C#基于Socket客户端和服务器通信
服务端
- 服务端界面
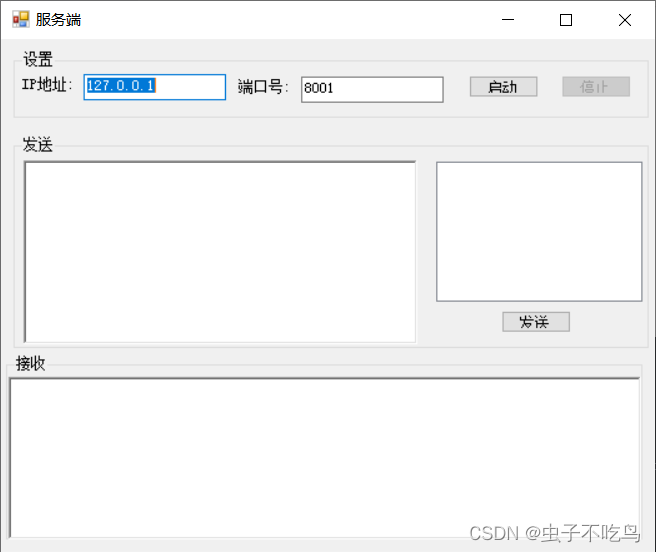 - 服务端代码
public partial class FormMain : Form
{
private List<Socket> ClientSockets;
private BindingList<string> ClientIPPorts;
private Socket socketWatch;
private Socket socketSend;//发送
private bool IsStart;
private Action<string> ShowMsgAction;
private Action UpdateListViewDataAction;
public FormMain()
{
InitializeComponent();
ShowMsgAction += new Action<string>(ShowMsg);
UpdateListViewDataAction += new Action(UpdateListViewData);
ClientSockets = new List<Socket>();
ClientIPPorts = new BindingList<string>();
BindingSource bs = new BindingSource(); //实例化对象
bs.DataSource = ClientIPPorts; //将List<T>绑定到BindingSource中
listBox1.DataSource = bs; //将BindingSource绑定至最终的使用者(控件)的数据源
}
private void btn_start_Click(object sender, EventArgs e)
{
Socket socketWatch = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
IPAddress ip = IPAddress.Any;
//创建对象端口
IPEndPoint point = new IPEndPoint(ip, Convert.ToInt32(tbox_port.Text));
socketWatch.Bind(point);//绑定端口号
ShowMsg("信息:监听成功!");
socketWatch.Listen(100);//允许连接的客户端数量
//创建监听线程
Thread thread = new Thread(Listen);
thread.IsBackground = true;
thread.Start(socketWatch);
IsStart = true;
ShowBtnState();
}
private void btn_stop_Click(object sender, EventArgs e)
{
socketWatch?.Close();
IsStart = false;
ShowBtnState();
ShowMsg("信息:停止监听!");
}
private void btn_send_Click(object sender, EventArgs e)
{
if (!IsStart) return;
string info = rtbox_send.Text;
if (info == "") return;
int index = listBox1.SelectedIndex;
if (index < 0) return;
if (ClientSockets.Count <= index) return;
socketSend = ClientSockets[index];
Send(info);
}
void Listen(object o)
{
try
{
Socket socketWatch = o as Socket;
while (IsStart)
{
socketSend = socketWatch.Accept();//等待接收客户端连接
ClientSockets.Add(socketSend);
ClientIPPorts.Add(socketSend.RemoteEndPoint.ToString());
this.BeginInvoke(UpdateListViewDataAction);
this.BeginInvoke(ShowMsgAction, socketSend.RemoteEndPoint.ToString() + ":" + "连接成功!");
//开启一个新线程,执行接收消息方法
Thread r_thread = new Thread(Received);
r_thread.IsBackground = true;
r_thread.Start(socketSend);
}
}
catch (Exception ex)
{
this.BeginInvoke(ShowMsgAction, "等待客户端监听发生异常:" + ex.Message);
}
}
/// <summary>
/// 服务器端不停的接收客户端发来的消息
/// </summary>
/// <param name="o"></param>
void Received(object o)
{
try
{
Socket socketSend = o as Socket;
if (!socketSend.Connected) return;
while (IsStart)
{
//客户端连接服务器成功后,服务器接收客户端发送的消息
byte[] buffer = new byte[1024 * 1024 * 3];
//实际接收到的有效字节数
int len = socketSend.Receive(buffer);
if (len == 0)
{
break;
}
string str = Encoding.UTF8.GetString(buffer, 0, len);
this.BeginInvoke(ShowMsgAction, socketSend.RemoteEndPoint + ":" + str);
}
}
catch (Exception ex)
{
this.BeginInvoke(ShowMsgAction, "接收客户端内容发生异常:" + ex.Message);
}
}
/// <summary>
/// 发送消息
/// </summary>
/// <param name="str"></param>
void Send(string str)
{
try
{
if (socketSend == null) return;
