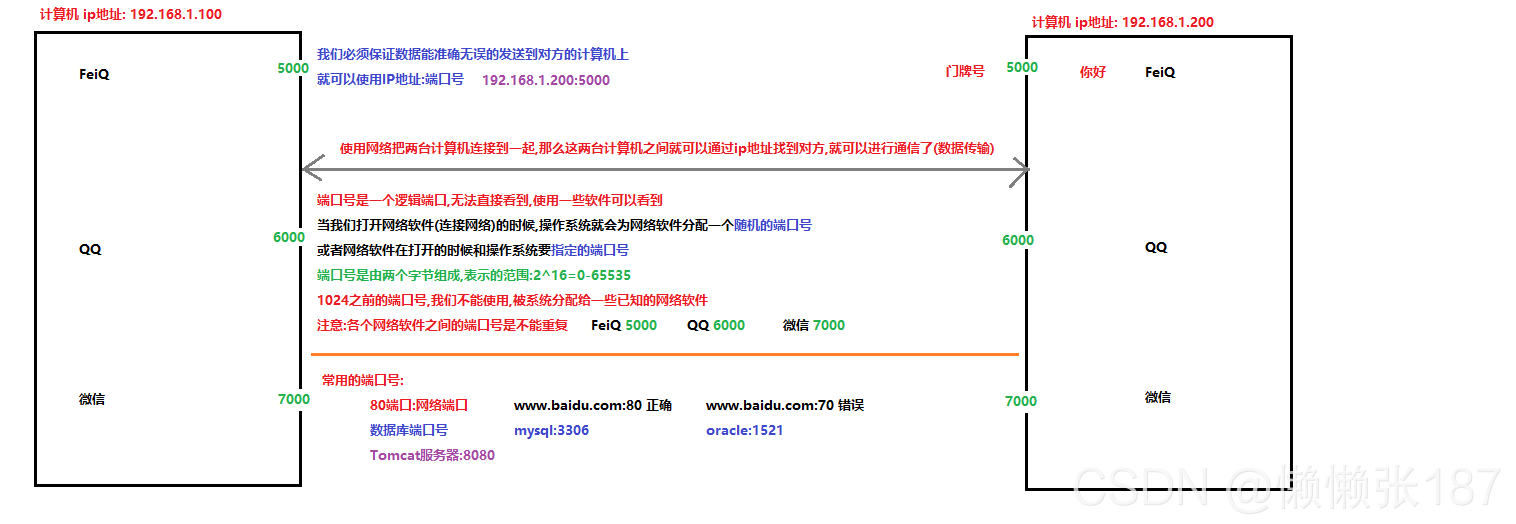
InetAddress类
public class Demo01InetAddress {
public static void main(String[] args) throws UnknownHostException {
show02();
}
private static void show02() throws UnknownHostException {
InetAddress inet = InetAddress.getByName("www.aiqiyi.com");
System.out.println(inet.getHostName());
System.out.println(inet.getHostAddress());
System.out.println(inet);
}
private static void show01() throws UnknownHostException {
InetAddress inet = InetAddress.getLocalHost();
System.out.println(inet);
String[] arr = inet.toString().split("/");
String name = arr[0];
String ip = arr[1];
System.out.println(name);
System.out.println(ip);
System.out.println("--------------------");
String hostName = inet.getHostName();
System.out.println(hostName);
String hostAddress = inet.getHostAddress();
System.out.println(hostAddress);
}
}
TCP通信程序
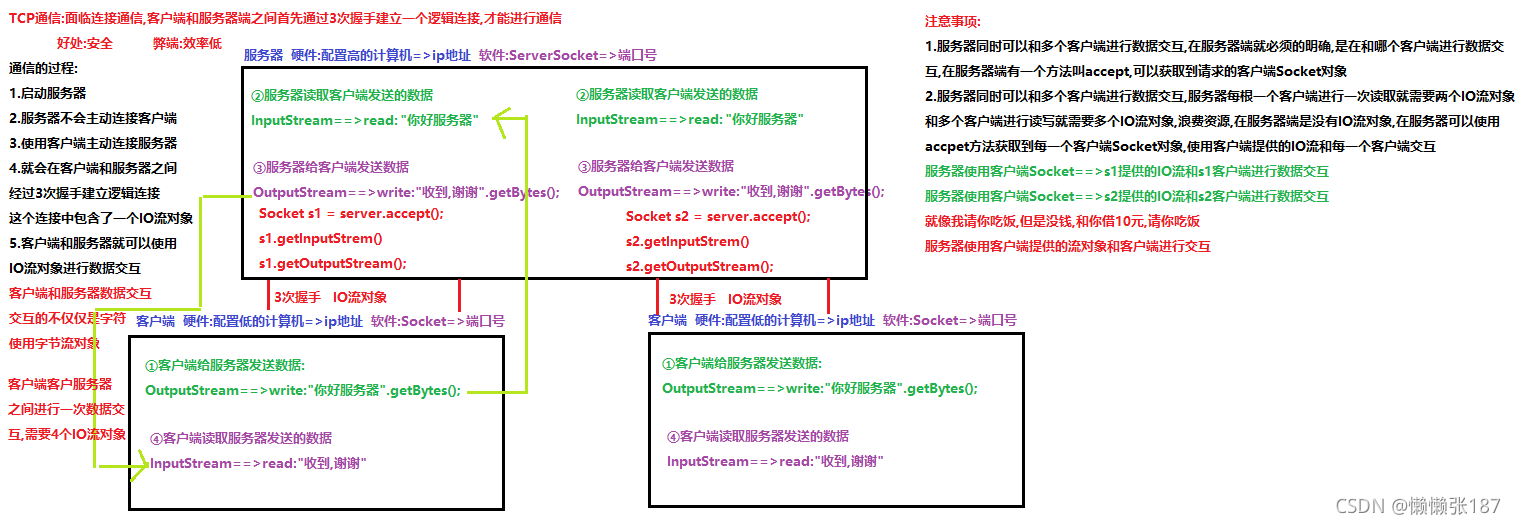
TCP通信客户端(重点)
作用:主动和服务器经过3次握手建立连接,给服务器发送数据,接收服务器回写的数据
public class Demo01TCPClient {
public static void main(String[] args) throws IOException {
Socket socket = new Socket("127.0.0.1",8888);
OutputStream os = socket.getOutputStream();
os.write("你好服务器".getBytes());
InputStream is = socket.getInputStream();
byte[] bytes = new byte[1024];
int len = is.read(bytes);
System.out.println(new String(bytes,0,len));
socket.close();
}
}
TCP通信服务器端(重点)
作用:接收客户端的请求,和客户端经过3次握手建立连接;接收客户端发送的数据,给客户端回写数据
public class Demo02TCPServer {
public static void main(String[] args) throws IOException {
