头文件: ip_input_edit.h
#ifndef IPLINEEDIT_H
#define IPLINEEDIT_H
#include <QLineEdit>
#include <QLabel>
#include <QWidget>
#include <QFocusEvent>
#include <QKeyEvent>
class IpPartLineEdit : public QLineEdit
{
Q_OBJECT
public:
IpPartLineEdit(QWidget *parent = 0);
~IpPartLineEdit();
void SetPreEdit(QLineEdit *pre);
void SetNextEdit(QLineEdit *next);
protected:
void focusInEvent(QFocusEvent *event);
void keyPressEvent(QKeyEvent *event);
private slots:
void OnTextEdited(const QString & text);
private:
QLineEdit *preEdit;
QLineEdit *nextEdit;
};
class IPLineEdit : public QFrame
{
Q_OBJECT
public:
IPLineEdit(QWidget *parent = 0);
~IPLineEdit();
QString GetText();
void SetStyleSheet(const QString &styleSheet);
public slots:
void setText(const QString &text);
signals:
void textChanged(const QString &text);
void textEdited(const QString &text);
private slots:
void OnTextChanged(const QString &text);
void OnTextEdited(const QString &text);
private:
IpPartLineEdit * pIpPart1;
IpPartLineEdit * pIpPart2;
IpPartLineEdit * pIpPart3;
IpPartLineEdit * pIpPart4;
QLabel * pDot1;
QLabel * pDot2;
QLabel * pDot3;
};
#endif
源文件: ip_input_edit.cpp
#include <QRegExpValidator>
#include <QLayout>
#include "ip_input_edit.h"
IPLineEdit::IPLineEdit(QWidget *parent) :
QFrame(parent)
{
this->setFrameShape(QFrame::Panel);
pIpPart1 = new IpPartLineEdit(this);
pIpPart2 = new IpPartLineEdit(this);
pIpPart3 = new IpPartLineEdit(this);
pIpPart4 = new IpPartLineEdit(this);
pIpPart1->setTextMargins(0,0,0,0);
pIpPart2->setTextMargins(0,0,0,0);
pIpPart3->setTextMargins(0,0,0,0);
pIpPart4->setTextMargins(0,0,0,0);
pDot1 = new QLabel(this);
pDot2 = new QLabel(this);
pDot3 = new QLabel(this);
pDot1->setMargin(0);
pDot2->setMargin(0);
pDot3->setMargin(0);
//QLayout *layout = this->layout();
QLayout *layout = new QHBoxLayout();
this->setLayout(layout);
layout->setContentsMargins(0,0,0,0);
//QLayout::SetNoConstraint
//m_pIpPart1->setGeometry(QRect(0, 0, 42, 27));
//m_pIpPart2->setGeometry(QRect(70, 0, 42, 27));
//m_pIpPart3->setGeometry(QRect(140, 0, 42, 27));
//m_pIpPart4->setGeometry(QRect(210, 0, 42, 27));
pDot1->setText(".");
//m_pDot1->setGeometry(QRect(48, 5, 16, 16));
//m_pDot1->setAlignment(Qt::AlignCenter);
pDot2->setText(".");
//m_pDot2->setGeometry(QRect(118, 5, 16, 16));
//m_pDot2->setAlignment(Qt::AlignCenter);
pDot3->setText(".");
//m_pDot3->setGeometry(QRect(188, 5, 16, 16));
//m_pDot3->setAlignment(Qt::AlignCenter);
layout->addWidget(pIpPart1);
