网络编程概述
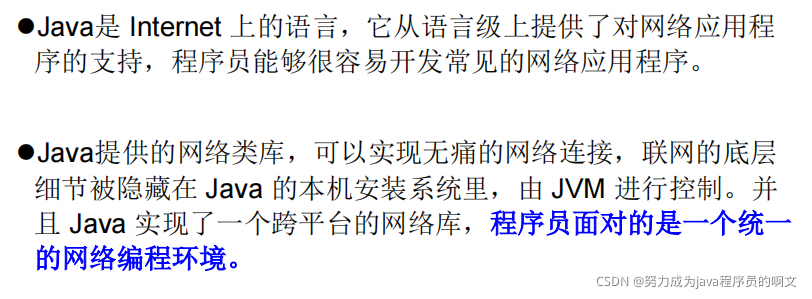 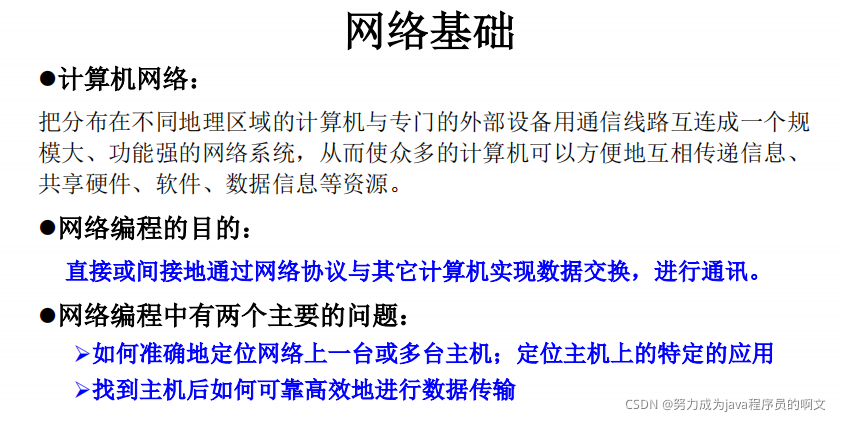
网络通信要素概述
IP 和端口号 网络通信协议 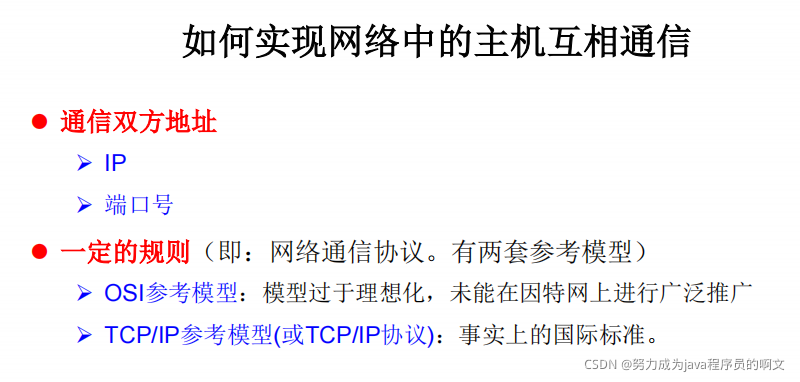 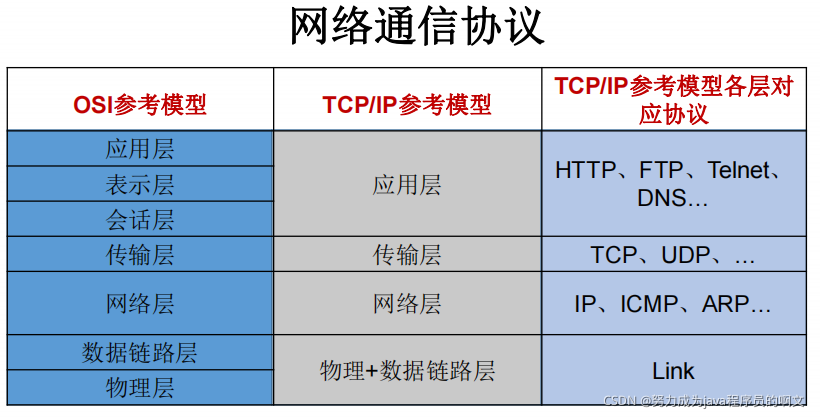 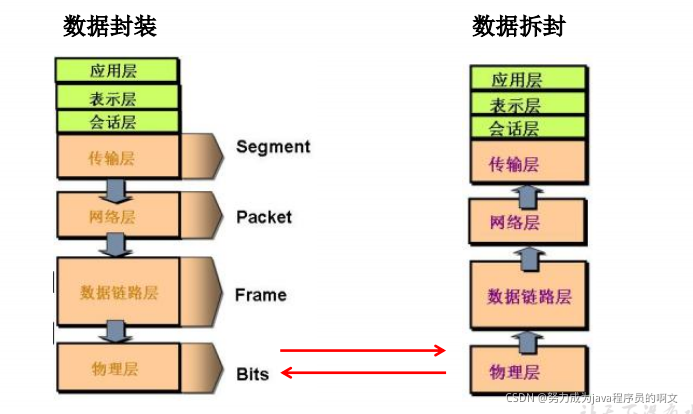
一、网络编程中有两个主要的问题:
1、如何准确地定位网络上一台或多台主机:定位主机上特定的应用
2、找到主机后如何可靠高效的进行数据传输
二、网络编程中的两个要素:
1、对应问题一:IP 和端口号
2、对应问题二、提供网络通信协议:TCP/IP 参考模型(应用层、传输层、网络层、物理+数据链路层)
通信要素1:IP和端口号
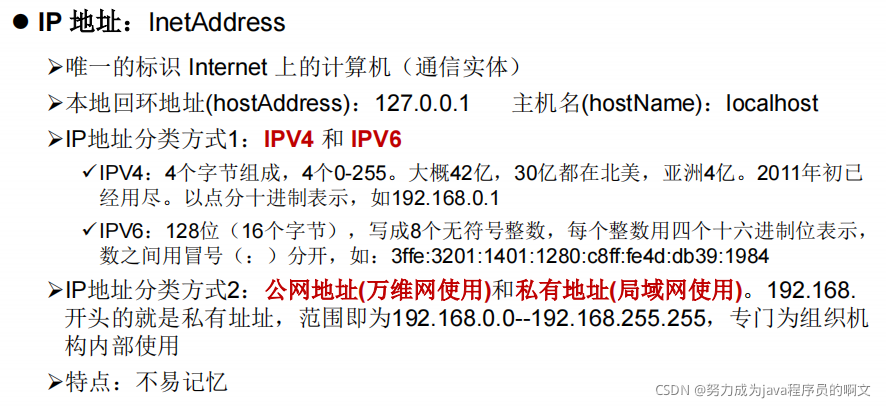 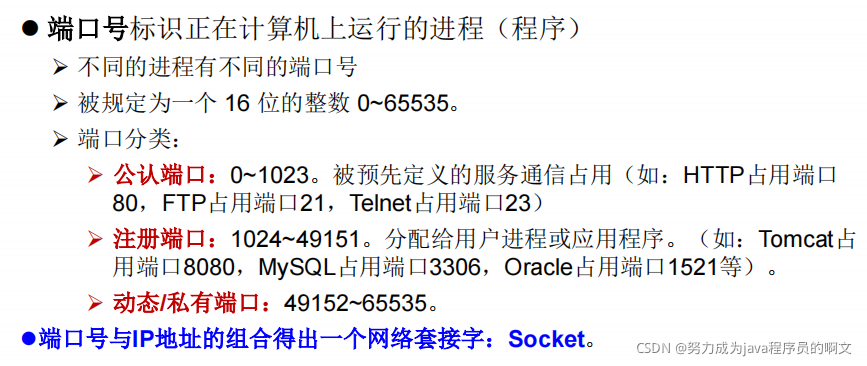 同一台主机上的两个不同的进程不能使用相同的端口号。
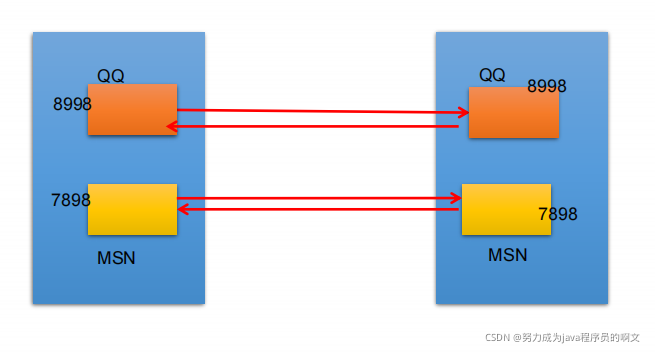 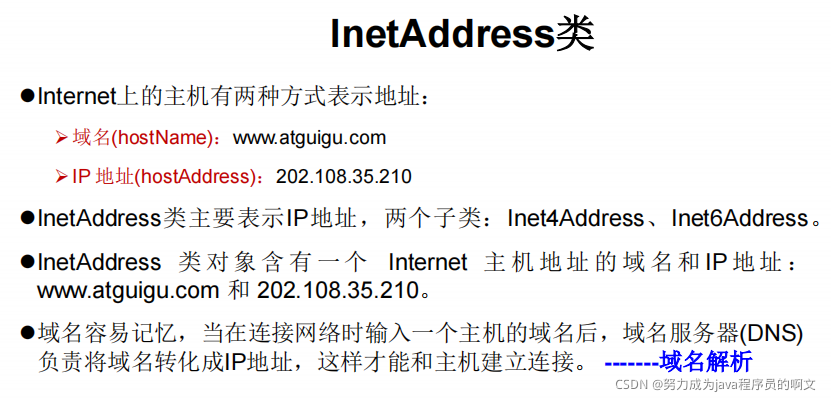 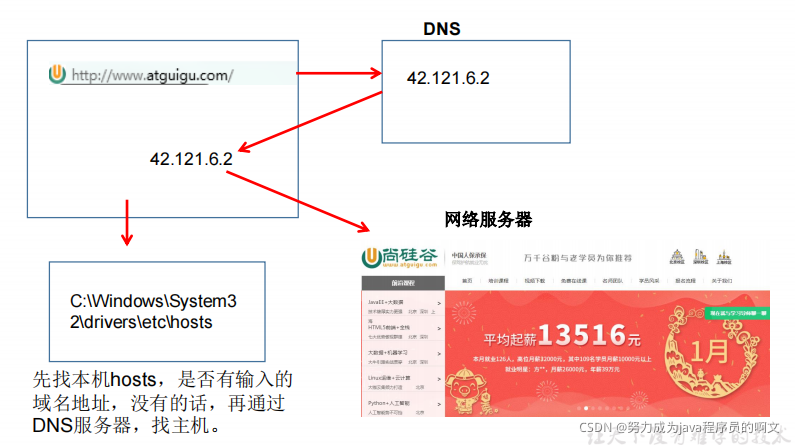
InetAdress 类
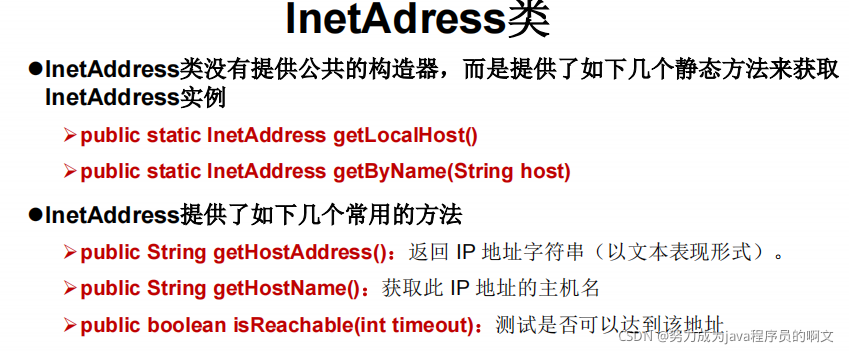
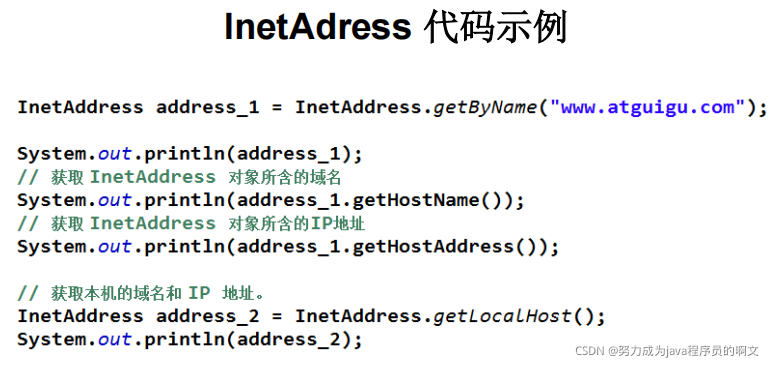
通信要素一:IP和端口号
1、IP:唯一的标识 Internet 上的计算机(通信实体)
2、在java中使用 InetAddress 类代表IP
3、IP的分类:IPv4和IPv6;万维网和局域网
4、域名:www.baidu.com www.mi.com DNS域名解析
5、本地贿赂地址:127.0.0.1 对应着:localhost
6、如何实例化 InetAddress : 两个方法
getByName(String host)
getLocalHost()
两个常用方法:getHostName() / getHostAddress()
7、端口号:标识正在计算机上运行的进程(程序)
要求:不同的进程有不同的端口号
范围:被规定为一个 16 位的整数 0~65535。
8、端口号与IP地址的组合得出了一个网络套接字:Socket
import java.net.InetAddress;
import java.net.UnknownHostException;
public class InetAddressTest {
public static void main(String[] args) {
try {
InetAddress int1 = InetAddress.getByName("192.168.163.138");
System.out.println(int1);
InetAddress int2 = InetAddress.getByName("www.baidu.com");
System.out.println(int2);
InetAddress localHost1 = InetAddress.getByName("127.0.0.1");
System.out.println(localHost1);
