websocket通过redis实现集群方案
一、前言
1、使用websocket前后端通信时,若后台是集群部署,那么连接只能与其中一台握手建立连接,当nginx做负载后触发节点在未与websocket建立连接的服务上,此时就会出现问题。
二、解决方案
1、使用redis的发布订阅方式。(mq同理) 2、当需要websocket需求发送消息时,使用redis的发布订阅功能,将消息推送到redis中,所有需求消息的服务都监听这个Topic,接收到消息后,判断是否与前端建立连接,如果建立连接,将消息通过websocket发送出去,前端接收。若没有连接直接跳过即可。
三、解决步骤
1、引入websocket和redis依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
2、建一个websocket的配置
@Configuration
public class WebSocketConfig {
@Bean
public ServerEndpointExporter serverEndpointExporter() {
return new ServerEndpointExporter();
}
}
3、建websocket核心服务
@Slf4j
@Component
@ServerEndpoint("/socketServer/{userCode}")
public class WebSocketServer {
private static int onlineCount = 0;
private static ConcurrentHashMap<String, WebSocketServer> webSocketMap = new ConcurrentHashMap<>();
private Session session;
private String userCode = "";
@OnOpen
public void onOpen(Session session, @PathParam("userCode") String userCode) {
this.session = session;
this.userCode = userCode;
if (webSocketMap.containsKey(userCode)) {
webSocketMap.remove(userCode);
webSocketMap.put(userCode, this);
} else {
webSocketMap.put(userCode, this);
addOnlineCount();
}
log.info("用户连接:" + userCode + ",当前在线人数为:" + getOnlineCount());
try {
sendMessage("连接成功");
} catch (IOException e) {
log.error("用户:" + userCode + ",网络异常");
}
}
@OnClose
public void onClose() {
if (webSocketMap.containsKey(userCode)) {
webSocketMap.remove(userCode);
subOnlineCount();
}
log.info("用户退出:" + userCode + ",当前在线人数为:" + getOnlineCount());
}
@OnMessage
public void onMessage(String message, Session session) {
log.info("用户消息:" + userCode + ",报文:" + message);
if (StringUtils.isNotBlank(message)) {
try {
JSONObject jsonObject = JSON.parseObject(message);
jsonObject.put("fromUserId", this.userCode);
String toUserCode = jsonObject.getString("toUserId");
if (StringUtils.isNotBlank(toUserCode) && webSocketMap.containsKey(toUserCode)) {
webSocketMap.get(toUserCode).sendMessage(jsonObject.toJSONString());
} else {
log.error("请求的toUserCode:" + toUserCode + "不在该服务器上");
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
@OnError
public void onError(Session session, Throwable error) {
log.error("用户错误:" + this.userCode + ",原因:" + error.getMessage());
error.printStackTrace();
}
public void sendMessage(String message) throws IOException {
this.session.getBasicRemote().sendText(message);
}
public static void sendInfo(String message, @PathParam("userId") String userId) throws IOException {
