-
发送邮件:SMTP 协议 -
接收邮件:POP3 协议 -
需要准备 JavaMail API 和 Java Activation Framework mail.jar 和 activation.jar -
要发送邮件,需要获得协议和支持(开启 POP3 和 SMTP 服务)
简单邮件发送
import com.sun.mail.util.MailSSLSocketFactory;
import javax.mail.*;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import java.util.Properties;
public class Mail {
public static void main(String[] args) throws Exception{
Properties properties = new Properties();
properties.setProperty("mail.host","smtp.qq.com");
properties.setProperty("mail.transport.protocol","smtp");
properties.setProperty("mail.smtp.auth","true");
MailSSLSocketFactory mailSSLSocketFactory = new MailSSLSocketFactory();
mailSSLSocketFactory.setTrustAllHosts(true);
properties.put("mail.smtp.ssl.enable","true");
properties.put("mail.smtp.ssl.socketFactory",mailSSLSocketFactory);
Session session=Session.getDefaultInstance(properties, new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("邮箱","授权码");
}
});
session.setDebug(true);
Transport transport = session.getTransport();
transport.connect("smtp.qq.com","邮箱","授权码");
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress("发件人邮箱"));
message.setRecipient(Message.RecipientType.TO,new InternetAddress("收件人邮箱"));
message.setSubject("只有文本的简单邮件");
message.setContent("你好","text/html;charset=utf-8");
transport.sendMessage(message,message.getAllRecipients());
transport.close();
}
}
MIME
MIME(多用途互联网邮件扩展类型)
一个互联网标准,扩展了电子邮件标准,使其能够支持:
- 非 ASCII 字符文本
- 非文本可是附件(二进制,声音,图像等)
- 由多部分组成的消息体
- 包含非 ASCII 字符的头消息
MimeBodypart 类
MimeMultipart 类
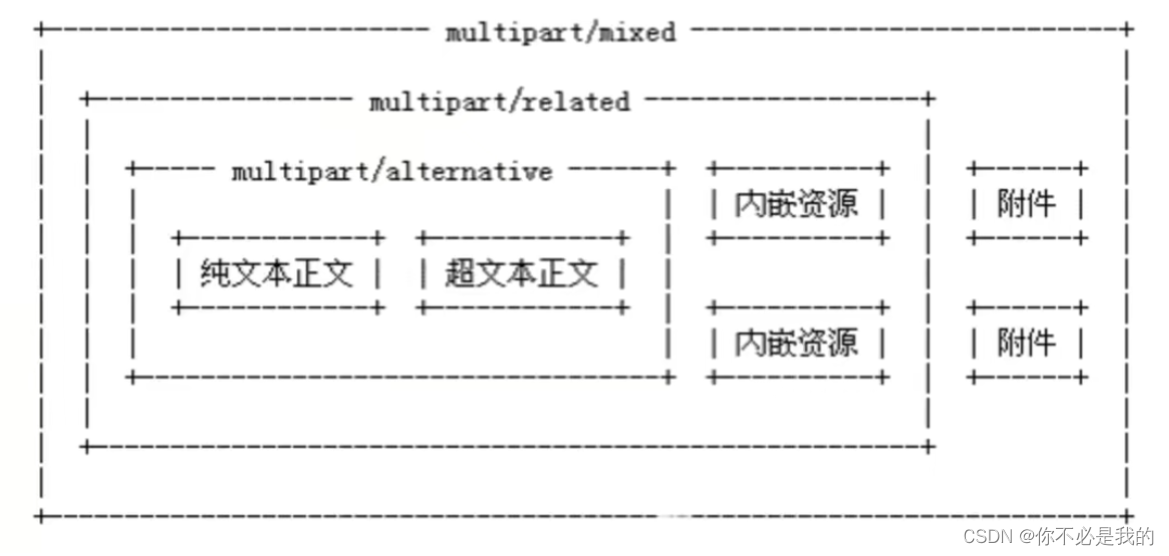
import com.sun.mail.util.MailSSLSocketFactory;
import javax.activation.DataHandler;
import javax.activation.FileDataSource;
import javax.mail.*;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
import java.util.Properties;
public class MailMime {
public static void main(String[] args) throws Exception{
Properties properties = new Properties();
properties.setProperty("mail.host","smtp.qq.com");
properties.setProperty("mail.transport.protocol","smtp");
properties.setProperty("mail.smtp.auth","true");
MailSSLSocketFactory mailSSLSocketFactory = new MailSSLSocketFactory();
mailSSLSocketFactory.setTrustAllHosts(true);
properties.put("mail.smtp.ssl.enable","true");
properties.put("mail.smtp.ssl.socketFactory",mailSSLSocketFactory);
Session session=Session.getDefaultInstance(properties, new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("邮箱","授权码");
}
});
session.setDebug(true);
Transport transport = session.getTransport();
transport.connect("smtp.qq.com","邮箱","授权码");
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress("发件人邮箱"));
message.setRecipient(Message.RecipientType.TO,new InternetAddress("收件人邮箱"));
message.setSubject("什么都有的邮件");
MimeBodyPart image = new MimeBodyPart();
DataHandler dh = new DataHandler(new FileDataSource("src/resources/第一天.png"));
image.setDataHandler(dh);
image.setContentID("1.png");
MimeBodyPart text = new MimeBodyPart();
text.setContent("这是一个封邮件正文带图片<img src='cid:1.png'>的邮件","text/html;charset=utf-8");
MimeBodyPart file = new MimeBodyPart();
DataHandler ddh = new DataHandler(new FileDataSource("src/resources/1.txt"));
file.setDataHandler(ddh);
file.setFileName("1.txt");
MimeMultipart mimeMultipart = new MimeMultipart();
mimeMultipart.addBodyPart(text);
mimeMultipart.addBodyPart(image);
mimeMultipart.setSubType("related");
MimeBodyPart content = new MimeBodyPart();
content.setContent(mimeMultipart);
MimeMultipart mimeMultipart1 = new MimeMultipart();
mimeMultipart1.addBodyPart(file);
mimeMultipart1.addBodyPart(content);
mimeMultipart1.setSubType("mixed");
message.setContent(mimeMultipart1);
message.saveChanges();
transport.sendMessage(message,message.getAllRecipients());
transport.close();
}
}
|