一、TCP协议
TCP/IP (Transmission Control Protocol/Internet Protocol,传输控制协议/网际协议)是指能够在多个不同网络间实现信息传输的协议簇。TCP/IP协议不仅仅指的是TCP 和IP两个协议,而是指一个由FTP、SMTP、TCP、UDP、IP等协议构成的协议簇, 只是因为在TCP/IP协议中TCP协议和IP协议最具代表性,所以被称为TCP/IP协议。TCP/IP协议在一定程度上参考了OSI的体系结构。OSI模型共有七层,从下到上分别是物理层、数据链路层、网络层、运输层、会话层、表示层和应用层。
TCP/IP协议在一定程度上参考了OSI的体系结构。OSI模型共有七层,从下到上分别是物理层、数据链路层、网络层、运输层、会话层、表示层和应用层。但是这显然是有些复杂的,所以在TCP/IP协议中,它们被简化为了四个层次 应用层: 应用层是TCP/IP协议的第一层,是直接为应用进程提供服务的。 (1)对不同种类的应用程序它们会根据自己的需要来使用应用层的不同协议,邮件传输应用使用了SMTP协议、万维网应用使用了HTTP协议、远程登录服务应用使用了有TELNET协议。 [1] (2)应用层还能加密、解密、格式化数据。 [1] (3)应用层可以建立或解除与其他节点的联系,这样可以充分节省网络资源。 [1] 运输层: 作为TCP/IP协议的第二层,运输层在整个TCP/IP协议中起到了中流砥柱的作用。且在运输层中,TCP和UDP也同样起到了中流砥柱的作用。 [1] 网络层: 网络层在TCP/IP协议中的位于第三层。在TCP/IP协议中网络层可以进行网络连接的建立和终止以及IP地址的寻找等功能。 [1] 网络接口层: 在TCP/IP协议中,网络接口层位于第四层。由于网络接口层兼并了物理层和数据链路层所以,网络接口层既是传输数据的物理媒介,也可以为网络层提供一条准确无误的线路。 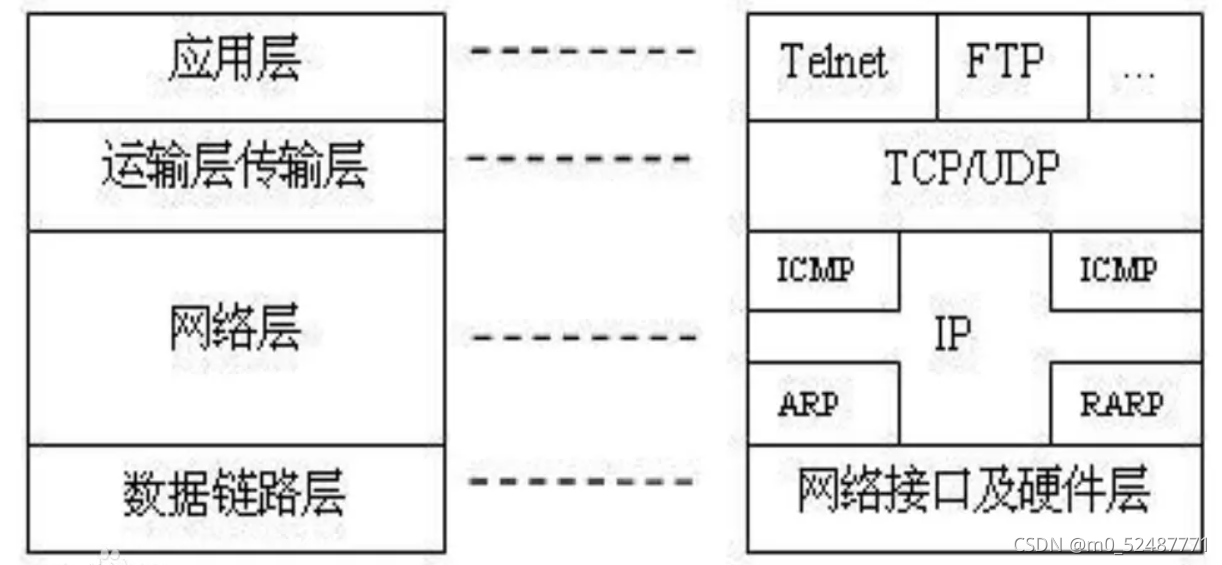
二、UDP协议
UDP协议提供了一种不同于TCP协议的端到端服务。UDP协议所提供的端到端传输服务是尽力而为(best-effort)的,即UDP套接字将尽可能地传送信息,但并不保证信息一定能成功到达目的地址,而且信息到达的顺序与其发送顺序不一定一致。 服务器端 ①创建一个socket,用函数socket() ②绑定IP地址、端口等信息到socket上,用函数bind() ③循环接收数据,用函数recvfrom() 客户端 ①创建一个socket,用函数socket() ②设置对方的IP地址和端口等属性 ③发送数据,用函数sendto() ④关闭
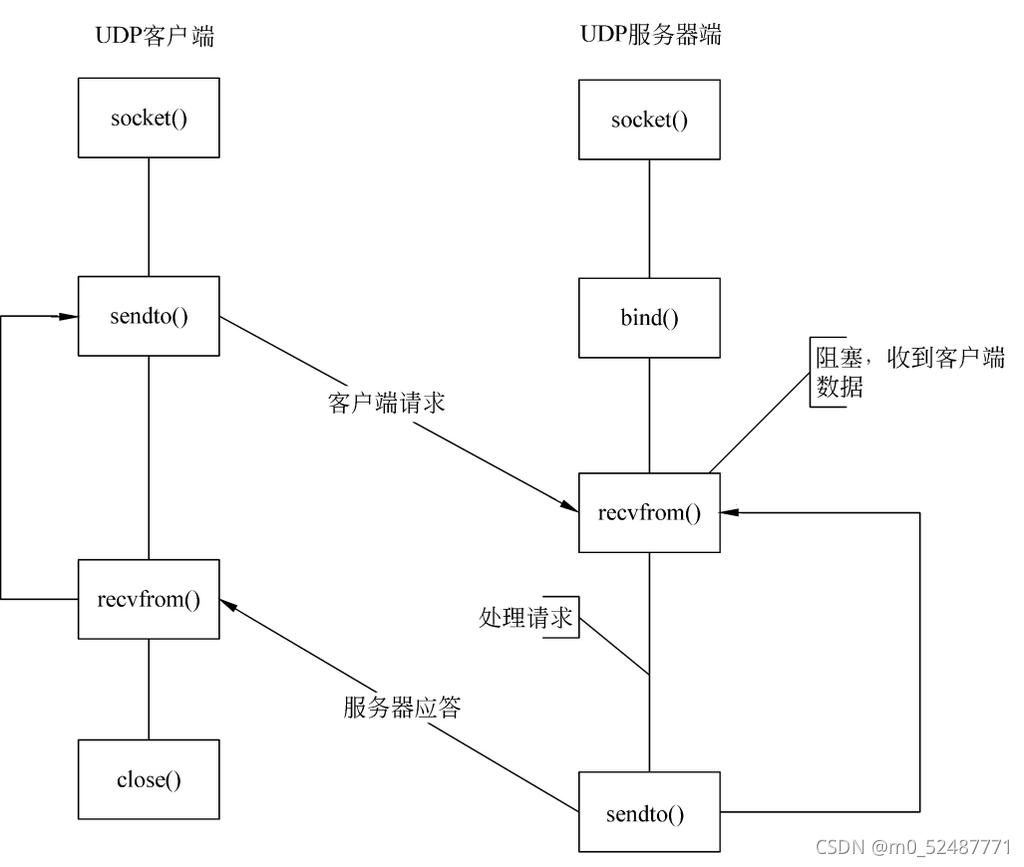
三、.C#实现连续输出HelloWorld
打开VS2019,选择c#,控制台应用(.NET Framework) 在自动生成的代码的main函数里添加
for(int i = 0; i < 50; i++)
{
Console.WriteLine("Hello World!");
Console.ReadKey();
}
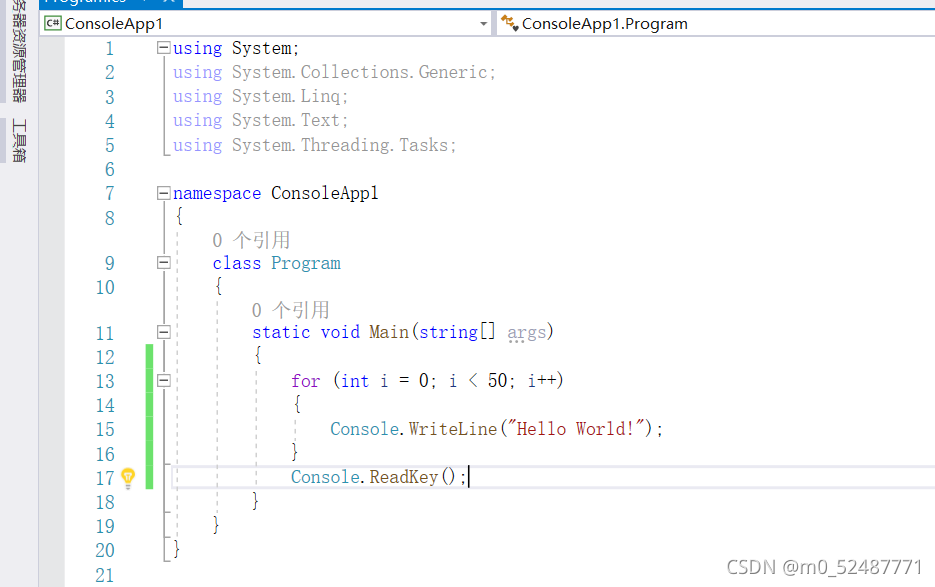 运行结果 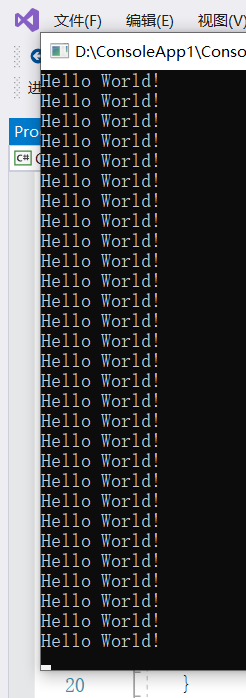
四、打开一个网络UDP 套接字,向自己电脑发送这50行消息
重新打开两个控制台,一个用来编写服务器端代码,另一个客户端代码 服务器端代码
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.Net.Sockets;
namespace UDPSever
{
internal class Program
{
static void Main(string[] args)
{
int recv;
byte[] data = new byte[1024];
IPEndPoint ip = new IPEndPoint(IPAddress.Any, 8001);
