1、 定义变量
定义一个channel组,管理所有的channel,再定义一个map,管理用户与channel的对应关系
public class ChannelConfigs {
private static ChannelGroup channelGroup = new DefaultChannelGroup(GlobalEventExecutor.INSTANCE);
private static ConcurrentHashMap<String, Channel> userChannelMap = new ConcurrentHashMap<>();
public static ChannelGroup getChannelGroup() {
return channelGroup;
}
public static ConcurrentHashMap<String, Channel> getUserChannelMap() {
return userChannelMap;
}
}
2、人数实体类
public class UserInfo {
private static AtomicInteger uidGener = new AtomicInteger(1000000);
private int userId;
private String userName;
private String addr;
private Channel channel;
.....
getter()和setter方法省略
}
3、消息推送初始化
public class PushMsgServer{
private static final Logger logger = LoggerFactory.getLogger(PushMsgServer.class);
@Override
public void init(String host, int port) {
EventLoopGroup bossGroup = new NioEventLoopGroup(1);
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast("http-codec", new HttpServerCodec());
ch.pipeline().addLast("aggregator", new HttpObjectAggregator(65536));
ch.pipeline().addLast("http-chunked", new ChunkedWriteHandler());
ch.pipeline().addLast(new IdleStateHandler(60, 60, 60, TimeUnit.SECONDS));
ch.pipeline().addLast("handler", new PushMsgServerHandler());
}
});
Channel ch = b.bind(8082).sync().channel();
if (ch.isOpen()) {
logger.info("推送消息服务器启动成功...");
}
ch.closeFuture().sync();
} catch (Exception e) {
e.printStackTrace();
}
finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
@Override
public void startServer(String host, int port) {
Thread t = new Thread(new Runnable() {
@Override
public void run() {
init(host, port);
}
});
t.start();
}
public static void main() {
try {
new PushMsgServer().startServer("49.232.66.48", 8082);
} catch (Exception e) {
e.printStackTrace();
}
}
}
4、消息推送Handler
public class PushMsgServerHandler extends SimpleChannelInboundHandler<Object> {
private static final Logger logger = LoggerFactory.getLogger(PushMsgServerHandler.class);
private static AtomicInteger userCount = new AtomicInteger(0);
private WebSocketServerHandshaker handshaker;
@Override
public void channelRead0(ChannelHandlerContext ctx, Object msg) throws Exception {
if (msg instanceof FullHttpRequest) {
handleHttpRequest(ctx, (FullHttpRequest) msg);
}
else if (msg instanceof WebSocketFrame) {
handleWebSocketFrame(ctx, (WebSocketFrame) msg);
}
}
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
