简介
我们以前写的 :java web 是 B/S 浏览器 和 服务端
现在这是 网络编程 是 C/S 客户端和服务端
实现网络通信的要素
ip
端口号
网络通讯协议 :重点是 TCP/IP
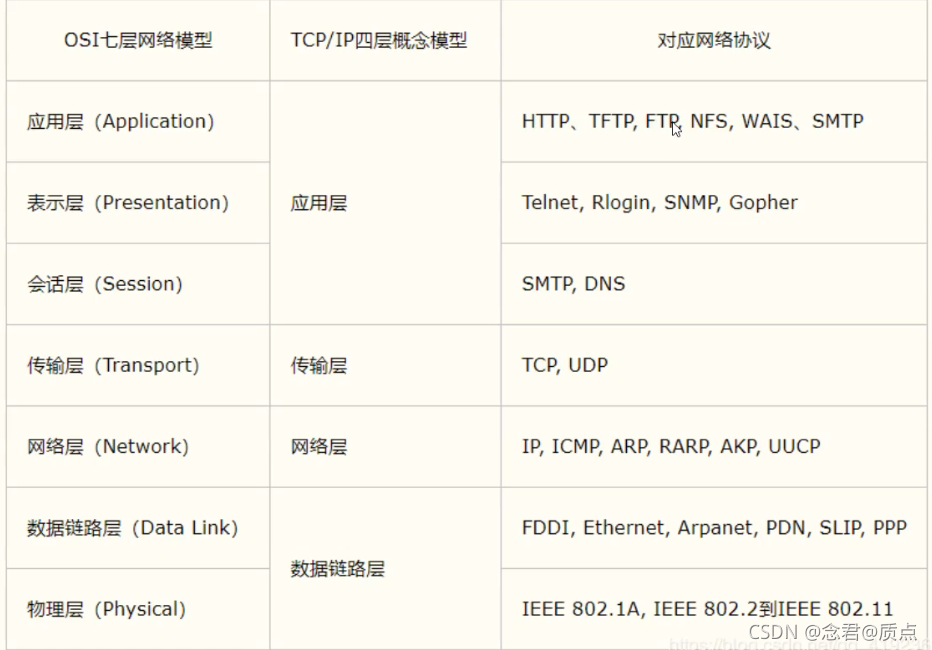
小结 :
网络编程中怎么定位别人的 ip
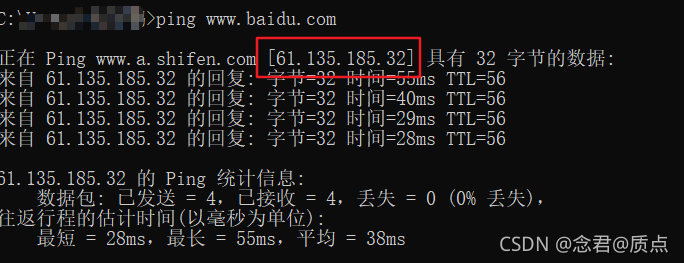
网络编程的要素:
IP 地址和端口号
网络通讯协议 TCP、UDP
IP地址
IP地址 可以 定位一台网络计算机
IP地址的分类 :
IPV4 / IPV6
公网(互联网)- 私网(局域网 一般是 192.168.xxx.xxx)
ABCD 类 ip 地址 (了解)
IPV4 就是127.0.0.1 ,由 4 个字节组成,每个字节是 0-255 ,一共有42亿个
IPV6 就是 8个无符号整数 组成 :fe80::a464:1d9b:867:79c4%3
测试 IP 的一些方法
public static void main(String[] args) throws Exception {
InetAddress address1 = InetAddress.getByName("127.0.0.1");
InetAddress address2 = InetAddress.getByName("localhost");
InetAddress address3 = InetAddress.getLocalHost();
InetAddress address4 = InetAddress.getByName("www.baidu.com");
System.out.println(address1);
System.out.println(address2);
System.out.println(address3);
System.out.println(address4);
System.out.println(address4.getHostAddress());
System.out.println(address4.getHostName());
}
端口
端口表示计算机上的一个程序的进程 ;
不同的进程有不同的端口号,用来区分软件
端口号被规定 0 ~ 65535
TCP 和 UDP :65535*2 ,单个协议下,端口不能冲突
端口的分类 :
共有端口 :0 - 1023
HTTP :80 例如 :http://www.baidu.com/ 就相当于 http://www.baidu.com:80/
HTTPS :443 例如 :https://www.baidu.com/ 就相当于 https://www.baidu.com:443/
FTP : 21
Telent : 23
程序注册的端口 :1024 - 49151
tomcat :8080
mysql :3306
动态 、私有 :49152 - 65535
netstat -ano
netstat -ano|findstr "8080"
tasklist|findstr "8540"
测试方法
public static void main(String[] args) {
InetSocketAddress socketAddress = new InetSocketAddress("127.0.0.1", 8080);
System.out.println(socketAddress);
System.out.println(socketAddress.getAddress());
System.out.println(socketAddress.getHostName());
System.out.println(socketAddress.getHostString());
System.out.println(socketAddress.getPort());
}
通信协议
协议 :就是约定
网络通信协议 包含 :速率、传输码率、代码结构…
TCP 与 UDP 对比 :
TCP : 用户传输协议(打电话)
-
连接稳定 -
三次握手,四次挥手 -
客户端、服务端 -
传输完成,释放连接,效率低
UDP : 用户数据报协议(发短信)
- 不连接不稳定
- 客户端、服务端(没有明确的界限)
- 不管有没有准备好,都可以发给你
- 类似导弹
- DDOS :洪水攻击(饱和攻击)
TCP 实现聊天
客户端
import java.io.IOException;
import java.io.OutputStream;
import java.net.InetAddress;
import java.net.Socket;
public class TcpClient1 {
public static void main(String[] args) {
Socket socket =null;
OutputStream os =null;
try {
InetAddress serverIP = InetAddress.getByName("127.0.0.1");
int port = 9999;
socket = new Socket(serverIP, port);
os = socket.getOutputStream();
os.write("Hello World !!!".getBytes());
} catch (Exception e) {
e.printStackTrace();
}finally {
if(os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
服务端
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.ServerSocket;
import java.net.Socket;
public class TcpServer {
public static void main(String[] args) {
ServerSocket serverSocket = null;
Socket socket = null;
InputStream is = null;
ByteArrayOutputStream baos = null;
try {
serverSocket = new ServerSocket(9999);
socket = serverSocket.accept();
is = socket.getInputStream();
baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = is.read(buffer)) != -1) {
baos.write(buffer, 0, len);
}
System.out.println(baos.toString());
} catch (IOException e) {
e.printStackTrace();
} finally {
if (baos != null) {
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket != null) {
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (serverSocket != null) {
try {
serverSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
测试结果 :服务端成功收到消息
注意 :启动时先启动服务端在启动客户端,因为服务端是等待连接的一端