Socket newsock = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp);
newsock.Bind(ip);
Console.WriteLine("This is a Server, host name is {0}", Dns.GetHostName());
Console.WriteLine("Waiting for a client");
IPEndPoint sender = new IPEndPoint(IPAddress.Any, 0);
EndPoint Remote = (EndPoint)(sender);
recv = newsock.ReceiveFrom(data, ref Remote);
Console.WriteLine("Message received from {0}: ", Remote.ToString());
Console.WriteLine(Encoding.UTF8.GetString(data, 0, recv));
string welcome = "你好 ! ";
data = Encoding.UTF8.GetBytes(welcome);
newsock.SendTo(data, data.Length, SocketFlags.None, Remote);
while (true)
{
data = new byte[1024];
recv = newsock.ReceiveFrom(data, ref Remote);
Console.WriteLine(Encoding.UTF8.GetString(data, 0, recv));
}
}
}
}
客户端代码,编写客户端代码前可以在控制台输入ipconfig查询自己的ip地址,IPEndPoint(IPAddress.Parse(“192.168.43.39”), 8001);这行代码的ip地址是你要发送的对象的电脑的ip地址。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.Net.Sockets;
namespace UDPclick
{
internal class Program
{
static void Main(string[] args)
{
byte[] data = new byte[1024];
string input, stringData;
Console.WriteLine("This is a Client, host name is {0}", Dns.GetHostName());
IPEndPoint ip = new IPEndPoint(IPAddress.Parse("192.168.43.39"), 8001);
Socket server = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp);
string welcome = "你好! ";
data = Encoding.UTF8.GetBytes(welcome);
server.SendTo(data, data.Length, SocketFlags.None, ip);
IPEndPoint sender = new IPEndPoint(IPAddress.Any, 0);
EndPoint Remote = (EndPoint)sender;
data = new byte[1024];
int recv = server.ReceiveFrom(data, ref Remote);
Console.WriteLine("Message received from {0}: ", Remote.ToString());
Console.WriteLine(Encoding.UTF8.GetString(data, 0, recv));
int i = 0;
while (true)
{
string s = "hello cqjtu!重交物联2019级" + i;