InetAddress localHost2 = InetAddress.getLocalHost();
System.out.println();
InetAddress jd = InetAddress.getByName("www.jd.com");
System.out.println(jd.getHostName());
System.out.println(jd.getHostAddress());
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
}
通信要素2:网络协议
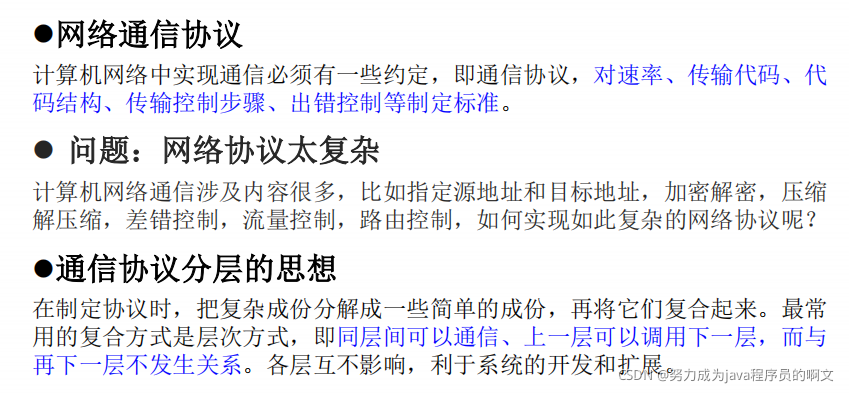 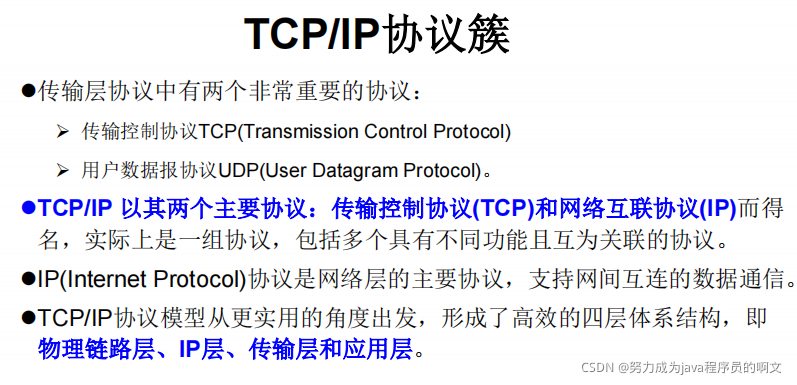 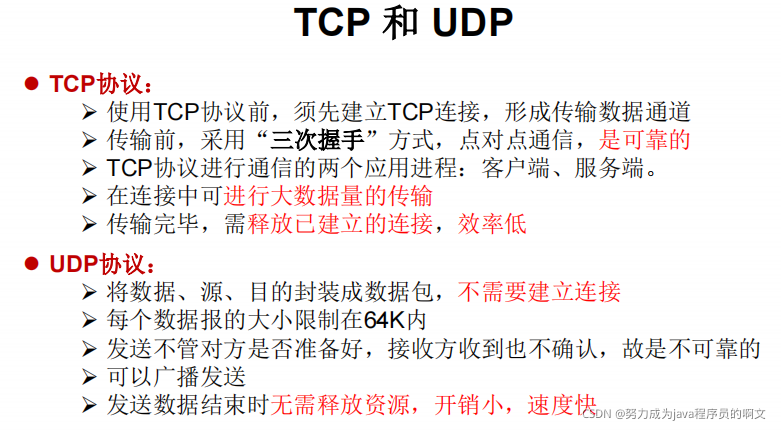 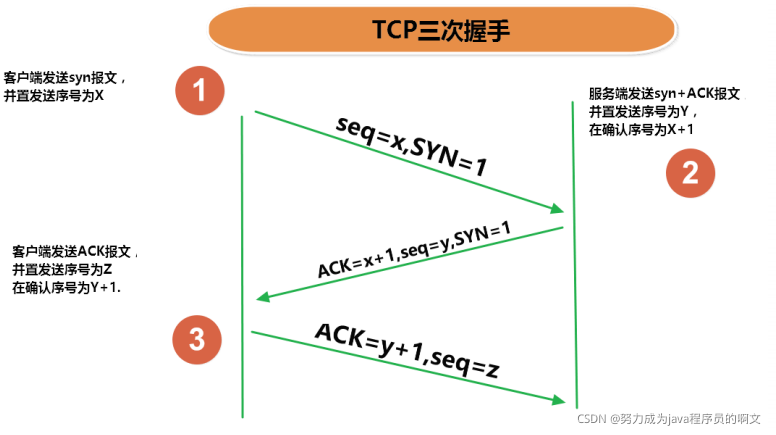 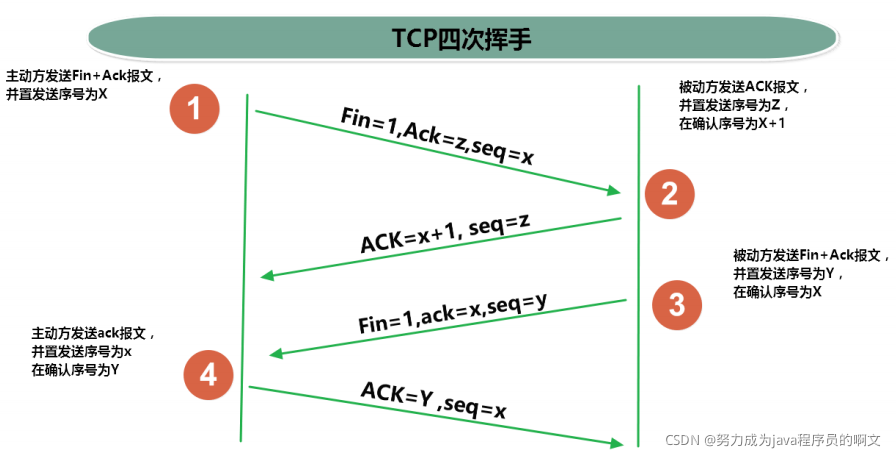
Socket
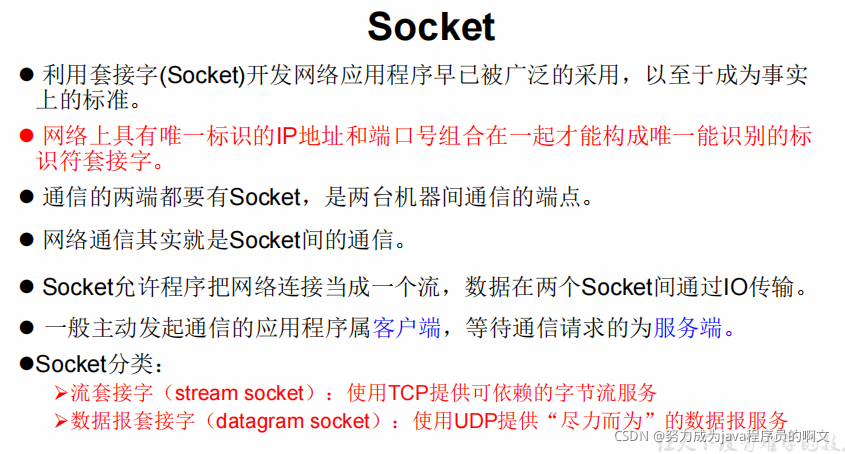 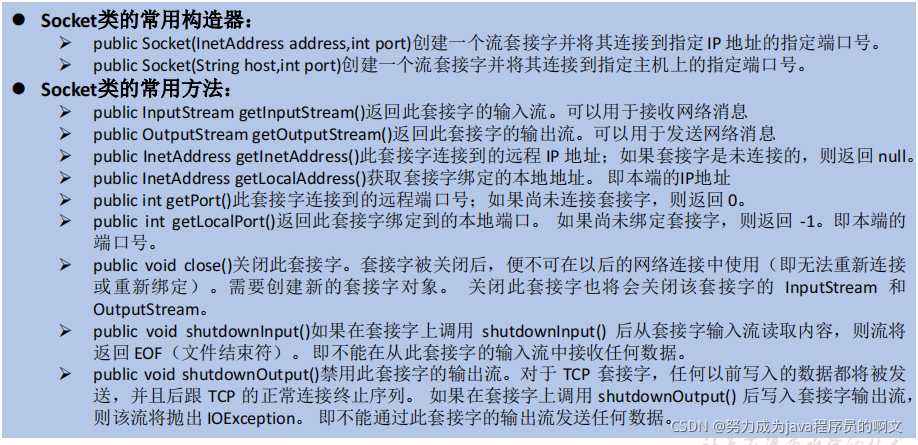
TCP网络编程
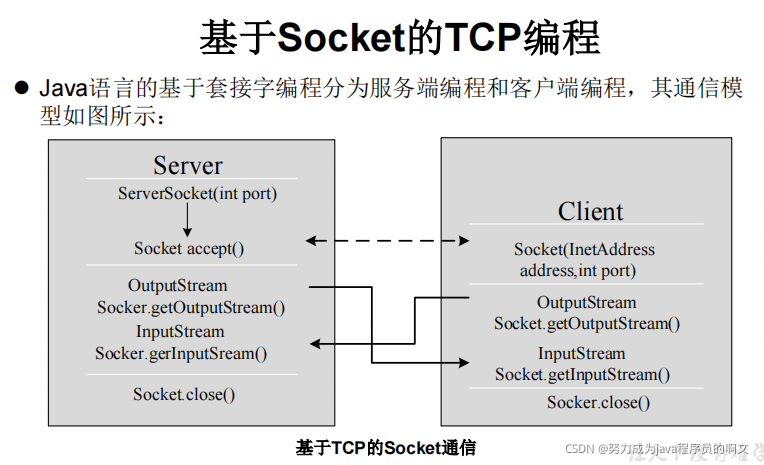 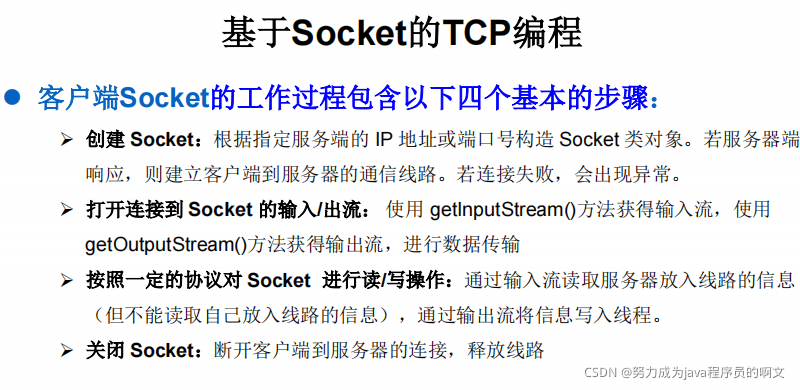 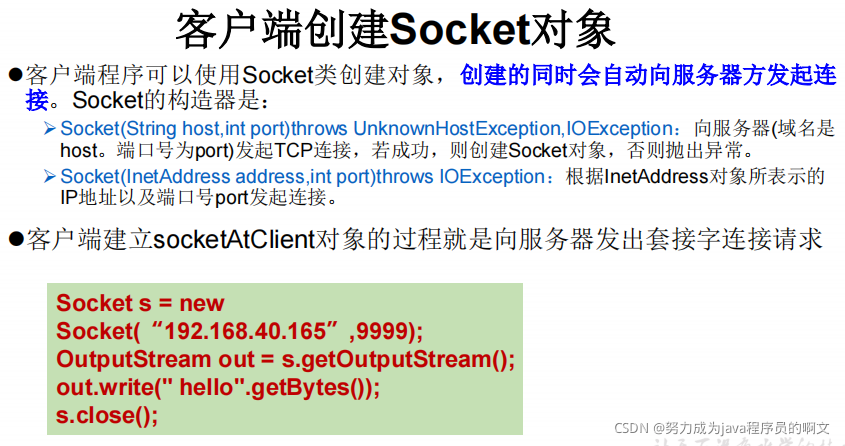 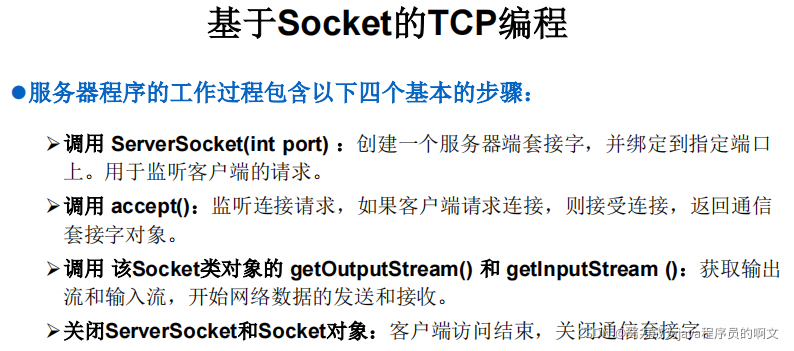 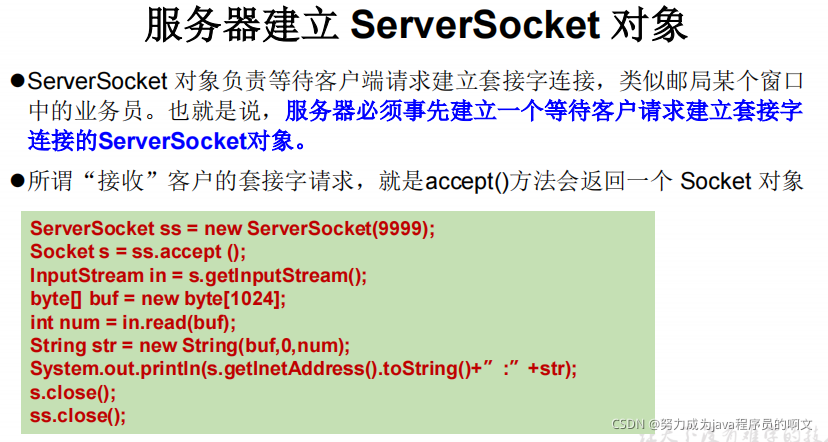 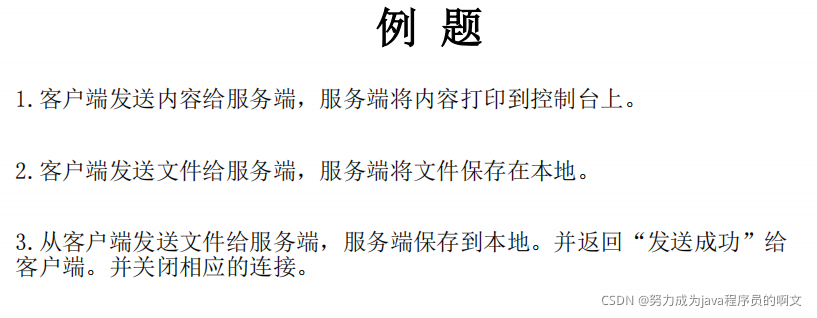
例子1:客户端发送信息给服务端,服务端将数据信息显示在控制台上
import org.junit.jupiter.api.Test;
import java.io.*;
import java.net.InetAddress;
import java.net.ServerSocket;
import java.net.Socket;
public class TCPTest1 {
@Test
public void client(){
Socket socket = null;
OutputStream os = null;
try {
InetAddress inet = InetAddress.getByName("127.0.0.1");
socket = new Socket(inet,8899);
os = socket.getOutputStream();
os.write("你好,这是客户端".getBytes());
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (os != null) {
os.close();
}
} catch (IOException e) {
e.printStackTrace();
}
try {
if (socket != null) {
socket.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Test