byte[] buffer = Encoding.UTF8.GetBytes(str);
socketSend.Send(buffer);
}
catch (Exception ex)
{
ShowMsg(ex.Message);
}
}
private void ShowMsg(string msg)
{
string info = string.Format("{0}:{1}\r\n", DateTime.Now.ToString("G"), msg);
rtbox_receive.AppendText(info);
}
private void ShowBtnState()
{
btn_start.Enabled = !IsStart;
btn_stop.Enabled = IsStart;
}
/// <summary>
/// 用于实时监测客户端是否断开连接
/// </summary>
private void timer1_Tick(object sender, EventArgs e)
{
if (ClientSockets.Count == 0) return;
for (int i = ClientSockets.Count-1; i >= 0; i--)
{
if (!ClientSockets[i].Connected)
{
ClientSockets.RemoveAt(i);
ClientIPPorts.RemoveAt(i);
this.BeginInvoke(UpdateListViewDataAction);
}
}
}
private void UpdateListViewData()
{
listBox1.DataSource = null;
listBox1.DataSource = ClientIPPorts;
//listBox1.Items.Clear();
//for (int i = 0; i < ClientIPPorts.Count; i++)
//{
//}
}
}
客户端
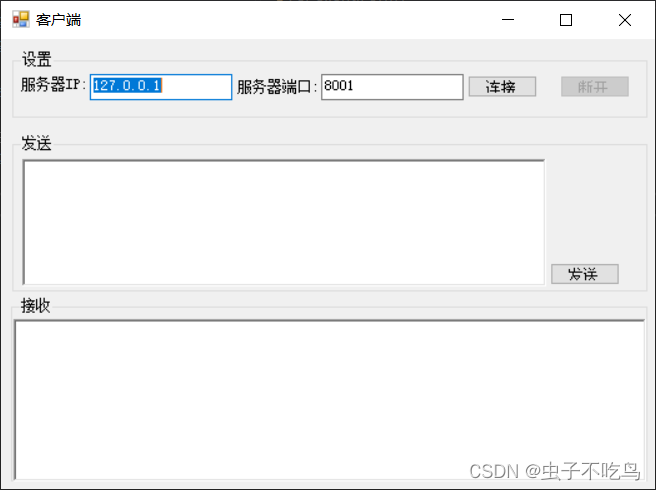
public partial class Form1 : Form
{
private Socket socketSend;
private bool IsStart;
private Action<string> ShowMsgAction;
public Form1()
{
InitializeComponent();
ShowMsgAction += new Action<string>(ShowMsg);
}
private void btn_connet_Click(object sender, EventArgs e)
{
try
{
//创建客户端Socket,获得远程ip和端口号
socketSend = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
IPAddress ip = IPAddress.Parse(tbox_ip.Text);
IPEndPoint point = new IPEndPoint(ip, Convert.ToInt32(tbox_port.Text));
socketSend.Connect(point);
ShowMsg("连接成功!");
//开启新的线程,不停的接收服务器发来的消息
Thread c_thread = new Thread(Received);
c_thread.IsBackground = true;
c_thread.Start();
IsStart = true;
ShowBtnState();
}
catch (Exception ex)
{
ShowMsg("连接失败:"+ex.Message);
}
}
private void btn_disconnect_Click(object sender, EventArgs e)
{
if (socketSend.Connected)
{
socketSend.Close();
}
IsStart = false;
ShowBtnState();
ShowMsg("信息:断开连接!");
}
private void btn_send_Click(object sender, EventArgs e)
{
if (!IsStart) return;
string info = rtbox_send.Text;
if (info == "") return;
Send(info);
}
/// <summary>
/// 接收线程
/// </summary>
private void Received()
{
while (IsStart)
{
try
{
if (!socketSend.Connected) return;
byte[] buffer = new byte[1024 * 1024 * 3];
//实际接收到的有效字节数
int len = socketSend.Receive(buffer);
if (len == 0)
{
break;
}
string str = Encoding.UTF8.GetString(buffer, 0, len);
this.BeginInvoke(ShowMsgAction, socketSend.RemoteEndPoint + ":" + str);
}
catch (Exception ex)
{
this.BeginInvoke(ShowMsgAction, ex.Message);
}
}
}
private void Send(string msg)
{
try
{
byte[] buffer = new byte[1024 * 1024 * 3];
buffer = Encoding.UTF8.GetBytes(msg);
socketSend.Send(buffer);
}
catch (Exception ex)
{
this.BeginInvoke(ShowMsgAction, ex.Message);
}
}
private void ShowMsg(string msg)
{
string info = string.Format("{0}:{1}\r\n", DateTime.Now.ToString("G"), msg);
rtbox_receive.AppendText(info);
}
private void ShowBtnState()
{
btn_connet.Enabled = !IsStart;
btn_disconnect.Enabled = IsStart;
}
}
|