Console.WriteLine(s);
server.SendTo(Encoding.UTF8.GetBytes(s), Remote);
if (i == 50)
{
break;
}
i++;
}
Console.WriteLine("Stopping Client.");
server.Close();
Console.ReadKey();
}
}
}
先运行服务器端代码,再运行客户端代码,运行结果 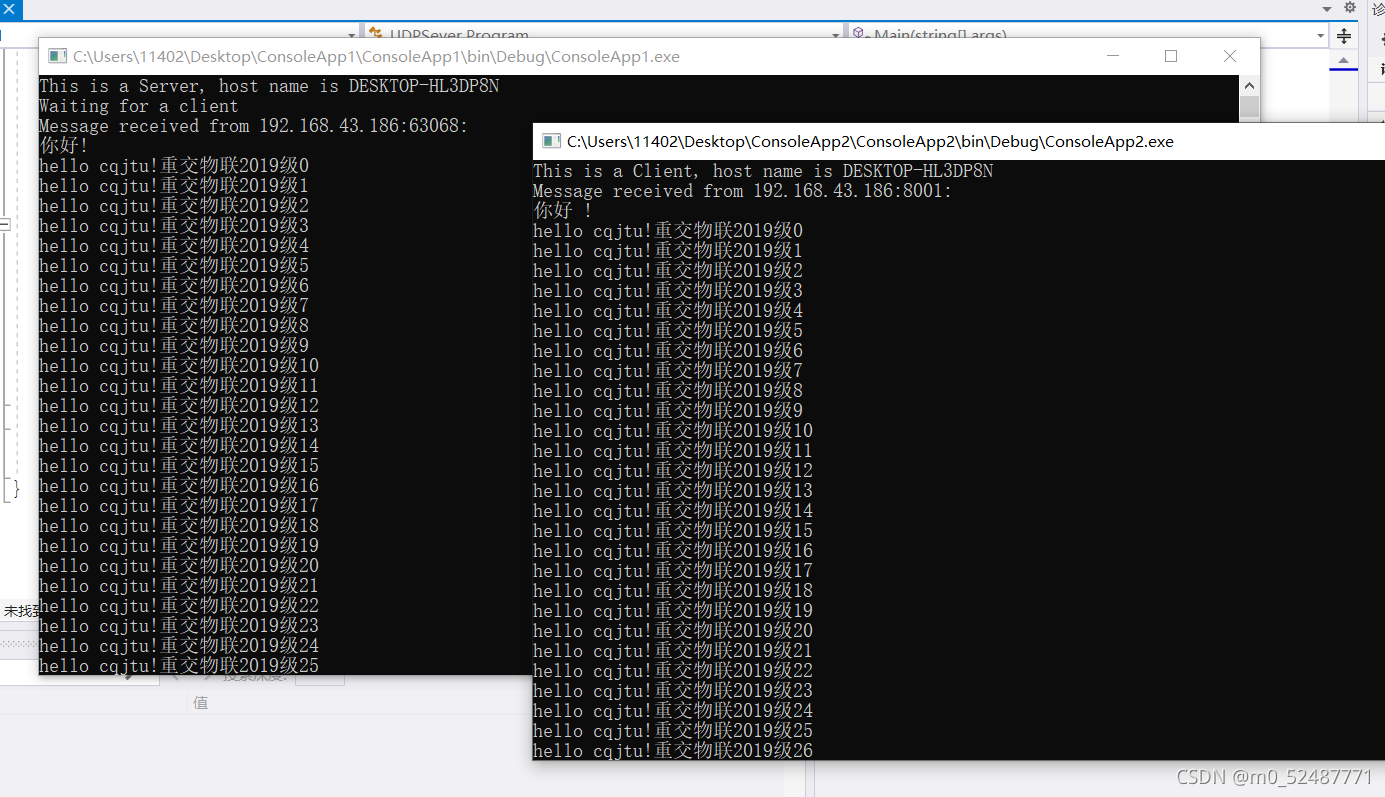
五、编写form窗口程序,采用UDP套接字发送程序
创建两个Windows窗体应用(.NET Framework),同样是一个服务器,一个客户端 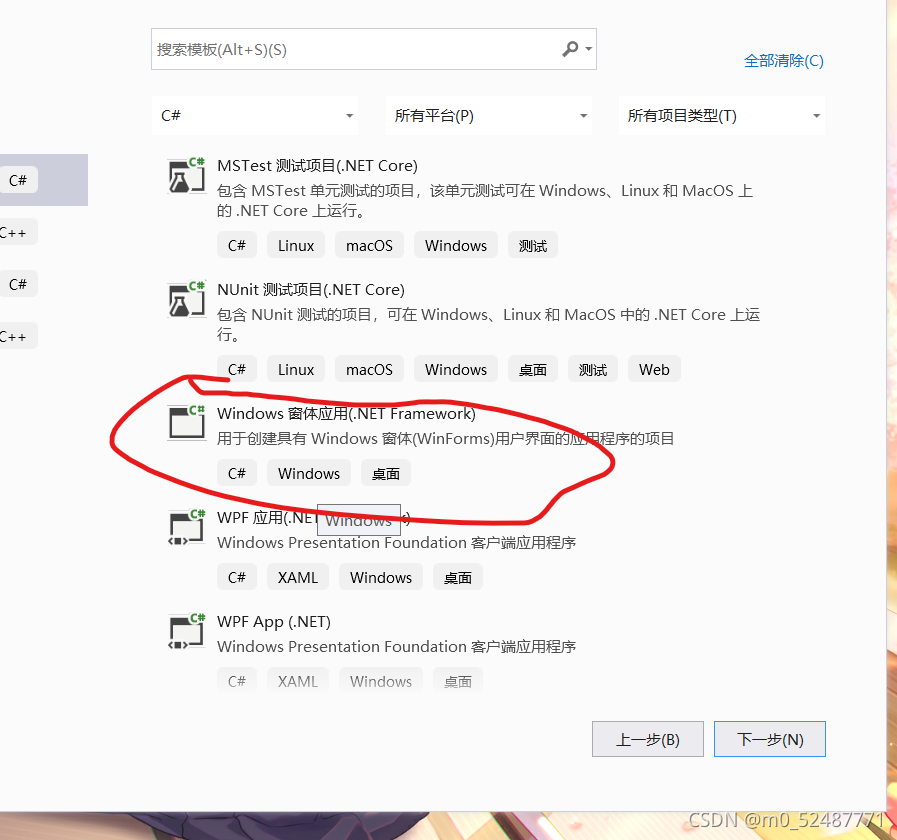 打开工具箱,拖动两个文本框textBox和两个按钮button到设计窗口上 客户端布局 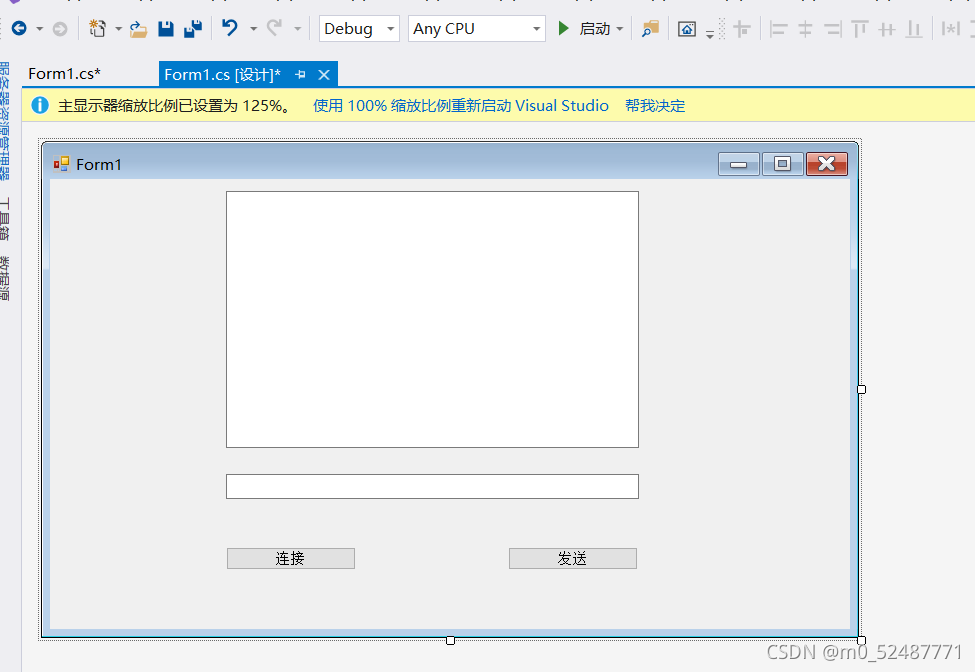 服务器端布局 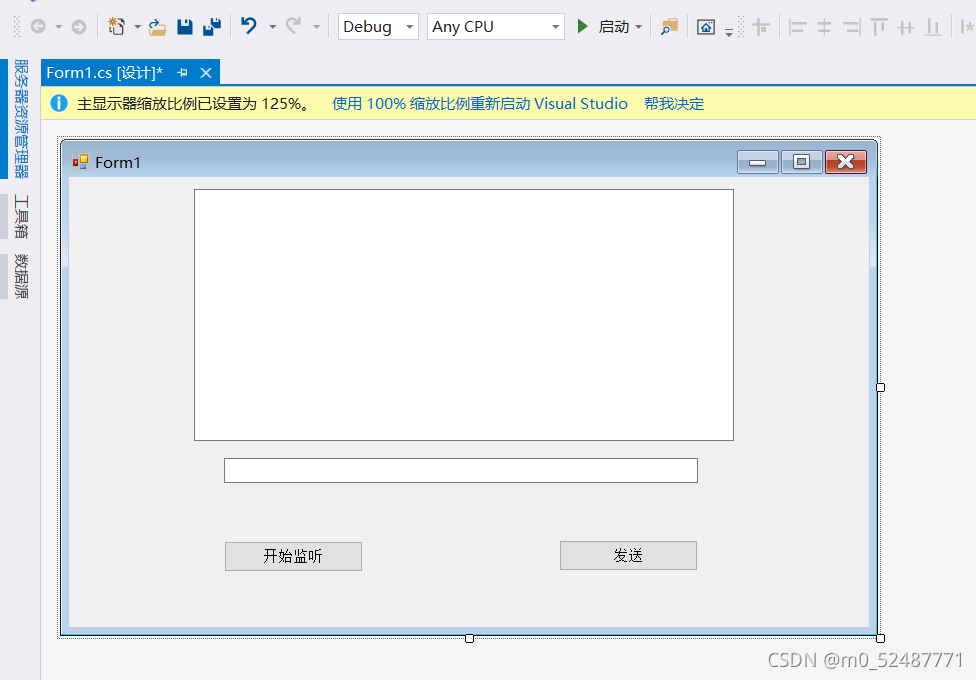 开始编写代码 客户端代码 双击连接按钮 为连接按钮编写代码,连接按钮外面创建socket对象Socket socketSend;
Socket socketSend;
private void button2_Click(object sender, EventArgs e)
{
socketSend = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
IPEndPoint point = new IPEndPoint(IPAddress.Parse("192.168.43.39"), 8001);
socketSend.Connect(point);
showMsg("连接成功!");
Thread th = new Thread(Receive);
th.IsBackground = true;
th.Start();
}
添加函数Receive()
void Receive()
{
try
{
while (true)
{
byte[] buffer = new byte[1024 * 1024 * 2];
int r = socketSend.Receive(buffer);
if (r == 0)
{
break;
}
string str = Encoding.UTF8.GetString(buffer, 0, r);
Invoke(new Action(() => {
showMsg(socketSend.RemoteEndPoint + ":" + str);
}));
}
}
catch
{ }
}
添加showMSG函数
void showMsg(string str)
{
textBox2.AppendText(str + "\r\n");
}
双击发送按钮添加
private void button1_Click(object sender, EventArgs e)
{
string str = textBox1.Text;
byte[] buffer = System.Text.Encoding.UTF8.GetBytes(str);
socketSend.Send(buffer);
}
服务器端代码 双击开始监听按钮
private void button2_Click(object sender, EventArgs e)
{
try
{
Socket socketwatch = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
IPAddress ip = IPAddress.Any;
IPEndPoint point = new IPEndPoint(ip, 8001);
socketwatch.Bind(point);
showMsg("监听成功!");
socketwatch.Listen(10);