public void server() {
ServerSocket ss = null;
Socket socket = null;
InputStream is = null;
ByteArrayOutputStream baos = null;
try {
ss = new ServerSocket(8899);
socket = ss.accept();
is = socket.getInputStream();
baos = new ByteArrayOutputStream();
byte[] buffer = new byte[5];
int len;
while((len=is.read(buffer))!=-1){
baos.write(buffer,0,len);
}
System.out.println(baos.toString());
System.out.println("收到了来自于:"+socket.getInetAddress().getHostAddress()+"的数据");
} catch (IOException e) {
e.printStackTrace();
} finally {
if (baos != null) {
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket != null) {
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (ss != null) {
try {
ss.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
例题2:客户端发送文件给服务端,服务端将文件保存在本地。
import org.junit.jupiter.api.Test;
import java.io.*;
import java.net.InetAddress;
import java.net.ServerSocket;
import java.net.Socket;
public class TCPTest2 {
@Test
public void client() {
OutputStream os = null;
FileInputStream fis = null;
try {
Socket socket = new Socket(InetAddress.getByName("127.0.0.1"),9090);
os = socket.getOutputStream();
fis = new FileInputStream("1.jpg");
byte[] buffer = new byte[1024];
int len;
while((len=fis.read(buffer))!=-1){
os.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fis != null) {
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (os != null) {
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void server() {
ServerSocket ss = null;
Socket accept = null;
InputStream is = null;
