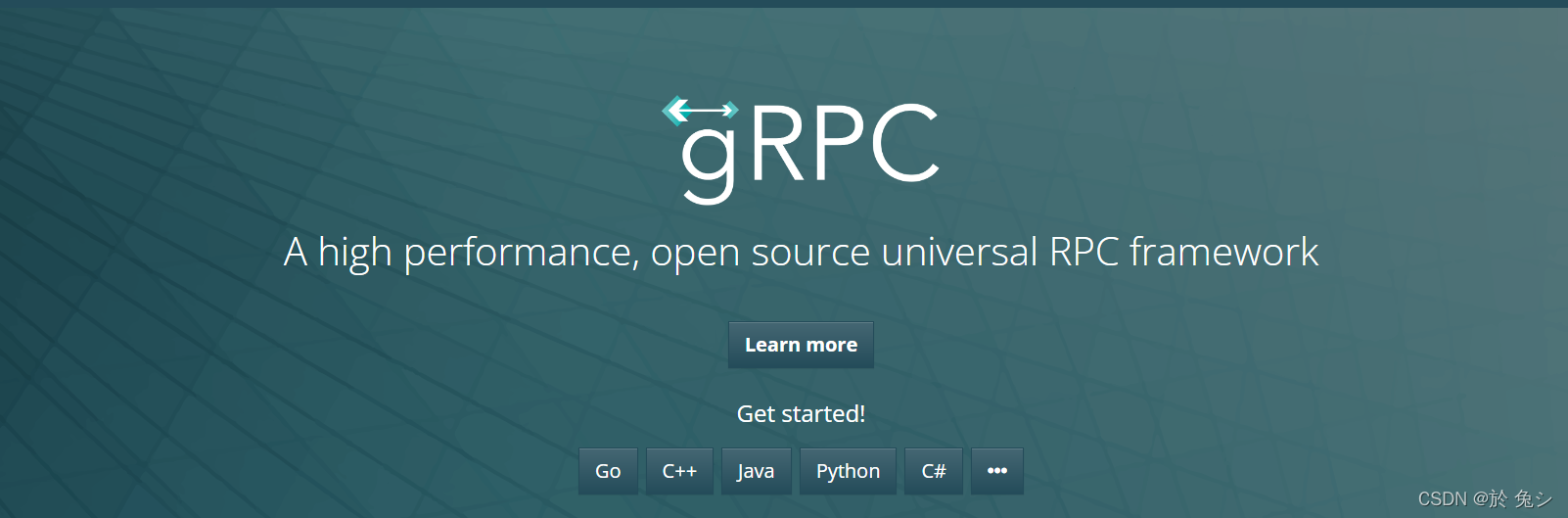
错误码与服务端客户端发送与接收错误信息
失败的code码表格
Code | 名称 | 解释 |
---|
1 | Canceled | 取消操作 | 2 | Unknown | 未知操作 | 3 | InvalidArgument | 客户端传入的参数有误 | 4 | DeadlineExceeded | 超时 | 5 | NotFound | 找不到资源 | 6 | AlreadyExsts | 资源已存在 | 7 | PremissionDenied | 无相关权限 | 8 | ResourceExhasted | 资源耗尽 (如分页没有数据了) | 9 | FailedPrecondition | 操作拒绝(如阻止删除数据库数据操作) | 10 | Aborted | 终止操作 | 11 | OutOfRange | 超过有效范围 | 12 | Unimplemented | 未执行或未支持的操作 | 13 | Internal | 发生了一些意外(逻辑认为很不好) | 14 | Unavailable | 服务不可用 | 15 | DataLoss | 数据丢失 不可恢复 | 16 | Unauthorized | 无效认证 |
代码示例
1. helloworld.proto:
syntax = "proto3";
package test;
service grpc_test {
rpc
test_client(stream test_client_requests)
returns(test_client_response){}
}
message test_client_requests{
string data = 1;
}
message test_client_response{
string result = 1;
}
2. server.py :
import time
import grpc
import helloworld_pb2 as pb2
import helloworld_pb2_grpc as pb2_grpc
from concurrent import futures
class grpc_test(pb2_grpc.grpc_testServicer):
def test_client(self, request_iterator, context):
index = 0
for request in request_iterator:
data = request.data
index += 1
result = f'receive {data},index:{index}'
print(result)
time.sleep(1)
if index == 2:
import json
response_data = {"sub_code": 40301, "message": 'xxx错误!'}
