网络编程
1.1 概述
地球村:
简单点说,就是:地球村是说地球虽然很大,但是由于信息传递越来越方便,大家交流就像在一个小村子里面一样便利,就称地球这个大家庭为“地球村”了。
计算机网络:
计算机网络是指将地理位置不同的具有独立功能的多台计算机及其外部设备,通过通信线路连接起来,在网络操作系统,网络管理软件及网络通信协议的管理和协调下,实现资源共享和信息传递的计算机系统。
网络编程的目的:
无线电台、传播交流信息、数据交换、通信
想要达到这个效果需要什么:
- 如何准确定位网络上的一台主机 192.168.31.59:端口 ,定位到这个计算机的资源
- 找到了这个主机。如何传输数据呢
javaweb : 网页编程 B/S
网络编程 : TCP/IP C/S
1.2 网络通信的要素
如何实现网络的通信?
通信双方地址:
规则:网络通信的协议:
TCP/IP参考模型:
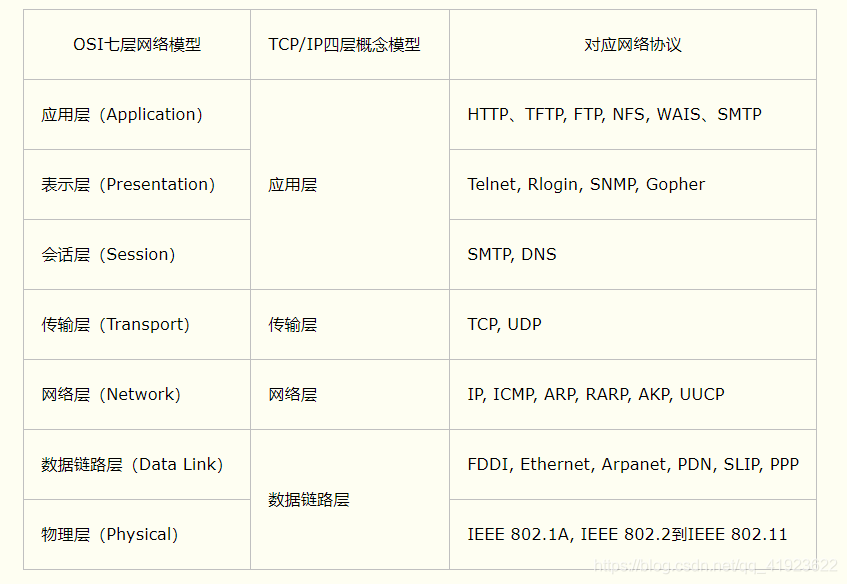
小结:
1.网络编程中两个主要问题
- 如何准确定位到网络上的一台或多台主机 cmd中 Ping + 域名
- 找到主机之后如何进行通信
2.网络编程中的要素
3.万物皆对象
1.3 IP
ip地址:InetAddress
- 唯一定位一台网络上计算机
- 127.0.0.1:本机 localhost
- ip地址的分类:
- ipv4/ipv6
- IPV4 127.0.0.1 ,4个字节组成。 0-255 ,42亿;30亿都在北美,亚洲4亿。2011年就用尽
- IPV6 128位。8个无符号整数!
- 域名:记忆IP问题
package Thread;
import java.net.InetAddress;
import java.net.UnknownHostException;
public class TestInetAddress {
public static void main(String[] args) {
try {
InetAddress address1 = InetAddress.getByName("127.0.0.1");
System.out.println(address1);
InetAddress address3 = InetAddress.getByName("localhost");
System.out.println(address3);
InetAddress address4 = InetAddress.getLocalHost();
System.out.println(address4);
InetAddress address2 = InetAddress.getByName("www.baidu.com");
System.out.println(address2);
System.out.println(address2.getCanonicalHostName());
System.out.println(address2.getHostAddress());
System.out.println(address2.getHostName());
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
}
/127.0.0.1
localhost/127.0.0.1
PC-202003271358/192.168.31.59
www.baidu.com/36.152.44.95
[B@74a14482
36.152.44.95
36.152.44.95
www.baidu.com
1.4 端口Port
端口表示计算机上的一个程序的进程:
package net;
import java.net.InetSocketAddress;
public class TestInetSocketAddress {
public static void main(String[] args) {
InetSocketAddress socketAddress = new InetSocketAddress("127.0.0.1",53331);
InetSocketAddress socketAddress1 = new InetSocketAddress("localhsot",53331);
System.out.println(socketAddress);
System.out.println(socketAddress1);
System.out.println(socketAddress.getAddress());
System.out.println(socketAddress.getHostName());
System.out.println(socketAddress.getPort());
}
}
/127.0.0.1:53331
localhsot:53331
/127.0.0.1
ieonline.microsoft.com
53331
1.5 通信协议
协议:约定,就好比我们说的是普通话
网络通信协议:速率,传输码率,代码结构,传输控制…
问题:非常的复杂?
