简介
官网:http://hc.apache.org/ 使用场景:公司需要跟分子公司做数据交互,需要做服务端和客户端,首先考虑的就是http和webservice,这里选用http jar包
<!-- httpclient传送文件用的 -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpmime</artifactId>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
jdk原生api发送http请求
用原生的jdk请求一个http,然后获取它的源码输出
@Test
void test() throws Exception {
String urlPath = "https://www.baidu.com/";
URL url = new URL(urlPath);
URLConnection urlConnection = url.openConnection();
HttpURLConnection httpURLConnection = (HttpURLConnection) urlConnection;
httpURLConnection.setRequestMethod("GET");
try (
InputStream inputStream = httpURLConnection.getInputStream();
InputStreamReader inputStreamReader = new InputStreamReader(inputStream, StandardCharsets.UTF_8);
BufferedReader bufferedReader = new BufferedReader(inputStreamReader);
) {
String line;
while ((line = bufferedReader.readLine()) != null) {
System.out.println(line);
}
}
}
httpclient
无参的get请求
@Test
void httpClientsTest() throws Exception {
CloseableHttpClient closeableHttpClient = HttpClients.createDefault();
String urlPath = "https://www.baidu.com/";
HttpGet httpGet=new HttpGet(urlPath);
httpGet.addHeader("User-Agent","Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/86.0.4240.198 Safari/537.36");
httpGet.addHeader("Referer","https://www.baidu.com/");
CloseableHttpResponse response=null;
try {
response=closeableHttpClient.execute(httpGet);
HttpEntity entity = response.getEntity();
String toStringResult = EntityUtils.toString(entity, StandardCharsets.UTF_8);
System.out.println(toStringResult);
EntityUtils.consume(entity);
}catch (Exception e){
e.printStackTrace();
}finally {
if (closeableHttpClient!=null){
closeableHttpClient.close();
}
if(response!=null){
response.close();
}
}
}
有参的请求
对比无参的请求,只修改了一部分
String pwd="abcd+ |2312";
pwd= URLEncoder.encode(pwd,StandardCharsets.UTF_8.name());
String urlPath = "http://localhost:8080/login?userName="+username+"&passWord="+pwd;
以下是修改后的整体代码
@Test
void httpClientsController() throws Exception {
CloseableHttpClient closeableHttpClient = HttpClients.createDefault();
String username="张三";
String pwd="abcd+ |2312";
pwd= URLEncoder.encode(pwd,StandardCharsets.UTF_8.name());
String urlPath = "http://localhost:8080/login?userName="+username+"&passWord="+pwd;
HttpGet httpGet=new HttpGet(urlPath);
httpGet.addHeader("User-Agent","Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/86.0.4240.198 Safari/537.36");
httpGet.addHeader("Referer","https://www.baidu.com/");
CloseableHttpResponse response=null;
try {
response=closeableHttpClient.execute(httpGet);
HttpEntity entity = response.getEntity();
String toStringResult = EntityUtils.toString(entity, StandardCharsets.UTF_8);
System.out.println(toStringResult);
EntityUtils.consume(entity);
}catch (Exception e){
e.printStackTrace();
}finally {
if (closeableHttpClient!=null){
closeableHttpClient.close();
}
if(response!=null){
response.close();
}
}
}
获取响应头
在有参请求的基础上,修改了部分代码
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if(HttpStatus.SC_OK==statusCode){
System.out.println(statusCode+"-请求成功");
Header[] headers = response.getAllHeaders();
for (Header header:headers){
System.out.println("响应头:"+header.getName()+"的值:"+header.getValue());
}
HttpEntity entity = response.getEntity();
System.out.println("content-type:"+entity.getContentType());
以下是修改后全代码
@Test
void httpClientsController() throws Exception {
CloseableHttpClient closeableHttpClient = HttpClients.createDefault();
String username="张三";
String pwd="abcd+ |2312";
pwd= URLEncoder.encode(pwd,StandardCharsets.UTF_8.name());