context.set_details(json.dumps(response_data))
context.set_code(grpc.StatusCode.DATA_LOSS)
raise context
return pb2.test_client_response(result="ok")
def run():
grpc_server = grpc.server(
futures.ThreadPoolExecutor(max_workers=4)
)
pb2_grpc.add_grpc_testServicer_to_server(grpc_test(), grpc_server)
grpc_server.add_insecure_port('0.0.0.0:50010')
print('server will start at 0.0.0.0:50010')
grpc_server.start()
try:
while 1:
time.sleep(3600)
except Exception as e:
grpc_server.stop(0)
print(e)
if __name__ == '__main__':
run()
3. client.py :
import json
import random
import time
import grpc
import helloworld_pb2 as pb2
import helloworld_pb2_grpc as pb2_grpc
def test():
"""
循环发送流数据
每一秒中向服务端发送流数据
:return:
"""
index = 0
while True:
index += 1
time.sleep(1)
str_random = str(random.random())
yield pb2.test_client_requests(
data=str_random
)
if index == 15:
break
def run():
conn = grpc.insecure_channel('127.0.0.1:50010')
client = pb2_grpc.grpc_testStub(channel=conn)
try:
response = client.test_client(test(), timeout=15)
print(response)
except Exception as e:
print(dir(e))
code_name = e.code().name
code_value = e.code().value
details = e.details()
print(code_name)
print(code_value)
print(json.loads(details))
if __name__ == '__main__':
run()
结果图:
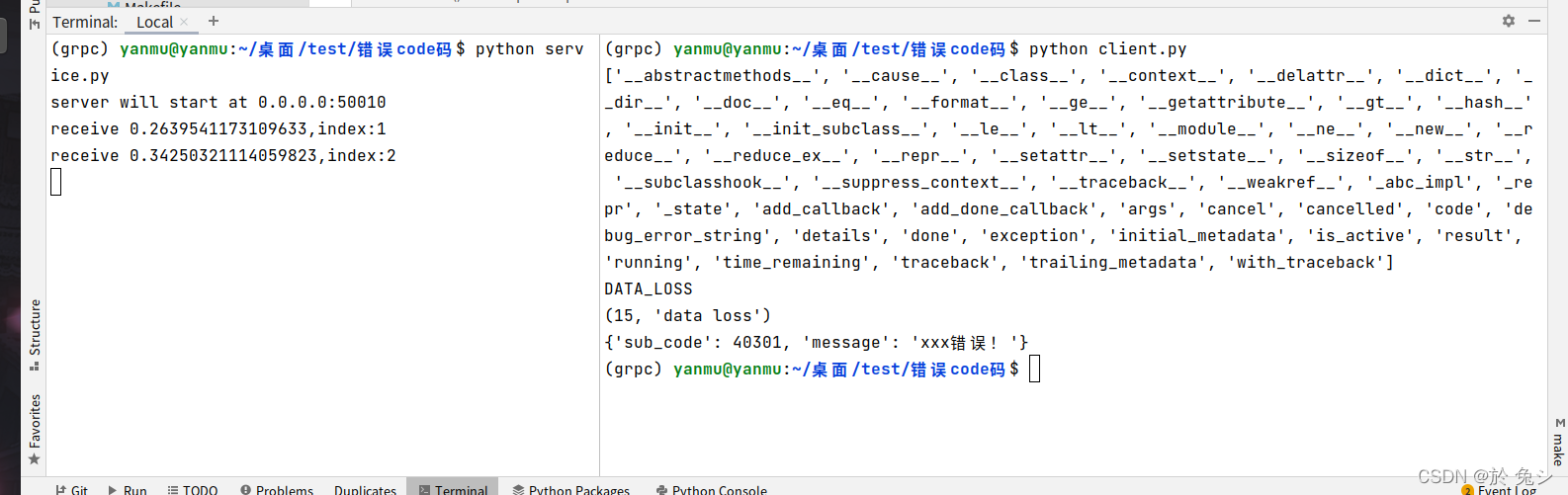
|