1、创建自签名证书
#使用JDK工具生成自签名证书JKS密钥库
keytool -genkeypair -alias tomcat_https -keypass 123456 -keyalg RSA -keysize 2048 -validity 365 -keystore d:/tomcat_https.keystore -storepass 123456
#从密钥库中导出数字证书
keytool -keystore d:/tomcat_https.keystore -export -alias tomcat_https -file d:/server.cer
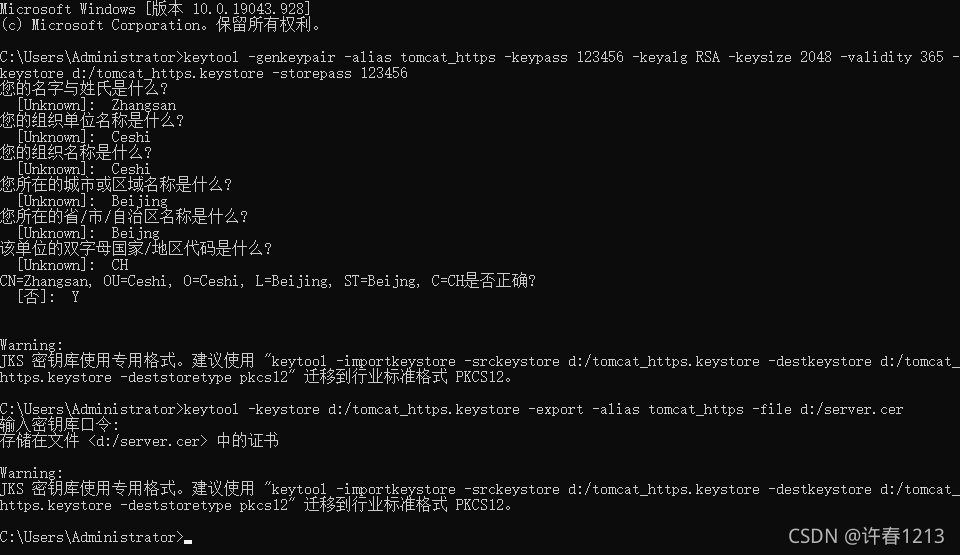 生成好的证书文件如下: 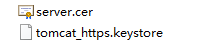
2、配置密钥库
将生成的密钥库文件和数字证书的拷贝至resource目录下并在application文件中配置相关信息:
#https默认端口:443,http默认端口:80
server.port=443
server.http-port=80
#开启https,配置跟证书一一对应
server.ssl.enabled=true
#指定证书
server.ssl.key-store=classpath:tomcat_https.keystore
server.ssl.key-store-type=JKS
#别名
server.ssl.key-alias=tomcat_https
#密码
server.ssl.key-password=123456
server.ssl.key-store-password=123456
spring.application.name=springboot-https
项目目录结构如下: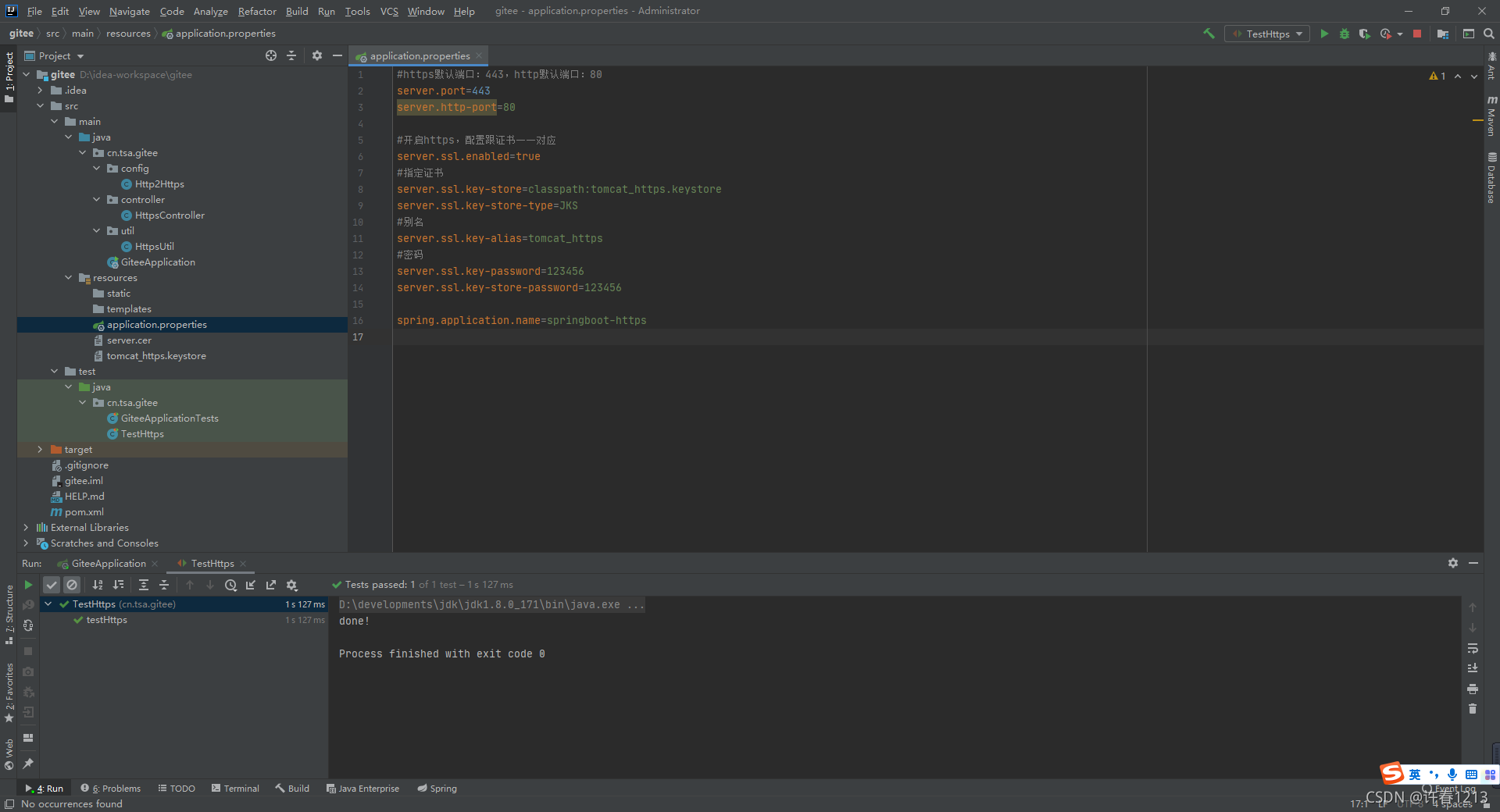
3、http强制跳转https
在config目录下新建Http2Https.java文件,内容如下:
package cn.tsa.gitee.config;
import org.apache.catalina.Context;
import org.apache.catalina.connector.Connector;
import org.apache.tomcat.util.descriptor.web.SecurityCollection;
import org.apache.tomcat.util.descriptor.web.SecurityConstraint;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.web.embedded.tomcat.TomcatServletWebServerFactory;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
/**
* http强制跳转https
*/
@Configuration
public class Http2Https {
@Value("${server.port}")
private int sslPort;//https的端口
@Value("${server.http-port}")
private int httpPort;//http的端口
@Bean
public TomcatServletWebServerFactory servletContainerFactory() {
TomcatServletWebServerFactory tomcat = new TomcatServletWebServerFactory() {
@Override
protected void postProcessContext(Context context) {
//设置安全性约束
SecurityConstraint securityConstraint = new SecurityConstraint();