Thread th = new Thread(listen);
th.IsBackground = true;
th.Start(socketwatch);
}
catch
{
showMsg("监听失败");
}
}
在Form函数前定义
string str = "没有改变";
添加监听函数listen
Socket socketSend;
void listen(Object o)
{
try
{
Socket socketwatch = o as Socket;
int i = 0;
while (true)
{
socketSend = socketwatch.Accept();
str = socketSend.RemoteEndPoint.ToString() + ":" + "连接成功!";
Invoke(new Action(() => {
showMsg(socketSend.RemoteEndPoint.ToString() + ":" + "连接成功!");
}));
Thread th = new Thread(Receive);
th.IsBackground = true;
th.Start(socketSend);
}
}
catch
{ }
}
双击发送按钮
private void button1_Click(object sender, EventArgs e)
{
string str = textBox1.Text;
Console.WriteLine(str);
byte[] buffer = System.Text.Encoding.UTF8.GetBytes(str);
socketSend.Send(buffer);
}
运行 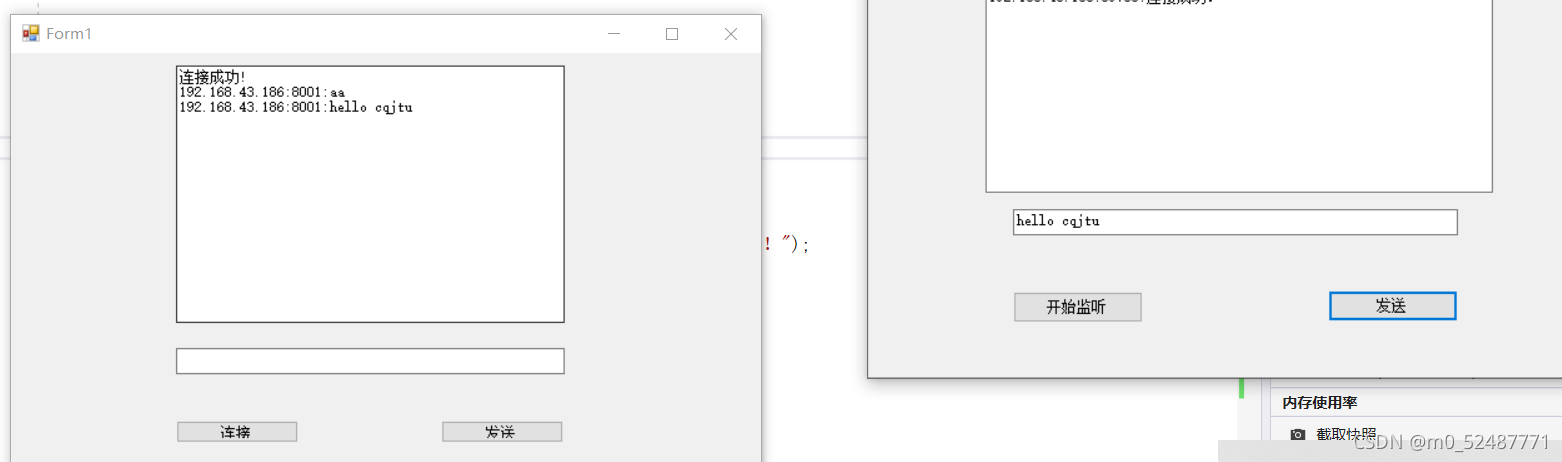
六、端口扫描器
单线程 创建窗体应用 界面设计 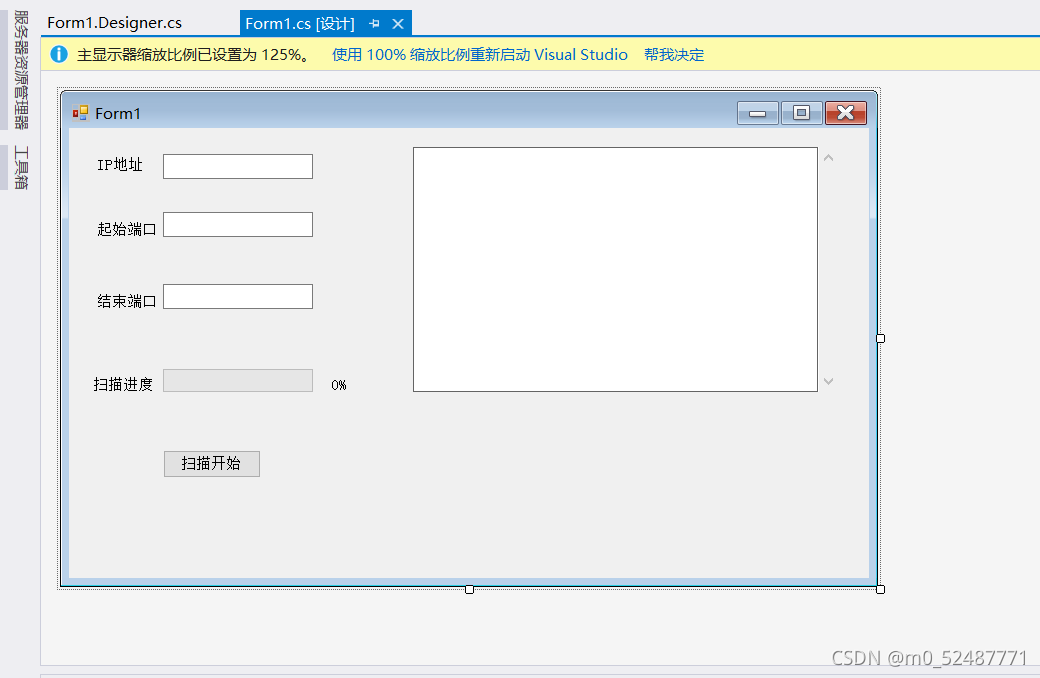 代码
namespace WindowsFormsApp2
{
partial class Form1
{
private System.ComponentModel.IContainer components = null;
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows 窗体设计器生成的代码
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.textBox1 = new System.Windows.Forms.TextBox();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.textBox2 = new System.Windows.Forms.TextBox();
this.textBox3 = new System.Windows.Forms.TextBox();
this.label4 = new System.Windows.Forms.Label();
this.progressBar1 = new System.Windows.Forms.ProgressBar();
this.button1 = new System.Windows.Forms.Button();
this.textBox4 = new System.Windows.Forms.TextBox();
this.label5 = new System.Windows.Forms.Label();
this.SuspendLayout();
this.label1.AutoSize = true;
this.label1.Location = new System.Drawing.Point(25, 29);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(53, 15);
this.label1.TabIndex = 0;
