TCP服务器
TCP服务器实现对TCP客户端的监听,实现对数据的发送与接收。 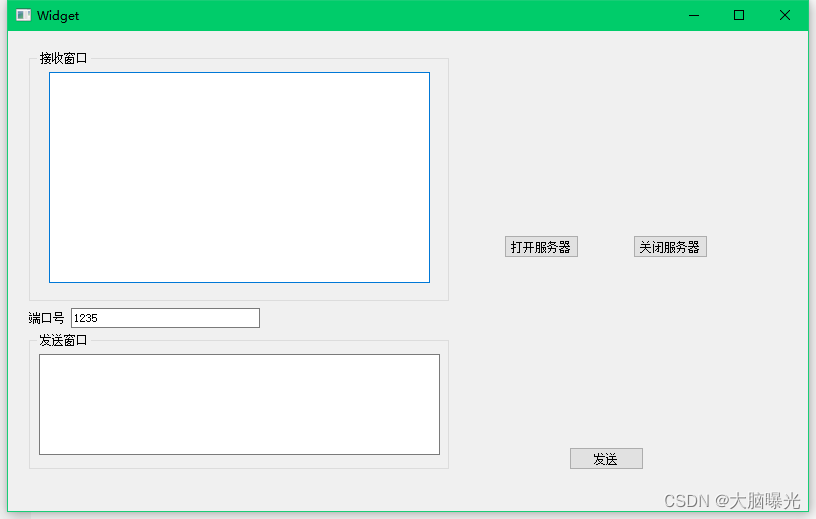 该TCP服务器入门级别,后面可以继续丰富。
界面
上图就是实现的界面,后面可以自己去丰富一下,上面的按钮,接收数据框,发送数据框,端口号输入框,文字显示等使用的控件为:
-
文字显示(除去按钮,按钮可以直接写) 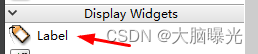 -
端口号输入框 和后面发送框一样的控件 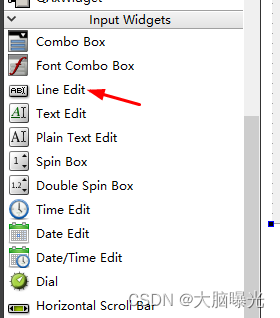 -
按钮 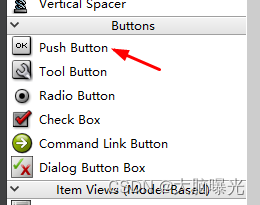 -
发送框 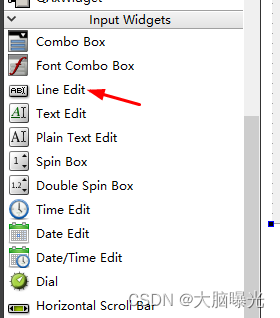 -
接收框
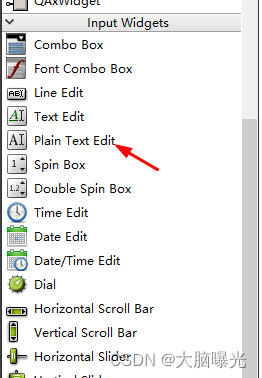 注意:此控件设置为只读模式,因为不需要我们输入。
逻辑代码
.pro文件
QT += core gui network
greaterThan(QT_MAJOR_VERSION, 4): QT += widgets
CONFIG += c++11
# The following define makes your compiler emit warnings if you use
# any Qt feature that has been marked deprecated (the exact warnings
# depend on your compiler). Please consult the documentation of the
# deprecated API in order to know how to port your code away from it.
DEFINES += QT_DEPRECATED_WARNINGS
# You can also make your code fail to compile if it uses deprecated APIs.
# In order to do so, uncomment the following line.
# You can also select to disable deprecated APIs only up to a certain version of Qt.
#DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0
SOURCES += \
main.cpp \
widget.cpp
HEADERS += \
widget.h
FORMS += \
widget.ui
# Default rules for deployment.
qnx: target.path = /tmp/$${TARGET}/bin
else: unix:!android: target.path = /opt/$${TARGET}/bin
!isEmpty(target.path): INSTALLS += target
.h文件
#ifndef WIDGET_H
#define WIDGET_H
#include <QWidget>
#include <QTcpServer>
#include <QTcpSocket>
#include <QHostAddress>
#include <QtNetwork>
QT_BEGIN_NAMESPACE
namespace Ui { class Widget; }
QT_END_NAMESPACE
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = nullptr);
~Widget();
QTcpServer *tcpServer;
QTcpSocket *tcpSocket;
private slots:
void newConnection_Slot();
void readyRead_Slot();
void disconnected_Slot();
void on_openbt_clicked();
void on_closeBt_clicked();
void on_sendBT_clicked();
private:
Ui::Widget *ui;
};
#endif
此处添加头文件,声明槽函数。
.cpp文件
#include "widget.h"
#include "ui_widget.h"
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
tcpServer = new QTcpServer(this);
tcpSocket = new QTcpSocket(this);
}
void Widget::newConnection_Slot()
{
static int i = 0;
tcpSocket = tcpServer->nextPendingConnection();
connect(tcpSocket, SIGNAL(readyRead()), this, SLOT(readyRead_Slot()));
connect(tcpSocket, SIGNAL(disconnected()), this, SLOT(disconnected_Slot()));
i++;
}
void Widget::readyRead_Slot()
{
QString buf;
buf = tcpSocket->readAll();
ui->receiveEdit->appendPlainText(buf);
}
void Widget::disconnected_Slot()
{
}
Widget::~Widget()
{
delete ui;
}
void Widget::on_openbt_clicked()
{
tcpServer->listen(QHostAddress::Any, ui->duankouEdit_2->text().toUInt());
connect(tcpServer, SIGNAL(newConnection()), this, SLOT(newConnection_Slot()));
QString localhostName = QHostInfo::localHostName();
QHostInfo info = QHostInfo::fromName(localhostName);
ui->receiveEdit->appendPlainText(localhostName);
ui->receiveEdit->appendPlainText(info.addresses().back().toString());
foreach(QHostAddress address,info.addresses())
{
if(address.protocol() == QAbstractSocket::IPv4Protocol)
ui->receiveEdit->appendPlainText(address.toString());
}
quint16 port = tcpServer->serverPort();
QString st = QString::number(port);
ui->receiveEdit->appendPlainText(st);
}
void Widget::on_closeBt_clicked()
{
tcpServer->close();
tcpSocket->close();
disconnect(tcpServer, SIGNAL(newConnection()), this, SLOT(newConnection_Slot()));
}
void Widget::on_sendBT_clicked()
{
tcpSocket->write(ui->sendEdit->text().toLocal8Bit().data());
}
打开服务器获取主机IP地址的两种方法:
QHostInfo info = QHostInfo::fromName(localhostName);
ui->receiveEdit->appendPlainText(localhostName);
ui->receiveEdit->appendPlainText(info.addresses().back().toString());
foreach(QHostAddress address,info.addresses())
{
if(address.protocol() == QAbstractSocket::IPv4Protocol)
ui->receiveEdit->appendPlainText(address.toString());
}
项目展示
tcp服务器: 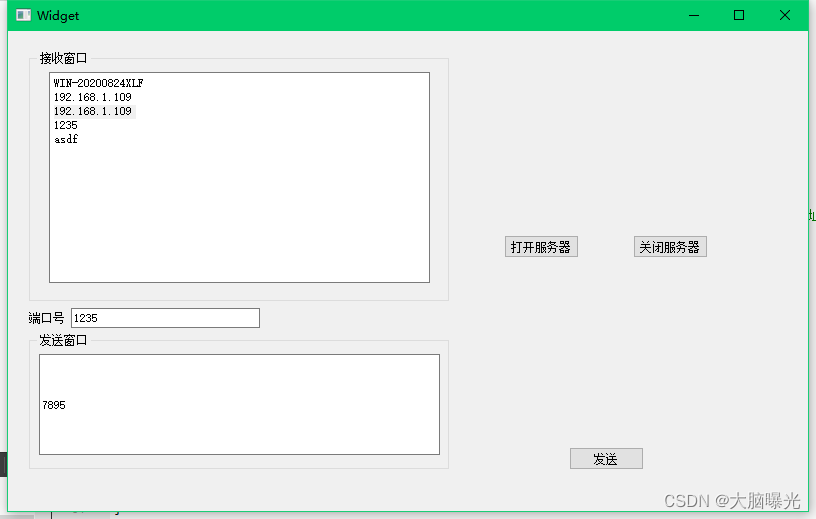 tcp客户端: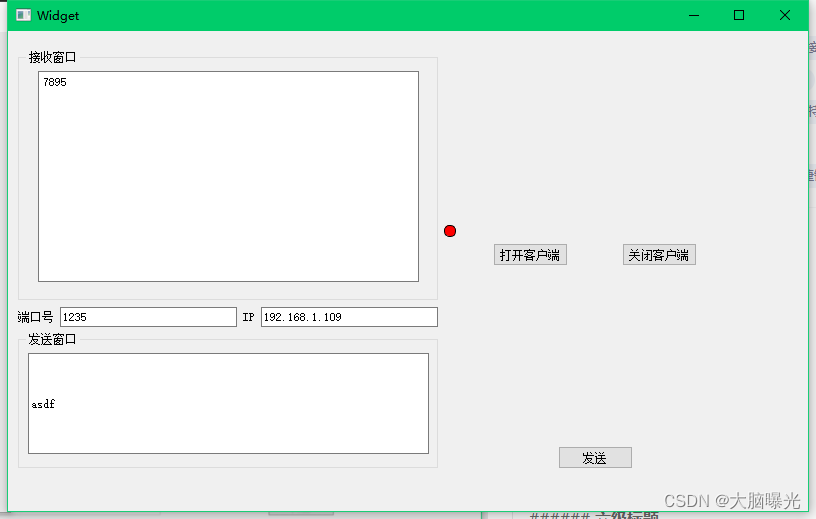 打开服务器的时候,服务器端会自动输出当前服务器的IP地址和端口号。 然后将IP地址和端口号输入到客户端里面,打开客户端连接就可以了。 实现了服务器与客户端的通信。
源码资源下载
https://download.csdn.net/download/qq_30255657/85675636
|