securityConstraint.setUserConstraint("CONFIDENTIAL");
//设置约束条件
SecurityCollection collection = new SecurityCollection();
//拦截所有请求
collection.addPattern("/*");
securityConstraint.addCollection(collection);
context.addConstraint(securityConstraint);
}
};
Connector connector = new Connector("org.apache.coyote.http11.Http11NioProtocol");
//设置将分配给通过此连接器接收到的请求的方案
connector.setScheme("http");
//true: http使用http, https使用https;
//false: http重定向到https;
connector.setSecure(false);
//设置监听请求的端口号,这个端口不能其他已经在使用的端口重复,否则会报错
connector.setPort(httpPort);
//重定向端口号(非SSL到SSL)
connector.setRedirectPort(sslPort);
tomcat.addAdditionalTomcatConnectors(connector);
return tomcat;
}
}
4、地址访问
启动项目,访问:http://localhost:80/hello或者https://localhost:443/hello,都会自动跳转到https://localhost/hello 
5、程序访问
测试程序访问接口: 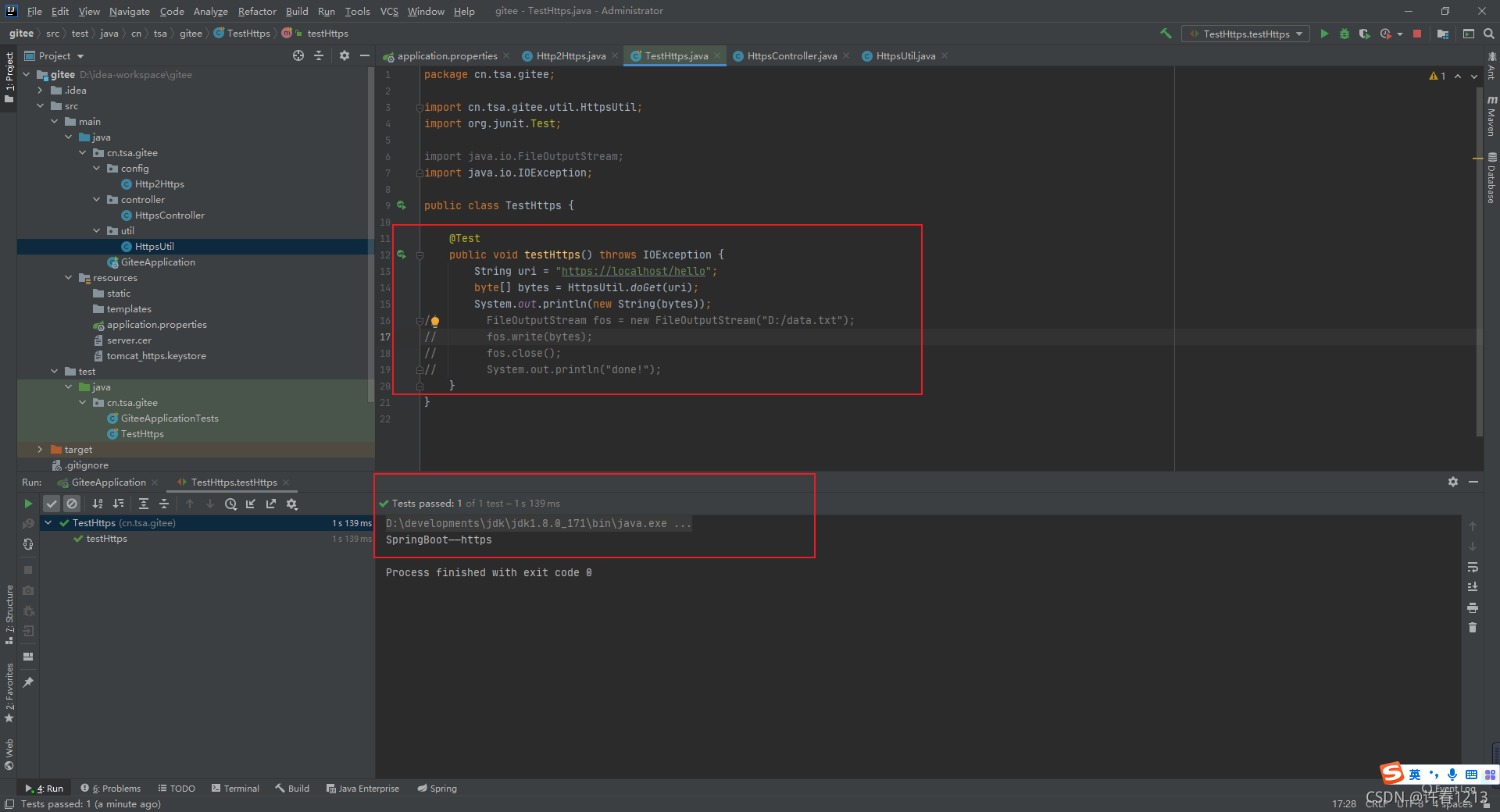 在util目录下新建HttpsUtil.java文件,内容如下:
package cn.tsa.gitee.util;
import java.io.*;
import java.net.URL;
import java.security.KeyManagementException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.KeyManager;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLSession;
import javax.net.ssl.SSLSocketFactory;
import javax.net.ssl.TrustManager;
import javax.net.ssl.X509TrustManager;
public class HttpsUtil {
private static final class DefaultTrustManager implements X509TrustManager {
@Override
public void checkClientTrusted(X509Certificate[] chain, String authType) throws CertificateException {
}
@Override
public void checkServerTrusted(X509Certificate[] chain, String authType) throws CertificateException {
}
@Override
public X509Certificate[] getAcceptedIssuers() {
return null;
}
}
private static HttpsURLConnection getHttpsURLConnection(String uri, String method) throws IOException {