this.label1.Text = "IP地址";
this.textBox1.Location = new System.Drawing.Point(94, 26);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(150, 25);
this.textBox1.TabIndex = 1;
this.label2.AutoSize = true;
this.label2.Location = new System.Drawing.Point(25, 94);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(67, 15);
this.label2.TabIndex = 2;
this.label2.Text = "起始端口";
this.label2.Click += new System.EventHandler(this.label2_Click);
this.label3.AutoSize = true;
this.label3.Location = new System.Drawing.Point(25, 166);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(67, 15);
this.label3.TabIndex = 3;
this.label3.Text = "结束端口";
this.textBox2.Location = new System.Drawing.Point(94, 84);
this.textBox2.Name = "textBox2";
this.textBox2.Size = new System.Drawing.Size(150, 25);
this.textBox2.TabIndex = 4;
this.textBox3.Location = new System.Drawing.Point(94, 156);
this.textBox3.Name = "textBox3";
this.textBox3.Size = new System.Drawing.Size(150, 25);
this.textBox3.TabIndex = 5;
this.label4.AutoSize = true;
this.label4.Location = new System.Drawing.Point(21, 249);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(67, 15);
this.label4.TabIndex = 6;
this.label4.Text = "扫描进度";
this.progressBar1.Location = new System.Drawing.Point(94, 241);
this.progressBar1.Name = "progressBar1";
this.progressBar1.Size = new System.Drawing.Size(150, 23);
this.progressBar1.TabIndex = 7;
this.button1.Location = new System.Drawing.Point(94, 322);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(98, 28);
this.button1.TabIndex = 8;
this.button1.Text = "扫描开始";
this.button1.UseVisualStyleBackColor = true;
this.button1.Click += new System.EventHandler(this.button1_Click);
this.textBox4.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle;