userCount.incrementAndGet();
logger.info("当前在线人数为{}:", userCount.intValue());
ChannelConfigs.getChannelGroup().add(ctx.channel());
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
logger.info("客户端断开连接:");
userCount.decrementAndGet();
logger.info("当前在线人数为{}:", userCount.intValue());
ChannelConfigs.getChannelGroup().remove(ctx.channel());
removeUserId(ctx);
}
@Override
public void userEventTriggered(ChannelHandlerContext ctx, Object evt) throws Exception {
if (evt instanceof IdleStateEvent) {
IdleStateEvent event = (IdleStateEvent) evt;
String type = "";
switch (event.state()) {
case READER_IDLE:
type = "读空闲";
break;
case WRITER_IDLE:
type = "写空闲";
break;
default:
type = "读写空闲";
}
logger.info("{}超时事件={}\n", ctx.channel().remoteAddress().toString(), type);
ctx.channel().close();
}
}
private void handleHttpRequest(ChannelHandlerContext ctx, FullHttpRequest req) throws Exception {
if (!req.decoderResult().isSuccess() || (!"websocket".equals(req.headers().get("Upgrade")))) {
sendHttpResponse(ctx, req, new DefaultFullHttpResponse(HTTP_1_1, BAD_REQUEST));
return;
}
WebSocketServerHandshakerFactory wsFactory = new WebSocketServerHandshakerFactory("ws://localhost:8082/websocket", null, false);
handshaker = wsFactory.newHandshaker(req);
if (handshaker == null) {
WebSocketServerHandshakerFactory.sendUnsupportedVersionResponse(ctx.channel());
} else {
handshaker.handshake(ctx.channel(), req);
}
}
private void handleWebSocketFrame(ChannelHandlerContext ctx, WebSocketFrame frame) {
if (frame instanceof CloseWebSocketFrame) {
handshaker.close(ctx.channel(), (CloseWebSocketFrame) frame.retain());
return;
}
if (frame instanceof PingWebSocketFrame) {
ctx.channel().write(new PongWebSocketFrame(frame.content().retain()));
return;
}
if (!(frame instanceof TextWebSocketFrame)) {
throw new UnsupportedOperationException(String.format("%s frame types not supported", frame.getClass().getName()));
}
String reqest = ((TextWebSocketFrame) frame).text();
ctx.channel().write(new TextWebSocketFrame(reqest + "这里是服务端:{}" + new Date()));
}
private void removeUserId(ChannelHandlerContext ctx) {
ChannelConfigs.getUserChannelMap().remove(ctx.channel());
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
ChannelConfigs.getChannelGroup().remove(ctx.channel());
removeUserId(ctx);
ctx.close();
}
private static void sendHttpResponse(ChannelHandlerContext ctx, FullHttpRequest req, FullHttpResponse res) {
if (res.getStatus().code() != 200) {