TCP 实现文件上传
public static void main(String[] args) throws Exception {
Socket socket = new Socket(InetAddress.getByName("127.0.0.1"), 9000);
OutputStream os = socket.getOutputStream();
FileInputStream fis = new FileInputStream(new File("java.jpg"));
byte[] buffer = new byte[1024];
int len;
while((len=fis.read(buffer))!=-1){
os.write(buffer,0,len);
}
fis.close();
os.close();
socket.close();
}
public static void main(String[] args) throws Exception {
ServerSocket serverSocket = new ServerSocket(9000);
Socket socket = serverSocket.accept();
InputStream is = socket.getInputStream();
FileOutputStream fos = new FileOutputStream(new File("receive.jpg"));
byte[] buffer = new byte[1024];
int len;
while ((len = is.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
fos.close();
is.close();
socket.close();
serverSocket.close();
}
UDP 发送消息
UDP 没有 服务端和 客户端的概念,不需要建立服务端,这里为了能够详细的说明,通过两个客户端来说明。
客户端1 :
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket();
String msg = "你好";
InetAddress byName = InetAddress.getByName("127.0.0.1");
int port = 9090;
DatagramPacket packet = new DatagramPacket(msg.getBytes(), 0, msg.getBytes().length, byName, port);
socket.send(packet);
socket.close();
}
客户端2 :
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket(9090);
byte[] buffer = new byte[1024];
DatagramPacket packet = new DatagramPacket(buffer, 0, buffer.length);
socket.receive(packet);
String msg = new String(packet.getData(),0,packet.getLength());
System.out.println(msg);
socket.close();
}
UDP 实现聊天
发送方
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket(8888);
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
while(true){
String data = reader.readLine();
byte[] datas = data.getBytes();
InetSocketAddress address = new InetSocketAddress("127.0.0.1", 6666);
DatagramPacket packet = new DatagramPacket(datas, 0, datas.length, address);
socket.send(packet);
if(data.equals("bye")){
break;
}
}
socket.close();
}
接收方
public static void main(String[] args) throws Exception{
DatagramSocket socket = new DatagramSocket(6666);
while(true){
byte[] container = new byte[1024];
DatagramPacket packet = new DatagramPacket(container, 0, container.length);
socket.receive(packet);
byte[] data = packet.getData();
String datas = new String(data,0,data.length);
System.out.println(datas);
if(datas.equals("bye")){
break;
}
}
socket.close();
}
URL
例如 :百度的 URL 是 http://www.baidu.com
URL : (统一资源定位符):定位互联网上的资源
DNS :域名解析
public static void main(String[] args) throws MalformedURLException {
URL url = new URL("http://localhost:8080/HelloWorld/hello.jps?username=root&password=admin");
System.out.println(url.getProtocol());
System.out.println(url.getHost());
System.out.println(url.getPort());
System.out.println(url.getPath());
System.out.println(url.getFile());
System.out.println(url.getQuery());
}
URL 下载资源
public static void main(String[] args) throws Exception {
URL url = new URL("https://s2.music.126.net/style/web2/img/foot_enter_tt.png?aa2b21084026ebae4709cea7b5d5c06a");
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
InputStream inputStream = urlConnection.getInputStream();
FileOutputStream fos = new FileOutputStream("ot_enter_tt.png");
byte[] buffer = new byte[1024];
int len;
while ((len=inputStream.read(buffer))!=-1){
fos.write(buffer,0,len);
}
fos.close();
inputStream.close();
urlConnection.disconnect();
}
|