HTTP请求
在Spring Security中的很多内容校验都是通过对HTTP请求中的数据项进行的判断
请求报文
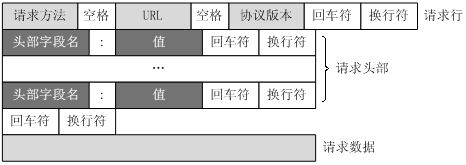
基于RestClient插件理解请求报文
RestClient是VSCode的API测试插件,创建后缀为.http 的文件则会被VSCode识别成Rest Client的工具文件
Get请求测试
构建Get请求
@GetMapping("/01")
public String firstApi() {
return "Hello World";
}
测试Get请求
GET http://localhost:8080/test/01
- 由于没有认证,Response Status为401
- 使用Basic Auth的方式添加请求头【注意:Basic后面需要跟base64编码的用户名和密码,但是Rest Client为了方便允许使用user + password的方式进行添加】
- 添加后发送请求
GET http://localhost:8080/test/01
Authorization: Basic user:0baf3007-ffa6-4ce1-96ea-efbb356cf3bd
Put请求测试
构建Put请求
@PutMapping("/02")
public String secApi(@RequestParam String name) {
return "Hello " + name;
}
测试PUT请求
- 直接编写Put请求头内容以及Authentication请求头并发送请求
PUT http://localhost:8080/test/02?name=Jack
Authorization: Basic user a08a4450-a0ff-4bdb-9b96-d31835ff2b40
- 发现请求始终提示403
- 开启对于security的debug日志级别
logging.level.org.springframework.security.web=DEBUG
- 发现日志中错误:
Invalid CSRF token found for http://localhost:8080/test/02?name=Jack - 添加security配置代码,预先关闭csrf filter
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf(AbstractHttpConfigurer::disable)
.httpBasic(Customizer.withDefaults())
.formLogin(form -> form.loginPage("/"));
}
}
Post请求测试
构建Post请求
@PostMapping("/03")
public String thiApi(@RequestBody User user) {
return "Hello " + user.getName();
}
@Data
private class User {
String name;
String gender;
}
测试Post请求
- 直接编写Post请求头内容,Authentication请求头以及请求体并发送请求
POST http://localhost:8080/test/03
Authorization: Basic user 5c11e9f9-c9d8-4322-8e5e-03fc36d91e6e
{
"name": "Susan",
"gender": "Man"
}
- 请求返回415
Unsupported Media Type - 添加Content-Type请求头
POST http://localhost:8080/test/03
Authorization: Basic user 5c11e9f9-c9d8-4322-8e5e-03fc36d91e6e
Content-Type: application/json
{
"name": "Susan",
"gender": "Man"
}
HTTP响应
响应报文
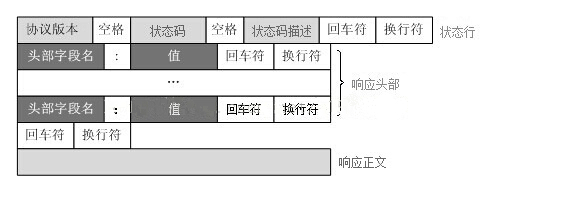
常用认证/授权相关状态码
- 401 - Unauthorized 未认证
- 403 - Forbidden 未授权
Http Basic Auth认证流程
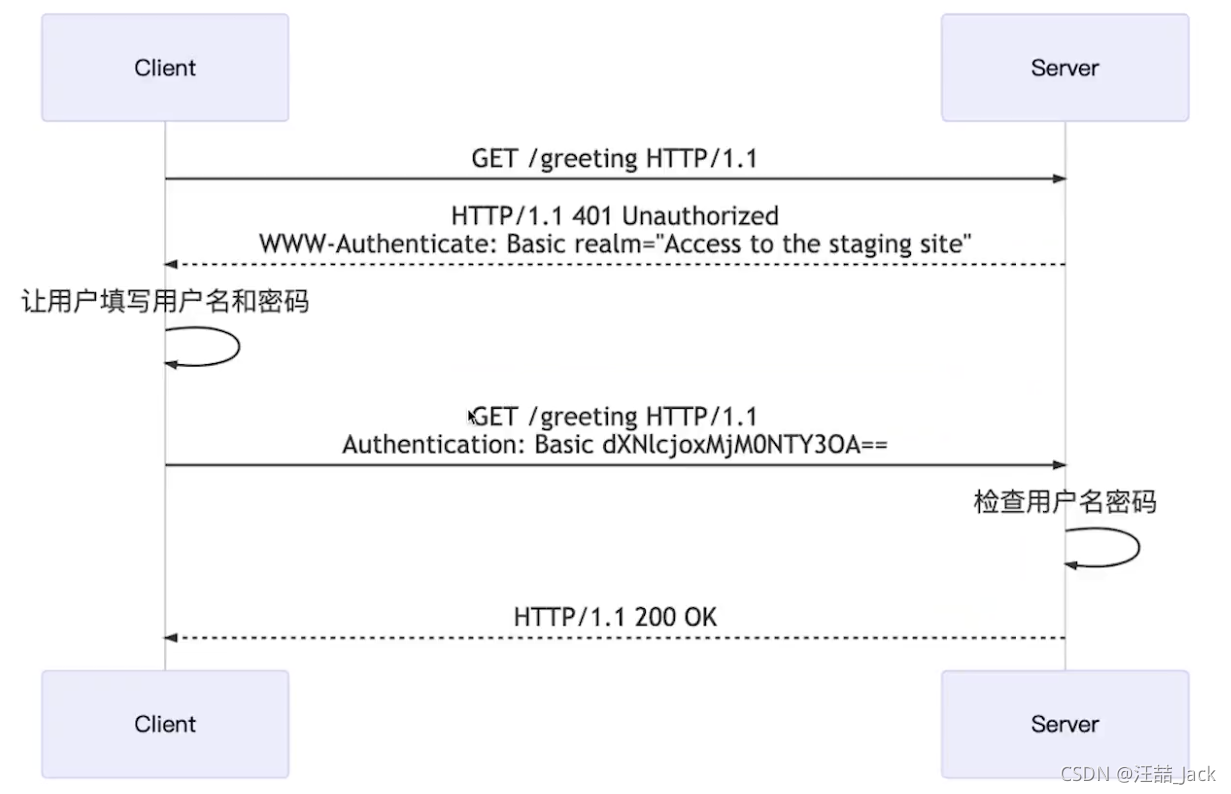
|