大事化了:分层!
TCP/IP协议簇:实际上是一组协议
重要:
出名的协议:
TCP UDP对比:
TCP:打电话
- 连接,稳定
- ‘三次握手’’四次挥手‘
- 客户端,服务端
- 传输完成,断开连接,效率低
UDP:发短信
- 不连接,不稳定
- 客户端,服务端:没有明确的界限
- 不管有没有准备好,都可以发给你
1.6 TCP
客户端:
- 连接服务器Socket
- 发送消息
package net;
import java.io.IOException;
import java.io.OutputStream;
import java.net.InetAddress;
import java.net.Socket;
public class TCPClientDemo01 {
public static void main(String[] args) {
Socket socket = null;
OutputStream os = null;
try {
InetAddress address = InetAddress.getByName("127.0.0.1");
int port = 9999;
socket = new Socket(address,port);
os = socket.getOutputStream();
os.write("阿马喜欢学习".getBytes());
} catch (Exception e) {
e.printStackTrace();
}finally {
if (os != null);{
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket != null);{
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
服务端:
- 建立服务的端口ServerSocket
- 等待用户的连接 accept
- 接收用的消息
package net;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.ServerSocket;
import java.net.Socket;
public class TCPServerDemo01 {
public static void main(String[] args) {
ServerSocket serverSocket = null;
Socket accept = null;
InputStream is = null;
ByteArrayOutputStream baos = null;
try {
serverSocket = new ServerSocket(9999);
while (true){
accept = serverSocket.accept();
is = accept.getInputStream();
baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len=is.read(buffer))!=-1){
baos.write(buffer,0,len);
}
System.out.println(baos.toString());
}
} catch (IOException e) {
e.printStackTrace();
}finally {
if (baos != null){
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (is != null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (accept != null){
try {
accept.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (serverSocket != null){
try {
serverSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
文件上传
客户端
package net;
import java.io.IOException;
import java.io.OutputStream;
import java.net.InetAddress;
import java.net.Socket;
public class TCPClientDemo01 {
public static void main(String[] args) {
Socket socket = null;
OutputStream os = null;
try {
InetAddress address = InetAddress.getByName("127.0.0.1");
int port = 9999;
socket = new Socket(address,port);
os = socket.getOutputStream();
os.write("阿马喜欢学习".getBytes());
} catch (Exception e) {
e.printStackTrace();
}finally {
if (os != null);{
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket != null);{
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
服务端
package net;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.ServerSocket;
import java.net.Socket;
public class TCPServerDemo01 {
public static void main(String[] args) {
ServerSocket serverSocket = null;
Socket accept = null;
InputStream is = null;
ByteArrayOutputStream baos = null;
try {
serverSocket = new ServerSocket(9999);
while (true){
accept = serverSocket.accept();
is = accept.getInputStream();
baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len=is.read(buffer))!=-1){
baos.write(buffer,0,len);
}
System.out.println(baos.toString());
}
} catch (IOException e) {
e.printStackTrace();
}finally {
if (baos != null){
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (is != null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (accept != null){
try {
accept.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (serverSocket != null){
try {
serverSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
Tomcat
服务端
客户端
1.7 UDP
发短信,不用连接,只需要知道对方地址!
