一、服务端代码
1.服务启动类
package com.example.netty.server;
import com.example.netty.server.init.NettyServerHttpInitializer;
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class NettyServer {
public static void main(String[] args) throws InterruptedException {
EventLoopGroup bossGroup = new NioEventLoopGroup(1);
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(bossGroup,workerGroup)
.channel(NioServerSocketChannel.class)
.option(ChannelOption.SO_BACKLOG,128)
.childOption(ChannelOption.SO_KEEPALIVE,true)
.childHandler(new NettyServerHttpInitializer());
System.out.println("Server is Ready");
ChannelFuture cf = bootstrap.bind(6668).sync();
cf.channel().closeFuture().sync();
}finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
}
2.管道初始化类
package com.example.netty.server.init;
import com.example.netty.server.handler.NettyServerHttpHandler;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.socket.SocketChannel;
import io.netty.handler.codec.http.HttpServerCodec;
public class NettyServerHttpInitializer extends ChannelInitializer<SocketChannel> {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast("httpDec",new HttpServerCodec()).addLast("httpHan",new NettyServerHttpHandler());
}
}
3.处理器类
package com.example.netty.server.handler;
import io.netty.buffer.ByteBuf;
import io.netty.buffer.Unpooled;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.handler.codec.http.*;
import io.netty.util.CharsetUtil;
public class NettyServerHttpHandler extends SimpleChannelInboundHandler<HttpObject> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, HttpObject msg) throws Exception {
if (msg instanceof HttpRequest){
System.out.println("MSG Class " + msg.getClass());
System.out.println("Client IP " + ctx.channel().remoteAddress());
ByteBuf content = Unpooled.copiedBuffer("Hello,server is busing", CharsetUtil.UTF_8);