ByteBuf buf = Unpooled.copiedBuffer(res.getStatus().toString(),
CharsetUtil.UTF_8);
res.content().writeBytes(buf);
buf.release();
HttpUtil.setContentLength(res, res.content().readableBytes());
}
ChannelFuture f = ctx.channel().writeAndFlush(res);
if (!HttpUtil.isKeepAlive(req) || res.status().code() != 200) {
f.addListener(ChannelFutureListener.CLOSE);
}
}
@Override
public void channelReadComplete(ChannelHandlerContext ctx) throws Exception {
ctx.flush();
}
}
4、消息推送Service
public interface PushService {
void pushMsgToOne(String userId, String msg);
void pushMsgToAll(String msg);
}
5、消息推送实现类
public class PushServiceImpl implements PushService {
@Override
public void pushMsgToOne(String userId, String msg) {
ConcurrentHashMap<String, Channel> userChannelMap = ChannelConfigs.getUserChannelMap();
Channel channel = userChannelMap.get(userId);
channel.writeAndFlush(new TextWebSocketFrame(msg));
}
@Override
public void pushMsgToAll(String msg) {
ChannelConfigs.getChannelGroup().writeAndFlush(new TextWebSocketFrame(msg));
}
}
6、前端测试
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
Netty WebSocket 时间服务器
</head>
<br>
<body>
<br>
<script type="text/javascript">
var socket;
if (!window.WebSocket) {
window.WebSocket = window.MozWebSocket;
}
if (window.WebSocket) {
socket = new WebSocket("ws://localhost:8082/websocket");
socket.onmessage = function (event) {
var ta = document.getElementById('responseText');
ta.value = "";
ta.value = event.data
};
socket.onopen = function (event) {
var ta = document.getElementById('responseText');
ta.value = "打开WebSocket服务正常,浏览器支持WebSocket!";
};
socket.onclose = function (event) {
var ta = document.getElementById('responseText');
ta.value = "";
ta.value = "WebSocket 关闭!";
};
}
else {
alert("抱歉,您的浏览器不支持WebSocket协议!");
}
function send(message) {
if (!window.WebSocket) {
return;
}
if (socket.readyState == WebSocket.OPEN) {
socket.send(message);
}
else {
alert("WebSocket连接没有建立成功!");
}
}
</script>
<form onsubmit="return false;">
<input type="text" name="message" value="Netty最佳实践"/>
<br><br>
<input type="button" value="发送WebSocket请求消息" onclick="send(this.form.message.value)"/>
<hr color="blue"/>
<h3>服务端返回的应答消息</h3>
<textarea id="responseText" style="width:500px;height:300px;"></textarea>
</form>
</body>
</html>
7、测试结果
开启三个客户端:这里加入了心跳机制,当超过一分钟没有写操作时,断开连接 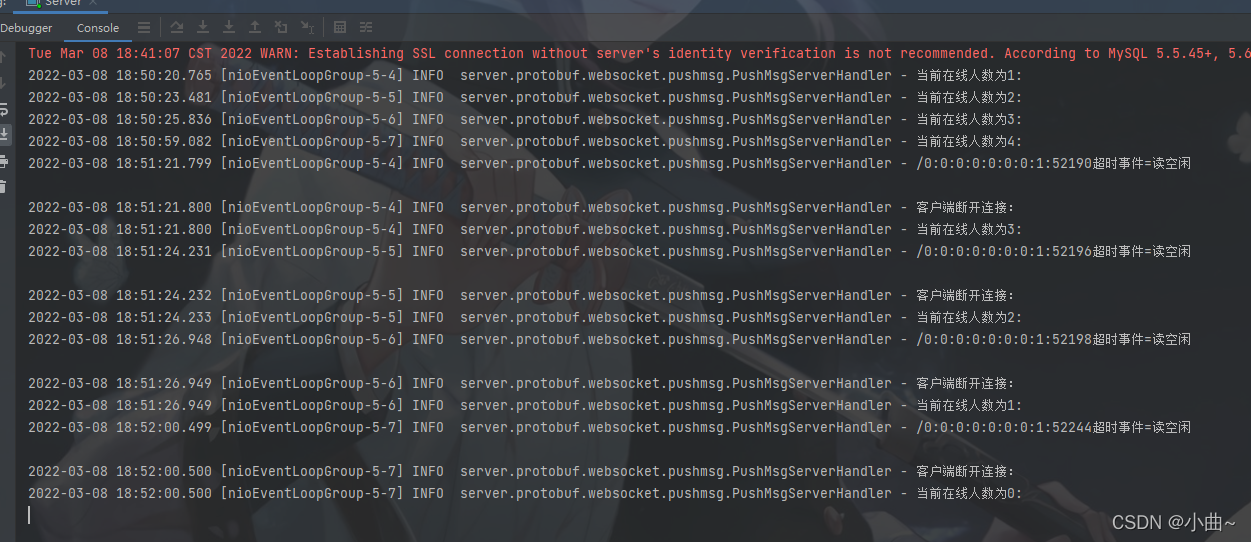
发送消息: 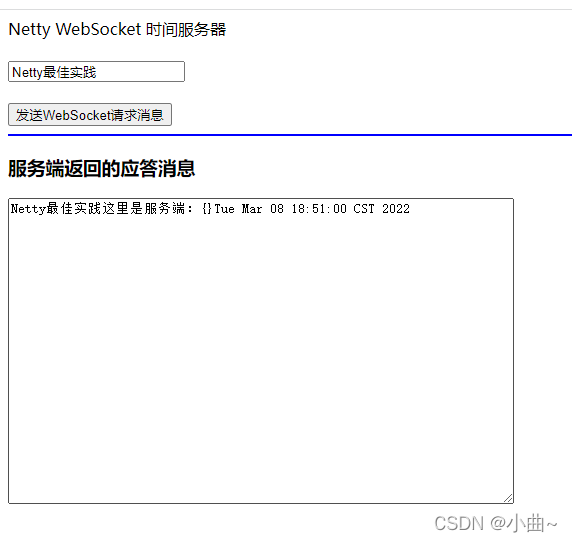 OVER!!!
|