1. 路由配置
package main
import (
"fmt"
"net/http"
"github.com/gin-gonic/gin"
)
type UserInfo struct {
Username string `form:"username" json:"username" binding:"required"`
Password string `form:"password" json:"password" binding:"required"`
Age int `form:"age" json:"age"`
}
type Department struct {
Id int `json:"id"`
DeptName string `json:"deptName"`
}
func main() {
r := gin.Default()
r.Static("/static", "./static")
r.GET("/", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "Hello world!",
})
})
r.GET("/login", func(c *gin.Context) {
username := c.Query("username")
password := c.DefaultQuery("password", "123")
c.JSON(http.StatusOK, gin.H{
"name": username,
"password": password,
})
})
r.GET("/login/:username", func(c *gin.Context) {
username := c.Param("username")
c.JSON(http.StatusOK, gin.H{
"name": username,
})
})
r.POST("/login", func(c *gin.Context) {
username := c.PostForm("username")
password := c.PostForm("password")
c.JSON(http.StatusOK, gin.H{
"name": username,
"password": password,
})
})
r.POST("/loginForm", func(c *gin.Context) {
user := &UserInfo{}
err := c.ShouldBind(&user)
if err == nil {
fmt.Printf("%#v \n", user)
c.JSON(http.StatusOK, user)
} else {
c.JSON(http.StatusBadRequest, gin.H{
"err": err.Error(),
})
}
})
r.POST("/loginJson", func(c *gin.Context) {
dept := &Department{}
err := c.ShouldBindJSON(&dept)
if err == nil {
fmt.Printf("%#v \n", dept)
c.JSON(http.StatusOK, dept)
} else {
c.JSON(http.StatusBadRequest, gin.H{
"err": err.Error(),
})
}
})
r.Run()
}
2. 获取get请求传值
r.GET("/login", func(c *gin.Context) {
username := c.Query("username")
password := c.DefaultQuery("password", "123")
