一.控制台程序使用 UDP 通信
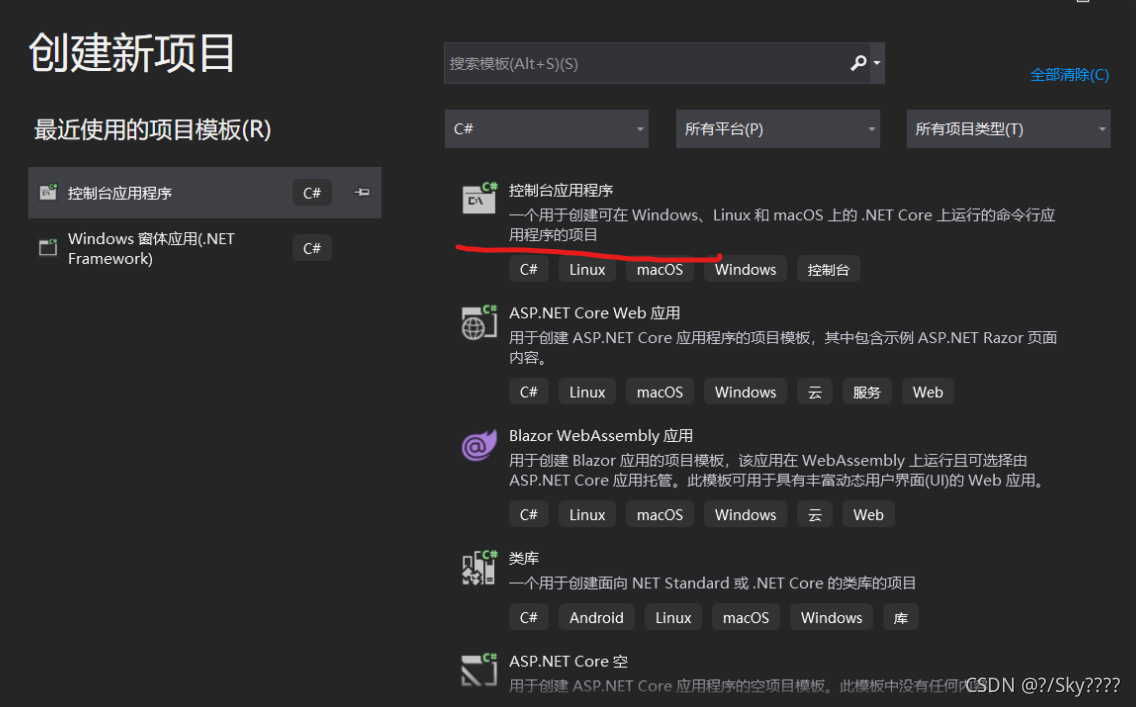
using System;
using System.Text;
using System.Net;
using System.Net.Sockets;
namespace Client
{
class Program
{
static void Main(string[] args)
{
byte[] data = new byte[1024];
Console.WriteLine("This is a Client, host name is {0}", Dns.GetHostName());
IPEndPoint ip = new IPEndPoint(IPAddress.Parse("192.168.0.178"), 8001);
Socket server = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp);
string welcome = "你好,服务器!";
data = Encoding.UTF8.GetBytes(welcome);
server.SendTo(data, data.Length, SocketFlags.None, ip);
IPEndPoint sender = new IPEndPoint(IPAddress.Any, 0);
EndPoint Remote = (EndPoint)sender;
data = new byte[1024];
int recv = server.ReceiveFrom(data, ref Remote);
Console.WriteLine("Message received from {0}: ", Remote.ToString());
Console.WriteLine(Encoding.UTF8.GetString(data, 0, recv));
int i = 0;
while (true)
{
string s = "hello cqjtu!重交物联2019级" + i;
Console.WriteLine(s);
server.SendTo(Encoding.UTF8.GetBytes(s), Remote);
if (i == 50)
{
break;
}
i++;
}
Console.WriteLine("Stopping Client.");
server.Close();
}
}
}
using System;
using System.Collections.Generic;
using System.Text;
using System.Net;
using System.Net.Sockets;
namespace Server
{
class Program
{
static void Main(string[] args)
{
int recv;
byte[] data = new byte[1024];
IPEndPoint ip = new IPEndPoint(IPAddress.Any, 8001);
Socket newsock = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp);
newsock.Bind(ip);
Console.WriteLine("This is a Server, host name is {0}", Dns.GetHostName());
Console.WriteLine("Waiting for a client");
while (true)
{
try
{
while (true)
{
IPEndPoint sender = new IPEndPoint(IPAddress.Any, 0);
EndPoint Remote = (EndPoint)(sender);
