WebSocket是双向的,在客户端-服务器通信的场景中使用的全双工协议,与HTTP不同,它以ws://或wss://开头。它是一个有状态协议,这意味着客户端和服务器之间的连接将保持活动状态,直到被任何一方(客户端或服务器)终止。在通过客户端和服务器中的任何一方关闭连接之后,连接将从两端终止。 以上概述转自知乎:https://zhuanlan.zhihu.com/p/113286469 服务器端:
const WebSocket = require('ws')
const wss = new WebSocket.Server({port:8000})
wss.on('connection',function(ws){
console.log('a new client is conntected');
ws.on('message',function(msg){
wss.clients.forEach(function each(client){
if(client !== ws && client.readyState === WebSocket.OPEN){
client.send(msg.toString())
}
})
})
})
客户端:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
</head>
<body>
<div id="app-4">
<ol>
<li v-for="todo in todos">
{{ todo.text }}
</li>
</ol>
<input type="text" v-model="val" name="" id="">
<button @click="add" type="button">发送</button>
</div>
</body>
<script>
var app4 = new Vue({
el: '#app-4',
data: {
todos: [
{ text: '学习 JavaScript' },
{ text: '学习 Vue' },
{ text: '整个牛项目' }
],
val:''
},
mounted(){
var _this = this
this.wsHandle = new WebSocket('ws://localhost:8000');
this.wsHandle.onopen = this.onOpen
this.wsHandle.onmessage = this.onmessage
},
methods:{
add(){
this.wsHandle.send(this.val)
this.todos.push({
text: this.val
})
this.val = ''
},
onOpen:function(){
console.log('client is connected');
},
onmessage: function(e){
this.todos.push({
text: e.data
})
}
}
})
</script>
</html>
打开浏览器F12调试工具,可以看到客户端与服务端建立连接 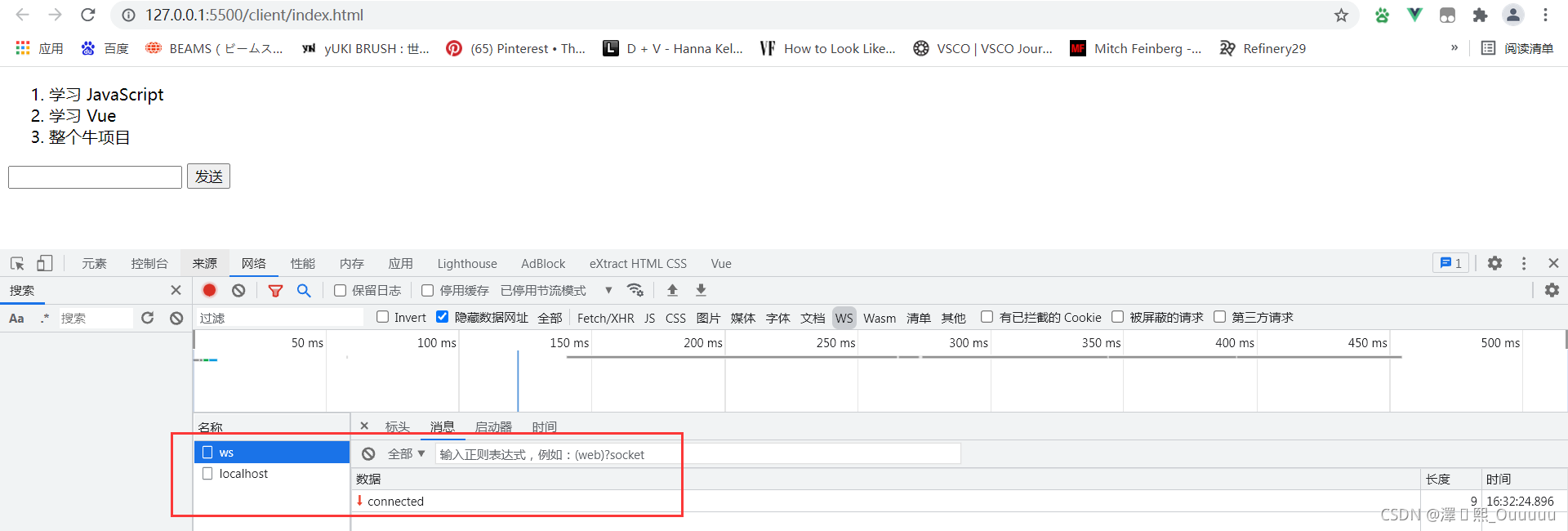 分别打开两个页面发送消息,通过服务端广播到其他用户 可以看到客户端1推送了一条消息 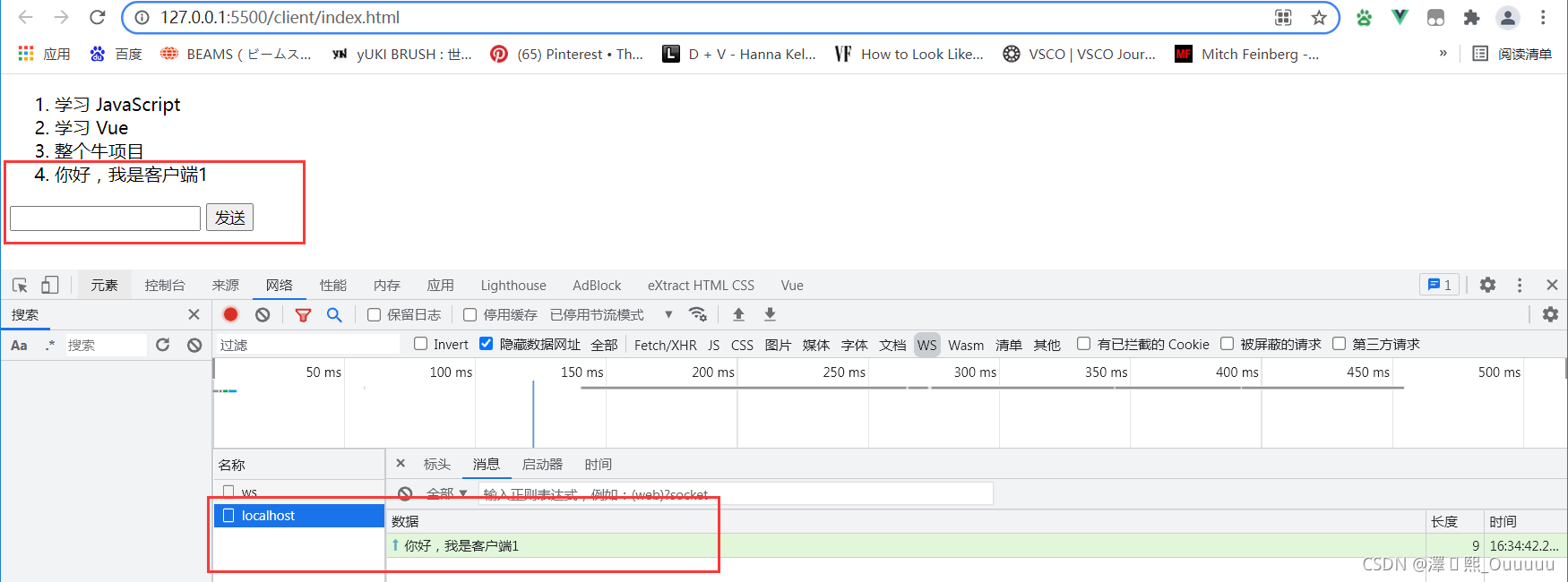 客户端2接收到了消息,并显示在消息列表上 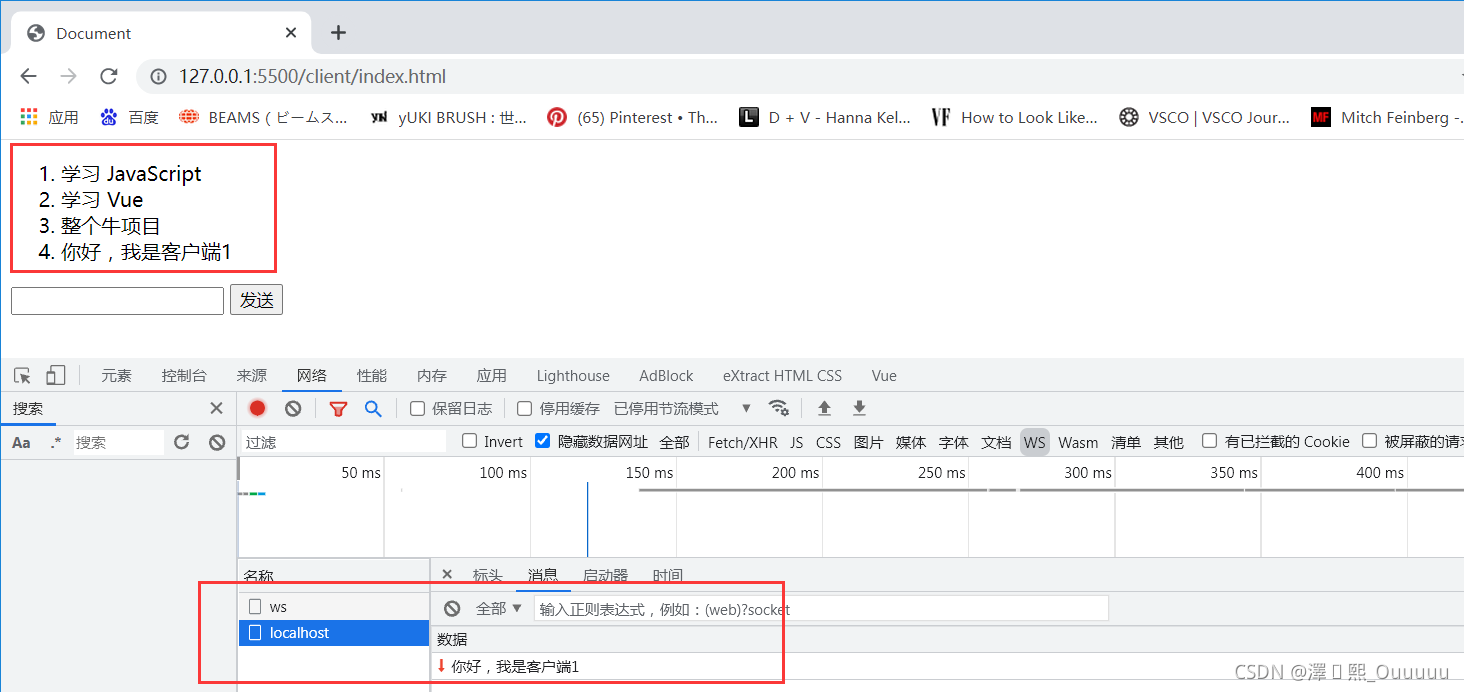
|