c.JSON(http.StatusOK, gin.H{
"name": username,
"password": password,
})
})
- http://localhost:8080/login?username=fisher
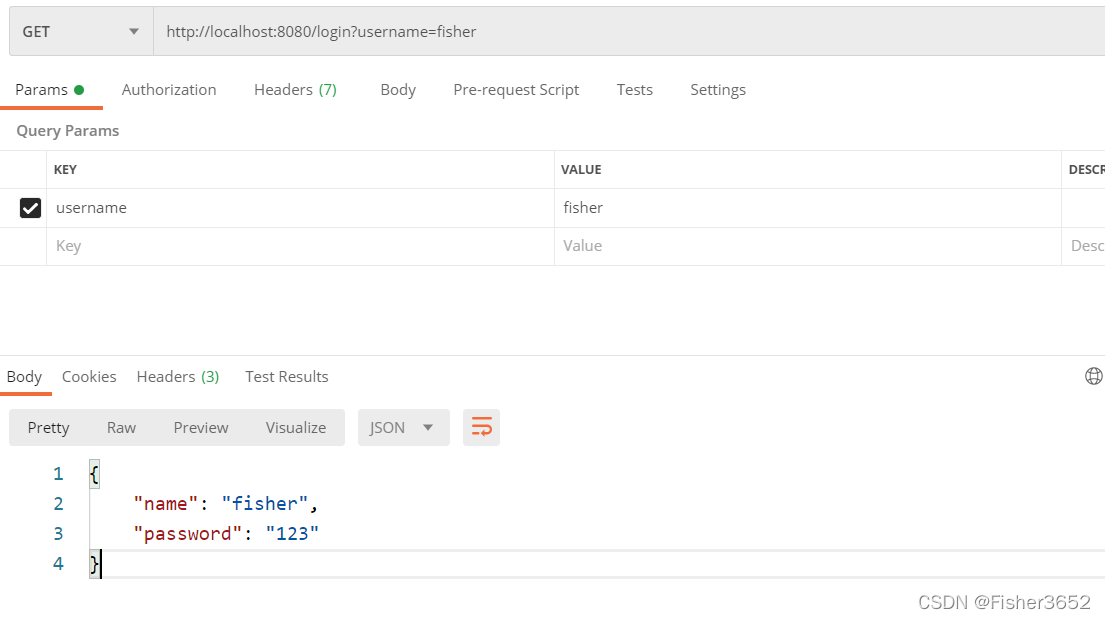
3. 获取get请求,path传值
r.GET("/login/:username", func(c *gin.Context) {
username := c.Param("username")
c.JSON(http.StatusOK, gin.H{
"name": username,
})
})
- http://localhost:8080/login/fisher
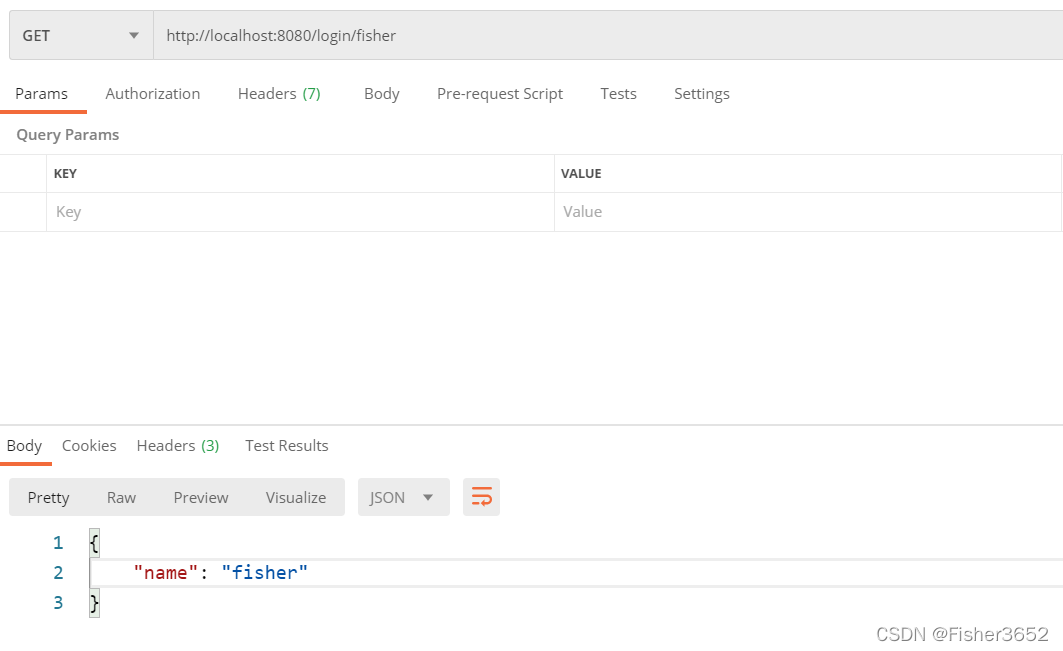
4. 获取post请求传值
r.POST("/login", func(c *gin.Context) {
username := c.PostForm("username")
password := c.PostForm("password")
c.JSON(http.StatusOK, gin.H{
"name": username,
"password": password,
})
})
- http://localhost:8080/login
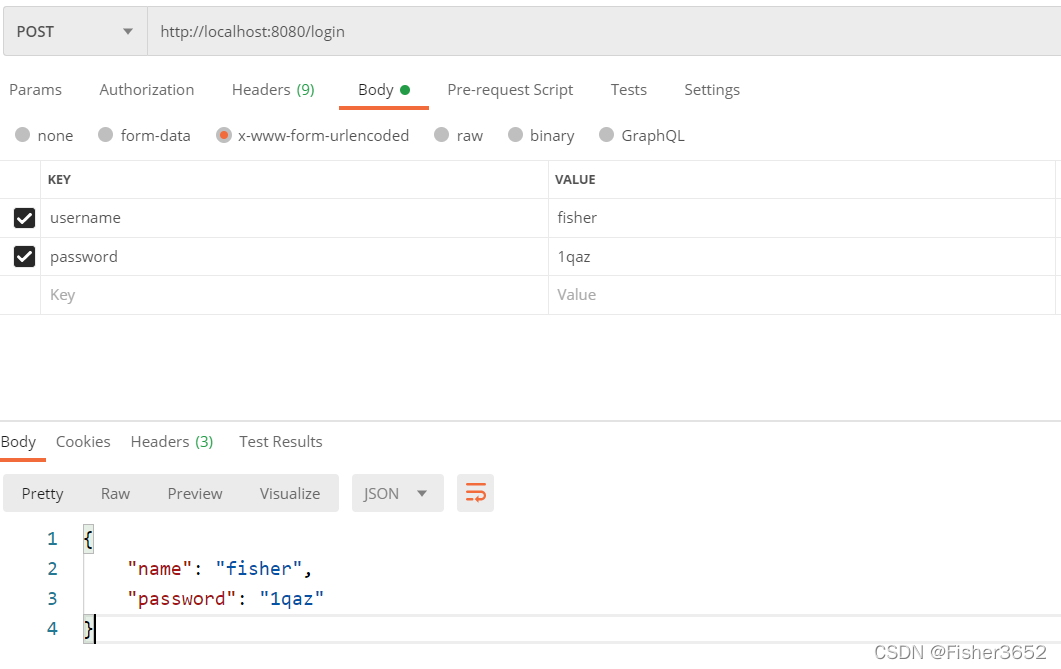
5. 获取post form请求,结构体传值
type UserInfo struct {
Username string `form:"username" json:"username" binding:"required"`