FileOutputStream fos = null;
try {
ss = new ServerSocket(9090);
accept = ss.accept();
is = accept.getInputStream();
fos = new FileOutputStream("2.jpg");
byte[] buffer = new byte[5];
int len;
while((len=is.read(buffer))!=-1){
fos.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (ss != null) {
try {
ss.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (accept != null) {
try {
accept.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
例题3:从客户端发送文件给服务端,服务端保存到本地。并返回“发送成功”给客户端。并关闭相应的连接。
import org.junit.jupiter.api.Test;
import java.io.*;
import java.net.InetAddress;
import java.net.ServerSocket;
import java.net.Socket;
public class TCPTest3 {
@Test
public void client() {
OutputStream os = null;
FileInputStream fis = null;
ByteArrayOutputStream baos=null;
try {
Socket socket = new Socket(InetAddress.getByName("127.0.0.1"),9090);
os = socket.getOutputStream();
fis = new FileInputStream("1.jpg");
byte[] buffer = new byte[1024];
int len;
while((len=fis.read(buffer))!=-1){
os.write(buffer,0,len);
}
socket.shutdownOutput();
InputStream is = socket.getInputStream();
baos = new ByteArrayOutputStream();
byte[] buffer2 = new byte[1024];
int len2;
while ((len2=is.read(buffer2))!=-1){
baos.write(buffer2,0,len2);
}
System.out.println(baos.toString());
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fis != null) {
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (os != null) {
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (baos != null) {
