俞渐俞烈
Success
Base64
???一个加密技术,由64个不同的字符进行加密,在本文章中,我传递过去的数据是加密后的字节,而提供者接收参数后将数据进行解析并且转换字节数组。 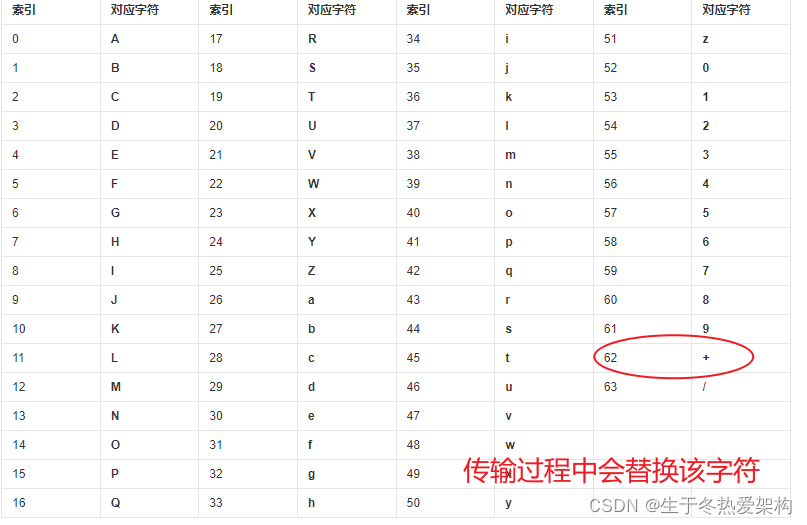
Base64依赖
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
<version>1.13</version>
</dependency>
OkHttp
???最近做了一个小测试,那就是如何通过HttpClient请求的时候进行传递字节数组,因为我们一般都是网页中请求,并且如果要是照片或者文件的话我们都会以流的行式进行传递,所以突发想象,想通过HttpClient的方式来传递字节数组,刚开始传递的时候发现如果不把字节进行加密传递的话,最终获取到的字节会和传递的字节不一致,当把字节加密后之后,是可以的,但是仅限于小文件或小图片(几kb),由于http的请求限制参数的最长长度,所以我们如果想要传递大图片或文件的话,需要通过body体来进行实现,HttpClient的方式可以实现,但是较为麻烦,又要设置头又要设置尾的,所以我就用了OkHttp(基于HttpClient的优化),它有个工具类,特别方便,只要将要放在body体中的参数传递过去即可,具体实现都由OkHttp帮我们做好了,它会把我们的参数放置到body体中,通过RequestBody来进行存储,而我们被请求端(可以理解为提供者)这边接收参数的时候只需要从body体中获取即可,无需在进行参数名称对应。 OkHttp依赖
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>3.14.7</version>
</dependency>
TestRequest.java方法中
class TestRequest {
public static void main(String[] args) {
result(url);
}
public static String result(String url) {
File f = new File("D:\\logs\\30.png");
byte [] fileByte = File2byte(f);
String post = null;
try {
post = HttpClientUtil.post(url, urlencode2(fileByte));
} catch (IOException e) {
e.printStackTrace();
}
System.out.println(post);
return null;
}
public static byte[] File2byte(File tradeFile){
byte[] buffer = null;
try
{
FileInputStream fis = new FileInputStream(tradeFile);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
byte[] b = new byte[1024];
int n;
while ((n = fis.read(b)) != -1)
{
bos.write(b, 0, n);
}
fis.close();
bos.close();
buffer = bos.toByteArray();
}catch (FileNotFoundException e){
e.printStackTrace();
}catch (IOException e){
e.printStackTrace();
}
return buffer;
}
public static String urlencode2(byte[] bytes) {
String str = Base64.encodeBase64String(bytes);
return str;
}
}
HttpClietUtil.java ???由OkHttp进行提供
public class HttpClientUtil {
private static final Logger log = LoggerFactory.getLogger(HttpClientUtil.class);
private static final MediaType JSON = MediaType.parse("application/json; charset=utf-8");
private static final OkHttpClient CLIENT = client();
private static OkHttpClient client() {
return new OkHttpClient.Builder()
.addInterceptor(new LogInterceptor())
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.writeTimeout(10, TimeUnit.SECONDS)
.build();
}
private static Request request(String uri) {
return new Request.Builder().url(uri).build();
}
public static String response(String uri) throws IOException {
log.info("HttpClientUtil-request: {}", uri);
try (Response response = CLIENT.newCall(request(uri)).execute()) {
if (!response.isSuccessful()) {
throw new IOException("Unexpected code " + response);
}
String string = response.body().string();
log.info("HttpClientUtil-responsebody: {}", string);
return string;
}
}