Password string `form:"password" json:"password" binding:"required"`
Age int `form:"age" json:"age"`
}
r.POST("/loginForm", func(c *gin.Context) {
user := &UserInfo{}
err := c.ShouldBind(&user)
if err == nil {
fmt.Printf("%#v \n", user)
c.JSON(http.StatusOK, user)
} else {
c.JSON(http.StatusBadRequest, gin.H{
"err": err.Error(),
})
}
})
- http://localhost:8080/loginForm
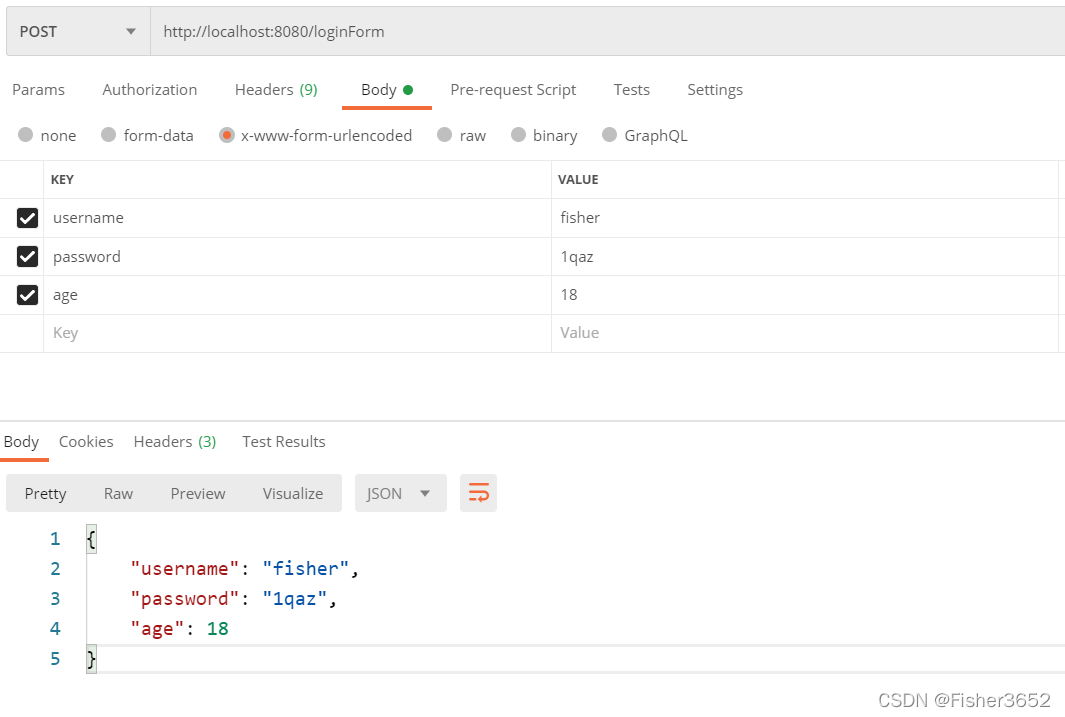
6. 获取post json请求,结构体传值
type Department struct {
Id int `json:"id"`
DeptName string `json:"deptName"`
}
r.POST("/loginJson", func(c *gin.Context) {
dept := &Department{}
err := c.ShouldBindJSON(&dept)
if err == nil {
fmt.Printf("%#v \n", dept)
c.JSON(http.StatusOK, dept)
} else {
c.JSON(http.StatusBadRequest, gin.H{
"err": err.Error(),
})
}
})
- http://localhost:8080/loginJson
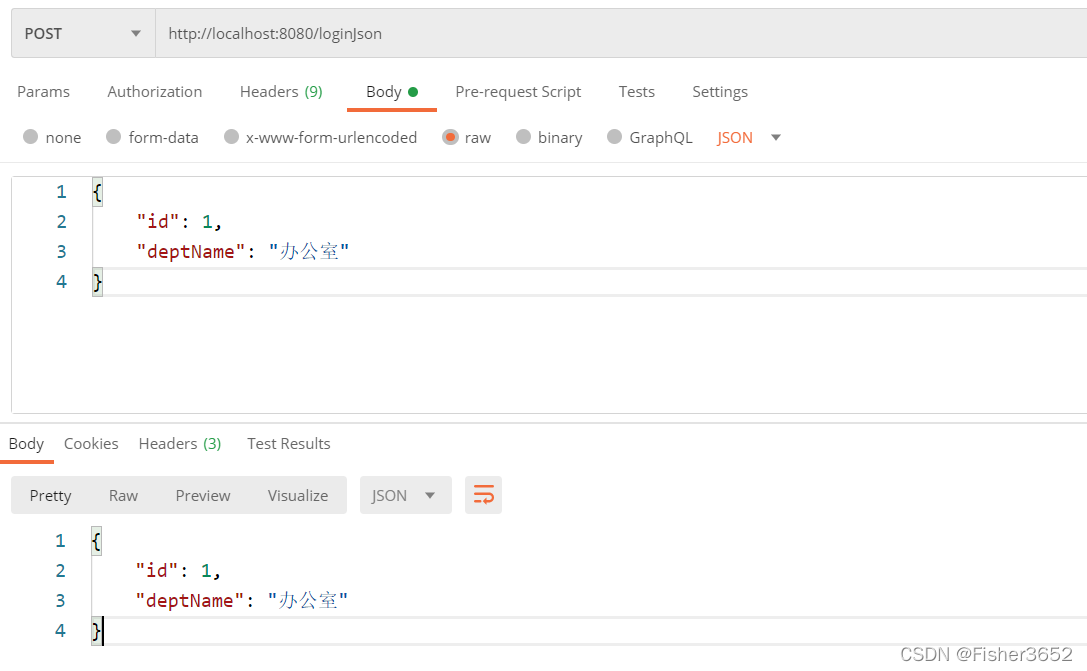
|