recv = newsock.ReceiveFrom(data, ref Remote);
Console.WriteLine("Message received from {0}: ", Remote.ToString());
Console.WriteLine(Encoding.UTF8.GetString(data, 0, recv));
string welcome = "你好 ! ";
data = Encoding.UTF8.GetBytes(welcome);
newsock.SendTo(data, data.Length, SocketFlags.None, Remote);
while (true)
{
if (!newsock.Poll(1000, SelectMode.SelectRead))
{
Console.WriteLine("连接中断!");
break;
}
data = new byte[1024];
recv = newsock.ReceiveFrom(data, ref Remote);
Console.WriteLine(Encoding.UTF8.GetString(data, 0, recv));
}
}
}
catch (System.Exception ex)
{
Console.WriteLine(ex.Message);
}
}
}
}
}
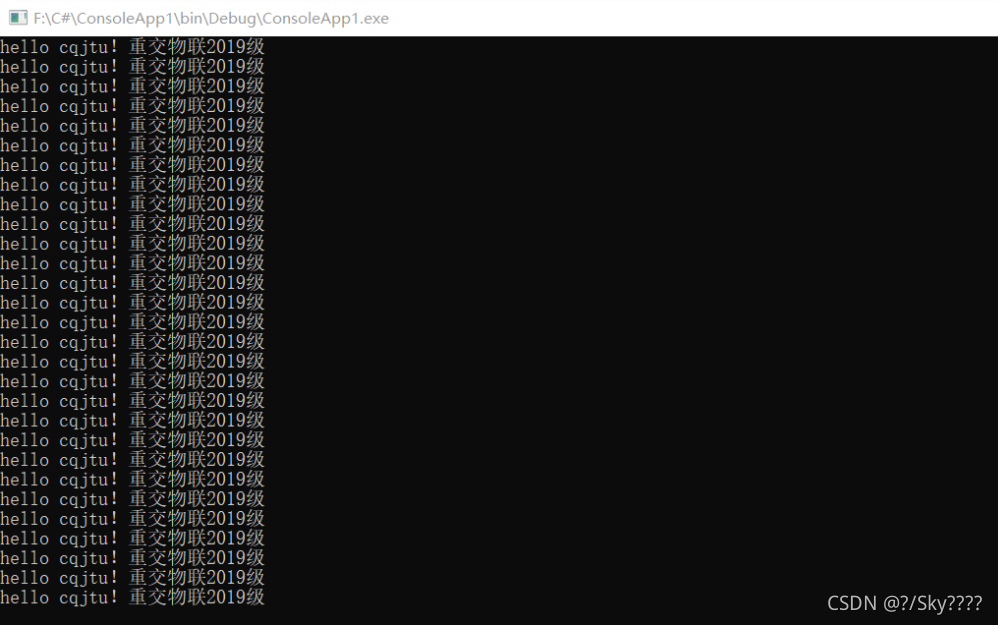
二.Form窗口程序使用 TCP 通信
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
try
{
string str = "The current time: ";
str += DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss");
textBox1.AppendText(str + Environment.NewLine);
int port = 2000;
string host = "192.168.0.178";
IPAddress ip = IPAddress.Parse(host);
IPEndPoint ipe = new IPEndPoint(ip, port);
Socket c = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
str = "Connect to server...";
textBox1.AppendText(str + Environment.NewLine);
c.Connect(ipe);
string sendStr = textBox2.Text;
str = "The message content: " + sendStr;
textBox1.AppendText(str + Environment.NewLine);
byte[] bs = Encoding.UTF8.GetBytes(sendStr);
str = "Send the message to the server...";
textBox1.AppendText(str + Environment.NewLine);
c.Send(bs, bs.Length, 0);
string recvStr = "";
byte[] recvBytes = new byte[1024];
int bytes;
bytes = c.Receive(recvBytes, recvBytes.Length, 0);
recvStr += Encoding.UTF8.GetString(recvBytes, 0, bytes);
str = "The server feedback: " + recvStr;
textBox1.AppendText(str + Environment.NewLine);
c.Close();
}
catch (ArgumentNullException f)