layout->addWidget(pDot1);
layout->addWidget(pIpPart2);
layout->addWidget(pDot2);
layout->addWidget(pIpPart3);
layout->addWidget(pDot3);
layout->addWidget(pIpPart4);
QWidget::setTabOrder(pIpPart1, pIpPart2);
QWidget::setTabOrder(pIpPart2, pIpPart3);
QWidget::setTabOrder(pIpPart3, pIpPart4);
pIpPart1->SetNextEdit(pIpPart2);
pIpPart2->SetNextEdit(pIpPart3);
pIpPart3->SetNextEdit(pIpPart4);
pIpPart2->SetPreEdit(pIpPart1);
pIpPart3->SetPreEdit(pIpPart2);
pIpPart4->SetPreEdit(pIpPart3);
connect(pIpPart1, SIGNAL(textChanged(const QString&)), this, SLOT(OnTextChanged(const QString&)));
connect(pIpPart2, SIGNAL(textChanged(const QString&)), this, SLOT(OnTextChanged(const QString&)));
connect(pIpPart3, SIGNAL(textChanged(const QString&)), this, SLOT(OnTextChanged(const QString&)));
connect(pIpPart4, SIGNAL(textChanged(const QString&)), this, SLOT(OnTextChanged(const QString&)));
connect(pIpPart1, SIGNAL(textEdited(const QString&)), this, SLOT(OnTextEdited(const QString&)));
connect(pIpPart2, SIGNAL(textEdited(const QString&)), this, SLOT(OnTextEdited(const QString&)));
connect(pIpPart3, SIGNAL(textEdited(const QString&)), this, SLOT(OnTextEdited(const QString&)));
connect(pIpPart4, SIGNAL(textEdited(const QString&)), this, SLOT(OnTextEdited(const QString&)));
}
IPLineEdit::~IPLineEdit()
{
}
void IPLineEdit::OnTextChanged(const QString &/*text*/)
{
QString strIpPart1 = pIpPart1->text();
QString strIpPart2 = pIpPart2->text();
QString strIpPart3 = pIpPart3->text();
QString strIpPart4 = pIpPart4->text();
QString strIpAddr = QString("%1.%2.%3.%4")
.arg(strIpPart1)
.arg(strIpPart2)
.arg(strIpPart3)
.arg(strIpPart4);
emit textChanged(strIpAddr);
}
void IPLineEdit::OnTextEdited(const QString &/*text*/)
{
QString strIpPart1 = pIpPart1->text();
QString strIpPart2 = pIpPart2->text();
QString strIpPart3 = pIpPart3->text();
QString strIpPart4 = pIpPart4->text();
QString strIpAddr = QString("%1.%2.%3.%4")
.arg(strIpPart1)
.arg(strIpPart2)
.arg(strIpPart3)
.arg(strIpPart4);
emit textEdited(strIpAddr);
}
void IPLineEdit::setText(const QString &text)
{
QString strIpAddr = text;
QRegExp regexp("((2[0-4]\\d|25[0-5]|[01]?\\d\\d?)\\.){3}(2[0-4]\\d|25[0-5]|[01]?\\d\\d?)");
QRegExpValidator regexp_validator(regexp, this);
int iPos = 0;
QValidator::State state = regexp_validator.validate(strIpAddr, iPos);
if(state != QValidator::Acceptable)
{
return ;
}
QStringList ipAddrList = text.split(".");
//int iCount = ipAddrList.size();