发送消息
发送端
package net;
import java.net.*;
public class UDPClientDemo01 {
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket();
String msg = "你好阿,服务器!";
InetAddress address = InetAddress.getByName("localhost");
int port = 9999;
DatagramPacket packet = new DatagramPacket(msg.getBytes(),msg.getBytes().length,address,port);
socket.send(packet);
socket.close();
}
}
接收端
package net;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.SocketException;
public class UDPServerDemo01 {
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket(9999);
byte[] buffer = new byte[1024];
DatagramPacket packet = new DatagramPacket(buffer,0,buffer.length);
socket.receive(packet);
String msg = new String(packet.getData(),0,packet.getLength());
System.out.println(packet.getAddress().getHostAddress());
System.out.println(msg);
socket.close();
}
}
循环发送消息
package net;
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
import java.net.SocketException;
public class UdpSenderDemo01 {
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket();
InetAddress address = InetAddress.getByName("localhost");
int port = 9999;
while (true){
InputStreamReader inputStreamReader = new InputStreamReader(System.in);
BufferedReader bufferedReader = new BufferedReader(inputStreamReader);
String data = bufferedReader.readLine();
byte[] buffer = data.getBytes();
DatagramPacket packet = new DatagramPacket(buffer,0,buffer.length,address,port);
socket.send(packet);
if (data.equals("bye")){
break;
}
}
socket.close();
}
}
循环接收消息
package net;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.SocketException;
public class UdpReceviceDemo01 {
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket(9999);
while (true){
byte[] container = new byte[1024];
DatagramPacket packet = new DatagramPacket(container,0,container.length);
socket.receive(packet);
byte[] data = packet.getData();
String receiveData = new String(data,0,data.length);
System.out.println(receiveData);
if (receiveData.equals("bye")){
break;
}
}
socket.close();
}
}
多线程在线咨询
两个人都可以时发送方,也可以是接收方
package net;
public class TalkStudent {
public static void main(String[] args) {
new Thread(new TalkSend(3333,6666,"localhost")).start();
new Thread(new TalkReceive(8888,"老师")).start();
}
}
package net;
public class TalkTeacher {
public static void main(String[] args) {
new Thread(new TalkSend(4444,8888,"localhost")).start();
new Thread(new TalkReceive(6666,"学生")).start();
}
}
发送信息类
package net;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
import java.net.SocketException;
public class TalkSend implements Runnable{
DatagramSocket socket = null;
BufferedReader bufferedReader = null;
InputStreamReader inputStreamReader = null;
private int fromPort;
private int toPort;
private String toIP;
public TalkSend(int fromPort,int toPort,String toIP){
this.fromPort = fromPort;
this.toPort = toPort;
this.toIP = toIP;
try{
socket = new DatagramSocket();
inputStreamReader = new InputStreamReader(System.in);
bufferedReader = new BufferedReader(inputStreamReader);
} catch (Exception e){
e.printStackTrace();
}
}
@Override
public void run() {
try {
while (true){
String data = bufferedReader.readLine();
byte[] buffer = data.getBytes();
DatagramPacket packet = new DatagramPacket(buffer,0,buffer.length,InetAddress.getByName(this.toIP),toPort);
socket.send(packet);
if (data.equals("bye")){
break;
}
}
socket.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
接收信息类
package net;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.SocketException;
public class TalkReceive implements Runnable{
DatagramSocket socket = null;
int port;
String msgFrom;
public TalkReceive(int port,String msgFrom){
this.msgFrom = msgFrom;
this.port = port;
try {
socket = new DatagramSocket(port);
} catch (SocketException e) {
e.printStackTrace();
}
}
@Override
public void run() {
while (true){
try{
byte[] container = new byte[1024];
DatagramPacket packet = new DatagramPacket(container,0,container.length);
socket.receive(packet);
byte[] data = packet.getData();
String receiveData = new String(data,0,data.length);
System.out.println(msgFrom+":"+receiveData);
if (receiveData.equals("bye")){
break;
}
}catch (Exception e){
e.printStackTrace();
}
}
socket.close();
}
}
|