SSLContext ctx = null;
try {
ctx = SSLContext.getInstance("TLS");
ctx.init(new KeyManager[0], new TrustManager[]{new DefaultTrustManager()}, new SecureRandom());
} catch (KeyManagementException e) {
e.printStackTrace();
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
SSLSocketFactory ssf = ctx.getSocketFactory();
URL url = new URL(uri);
HttpsURLConnection httpsConn = (HttpsURLConnection) url.openConnection();
httpsConn.setSSLSocketFactory(ssf);
httpsConn.setHostnameVerifier(new HostnameVerifier() {
@Override
public boolean verify(String arg0, SSLSession arg1) {
return true;
}
});
httpsConn.setRequestMethod(method);
httpsConn.setDoInput(true);
httpsConn.setDoOutput(true);
return httpsConn;
}
private static byte[] getBytesFromStream(InputStream is) throws IOException {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] kb = new byte[1024];
int len;
while ((len = is.read(kb)) != -1) {
baos.write(kb, 0, len);
}
byte[] bytes = baos.toByteArray();
baos.close();
is.close();
return bytes;
}
private static void setBytesToStream(OutputStream os, byte[] bytes) throws IOException {
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
byte[] kb = new byte[1024];
int len;
while ((len = bais.read(kb)) != -1) {
os.write(kb, 0, len);
}
os.flush();
os.close();
bais.close();
}
public static byte[] doGet(String uri) throws IOException {
HttpsURLConnection httpsConn = getHttpsURLConnection(uri, "GET");
return getBytesFromStream(httpsConn.getInputStream());
}
public static byte[] doPost(String uri, String data) throws IOException {
HttpsURLConnection httpsConn = getHttpsURLConnection(uri, "POST");
setBytesToStream(httpsConn.getOutputStream(), data.getBytes());
return getBytesFromStream(httpsConn.getInputStream());
}
}
|