this.textBox4.Enabled = false;
this.textBox4.Location = new System.Drawing.Point(344, 19);
this.textBox4.Multiline = true;
this.textBox4.Name = "textBox4";
this.textBox4.ScrollBars = System.Windows.Forms.ScrollBars.Vertical;
this.textBox4.Size = new System.Drawing.Size(426, 245);
this.textBox4.TabIndex = 9;
this.label5.AutoSize = true;
this.label5.Location = new System.Drawing.Point(259, 249);
this.label5.Name = "label5";
this.label5.Size = new System.Drawing.Size(23, 15);
this.label5.TabIndex = 10;
this.label5.Text = "0%";
this.AutoScaleDimensions = new System.Drawing.SizeF(8F, 15F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(800, 450);
this.Controls.Add(this.label5);
this.Controls.Add(this.textBox4);
this.Controls.Add(this.button1);
this.Controls.Add(this.progressBar1);
this.Controls.Add(this.label4);
this.Controls.Add(this.textBox3);
this.Controls.Add(this.textBox2);
this.Controls.Add(this.label3);
this.Controls.Add(this.label2);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.label1);
this.Name = "Form1";
this.Text = "Form1";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.TextBox textBox3;
private System.Windows.Forms.Label label4;
private System.Windows.Forms.ProgressBar progressBar1;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.TextBox textBox4;
private System.Windows.Forms.Label label5;
}
}
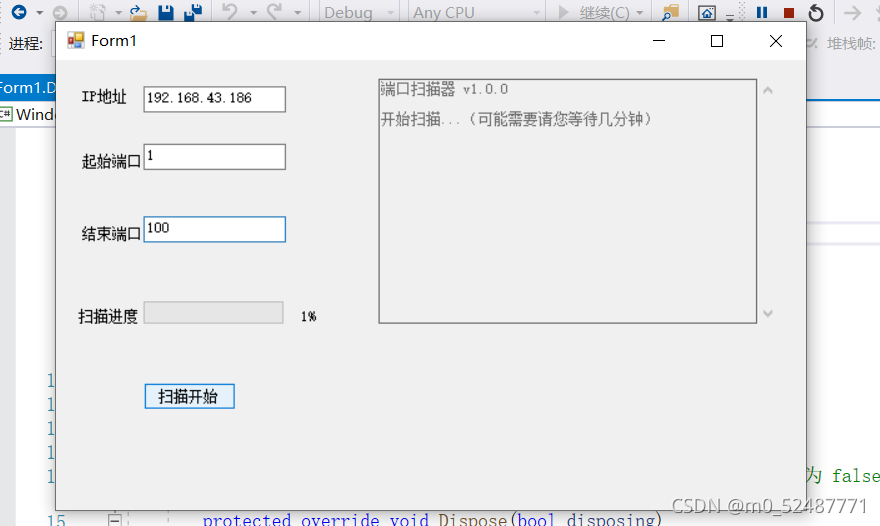 多线程 界面设计 代码
namespace WindowsFormsApp3
{
partial class Form1
{
private System.ComponentModel.IContainer components = null;
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows 窗体设计器生成的代码
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.button1 = new System.Windows.Forms.Button();
this.textBox1 = new System.Windows.Forms.TextBox();
this.textBox2 = new System.Windows.Forms.TextBox();