QString strIpPart1 = ipAddrList.at(0);
QString strIpPart2 = ipAddrList.at(1);
QString strIpPart3 = ipAddrList.at(2);
QString strIpPart4 = ipAddrList.at(3);
pIpPart1->setText(strIpPart1);
pIpPart2->setText(strIpPart2);
pIpPart3->setText(strIpPart3);
pIpPart4->setText(strIpPart4);
}
QString IPLineEdit::GetText()
{
QString strIpPart1 = pIpPart1->text();
QString strIpPart2 = pIpPart2->text();
QString strIpPart3 = pIpPart3->text();
QString strIpPart4 = pIpPart4->text();
return QString("%1.%2.%3.%4")
.arg(strIpPart1)
.arg(strIpPart2)
.arg(strIpPart3)
.arg(strIpPart4);
}
void IPLineEdit::SetStyleSheet(const QString &styleSheet)
{
pIpPart1->setStyleSheet(styleSheet);
pIpPart2->setStyleSheet(styleSheet);
pIpPart3->setStyleSheet(styleSheet);
pIpPart4->setStyleSheet(styleSheet);
}
IpPartLineEdit::IpPartLineEdit(QWidget *parent)
: QLineEdit(parent)
{
preEdit = nullptr;
nextEdit = nullptr;
this->setMaxLength(3);
this->setFrame(false);
this->setAlignment(Qt::AlignCenter);
QValidator *validator = new QIntValidator(0, 255, this);
this->setValidator(validator);
connect(this, SIGNAL(textEdited(const QString&)),
this, SLOT(OnTextEdited(const QString&)));
}
IpPartLineEdit::~IpPartLineEdit()
{
}
void IpPartLineEdit::SetPreEdit(QLineEdit *pre)
{
preEdit = pre;
}
void IpPartLineEdit::SetNextEdit(QLineEdit *next)
{
nextEdit = next;
}
void IpPartLineEdit::focusInEvent(QFocusEvent *event)
{
this->selectAll();
QLineEdit::focusInEvent(event);
}
void IpPartLineEdit::keyPressEvent(QKeyEvent *event)
{
if(event->key() == Qt::Key_Period)
{
if(nextEdit &&
(this->text().size()!=0) &&
(!this->hasSelectedText()))
{
nextEdit->setFocus();
nextEdit->selectAll();
}
}
if(event->key() == Qt::Key_Backspace)
{
if(preEdit &&
(this->text().size() == 0))
{
preEdit->setFocus();
preEdit->setCursorPosition(preEdit->text().size() );
}
}
QLineEdit::keyPressEvent(event);
}
void IpPartLineEdit::OnTextEdited(const QString & text)
{
QIntValidator v(0, 255, this);
QString ipAddr = text;
int iPos = 0;
QValidator::State state = v.validate(ipAddr, iPos);
if(state != QValidator::Acceptable)
{
return ;
}
if(ipAddr.size() <= 1)
{
return ;
}
if(ipAddr.size() == 2)
{
int iIpNum = ipAddr.toInt();
if(iIpNum > 25)
{
if(nextEdit)
{
nextEdit->setFocus();
nextEdit->selectAll();
}
}
}
else
{
if(nextEdit)
{
nextEdit->setFocus();
nextEdit->selectAll();
}
}
}
然后拖拽一个QFrame控件,右键提升为… 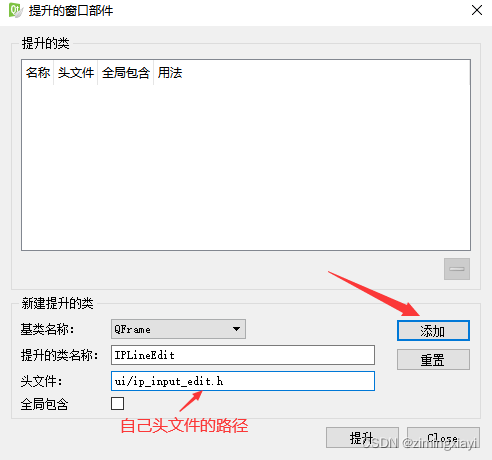 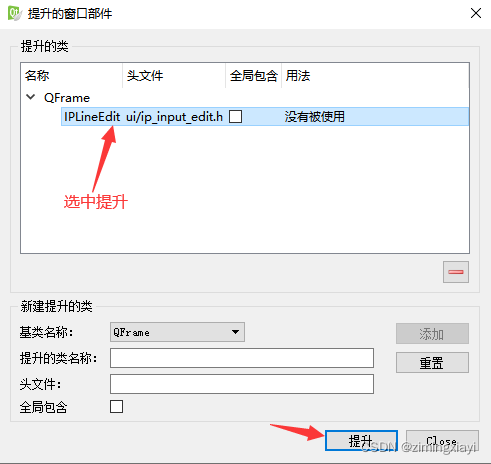
|