String urlPath = "http://localhost:8080/login?userName="+username+"&passWord="+pwd;
HttpGet httpGet=new HttpGet(urlPath);
httpGet.addHeader("User-Agent","Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/86.0.4240.198 Safari/537.36");
httpGet.addHeader("Referer","https://www.baidu.com/");
CloseableHttpResponse response=null;
try {
response=closeableHttpClient.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if(HttpStatus.SC_OK==statusCode){
System.out.println(statusCode+"-请求成功");
Header[] headers = response.getAllHeaders();
for (Header header:headers){
System.out.println("响应头:"+header.getName()+"的值:"+header.getValue());
}
HttpEntity entity = response.getEntity();
System.out.println("content-type:"+entity.getContentType());
String toStringResult = EntityUtils.toString(entity, StandardCharsets.UTF_8);
System.out.println(toStringResult);
EntityUtils.consume(entity);
}else{
System.out.println(statusCode+"-请求异常");
}
}catch (Exception e){
e.printStackTrace();
}finally {
if (closeableHttpClient!=null){
closeableHttpClient.close();
}
if(response!=null){
response.close();
}
}
}
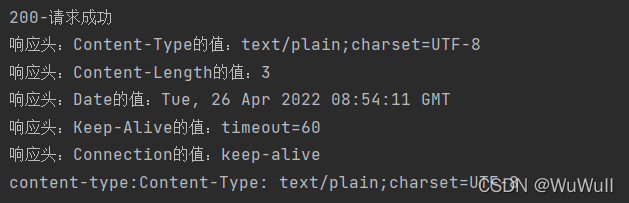
图片保存到本地
@Test
void httpClientsSavePicture() throws Exception {
CloseableHttpClient closeableHttpClient = HttpClients.createDefault();
String urlPath = "https://www.baidu.com/img/PCtm_d9c8750bed0b3c7d089fa7d55720d6cf.png";
HttpGet httpGet=new HttpGet(urlPath);
CloseableHttpResponse response=null;
try {
response=closeableHttpClient.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if(HttpStatus.SC_OK==statusCode){
System.out.println(statusCode+"-请求成功");
HttpEntity entity = response.getEntity();
String contentType = entity.getContentType().getValue();
String suffix=".jpg";
if(contentType.contains("jpg")||contentType.contains("jpeg")){
suffix=".jpg";
}else if(contentType.contains("png")){
suffix=".png";
}
byte[] bytes = EntityUtils.toByteArray(entity);
String localAbsPath="D:\\123"+suffix;
FileOutputStream fileOutputStream=new FileOutputStream(localAbsPath);
fileOutputStream.write(bytes);
fileOutputStream.close();
EntityUtils.consume(entity);
}else{
System.out.println(statusCode+"-请求异常");
}
}catch (Exception e){
e.printStackTrace();
}finally {
if (closeableHttpClient!=null){
closeableHttpClient.close();
}
if(response!=null){
response.close();
}
}
}
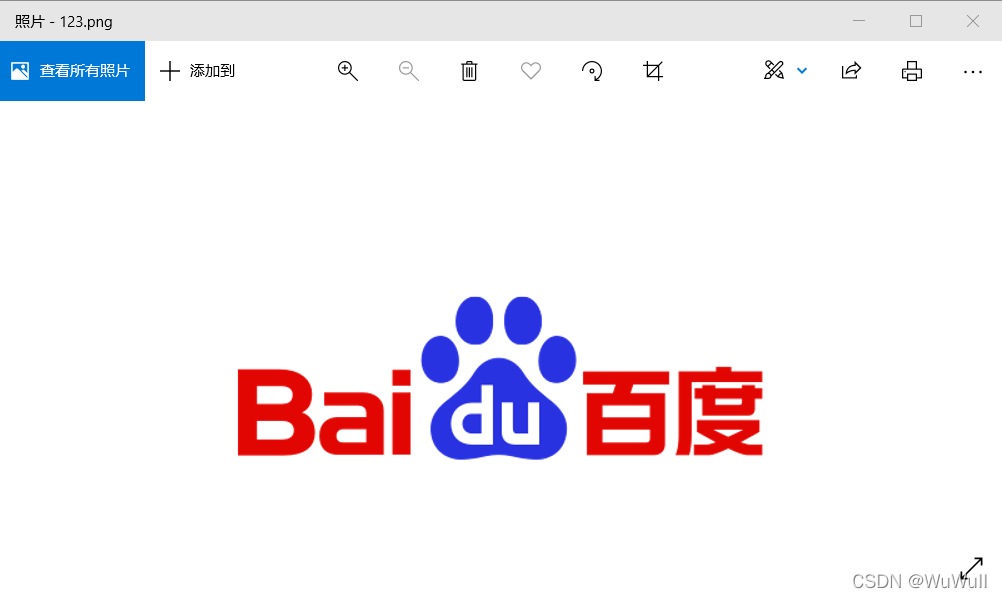
设置访问代理
设置访问代理
HttpHost proxy=new HttpHost("203.30.191.46",80);
RequestConfig requestConfig = RequestConfig.custom().setProxy(proxy).build();
httpGet.setConfig(requestConfig);
全代码
@Test
void httpClientsProxy() throws Exception {
CloseableHttpClient closeableHttpClient = HttpClients.createDefault();
String urlPath = "https://www.baidu.com/";
HttpGet httpGet=new HttpGet(urlPath);
HttpHost proxy=new HttpHost("203.30.191.46",80);
RequestConfig requestConfig = RequestConfig.custom().setProxy(proxy).build();
httpGet.setConfig(requestConfig);
CloseableHttpResponse response=null;
try {
response=closeableHttpClient.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if(HttpStatus.SC_OK==statusCode){
System.out.println(statusCode+"-请求成功");
HttpEntity entity = response.getEntity();
String toStringResult = EntityUtils.toString(entity, StandardCharsets.UTF_8);
System.out.println(toStringResult);
EntityUtils.consume(entity);
}else{
System.out.println(statusCode+"-请求异常");
}
}catch (Exception e){
e.printStackTrace();
}finally {
if (closeableHttpClient!=null){
closeableHttpClient.close();
}
if(response!=null){
response.close();
}
}
}
连接超时和读取超时
设置超时代码