this.textBox3 = new System.Windows.Forms.TextBox();
this.label4 = new System.Windows.Forms.Label();
this.label5 = new System.Windows.Forms.Label();
this.progressBar1 = new System.Windows.Forms.ProgressBar();
this.textBox4 = new System.Windows.Forms.TextBox();
this.SuspendLayout();
this.label1.AutoSize = true;
this.label1.Location = new System.Drawing.Point(45, 63);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(53, 15);
this.label1.TabIndex = 0;
this.label1.Text = "IP地址";
this.label2.AutoSize = true;
this.label2.Location = new System.Drawing.Point(45, 123);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(67, 15);
this.label2.TabIndex = 1;
this.label2.Text = "起始端口";
this.label3.AutoSize = true;
this.label3.Location = new System.Drawing.Point(45, 185);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(67, 15);
this.label3.TabIndex = 2;
this.label3.Text = "结束端口";
this.button1.Location = new System.Drawing.Point(48, 299);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(87, 23);
this.button1.TabIndex = 3;
this.button1.Text = "扫描";
this.button1.UseVisualStyleBackColor = true;
this.button1.Click += new System.EventHandler(this.button1_Click);
this.textBox1.Location = new System.Drawing.Point(118, 60);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(134, 25);
this.textBox1.TabIndex = 4;
this.textBox2.Location = new System.Drawing.Point(118, 120);
this.textBox2.Name = "textBox2";
this.textBox2.Size = new System.Drawing.Size(134, 25);
this.textBox2.TabIndex = 5;
this.textBox3.Location = new System.Drawing.Point(118, 182);
this.textBox3.Name = "textBox3";
this.textBox3.Size = new System.Drawing.Size(134, 25);
this.textBox3.TabIndex = 6;
this.label4.AutoSize = true;
