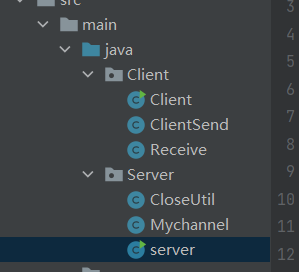
视频教程连接 server.java
package Server;
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.ArrayList;
import java.util.List;
public class server {
public static List<Mychannel> list=new ArrayList<Mychannel>();
public static void main(String[] args) throws IOException {
System.out.println("服务器已开启");
ServerSocket server=new ServerSocket(9999);
while (true){
Socket socket= server.accept();
Mychannel mychannel=new Mychannel(socket);
list.add(mychannel);
new Thread(mychannel).start();
}
}
}
CloseUtil.java
package Server;
import java.io.Closeable;
import java.io.IOException;
public class CloseUtil {
public static void CloseAll(Closeable...able) {
for (Closeable closeable:able){
if(closeable!=null){
try {
closeable.close();
}catch (IOException e){
e.printStackTrace();
}
}
}
}
}
Mychannel.java
package Server;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.net.Socket;
import java.util.List;
public class Mychannel implements Runnable {
private DataInputStream dis;
private DataOutputStream dos;
private boolean flag=true;
public Mychannel(Socket client) {
try {
dis=new DataInputStream(client.getInputStream());
dos=new DataOutputStream(client.getOutputStream());
}catch (IOException e){
flag=false;
CloseUtil.CloseAll(dis,dos);
}
}
private String recevie(){
String str="";
try {
str=dis.readUTF();
}catch (IOException e){
flag=false;
CloseUtil.CloseAll(dis,dos);
server.list.remove(this);
}
return str;
}
private void Send(String str){
if(str!=null&&str.length()!=0){
try {
dos.writeUTF(str);
dos.flush();
}catch (IOException e){
flag=false;
CloseUtil.CloseAll(dos,dis);
server.list.remove(this);
}
}
}
private void sendother(){
String str=this.recevie();
List<Mychannel> list=server.list;
for (Mychannel other:list){
if (other==this){
continue;
}
other.Send(str);
}
}
@Override
public void run(){
while (flag)
sendother();
}
}
Client.java
package Client;
import javax.sound.midi.MidiMessage;
import javax.sound.midi.Receiver;
import java.io.IOException;
import java.net.Socket;
public class Client {
public static void main(String[] args) throws IOException {
Socket client=new Socket("localhost",9999);
ClientSend clientSend=new ClientSend(client);
Receive receive=new Receive(client);
new Thread(clientSend).start();
new Thread(receive).start();
}
}
ClientSend.java
package Client;
import Server.CloseUtil;
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.Socket;
public class ClientSend implements Runnable{
private BufferedReader br;
private DataOutputStream dos;
private boolean flag=true;
public ClientSend(){
br=new BufferedReader(new InputStreamReader(System.in));
}
public ClientSend(Socket client) {
try {
br=new BufferedReader(new InputStreamReader(System.in));
dos=new DataOutputStream(client.getOutputStream());
}catch (IOException e){
flag=false;
CloseUtil.CloseAll(dos,client);
}
}
private String getMessage(){
String str="";
try {
str=br.readLine();
}catch (IOException e){
flag=false;
CloseUtil.CloseAll(br);
}
return str;
}
public void send(String str){
try {
dos.writeUTF(str);
dos.flush();
}catch (IOException e){
flag=false;
CloseUtil.CloseAll(dos);
}
}
@Override
public void run() {
while (flag){
this.send(getMessage());
}
}
}
Receive.java
package Client;
import Server.CloseUtil;
import java.io.DataInputStream;
import java.io.IOException;
import java.net.Socket;
public class Receive implements Runnable{
private DataInputStream dis;
private boolean flag=true;
public Receive(Socket client) {
try {
dis=new DataInputStream(client.getInputStream());
}catch (IOException e){
flag=false;
CloseUtil.CloseAll(dis,client);
}
}
private String getMessage(){
String str="";
try {
str=dis.readUTF();
}catch (IOException e){
flag=false;
CloseUtil.CloseAll(dis);
}
return str;
}
@Override
public void run(){
while (flag){
System.out.println(this.getMessage());
}
}
}
|