openssl
openssl支持各种算法,在网络传输中我们需要用到各类算法,尤其是在安全协议里面,hmac算法是必须要用得,dtls协议里面也会用到各类对称算法,下面定义一个类,使用openssl得常用算法中得基本算法。
#pragma once
#include <stdio.h>
#include <string.h>
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
class c_hmac
{
string v_key;
string v_data;
string v_name;
public:
c_hmac(string alo_name,string key, string data)
{
v_key = key;
v_data = data;
v_name = alo_name;
}
uint8_t * hmac_algo(unsigned int &len);
};
实现
实现比较简单,版本使用openssl 1.1.1 版
#include "hmac.h"
#include <openssl/hmac.h>
#include <string.h>
#include <iostream>
#pragma comment(lib,"libcrypto.lib")
using namespace std;
#define strcasecmp _stricmp
uint8_t * c_hmac::hmac_algo(unsigned int &len) {
const EVP_MD * engine = NULL;
const char *name = v_name.c_str();
if (strcasecmp("sha512", name) == 0) {
engine = EVP_sha512();
}
else if (strcasecmp("sha256", name) == 0) {
engine = EVP_sha256();
}
else if (strcasecmp("sha1", name) == 0) {
engine = EVP_sha1();
}
else if (strcasecmp("md5", name) == 0) {
engine = EVP_md5();
}
else if (strcasecmp("sha224", name) == 0) {
engine = EVP_sha224();
}
else if (strcasecmp("sha384", name) == 0) {
engine = EVP_sha384();
}
else {
cout << "Algorithm " << name << " is not supported now!" << endl;
return NULL;
}
uint8_t *output = (uint8_t *)malloc(EVP_MAX_MD_SIZE);
HMAC_CTX *hctx = HMAC_CTX_new();
HMAC_CTX_reset(hctx);
HMAC_Init_ex(hctx, v_key.c_str(), v_key.size(), engine, NULL);
HMAC_Update(hctx, (uint8_t *)v_data.c_str(), v_data.size());
HMAC_Final(hctx, output, &len);
HMAC_CTX_free(hctx);
return output;
}
调用
调用分别是密码方法名称,我们使用sha256,关键密字和需要加密得数据,调用如下
#include "hmac.h"
using namespace std;
int main(int argc, char * argv[])
{
c_hmac hmac("sha256","qianbo", "this is a test");
unsigned int len = 0;
uint8_t *ret = hmac.hmac_algo(len);
if (NULL == ret) {
cout << "Algorithm fail!" << endl;
}
for (unsigned int i = 0; i < len; i++) {
printf("%02x-", (unsigned int)ret[i]);
}
cout << endl;
if (ret) {
free(ret);
}
return 0;
}
结果
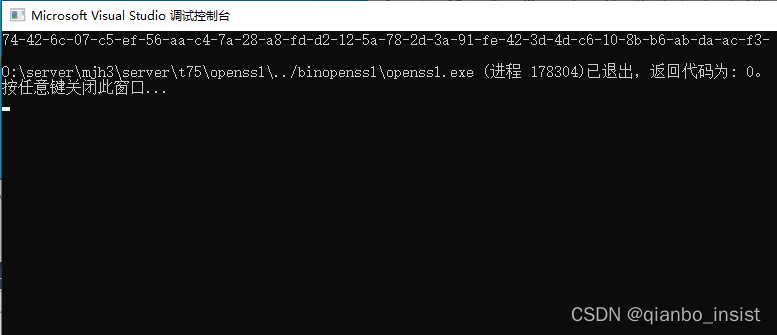 计算得结果如图所示,结果为32字节
|