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void server() {
ServerSocket ss = null;
Socket socket = null;
InputStream is = null;
FileOutputStream fos = null;
OutputStream os=null;
try {
ss = new ServerSocket(9090);
socket = ss.accept();
is = socket.getInputStream();
fos = new FileOutputStream("2.jpg");
byte[] buffer = new byte[5];
int len;
while((len=is.read(buffer))!=-1){
fos.write(buffer,0,len);
}
os = socket.getOutputStream();
os.write("文件已接收".getBytes());
} catch (IOException e) {
e.printStackTrace();
} finally {
if (is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (ss != null) {
try {
ss.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket != null) {
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (os != null) {
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
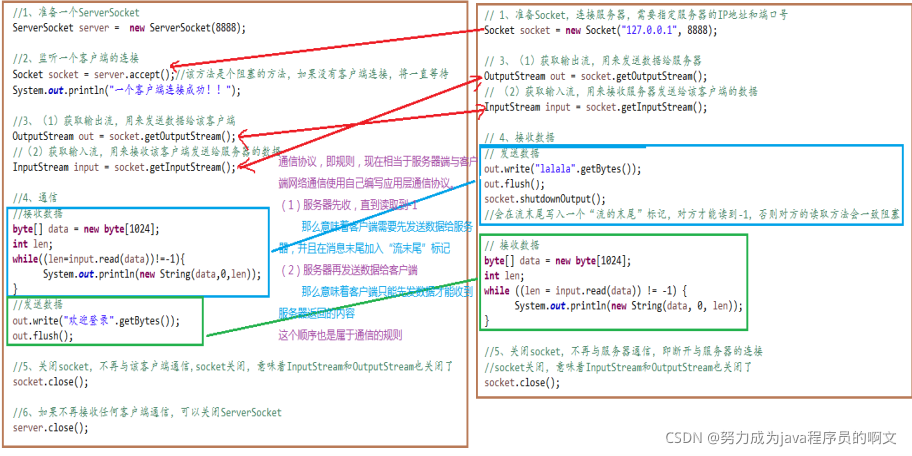 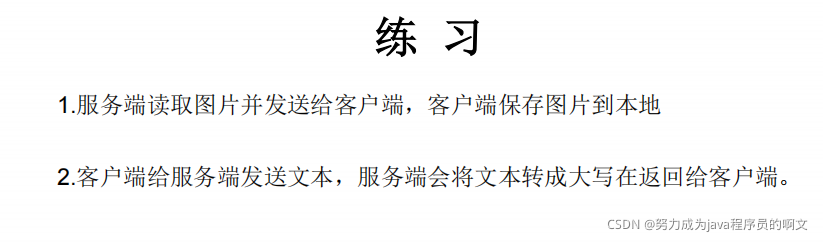
import java.io.*;
import java.net.InetAddress;
import java.net.ServerSocket;
import java.net.Socket;
public class TCPTest4 {
@Test
public void client() throws IOException {
Socket socket = new Socket(InetAddress.getByName("127.0.0.1"),9988);
InputStream is = socket.getInputStream();
FileOutputStream fos = new FileOutputStream("3.jpg");
byte[] buffer = new byte[20];
int len;
while((len=is.read(buffer))!=-1){
fos.write(buffer,0,len);
}
OutputStream os = socket.getOutputStream();
os.write("文件已收到".getBytes());
socket.close();
is.close();
fos.close();
os.close();
}
@Test
public void server() throws IOException {