{
string str = "ArgumentNullException: " + f.ToString();
textBox1.AppendText(str + Environment.NewLine);
}
catch (SocketException f)
{
string str = "ArgumentNullException: " + f.ToString();
textBox1.AppendText(str + Environment.NewLine);
}
textBox1.AppendText("" + Environment.NewLine);
textBox2.Text = "";
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp3
{
class Program
{
static void Main(string[] args)
{
int i = 0;
int port = 2000;
string host = "192.168.0.178";
IPAddress ip = IPAddress.Parse(host);
IPEndPoint ipe = new IPEndPoint(ip, port);
Socket s = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
s.Bind(ipe);
while (true)
{
i++;
try
{
Console.WriteLine("\t-----------------------------------------------");
Console.Write("Perform operations {0} :", i);
s.Listen(0);
Console.WriteLine("1. Wait for connect...");
Socket temp = s.Accept();
Console.WriteLine("2. Get a connect");
string recvStr = "";
byte[] recvBytes = new byte[1024];
int bytes;
bytes = temp.Receive(recvBytes, recvBytes.Length, 0);
recvStr += Encoding.UTF8.GetString(recvBytes, 0, bytes);
Console.WriteLine("3. Server Get Message:{0}", recvStr);
string sendStr = "Ok!Client send message sucessful!";
byte[] bs = Encoding.UTF8.GetBytes(sendStr);
temp.Send(bs, bs.Length, 0);
temp.Close();
Console.WriteLine("4. Completed...");
Console.WriteLine("-----------------------------------------------------------------------");
Console.WriteLine("");
}
catch (ArgumentNullException e)
{
Console.WriteLine("ArgumentNullException: {0}", e);
}
catch (SocketException e)
{
Console.WriteLine("SocketException: {0}", e);
}
}
}
}
}
效果: 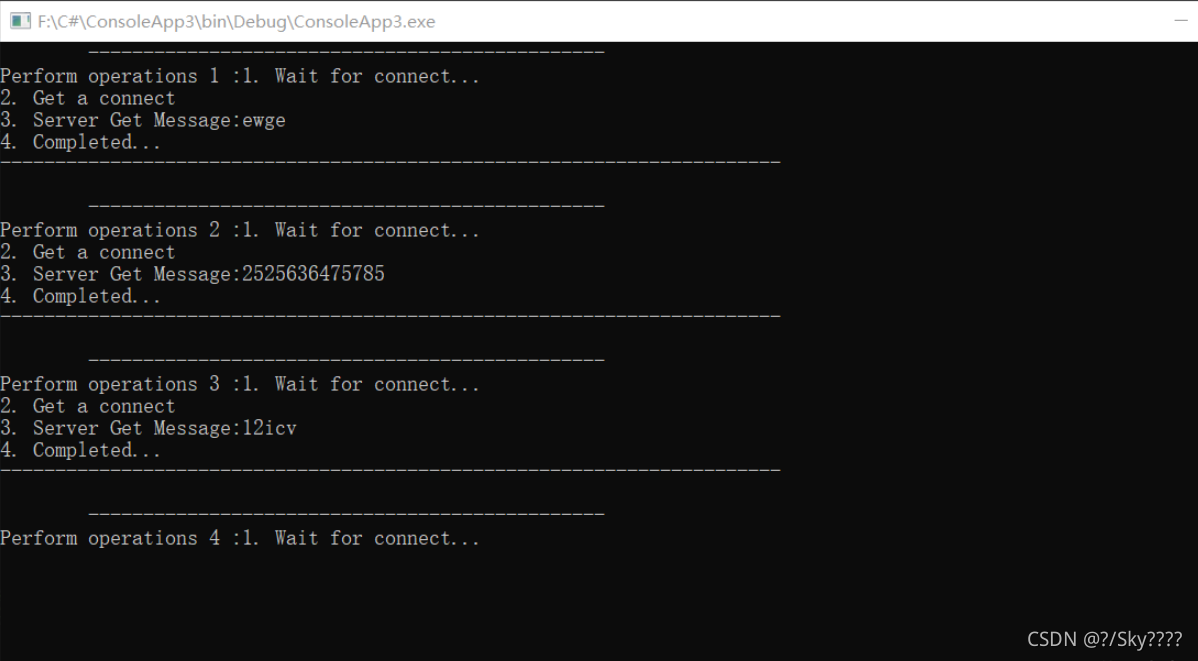
参考
https://blog.csdn.net/m0_51120713/article/details/121408110
|