grpc学习笔记
在python中使用
安装
conda create -n grpc python=3.8
conda activate grpc
pip install grpcio
// 其中有一些插件用来利用.proto文件生成server和client,以及protoc编译器
pip install grpcio-tools
cd PycharmProjects
git clone -b v1.42.0 git@github.com:grpc/grpc
gRPC
位于不同设备,不同语言开发的client程序可以调用server的方法
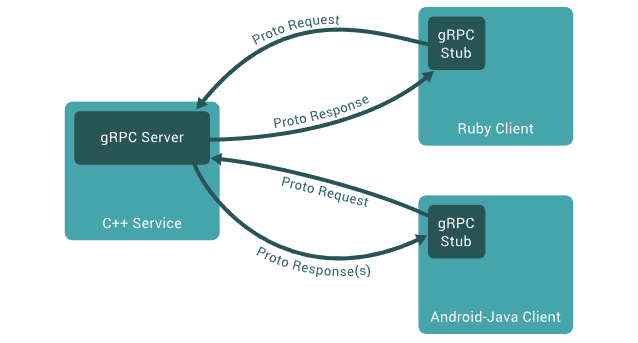
protocol buffer
用来序列化结构数据
首先需要在.proto文件里面定义想要序列化的数据,用message定义
message Person {
string name = 1;
int32 id = 2;
bool has_ponycopter = 3;
}
之后使用protoc编译器来根据该文件生成对应的语言写出的类,类中包含简单的setter和getter以及序列化,反序列化的方法
然后在.proto文件中还要定义service,服务的参数和返回都用message来定义
// The greeter service definition.
service Greeter {
// Sends a greeting
rpc SayHello (HelloRequest) returns (HelloReply) {}
// Sends another greeting
rpc SayHelloAgain (HelloRequest) returns (HelloReply) {}
}
// The request message containing the user's name.
message HelloRequest {
string name = 1;
}
// The response message containing the greetings
message HelloReply {
string message = 1;
}
使用
在.proto文件中定义一个服务
下面是一个实例,该服务中包含四种类型的方法
syntax = "proto3";
option java_multiple_files = true;
option java_package = "io.grpc.examples.routeguide";
option java_outer_classname = "RouteGuideProto";
option objc_class_prefix = "RTG";
package routeguide;
// Interface exported by the server.
service RouteGuide {
// A simple RPC.
// client和server都是一次性的传入或者返回数据
// Obtains the feature at a given position.
//
// A feature with an empty name is returned if there's no feature at the given
// position.
rpc GetFeature(Point) returns (Feature) {}
// A server-to-client streaming RPC.
// server返回的是一个流,
// Obtains the Features available within the given Rectangle. Results are
// streamed rather than returned at once (e.g. in a response message with a
// repeated field), as the rectangle may cover a large area and contain a
// huge number of features.
rpc ListFeatures(Rectangle) returns (stream Feature) {}
// A client-to-server streaming RPC.
// client传入的是一个流
// Accepts a stream of Points on a route being traversed, returning a
// RouteSummary when traversal is completed.
rpc RecordRoute(stream Point) returns (RouteSummary) {}
// A Bidirectional streaming RPC.
//
// Accepts a stream of RouteNotes sent while a route is being traversed,
// while receiving other RouteNotes (e.g. from other users).
rpc RouteChat(stream RouteNote) returns (stream RouteNote) {}
}
// Points are represented as latitude-longitude pairs in the E7 representation
// (degrees multiplied by 10**7 and rounded to the nearest integer).
// Latitudes should be in the range +/- 90 degrees and longitude should be in
// the range +/- 180 degrees (inclusive).
message Point {
int32 latitude = 1;
int32 longitude = 2;
}
// A latitude-longitude rectangle, represented as two diagonally opposite
// points "lo" and "hi".
message Rectangle {
// One corner of the rectangle.
Point lo = 1;
// The other corner of the rectangle.
Point hi = 2;
}
// A feature names something at a given point.
//
// If a feature could not be named, the name is empty.
message Feature {
// The name of the feature.
string name = 1;
// The point where the feature is detected.
Point location = 2;
}
// A RouteNote is a message sent while at a given point.
message RouteNote {
// The location from which the message is sent.
Point location = 1;
// The message to be sent.
string message = 2;
}
// A RouteSummary is received in response to a RecordRoute rpc.
//
// It contains the number of individual points received, the number of
// detected features, and the total distance covered as the cumulative sum of
// the distance between each point.
message RouteSummary {
// The number of points received.
int32 point_count = 1;
// The number of known features passed while traversing the route.
int32 feature_count = 2;
// The distance covered in metres.
int32 distance = 3;
// The duration of the traversal in seconds.
int32 elapsed_time = 4;
}
利用grpcio-tools里面的protocol buffer compiler protoc来生成server和client的代码
生成的主要包括service输入的参数以及返回的类,还包括service和client,这些生成的类在后面的自定义代码位置可以调用
- 在.proto文件中定义的message生成的类文件,在XXX_pb2.py文件中
- XXXStub类:生成之后client用来调用server的服务的,在XXX_pb2_grpc.py文件中
- XXXServicer:server及其中的方法的接口,和XXXStub类中的方法一一对应,无实现,仅方法接口,在XXX_pb2_grpc.py文件中
- add_XXXServicer_to_server:将servicer添加到grpc.Server中去
利用生成的接口来编写客户端和服务器的代码
实现servicer
创建一个类继承自生成的接口类,实现接口类XXXServicer中的方法,然后使用add_XXXServicer_to_server将servicer添加到server中,之后通过server.start()开启
实现client
首先创建一个XXXStub的实例,然后调用,当返回的response只有一个的时候调用有同步和异步两种方式
|