FullHttpResponse response = new DefaultFullHttpResponse(HttpVersion.HTTP_1_1,HttpResponseStatus.OK,content);
response.headers().set(HttpHeaderNames.CONTENT_TYPE,"text/plain");
response.headers().set(HttpHeaderNames.CONTENT_LENGTH,content.readableBytes());
ctx.writeAndFlush(response);
}
}
}
4.启动服务,调用演示
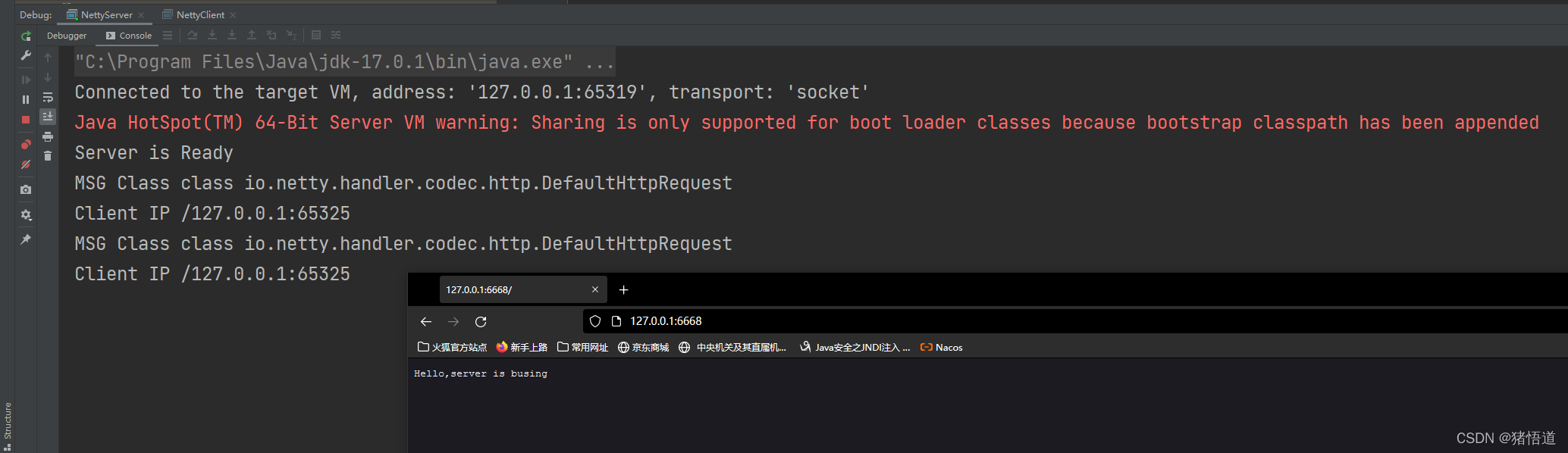
5.如果出现6668不安全提示,可以将端口号修改为 > 8000 或修改浏览器配置
6.一次调用打印两次日志
查看浏览器控制台发现,回车发送请求,会默认查询站点图标 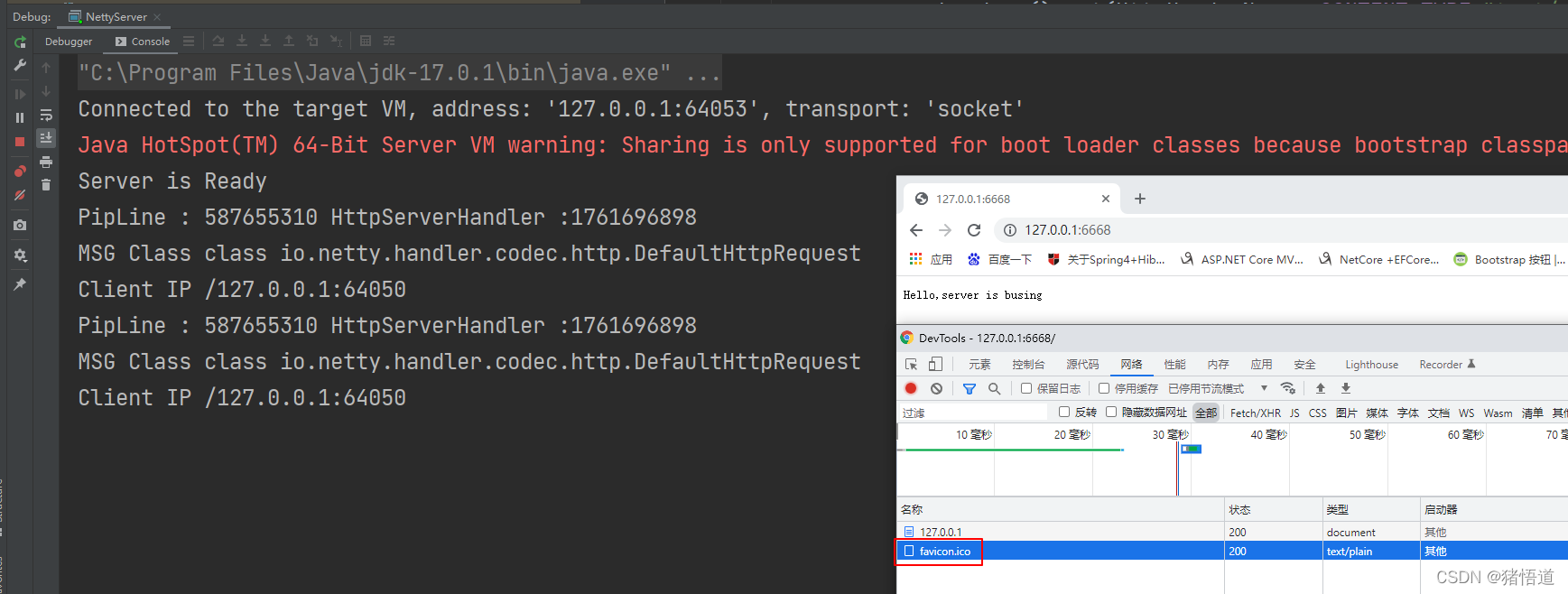
尝试在服务端过滤该请求,不做响应;处理器中插入以下代码段
String web_pic = "/favicon.ico";
HttpRequest httpRequest = (HttpRequest)msg;
URI uri = new URI(httpRequest.uri());
if (web_pic.equals(uri.getPath())){
System.out.println("Request Ico,do not response...");
return;
}
重启服务后,在次请求,查看结果如下 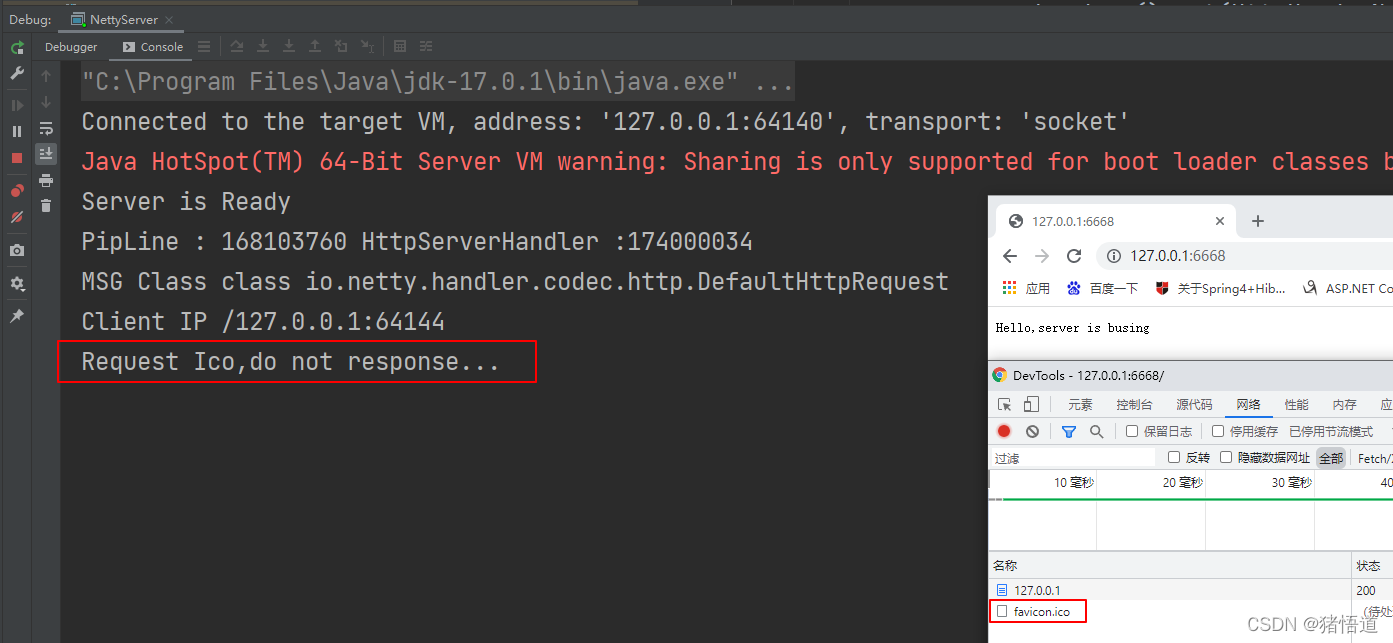
7.通道分析(服务端如何分配管道)
http 协议为无状态协议,通信完成即会断开连接,重复请求会重新分配管道 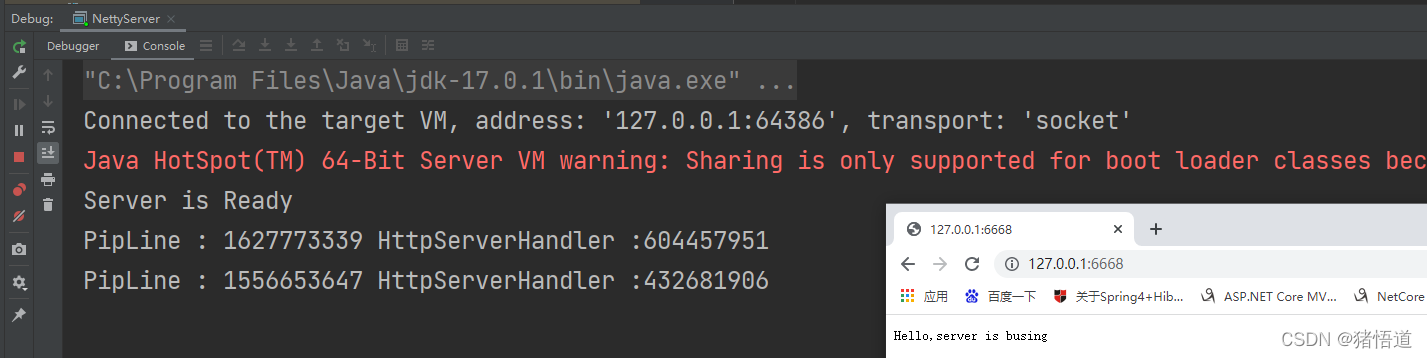
|