成果 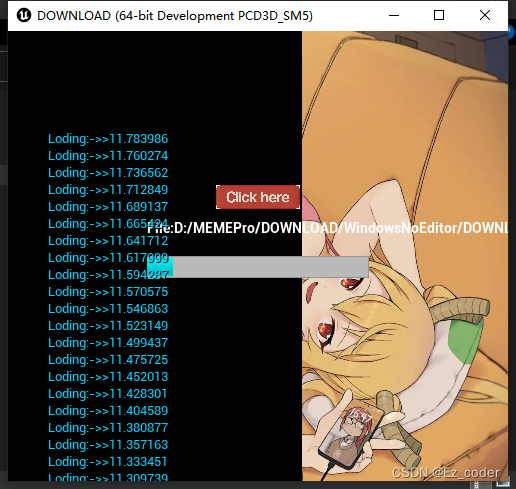 功能:点击一个按钮可以远程下载文件 并看得到进度条
先上代码 .h文件
#pragma once
#include "CoreMinimal.h"
#include "UObject/Object.h"
#include "Interfaces/IHttpRequest.h"
#include "RuntimeFilesDownloaderLibrary.generated.h"
UENUM(BlueprintType, Category = "Runtime Files Downloader")
enum DownloadResult
{
SuccessDownloading UMETA(DisplayName = "Success"),
DownloadFailed UMETA(DisplayName = "Download failed"),
SaveFailed UMETA(DisplayName = "Save failed"),
DirectoryCreationFailed UMETA(DisplayName = "Directory creation failed")
};
DECLARE_DYNAMIC_MULTICAST_DELEGATE_ThreeParams(FOnFilesDownloaderProgress, const int32, BytesSent, const int32, BytesReceived,
const int32, ContentLength);
DECLARE_DYNAMIC_MULTICAST_DELEGATE_OneParam(FOnFilesDownloaderResult, TEnumAsByte < DownloadResult >, Result);
UCLASS()
class DOWNLOAD_API URuntimeFilesDownloaderLibrary : public UObject
{
GENERATED_BODY()
public:
UPROPERTY(BlueprintAssignable, Category = "Runtime Files Downloader")
FOnFilesDownloaderProgress OnProgress;
UPROPERTY(BlueprintAssignable, Category = "Runtime Files Downloader")
FOnFilesDownloaderResult OnResult;
UPROPERTY(BlueprintReadOnly, Category = "Runtime Files Downloader")
FString FileURL;
UPROPERTY(BlueprintReadOnly, Category = "Runtime Files Downloader")
FString FileSavePath;
UFUNCTION(BlueprintCallable, Category = "Runtime Files Downloader")
static URuntimeFilesDownloaderLibrary* CreateDownloader();
UFUNCTION(BlueprintCallable, Category = "Runtime Files Downloader")
bool DownloadFile(const FString& URL, const FString& SavePath, float TimeOut = 5);
private:
void OnProgress_Internal(FHttpRequestPtr Request, int32 BytesSent, int32 BytesReceived);
void OnReady_Internal(FHttpRequestPtr Request, FHttpResponsePtr Response, bool bWasSuccessful);
};
.cpp文件
#include "RuntimeFilesDownloaderLibrary.h"
#include "HttpModule.h"
#include "Interfaces/IHttpRequest.h"
#include "Interfaces/IHttpResponse.h"
URuntimeFilesDownloaderLibrary* URuntimeFilesDownloaderLibrary::CreateDownloader()
{
URuntimeFilesDownloaderLibrary* Downloader = NewObject<URuntimeFilesDownloaderLibrary>();
Downloader->AddToRoot();
return Downloader;
}