ServerSocket ss = new ServerSocket(9988);
Socket socket = ss.accept();
FileInputStream fis= new FileInputStream("1.jpg");
OutputStream os = socket.getOutputStream();
byte[] buffer = new byte[20];
int len;
while((len=fis.read(buffer))!=-1){
os.write(buffer,0,len);
}
socket.shutdownOutput();
InputStream is = socket.getInputStream();
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] buffer2 = new byte[20];
int len2;
while((len2=is.read(buffer2))!=-1){
baos.write(buffer2,0,len2);
}
System.out.println(baos.toString());
ss.close();
socket.close();
fis.close();
os.close();
is.close();
baos.close();
}
}
import org.junit.jupiter.api.Test;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.InetAddress;
import java.net.ServerSocket;
import java.net.Socket;
public class TCPTest5 {
@Test
public void client() throws IOException {
Socket socket = new Socket(InetAddress.getByName("127.0.0.1"),9990);
OutputStream os = socket.getOutputStream();
os.write("abcdefghijklmm".getBytes());
socket.shutdownOutput();
InputStream is = socket.getInputStream();
byte[] buffer = new byte[20];
int len;
ByteArrayOutputStream baos = new ByteArrayOutputStream();
while ((len=is.read(buffer))!=-1){
baos.write(buffer,0,len);
}
System.out.println(baos.toString());
socket.close();
os.close();
is.close();
baos.close();
}
@Test
public void server() throws IOException {
ServerSocket ss = new ServerSocket(9990);
Socket socket = ss.accept();
InputStream is = socket.getInputStream();
byte[] buffer = new byte[20];
int len;