ServerSocket server = new ServerSocket(8888);
Socket socket = server.accept();
InputStream is = socket.getInputStream();
byte[] bytes = new byte[1024];
int len = is.read(bytes);
System.out.println(new String(bytes,0,len));
OutputStream os = socket.getOutputStream();
os.write("收到,谢谢".getBytes());
socket.close();
server.close();
}
}
服务器启动之后,服务器的accpet方法一直处于监听状态,等待客户端连接 
综合案例
文件上传案例需求
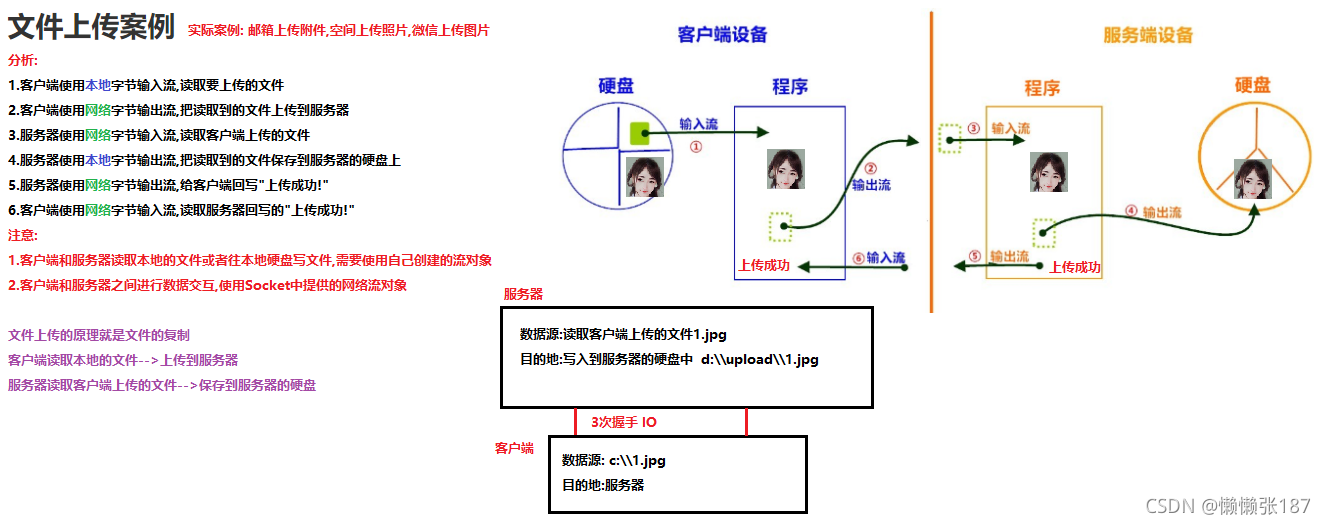
文件上传客户端(重点)
public class Demo01TCPClient {
public static void main(String[] args) throws IOException {
FileInputStream fis = new FileInputStream("c:\\1.jpg");
Socket socket = new Socket("127.0.0.1",9999);
OutputStream os = socket.getOutputStream();
byte[] bytes = new byte[1024];
int len = 0;
while ((len = fis.read(bytes))!=-1){
os.write(bytes,0,len);
}
InputStream is = socket.getInputStream();
while ((len = is.read(bytes))!=-1){
System.out.println(new String(bytes,0,len));
}
fis.close();
socket.close();
}
}
文件上传服务器端(重点)
public class Demo02TCPServer {
public static void main(String[] args) throws IOException {
File file = new File("d:\\upload");
if(!file.exists()){
file.mkdir();
}
ServerSocket server = new ServerSocket(9999);
Socket socket = server.accept();
InputStream is = socket.getInputStream();
FileOutputStream fos = new FileOutputStream(file+"\\1.jpg");
byte [] bytes = new byte[1024];
int len = 0;
while ((len = is.read(bytes))!=-1){
fos.write(bytes,0,len);
}
socket.getOutputStream().write("上传成功!".getBytes());
fos.close();
socket.close();
server.close();
}
}
文件上传的阻塞问题(重点)
