完整代码:
using System;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading;
using UnityEngine;
public class UDPClientController : MonoBehaviour
{
[Header("目标IP (Debug Only)")] [SerializeField] private string targetIp;
[Header("目标端口号")] [SerializeField] public int ___targetPort = 8418;
[Header("自身端口号")] [SerializeField] public int ___myPort = 8419;
[Header("是否接收数据")] [SerializeField] private bool isStartReceiver;
public string ___targetIp
{
get => targetIp;
set
{
if (targetIp != value)
{
targetIp = value;
try
{
targetIpEndPoint = new IPEndPoint(IPAddress.Parse(targetIp), ___targetPort);
}
catch { }
}
}
}
private const int threadWaitTime = 10;
private IPEndPoint targetIpEndPoint;
private UdpClient udpClient;
private static bool isHaveData;
private static byte[] receiveBytes1;
private static byte[] receiveBytes
{
get => receiveBytes1;
set
{
receiveBytes1 = value;
isHaveData = true;
}
}
#region 单例
public static UDPClientController a;
private void Awake() => a = this;
#endregion
private void Start()
{
udpClient = new UdpClient(___myPort);
if (!string.IsNullOrEmpty(___targetIp))
___targetIp = targetIp;
if (isStartReceiver)
_____StartReceive();
}
private void Update()
{
if (isHaveData)
{
isHaveData = false;
string str = Encoding.Default.GetString(receiveBytes);
print("接受到内容" + str);
}
}
public void _____SendString(string str)
{
SendBytes(Encoding.Default.GetBytes(str));
}
public void _____SendT2dJPG(Texture2D t2d)
{
SendBytes(t2d.EncodeToJPG());
}
private void _____StartReceive()
{
udpClient.BeginReceive(ReceiveCallback, udpClient);
}
private static void ReceiveCallback(IAsyncResult result)
{
UdpClient udpClient = (UdpClient)result.AsyncState;
IPEndPoint iPEndPoint = null;
receiveBytes = udpClient.EndReceive(result, ref iPEndPoint);
Thread.Sleep(threadWaitTime);
udpClient.BeginReceive(ReceiveCallback, udpClient);
}
private void SendBytes(byte[] bs)
{
print("字节长度:" + bs.Length);
if (targetIpEndPoint != null)
udpClient.BeginSend(bs, bs.Length, targetIpEndPoint, SendCallback, udpClient);
else
Debug.LogError("“目标IP” 没有设置!");
}
private static void SendCallback(IAsyncResult result)
{
UdpClient u = (UdpClient)result.AsyncState;
}
}
使用说明:
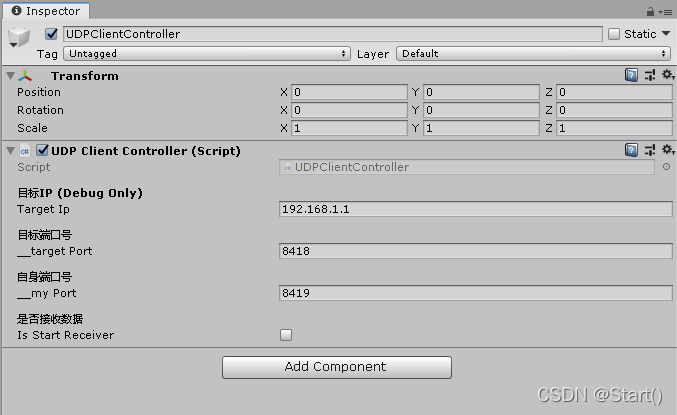
更改目标IP:
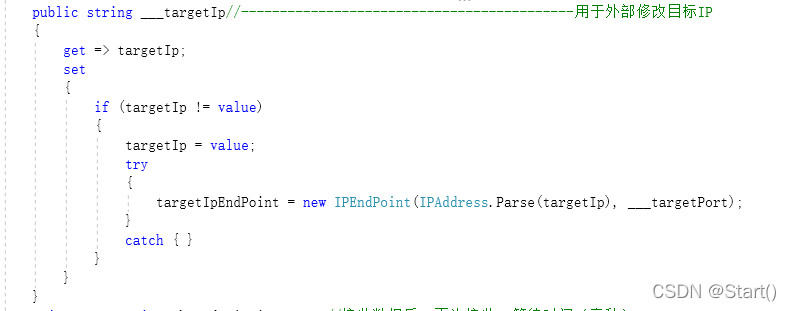
发送:
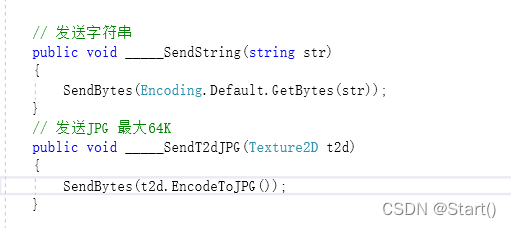
接收:
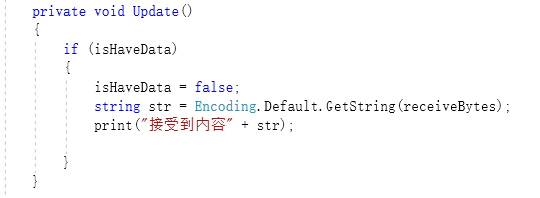
|