前言
websockt是需要依赖http1.1 然后返回其响应码101 再开始由http协议转为 webSocket
1、MyServer
/**
* @author wzcstart
* @date 2021/6/30 - 1:49
*/
public class MyServer {
public static void main(String[] args) throws Exception{
EventLoopGroup bossGroup = new NioEventLoopGroup(1);
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.handler(new LoggingHandler(LogLevel.INFO))//netty自带得日志处理器
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
//拿到pipeline
ChannelPipeline pipeline = ch.pipeline();
//webSocket 协议是 需要依赖 http1.1 协议
//添加http 编解码器
pipeline.addLast(new HttpServerCodec());
//是以块方式写,添加ChunkedWriteHandler处理器
pipeline.addLast(new ChunkedWriteHandler());
/*
说明
1. http数据在传输过程中是分段的,HttpObjectAggregator,就是可以将多个段聚合
2. 这就就是为什么,当浏览器发送大量数据时,就会发出多次http请求
*/
pipeline.addLast(new HttpObjectAggregator(1024*8));
/*
说明
1. 对应webSocket,它的数据是以 帧(frame)的形式传递
2. 可以看到WebSocketFrame 下面有六个子类
3. 浏览器请求时ws://localhost:port/hello 表示请求的uri 必须对应
4. WebSocketServerProtocolHandler 核心作用时将 http 转换为 ws 协议,保持长连接
*/
pipeline.addLast(new WebSocketServerProtocolHandler("/hello"));
//定义自己的业务处理子类
pipeline.addLast(new MyTextWebSocketFrameHandler());
}
});
//启动服务器
ChannelFuture channelFuture = bootstrap.bind(8080).sync();
channelFuture.channel().closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
}
2、MyTextWebSocketFrameHandler
/**
* @author wzcstart
* @date 2021/6/30 - 4:03
*/
public class MyTextWebSocketFrameHandler extends SimpleChannelInboundHandler<TextWebSocketFrame> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, TextWebSocketFrame msg) throws Exception {
//显示信息
System.out.println("服务器收到消息:"+msg.text());
System.out.println("非唯一:"+ctx.channel().id().asShortText());
//回显
ctx.channel().writeAndFlush(new TextWebSocketFrame("服务器时间 "+LocalDateTime.now()+" "+msg.text()));
}
//web客户端创建连接
@Override