ByteArrayOutputStream baos = new ByteArrayOutputStream();
while ((len=is.read(buffer))!=-1){
baos.write(buffer,0,len);
}
String str = baos.toString().toUpperCase();
OutputStream os = socket.getOutputStream();
os.write(str.getBytes());
ss.close();
socket.close();
is.close();
baos.close();
os.close();
}
}
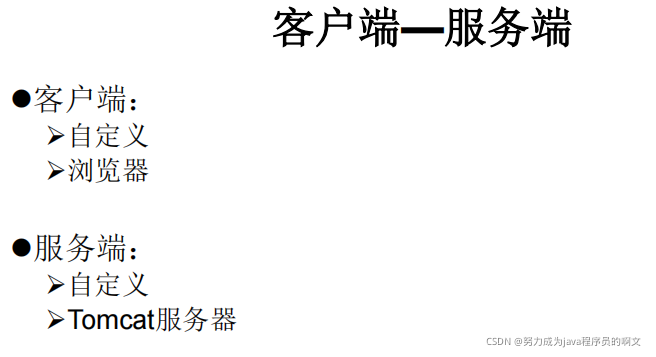
UDP网络编程
UDP 是一种不可靠链接 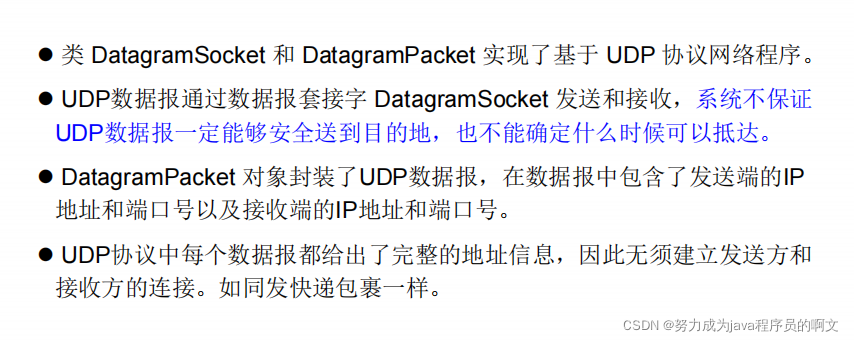 UDP协议:数据报限定为64kb,效率高但不可靠。 TCP协议:进行大量数据传输,效率低但可靠。
DatagramSocket 类的常用方法
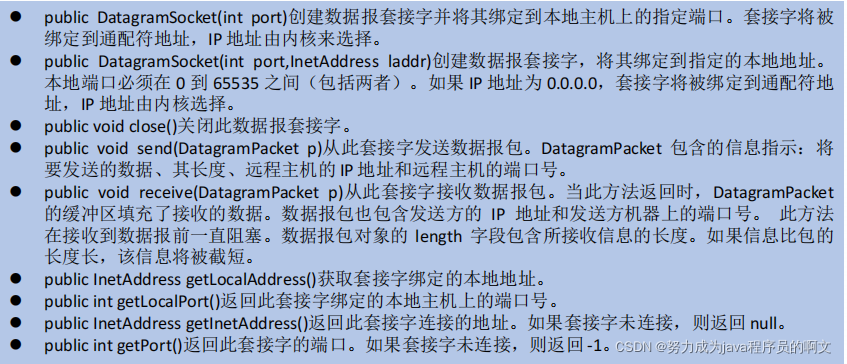
DatagramPacket类的常用方法
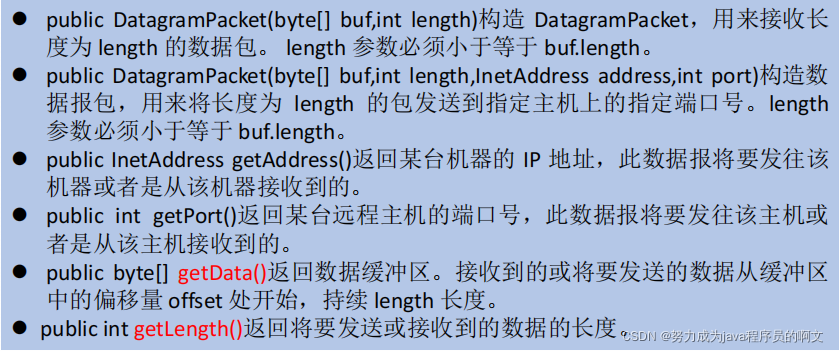
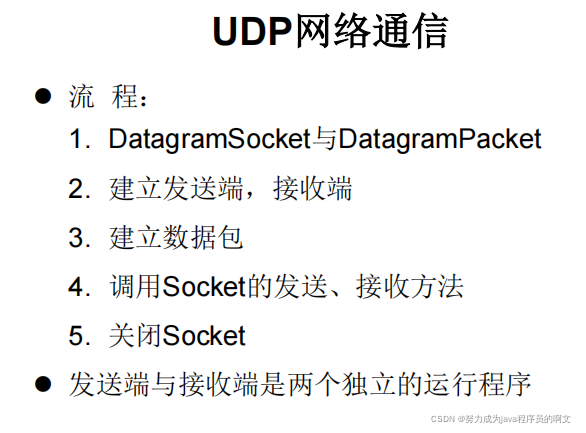
import org.junit.jupiter.api.Test;
import java.io.IOException;
import java.net.*;
public class UDPTest {
@Test
public void sender() throws IOException {
DatagramSocket socket = new DatagramSocket();
String str = "UDP 方式发送数据!";
byte[] data = str.getBytes();
InetAddress inet = InetAddress.getLocalHost();
DatagramPacket packet = new DatagramPacket(data,0,data.length,inet,9090);
socket.send(packet);
socket.close();
}
@Test
public void receiver() throws IOException {
DatagramSocket socket = new DatagramSocket(9090);
byte[] buffer = new byte[100];
DatagramPacket packet = new DatagramPacket(buffer,0,buffer.length);
socket.receive(packet);
System.out.println(new String(packet.getData(),0,packet.getLength()));
socket.close();
}
}
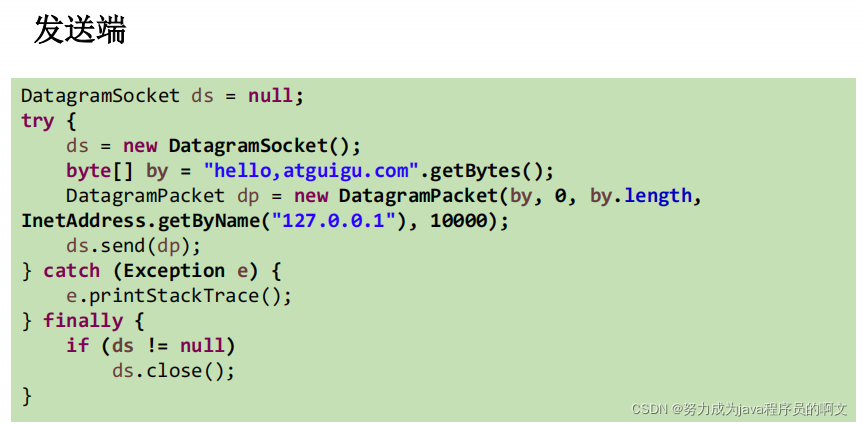 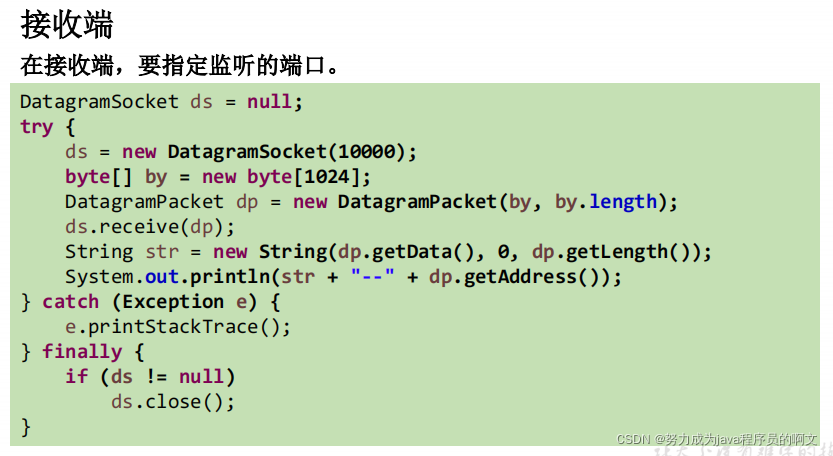
URL编程
URL类