public static String post(String url, String json) throws IOException {
RequestBody body = RequestBody.create(JSON, json);
Request request = new Request.Builder()
.url(url)
.post(body)
.build();
Response response = CLIENT.newCall(request).execute();
return response.body().string();
}
public static String get(String url, Map<String, String> params, Map<String, String> headMap) throws IOException{
String result = null;
url += getParams(params);
Headers setHeaders = SetHeaders(headMap);
Request request = new Request.Builder().url(url).headers(setHeaders).build();
Call call = CLIENT.newCall(request);
try {
Response response = call.execute();
result = response.body().string();
} catch (Exception e) {
throw new IOException("Unexpected code " + e);
}
return result;
}
public static String getParams(Map<String, String> params) {
StringBuffer sb = new StringBuffer("?");
if (!params.isEmpty()) {
for (Map.Entry<String, String> item : params.entrySet()) {
String value = item.getValue();
if (StringUtils.isNotEmpty(value)) {
sb.append("&");
sb.append(item.getKey());
sb.append("=");
sb.append(value);
}
}
return sb.toString();
} else {
return "";
}
}
public static Headers SetHeaders(Map<String, String> headersParams) {
Headers headers = null;
Headers.Builder headersbuilder = new Headers.Builder();
if (!headersParams.isEmpty()) {
Iterator<String> iterator = headersParams.keySet().iterator();
String key = "";
while (iterator.hasNext()) {
key = iterator.next().toString();
headersbuilder.add(key, headersParams.get(key));
}
}
headers = headersbuilder.build();
return headers;
}
private static class LogInterceptor implements Interceptor {
@Override
public Response intercept(Chain chain) throws IOException {
Request request = chain.request();
Response response = chain.proceed(request);
MediaType mediaType = response.body().contentType();
String str = response.body().string();
return response.newBuilder().body(ResponseBody.create(mediaType, str)).build();
}
}
}
提供者
@PostMapping(value = "/demoArray")
@ResponseBody
public String index3(@RequestBody String val) {
String replace = val.replace(" ", "+");
byte2File(Base64.decodeBase64(replace), "D:\\logs\\13.png");
return "success";
}
public void byte2File(byte[] b, String outputFile) {
File file = null;
FileOutputStream output = null;
try {
file = new File(outputFile);
if (file.exists()) {
file.delete();
}
output = new FileOutputStream(file);
output.write(b);
output.flush();
} catch (Exception e) {
e.printStackTrace();
} finally {
if (output != null) {
try {
output.close();
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
}
效果图
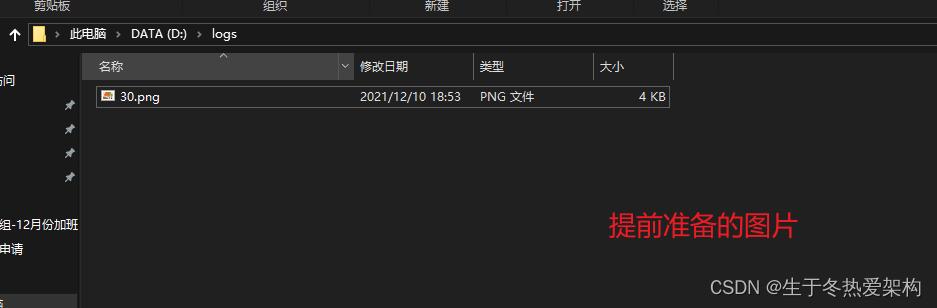 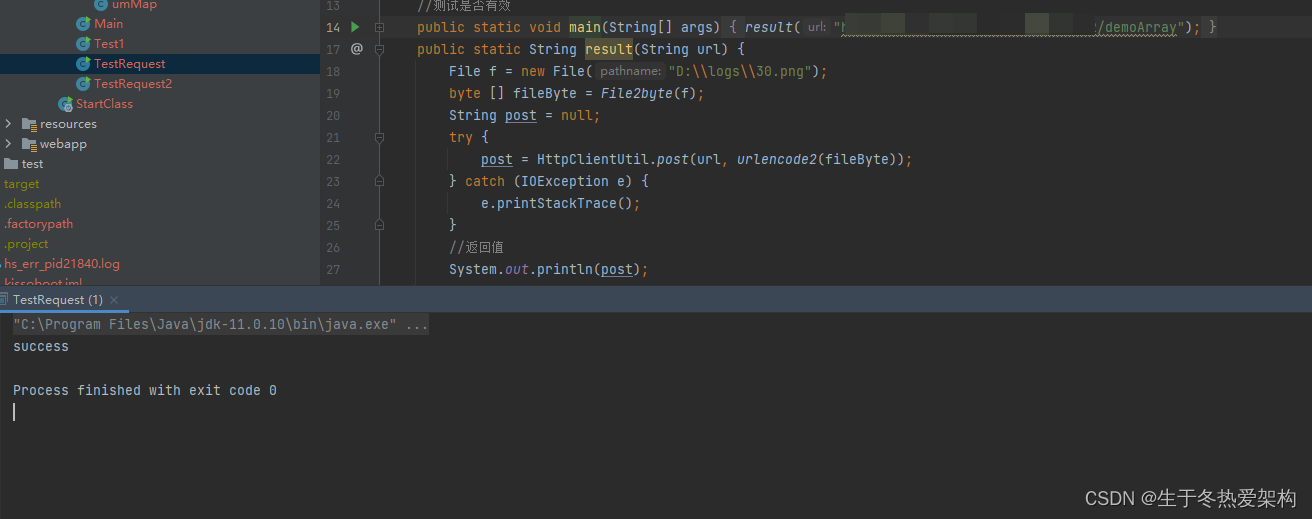 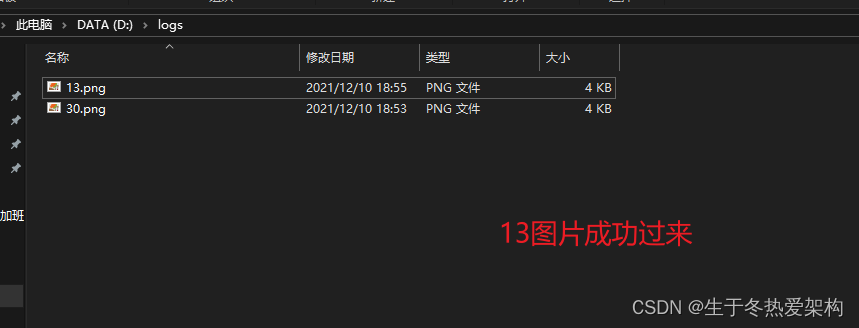
|