RequestConfig requestConfig = RequestConfig.custom()
.setConnectTimeout(5000)
.setSocketTimeout(5000)
.setConnectionRequestTimeout(5000)
.build();
httpGet.setConfig(requestConfig);
全代码
@Test
void httpClientsTimeOut() throws Exception {
CloseableHttpClient closeableHttpClient = HttpClients.createDefault();
String username = "张三";
String pwd = "abcd+ |2312";
pwd = URLEncoder.encode(pwd, StandardCharsets.UTF_8.name());
String urlPath = "http://localhost:8080/login?userName=" + username + "&passWord=" + pwd;
HttpGet httpGet = new HttpGet(urlPath);
RequestConfig requestConfig = RequestConfig.custom()
.setConnectTimeout(5000)
.setSocketTimeout(5000)
.setConnectionRequestTimeout(5000)
.build();
httpGet.setConfig(requestConfig);
CloseableHttpResponse response = null;
try {
response = closeableHttpClient.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (HttpStatus.SC_OK == statusCode) {
System.out.println(statusCode + "-请求成功");
HttpEntity entity = response.getEntity();
String toStringResult = EntityUtils.toString(entity, StandardCharsets.UTF_8);
System.out.println(toStringResult);
EntityUtils.consume(entity);
} else {
System.out.println(statusCode + "-请求异常");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (closeableHttpClient != null) {
closeableHttpClient.close();
}
if (response != null) {
response.close();
}
}
}
post请求
发送application/x-www-form-urlencoded类型的post请求
主要代码
HttpPost httpPost=new HttpPost(urlPath);
httpPost.setHeader("Content-Type","application/x-www-form-urlencoded;chartset=utf-8");
List<NameValuePair> list=new ArrayList<>();
list.add(new BasicNameValuePair("userName","张三"));
list.add(new BasicNameValuePair("passWord","13579"));
UrlEncodedFormEntity formEntity=new UrlEncodedFormEntity(list,Consts.UTF_8);
httpPost.setEntity(formEntity);
全代码
@Test
void httpClientsPost() throws Exception {
CloseableHttpClient closeableHttpClient = HttpClients.createDefault();
String urlPath = "http://localhost:8080/testPost";
HttpPost httpPost=new HttpPost(urlPath);
httpPost.setHeader("Content-Type","application/x-www-form-urlencoded;chartset=utf-8");
List<NameValuePair> list=new ArrayList<>();
list.add(new BasicNameValuePair("userName","张三"));
list.add(new BasicNameValuePair("passWord","13579"));
UrlEncodedFormEntity formEntity=new UrlEncodedFormEntity(list,Consts.UTF_8);
httpPost.setEntity(formEntity);
CloseableHttpResponse response = null;
try {
response = closeableHttpClient.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (HttpStatus.SC_OK == statusCode) {
System.out.println(statusCode + "-请求成功");
HttpEntity entity = response.getEntity();
String toStringResult = EntityUtils.toString(entity, StandardCharsets.UTF_8);
System.out.println(toStringResult);
EntityUtils.consume(entity);
} else {
System.out.println(statusCode + "-请求异常");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (closeableHttpClient != null) {
closeableHttpClient.close();
}
if (response != null) {
response.close();
}
}
}
发送application/json类型的post请求
主要代码
HttpPost httpPost=new HttpPost(urlPath);
JSONObject jsonObject=new JSONObject();
jsonObject.put("userName","张三");
jsonObject.put("passWord","1231313");
StringEntity jsonEntity=new StringEntity(jsonObject.toString(),Consts.UTF_8);
jsonEntity.setContentType(new BasicHeader("Content-Type","application/json;utf-8"));
jsonEntity.setContentEncoding(Consts.UTF_8.name());
httpPost.setEntity(jsonEntity);
全代码
@Test
void httpClientsPost1() throws Exception {
CloseableHttpClient closeableHttpClient = HttpClients.createDefault();
String urlPath = "http://localhost:8080/testJson";