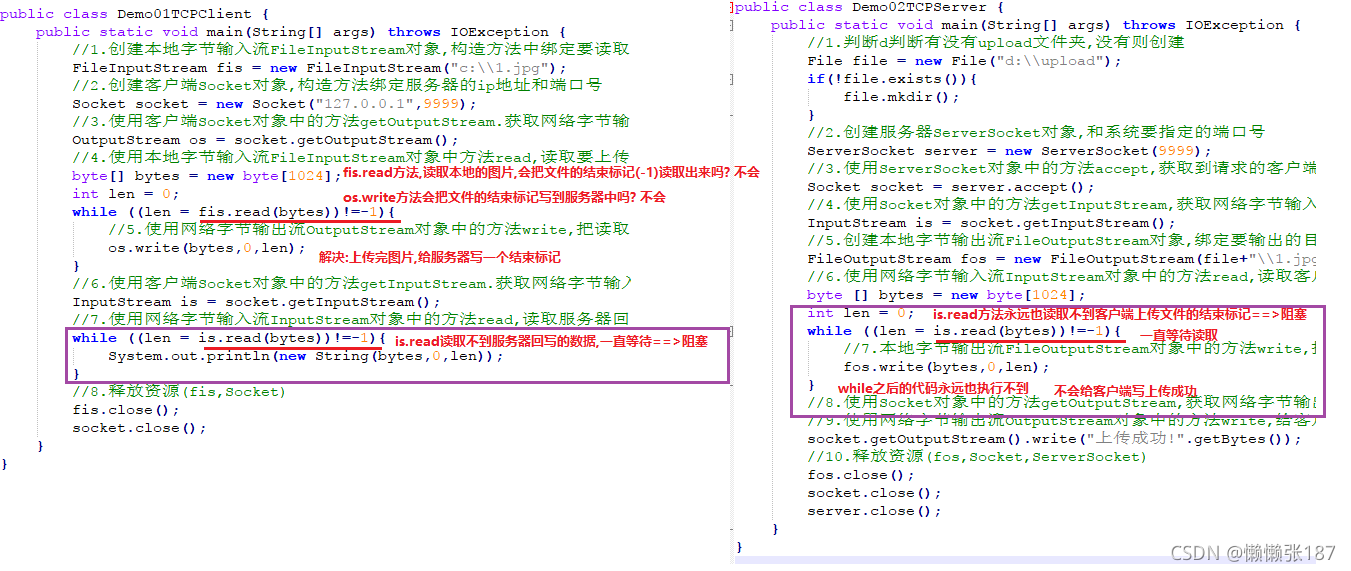
socket.shutdownOutput();
文件上传自定义文件名称命名规则
public class Demo02TCPServer {
public static void main(String[] args) throws IOException {
File file = new File("d:\\upload");
if(!file.exists()){
file.mkdir();
}
ServerSocket server = new ServerSocket(9999);
Socket socket = server.accept();
InputStream is = socket.getInputStream();
String fileName = "snapictrue"+System.currentTimeMillis()+new Random().nextInt(999999)+".jpg";
FileOutputStream fos = new FileOutputStream(file+File.separator+fileName);
byte [] bytes = new byte[1024];
int len = 0;
while ((len = is.read(bytes))!=-1){
fos.write(bytes,0,len);
}
socket.getOutputStream().write("上传成功!".getBytes());
fos.close();
socket.close();
server.close();
}
}
多线程版本服务器
public class Demo02TCPServer {
public static void main(String[] args) throws IOException {
File file = new File("d:\\upload");
if(!file.exists()){
file.mkdir();
}
ServerSocket server = new ServerSocket(9999);
while (true){
Socket socket = server.accept();
new Thread(new Runnable() {
@Override
public void run() {
try {
InputStream is = socket.getInputStream();
String fileName = "snapictrue"+System.currentTimeMillis()+new Random().nextInt(999999)+".jpg";
FileOutputStream fos = new FileOutputStream(file+File.separator+fileName);
byte [] bytes = new byte[1024];
int len = 0;
while ((len = is.read(bytes))!=-1){
fos.write(bytes,0,len);
}
socket.getOutputStream().write("上传成功!".getBytes());
fos.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}).start();
}
}
}
|