public void handlerAdded(ChannelHandlerContext ctx) throws Exception {
//asLongText()是唯一id,asShortText()不是
System.out.println("handlerAdded被调用:"+ctx.channel().id().asLongText());
System.out.println("handlerAdded被调用:"+ctx.channel().id().asShortText());
}
//web客户端断开连接
@Override
public void handlerRemoved(ChannelHandlerContext ctx) throws Exception {
System.out.println("handlerRemoved被调用:"+ctx.channel().id().asLongText());
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
System.out.println("异常发生:"+cause.getMessage());
ctx.close();
}
}
3、hello.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script>
if (window.WebSocket){
var socket = new WebSocket("ws://localhost:8080/hello");
//相当于channelRead0,ev相当于消息
socket.onmessage = function (ev) {
var rt = document.getElementById("responseText");
rt.value = rt.value + "\n" +ev.data;
}
//相当于连接开始(感知到连接开始)
socket.onopen = function (ev) {
var rt = document.getElementById("responseText");
rt.value = "连接开始了~";
}
//相当于连接开始(感知到连接开始)
socket.onclose = function (ev) {
var rt = document.getElementById("responseText");
rt.value = rt.value + "\n" +"连接关闭了~";
}
}else {
alert("浏览器不支持webSocket")
}
//发送消息到服务端
function send(message) {
//先判断socket是否创建好了
if (!window.socket){
return;
}
if (socket.readyState==WebSocket.OPEN){
//通过socket发送消息
socket.send(message)
}else {
alert("连接没有开启")
}
}
</script>
</head>
<body>
<form οnsubmit="return false">
<textarea name="message" style="height: 300px; width: 300px"></textarea>
<input type="button" value="提交消息" οnclick="send(this.form.message.value)">
<textarea id="responseText" style="height: 300px; width: 300px"></textarea>
<input type="button" value="清空结果" οnclick="clear(document.getElementById('responseText').value='')">
</form>
</body>
</html>
4、效果展示
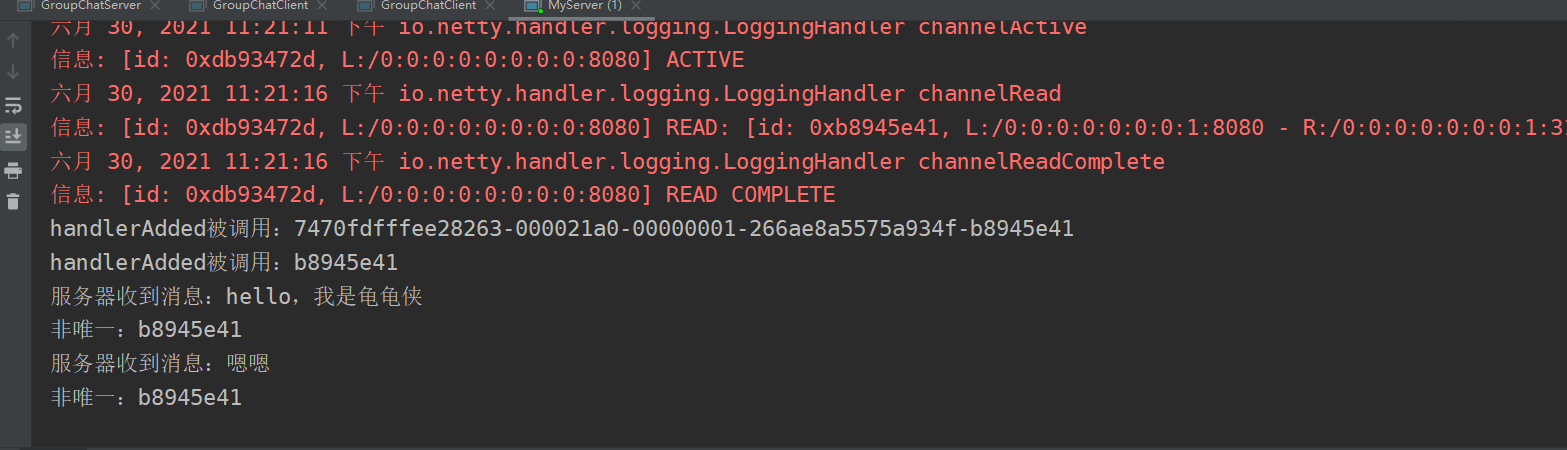
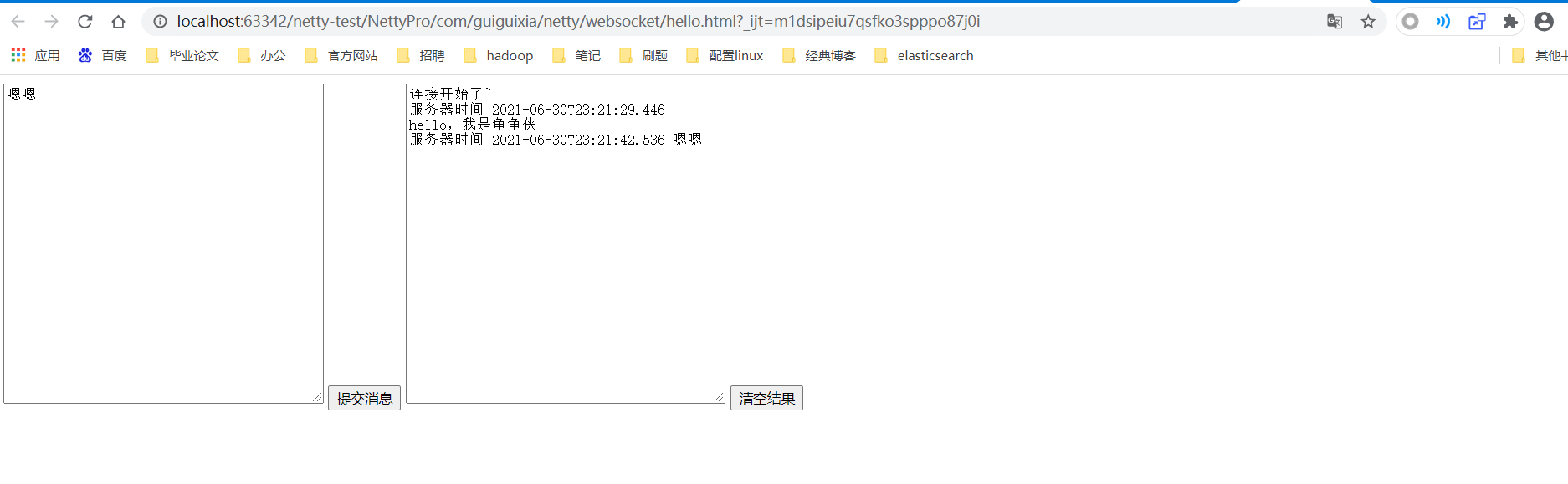
|