this.label4.Location = new System.Drawing.Point(45, 239);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(67, 15);
this.label4.TabIndex = 8;
this.label4.Text = "扫描进度";
this.label5.AutoSize = true;
this.label5.Location = new System.Drawing.Point(269, 239);
this.label5.Name = "label5";
this.label5.Size = new System.Drawing.Size(23, 15);
this.label5.TabIndex = 10;
this.label5.Text = "0%";
this.progressBar1.Location = new System.Drawing.Point(118, 231);
this.progressBar1.Name = "progressBar1";
this.progressBar1.Size = new System.Drawing.Size(134, 23);
this.progressBar1.TabIndex = 11;
this.textBox4.Location = new System.Drawing.Point(346, 60);
this.textBox4.Multiline = true;
this.textBox4.Name = "textBox4";
this.textBox4.Size = new System.Drawing.Size(333, 245);
this.textBox4.TabIndex = 12;
this.AutoScaleDimensions = new System.Drawing.SizeF(8F, 15F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(800, 450);
this.Controls.Add(this.textBox4);
this.Controls.Add(this.progressBar1);
this.Controls.Add(this.label5);
this.Controls.Add(this.label4);
this.Controls.Add(this.textBox3);
this.Controls.Add(this.textBox2);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.button1);
this.Controls.Add(this.label3);
this.Controls.Add(this.label2);
this.Controls.Add(this.label1);
this.Name = "Form1";
this.Text = "Form1";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.TextBox textBox3;
private System.Windows.Forms.Label label4;
private System.Windows.Forms.Label label5;
private System.Windows.Forms.ProgressBar progressBar1;
private System.Windows.Forms.TextBox textBox4;
}
}
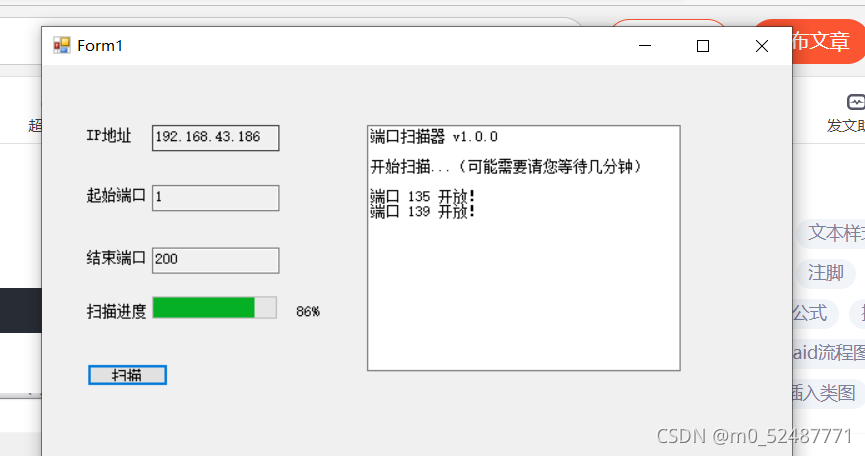
七、wireshark抓包
将代码的IP改为另一台电脑的IP地址 点击发送内容,可以看到抓到以下包
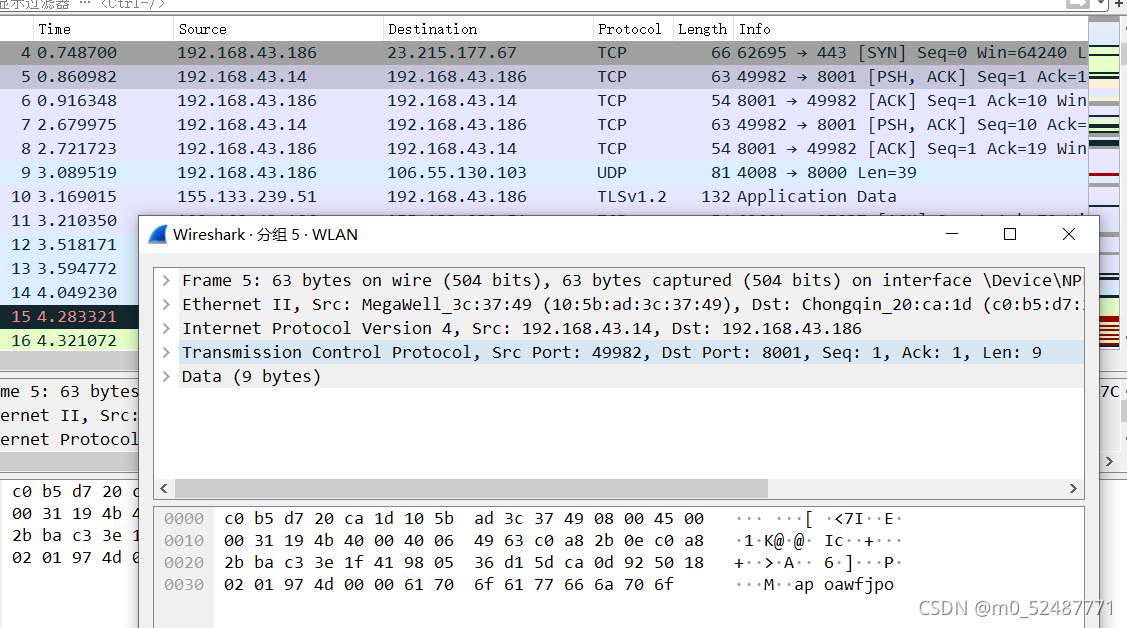 可以看到发送接收端口 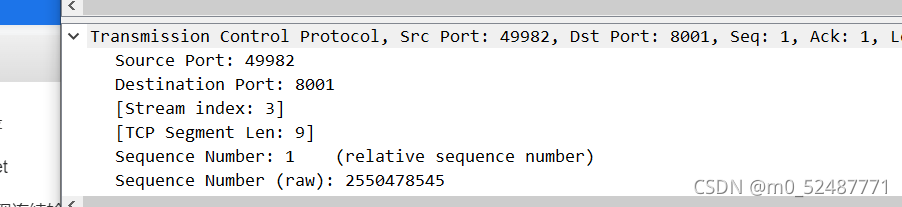
|