log.info("发送消息到:" + userId + ",报文:" + message);
if (StringUtils.isNotBlank(userId) && webSocketMap.containsKey(userId)) {
webSocketMap.get(userId).sendMessage(message);
} else {
log.error("用户" + userId + ",不在线!");
}
}
public static synchronized int getOnlineCount() {
return onlineCount;
}
public static synchronized void addOnlineCount() {
WebSocketServer.onlineCount++;
}
public static synchronized void subOnlineCount() {
WebSocketServer.onlineCount--;
}
}
4、前端代码
if (typeof (WebSocket) == "undefined") {
console.log("您的浏览器不支持WebSocket");
} else {
const _this = this;
console.log("您的浏览器支持WebSocket");
var socketUrl = "http://localhost:80/socketServer/" + this.userCode;
socketUrl = socketUrl.replace("https", "ws").replace("http", "ws");
console.log(socketUrl);
if (this.socket != null) {
this.socket.close();
this.socket = null;
}
this.socket = new WebSocket(socketUrl);
this.socket.onopen = function () {
console.log("websocket已打开");
};
this.socket.onmessage = function (msg) {
console.log(msg.data);
_this.getList();
};
this.socket.onclose = function () {
console.log("websocket已关闭");
};
this.socket.onerror = function () {
console.log("websocket发生了错误");
}
}
5、测试是否可以连接 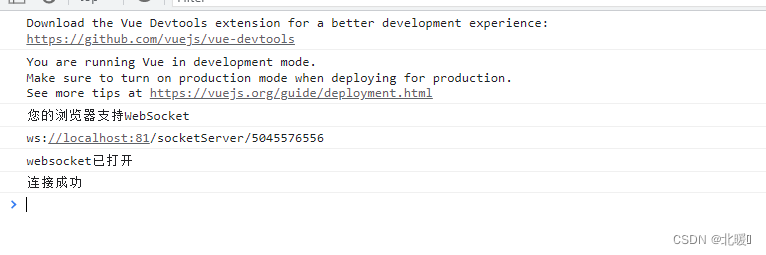 6、加入redis发布订阅 (1)redis发布消息 topic:topic名称 msg:发送的消息内容
@Component
public class RedisTopicSendTemplate {
@Autowired
private RedisTemplate<String, Object> redisTemplate;
public void sendMsg(String topic, String msg) {
redisTemplate.convertAndSend(topic, msg);
}
}
(2)监听消息
@Component
@Slf4j
public class SysUserListener implements MessageListener {
@SneakyThrows
@Override
public void onMessage(Message message, byte[] pattern) {
log.info("监听到redis消息:{}", message);
byte[] body = message.getBody();
if (body.length > 0){
String userCode = new String(message.getBody(), StandardCharsets.UTF_8).trim().replaceAll("\"", "");
WebSocketServer.sendInfo(userCode, userCode);
}
}
}
@Configuration
public class SubscriptionConfig {
@Bean
MessageListenerAdapter messageListener() {
return new MessageListenerAdapter(new SysUserListener());
}
@Bean
RedisMessageListenerContainer redisContainer(RedisConnectionFactory factory) {
RedisMessageListenerContainer container = new RedisMessageListenerContainer();
container.setConnectionFactory(factory);
container.addMessageListener(messageListener(), new ChannelTopic(RedisConstant.SYS_USER_TOPIC));
return container;
}
}
7、nginx配置 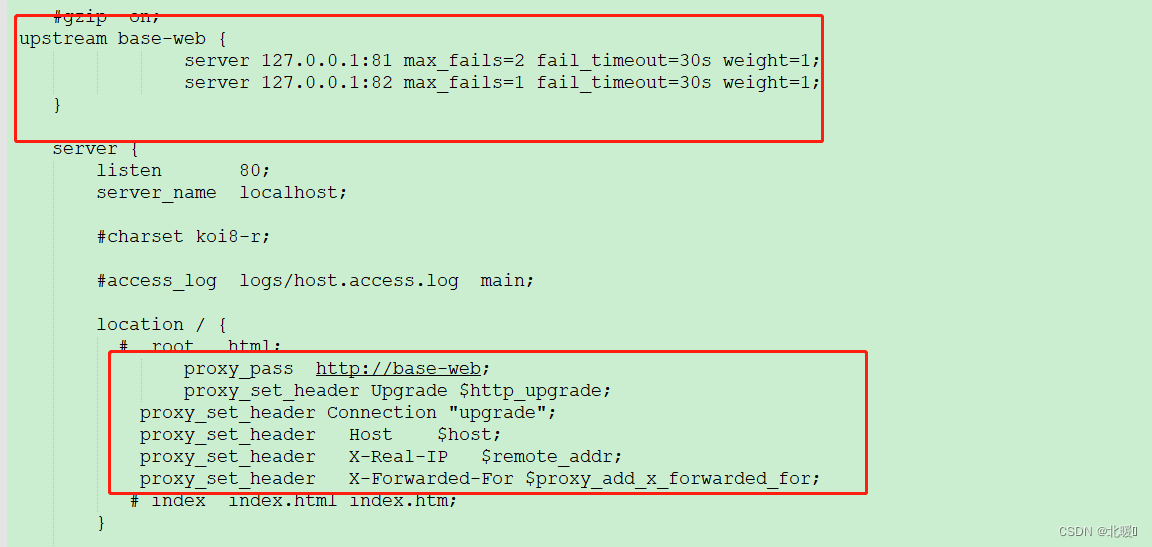
|