使用udp通信向服务器端发送文件:word.txt
服务器接收到文件后保存到项目文件: aaa.txt
需要通过字节数组缓冲流的形式发送文件
思路:
先在客户端创建字节输入流将需要发送的数据加载到字节数组里,每次发送的字节数组大小是1024。
然后循环发送该文件的数据到服务器端中。
服务器端先使用字节数组循环接受客户端发送过来的数据。然后创建字节输出流用于将客户端发送过来的数据保存到本地。
客户端:
public static void main(String[] args) throws Exception{
log.info("------------开始-----------");
DatagramSocket ss = new DatagramSocket(8080);
BufferedInputStream bis = new BufferedInputStream(new FileInputStream("word.txt"));
// 每次传输的字节大小是1024
byte bytes[] = new byte[1024];
int len;
while ((len = bis.read(bytes,0, bytes.length)) != -1){
DatagramPacket date = new DatagramPacket(bytes,0,bytes.length,InetAddress.getByName("127.0.0.1"),8090);
ss.send(date);
}
bytes = "quit".getBytes();
ss.send(new DatagramPacket(bytes,0,bytes.length,InetAddress.getByName("127.0.0.1"),8090));
log.info("数据发送成功");
bis.close();
ss.close();
log.info("------------结束-----------");
}
服务器端:
public static void main(String[] args) throws Exception{
log.info("------------开始-----------");
DatagramSocket ss = new DatagramSocket(8090); // 服务器端的端口
byte bytes[] = new byte[1024]; // 用来接收从客户端发送过来的数据
// 服务器端接收客户端发送过来的数据并保存到 "aaa.txt"文件中
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream("aaa.txt"));
// 使用一个长度为bytes.length的字节数组存储从客户端发送过来的数据
DatagramPacket date = new DatagramPacket(bytes,0,bytes.length);
// 接收客户端发送过来的数据
while (true){
ss.receive(date);
bytes = date.getData();
bos.write(bytes,0, date.getLength());
bos.flush();
if(new String(bytes,0, date.getLength()).equals("quit") ){
break;
}
}
// 将数据写到缓冲区里
// String str = new String(date.getData(),0, date.getLength());
// 将接收到的数据保存到字节数组里
bos.close();
ss.close();
log.info("");
log.info("------------结束-----------");
}
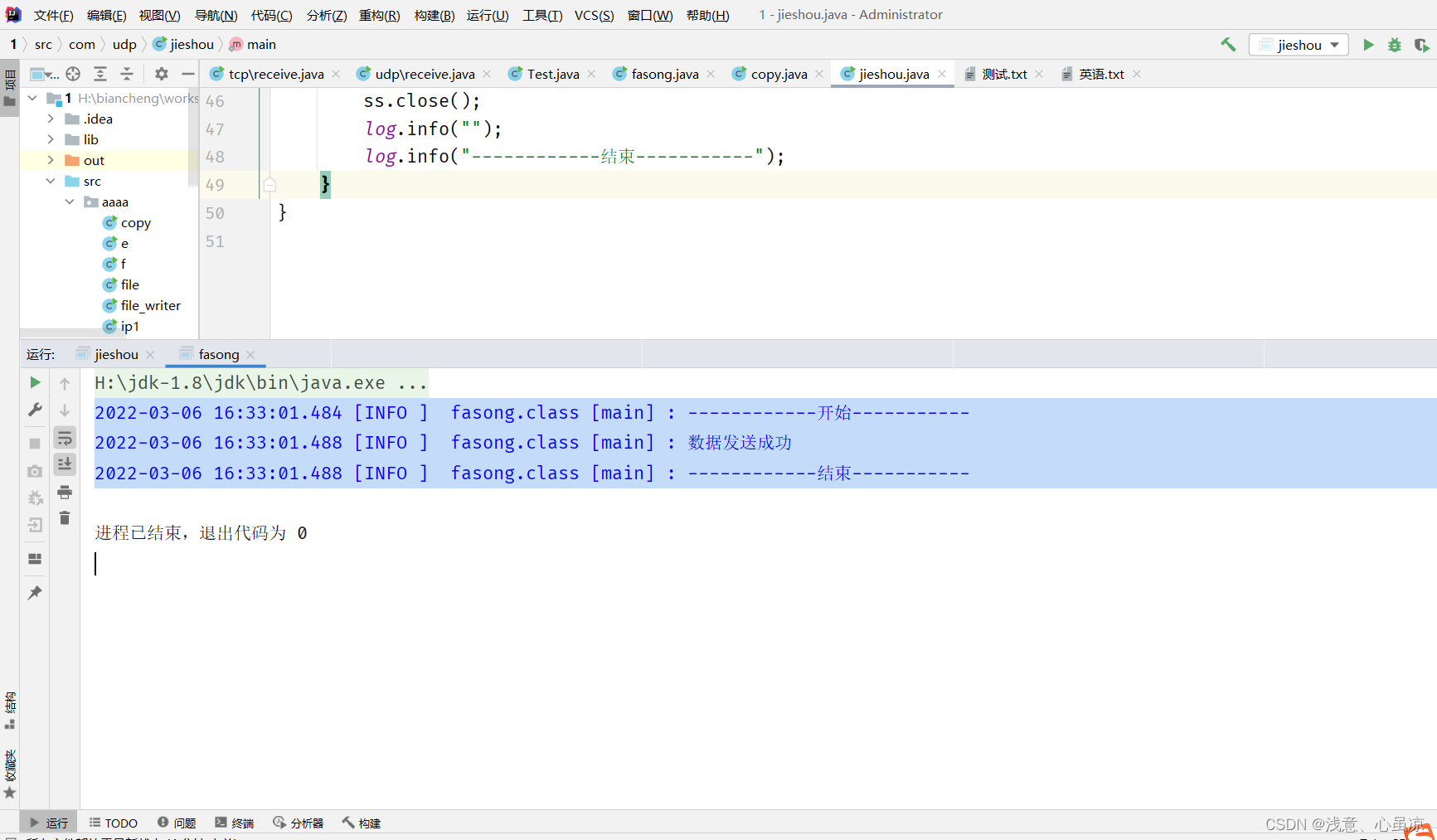
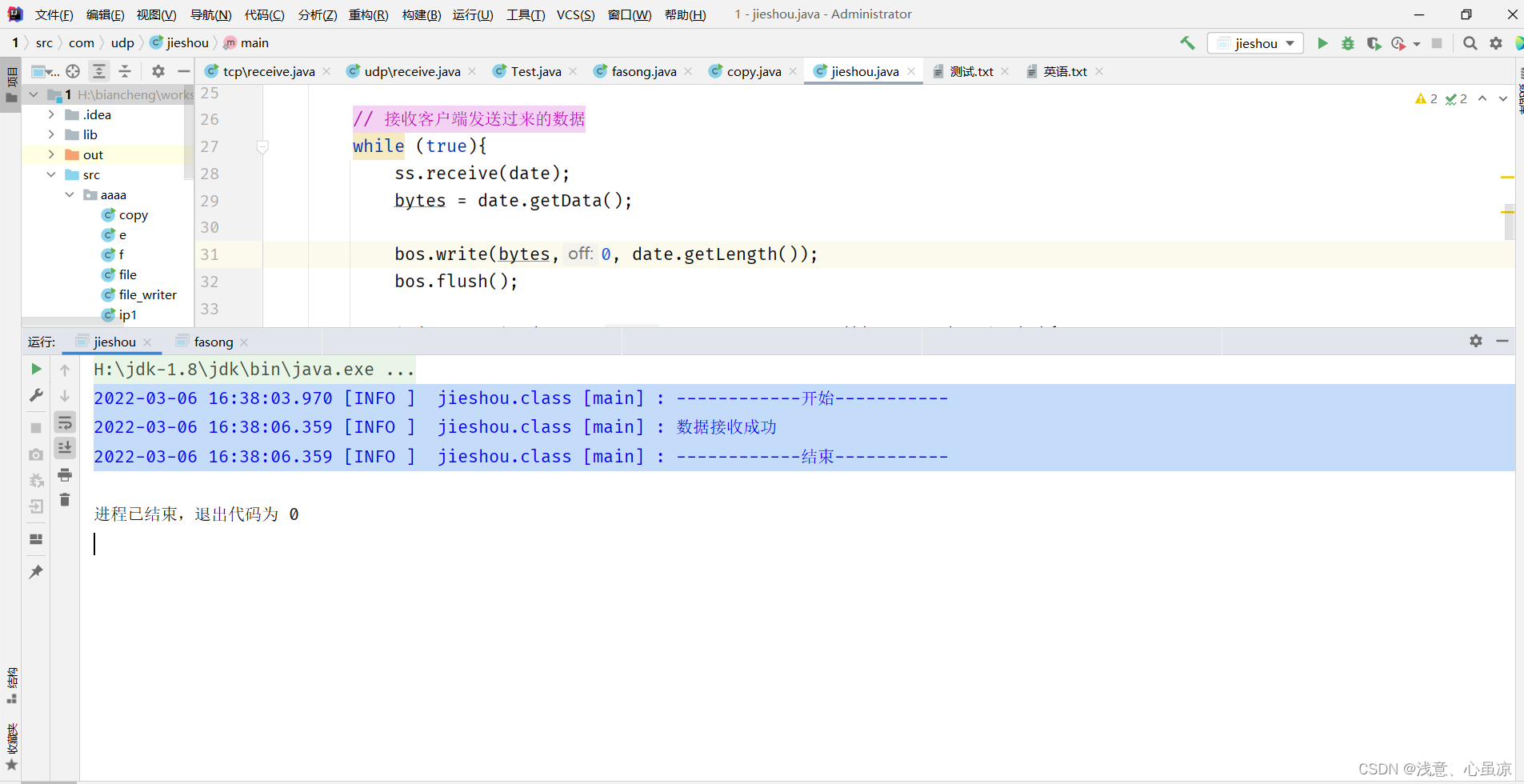
|