HttpPost httpPost=new HttpPost(urlPath);
JSONObject jsonObject=new JSONObject();
jsonObject.put("userName","张三");
jsonObject.put("passWord","1231313");
StringEntity jsonEntity=new StringEntity(jsonObject.toString(),Consts.UTF_8);
jsonEntity.setContentType(new BasicHeader("Content-Type","application/json;utf-8"));
jsonEntity.setContentEncoding(Consts.UTF_8.name());
httpPost.setEntity(jsonEntity);
CloseableHttpResponse response = null;
try {
response = closeableHttpClient.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (HttpStatus.SC_OK == statusCode) {
System.out.println(statusCode + "-请求成功");
Header[] headers = response.getAllHeaders();
for (Header header:headers){
System.out.println("响应头:"+header.getName()+"的值:"+header.getValue());
}
HttpEntity entity = response.getEntity();
String toStringResult = EntityUtils.toString(entity, StandardCharsets.UTF_8);
System.out.println(toStringResult);
EntityUtils.consume(entity);
} else {
System.out.println(statusCode + "-请求异常");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (closeableHttpClient != null) {
closeableHttpClient.close();
}
if (response != null) {
response.close();
}
}
}
httpclient文件请求
主要代码
MultipartEntityBuilder multipartEntityBuilder=MultipartEntityBuilder.create();
multipartEntityBuilder.setCharset(Consts.UTF_8);
multipartEntityBuilder.setContentType(ContentType.create("multipart/form-data", Consts.UTF_8));
multipartEntityBuilder.setMode(HttpMultipartMode.BROWSER_COMPATIBLE);
FileBody fileBody=new FileBody(new File("D:\\day42.log"));
StringBody stringBody=new StringBody("张三",ContentType.create("text/plan", Consts.UTF_8));
HttpEntity httpEntity = multipartEntityBuilder.addPart("fileName", fileBody)
.addBinaryBody("fileName", new File("D:\\11.jpg"))
.addPart("userName",stringBody)
.addTextBody("passWord", "154sadas").build();
httpPost.setEntity(httpEntity);
全部代码
@Test
void testMultipartFile() throws Exception {
CloseableHttpClient closeableHttpClient = HttpClients.createDefault();
String urlPath = "http://localhost:8080/testMultipartFile";
HttpPost httpPost=new HttpPost(urlPath);
MultipartEntityBuilder multipartEntityBuilder=MultipartEntityBuilder.create();
multipartEntityBuilder.setCharset(Consts.UTF_8);
multipartEntityBuilder.setContentType(ContentType.create("multipart/form-data", Consts.UTF_8));
multipartEntityBuilder.setMode(HttpMultipartMode.BROWSER_COMPATIBLE);
FileBody fileBody=new FileBody(new File("D:\\day42.log"));
StringBody stringBody=new StringBody("张三",ContentType.create("text/plan", Consts.UTF_8));
HttpEntity httpEntity = multipartEntityBuilder.addPart("fileName", fileBody)
.addBinaryBody("fileName", new File("D:\\11.jpg"))
.addPart("userName",stringBody)
.addTextBody("passWord", "154sadas").build();
httpPost.setEntity(httpEntity);
CloseableHttpResponse response = null;
try {
response = closeableHttpClient.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (HttpStatus.SC_OK == statusCode) {
System.out.println(statusCode + "-请求成功");
Header[] headers = response.getAllHeaders();
for (Header header:headers){
System.out.println("响应头:"+header.getName()+"的值:"+header.getValue());
}
HttpEntity entity = response.getEntity();
String toStringResult = EntityUtils.toString(entity, StandardCharsets.UTF_8);
System.out.println(toStringResult);
EntityUtils.consume(entity);
} else {
System.out.println(statusCode + "-请求异常");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (closeableHttpClient != null) {
closeableHttpClient.close();
}
if (response != null) {
response.close();
}
}
}
|