Servlet中的三大域对象
共同的功能
存值/取值
getAttribute 存值
setAttribute 取值
1.request 请求域
使用范围: 一次请求
@WebServlet("/as")
public class AServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.setAttribute("salary",10000);
}
}
@WebServlet("/bs")
public class BServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
Object salary = req.getAttribute("salary");
System.out.println(salary);
}
}
2.session 会话域
使用范围: 多次请求
@WebServlet("/cs")
public class CServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("====在cs中向session域存值====");
req.getSession().setAttribute("salary",1000000);
}
}
@WebServlet("/ds")
public class DServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("====在ds中从session域取值====");
Object salary = req.getSession().getAttribute("salary");
System.out.println(salary);
}
}
3.application 全局域
使用范围:与服务器相同寿命
@WebServlet("/es")
public class EServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("====在es中向application域存值====");
req.getSession().getServletContext().setAttribute("salary",123456);
}
}
@WebServlet("/fs")
public class FServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("====在fs中从application域中取值====");
Object salary = req.getSession().getServletContext().getAttribute("salary");
System.out.println(salary);
}
}
去浏览器输入:
     
输出的结果:
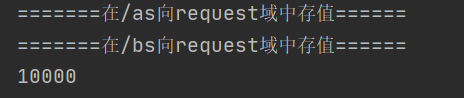 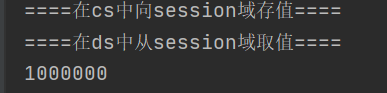 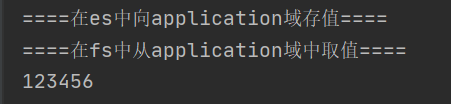 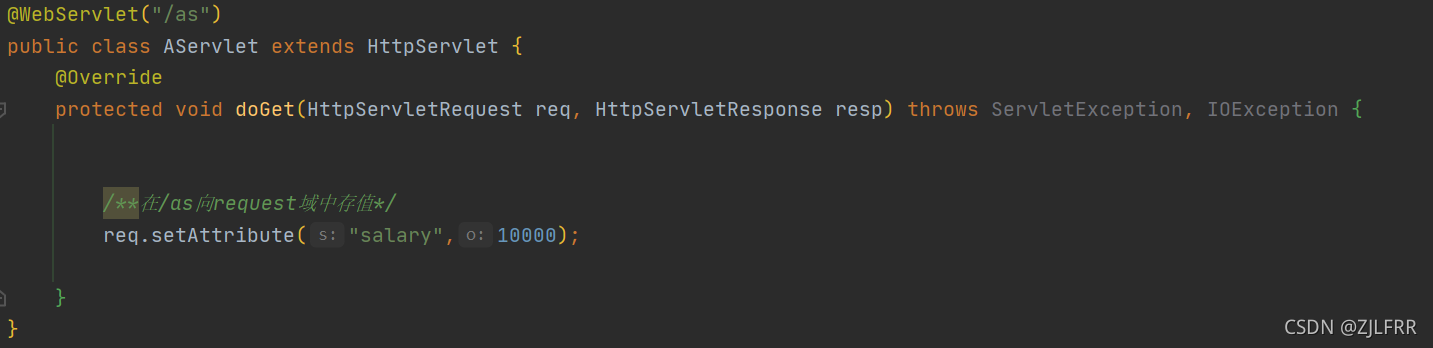
发现:
request只能用一次
session在网页没有关闭的时候可以一直使用,但关闭网页就不可以
application在网页上可以一直使用,浏览器从新打开也可以继续使用,直到关闭服务器不可以使用
|