bool URuntimeFilesDownloaderLibrary::DownloadFile(const FString& URL, const FString& SavePath, float TimeOut)
{
if (URL.IsEmpty() || SavePath.IsEmpty() || TimeOut <= 0)
{
return false;
}
FileURL = URL;
FileSavePath = SavePath;
TSharedPtr<IHttpRequest, ESPMode::ThreadSafe> HttpRequest = FHttpModule::Get().CreateRequest();
HttpRequest->SetVerb("GET");
HttpRequest->SetURL(FileURL);
HttpRequest->OnProcessRequestComplete().BindUObject(this, &URuntimeFilesDownloaderLibrary::OnReady_Internal);
HttpRequest->OnRequestProgress().BindUObject(this, &URuntimeFilesDownloaderLibrary::OnProgress_Internal);
HttpRequest->ProcessRequest();
return true;
}
void URuntimeFilesDownloaderLibrary::OnProgress_Internal(FHttpRequestPtr Request, int32 BytesSent, int32 BytesReceived)
{
const FHttpResponsePtr Response = Request->GetResponse();
if (Response.IsValid())
{
const int32 FullSize = Response->GetContentLength();
OnProgress.Broadcast(BytesSent, BytesReceived, FullSize);
}
}
void URuntimeFilesDownloaderLibrary::OnReady_Internal(FHttpRequestPtr Request, FHttpResponsePtr Response,
bool bWasSuccessful)
{
RemoveFromRoot();
Request->OnProcessRequestComplete().Unbind();
if (Response.IsValid() && EHttpResponseCodes::IsOk(Response->GetResponseCode()) && bWasSuccessful)
{
IPlatformFile& PlatformFile = FPlatformFileManager::Get().GetPlatformFile();
FString Path, Filename, Extension;
FPaths::Split(FileSavePath, Path, Filename, Extension);
if (!PlatformFile.DirectoryExists(*Path))
{
if (!PlatformFile.CreateDirectoryTree(*Path))
{
OnResult.Broadcast(DirectoryCreationFailed);
return;
}
}
IFileHandle* FileHandle = PlatformFile.OpenWrite(*FileSavePath);
if (FileHandle)
{
FileHandle->Write(Response->GetContent().GetData(), Response->GetContentLength());
delete FileHandle;
OnResult.Broadcast(SuccessDownloading);
}
else
{
OnResult.Broadcast(SaveFailed);
}
}
else
{
OnResult.Broadcast(DownloadFailed);
}
}
代码参考的知乎大佬 注意UE4.27 http里
TSharedRef<IHttpRequest> HttpRequest = FHttpModule::Get().CreateRequest();注意这段代码已经不适用了 根据UE官方源码4.27已经修改成
TSharedPtr<IHttpRequest, ESPMode::ThreadSafe> Request = FHttpModule::Get().CreateRequest();
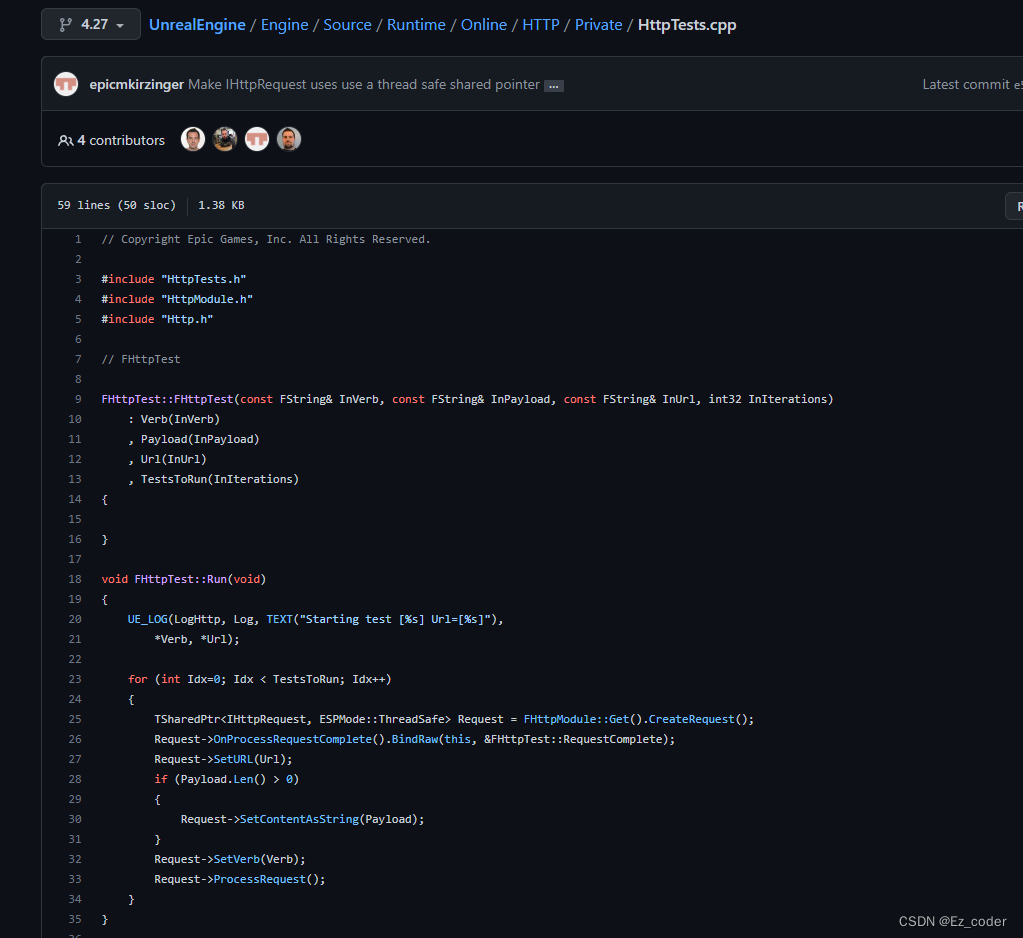
|