URL:统一资源定位符 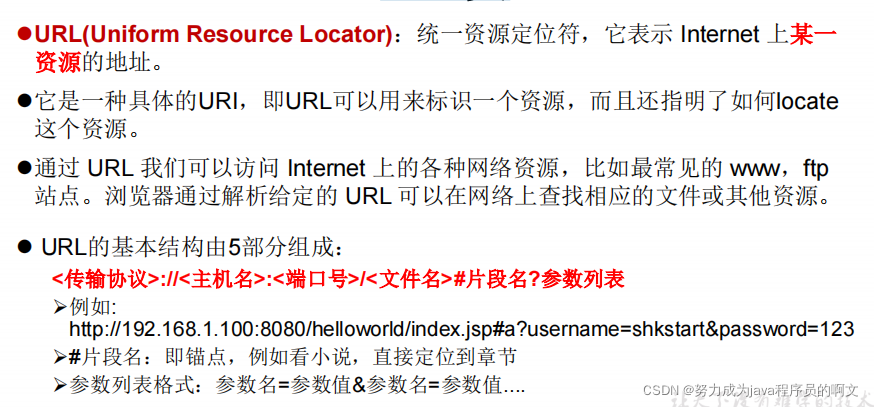 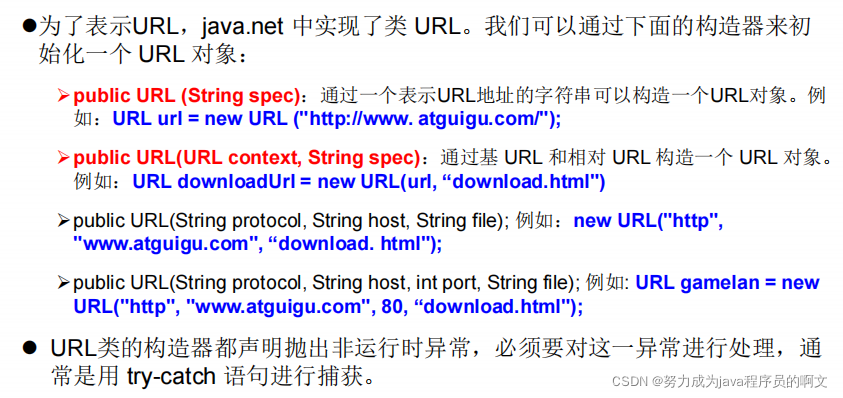 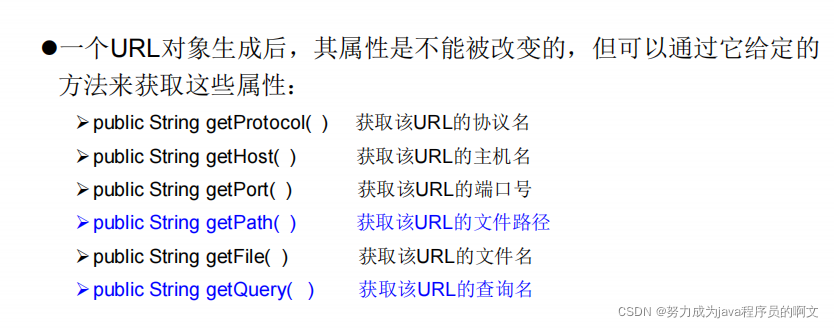
import java.net.MalformedURLException;
import java.net.URL;
public class URLTest {
public static void main(String[] args) {
try {
URL url = new URL("https://localhost:8080//workspace//InetAddressTest//1.jpg");
System.out.println(url.getProtocol());
System.out.println(url.getHost());
System.out.println(url.getPort());
System.out.println(url.getPath());
System.out.println(url.getFile());
System.out.println(url.getQuery());
} catch (MalformedURLException e) {
e.printStackTrace();
}
}
}
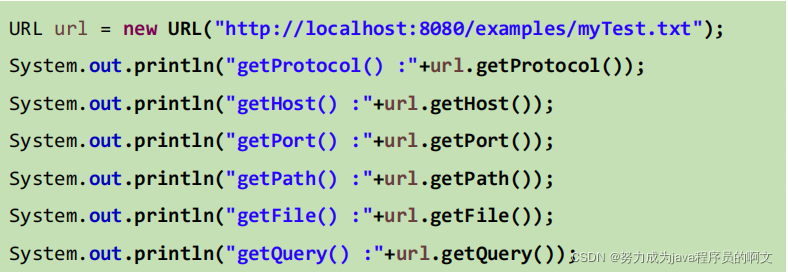
针对HTTP协议的URLConnection类
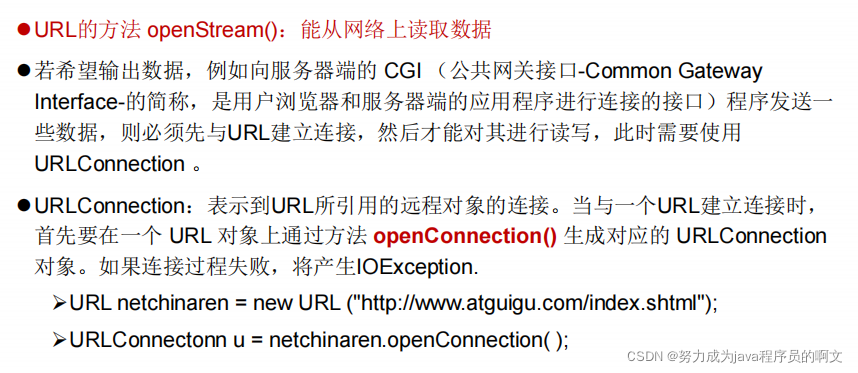 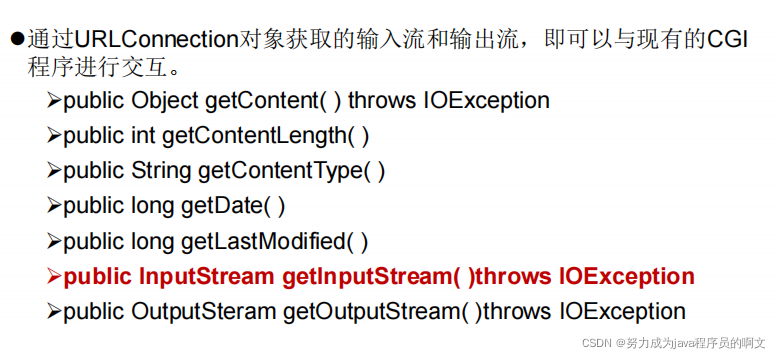
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLConnection;
public class URLTest2 {
public static void main(String[] args) {
HttpURLConnection urlConnection = null;
InputStream is = null;
FileOutputStream fos = null;
try {
URL url = new URL("https://scpic.chinaz.net/files/pic/pic9/202009/apic27858.jpg");
urlConnection = (HttpURLConnection) url.openConnection();
urlConnection.connect();
is = urlConnection.getInputStream();
fos = new FileOutputStream("5.jpg");
byte[] buffer = new byte[1024];
int len;
while((len = is.read(buffer))!=-1){
fos.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (urlConnection != null) {
urlConnection.disconnect();
}
}
}
}
URI、URL和URN的区别
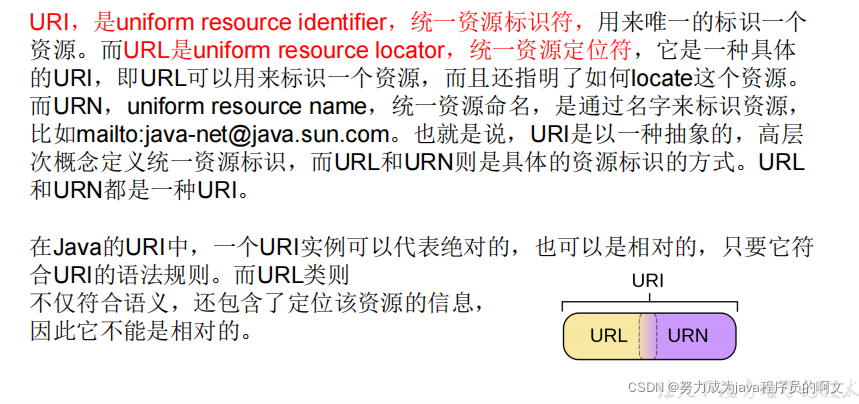
小 结
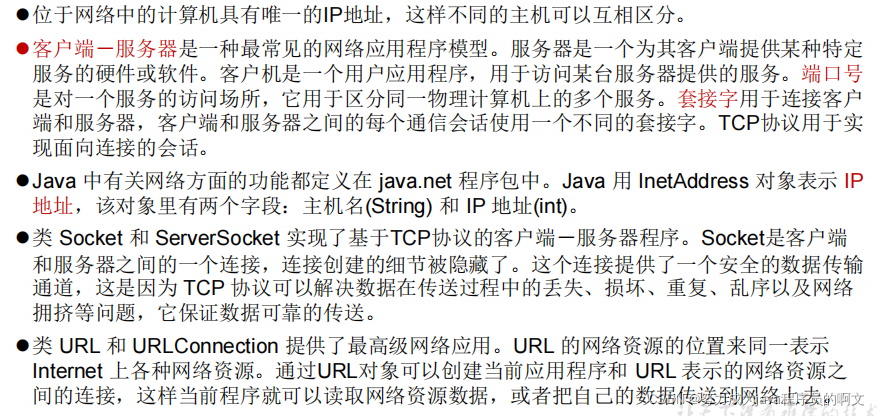
|