在过去,Android 上发送 HTTP 请求一般有两种方式:HttpURLConnection 和 HttpClient,不过在 Android 6.0 系统中,HttpClient 被完全移除了,因此推荐使用 HttpURLConnection。
HttpURLConnection
下面的测试代码主要实现对百度首页数据的获取。
- 首先添加网络权限
<uses-permission android:name="android.permission.INTERNET" />
- 布局文件
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_height="match_parent"
android:layout_width="match_parent"
android:orientation="vertical">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="send"
android:layout_gravity="center"/>
<ScrollView
android:id="@+id/scrollView"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/text"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</ScrollView>
</LinearLayout>
- java文件
对部分源码的分析: 按钮点击调用sendHttpURL()方法,我们在这个方法里实现对数据的获取打印。 具体步骤:
- 我们获取需要获取到HttpURLConnection实例,new一个URL对象,放入网址,调用openConnection()方法。
java Connection=new URL("http://www.baidu.com").openConnection(); - Http请求的方法有post和get两种方法,post提交数据,get获取数据。
Connection.setRequestMethod("GET"); - 设置连接超时、读取超时的毫秒数
Connection.setConnectTimeout(8000);Connection.setReadTimeout(8000); - 获取输入流

public class MainActivity extends AppCompatActivity {
private TextView responseText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if (getSupportActionBar() != null)
getSupportActionBar().hide();
Button button01=findViewById(R.id.button);
responseText=findViewById(R.id.text);
button01.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
sendHttpURL();
}
});
}
private void sendHttpURL()
{
new Thread(new Runnable() {
@Override
public void run() {
HttpURLConnection Connection=null;
BufferedReader reader=null;
try {
URL url=new URL("https://www.baidu.com/");
Connection=(HttpURLConnection)url.openConnection();
Connection.setRequestMethod("GET");
Connection.setConnectTimeout(8000);
Connection.setReadTimeout(8000);
InputStream inputStream=Connection.getInputStream();
InputStreamReader inputStreamReader=new InputStreamReader(inputStream);
reader= new BufferedReader(inputStreamReader);
StringBuffer response=new StringBuffer();
String line;
while ((line=reader.readLine())!=null)
{
response.append(line);
}
showResponse(response.toString());
}catch (Exception e)
{
e.printStackTrace();
}finally {
if(reader!=null)
{
try {
reader.close();
}catch (IOException e) {
e.printStackTrace();
}
}
if (Connection!=null)
Connection.disconnect();
}
}
}).start();
}
private void showResponse(final String response){
runOnUiThread(new Runnable() {
@Override
public void run() {
responseText.setText(response);
}
});
}
}
OkHttp
和上面的HttpURLConnect共用一个布局文件。 在使用 OkHttp 之前,我们需要先在项目中添加 OkHttp 库的依赖,编辑 app/build.gradle 文件,在 dependencies 闭包中添加如下内容:
/* okhttp3.0依赖库 */
implementation 'com.squareup.okhttp3:okhttp:3.12.13'
implementation 'com.squareup.okio:okio:1.15.0'
OkHttp的具体用法:
private void sendOkHttpURL() {
new Thread(new Runnable() {
@Override
public void run() {
Request request;
Response response = null;
try {
OkHttpClient client = new OkHttpClient();
request = new Request.Builder().url("https://www.baidu.com").build();
response = client.newCall(request).execute();
String responseData = response.body().string();
showResponse(responseData);
} catch (Exception e) {
e.printStackTrace();
} finally {
if (response != null) {
try {
response.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
}).start();
}
下面是向服务器发送数据,示例代码中没有使用。
val requestBody = FormBody.Builder()
.add("username", "admin")
.add("password", "123")
.build()
val request = Request.Builder()
.url("https://www.baidu.com")
.post(requestBody)
.build()
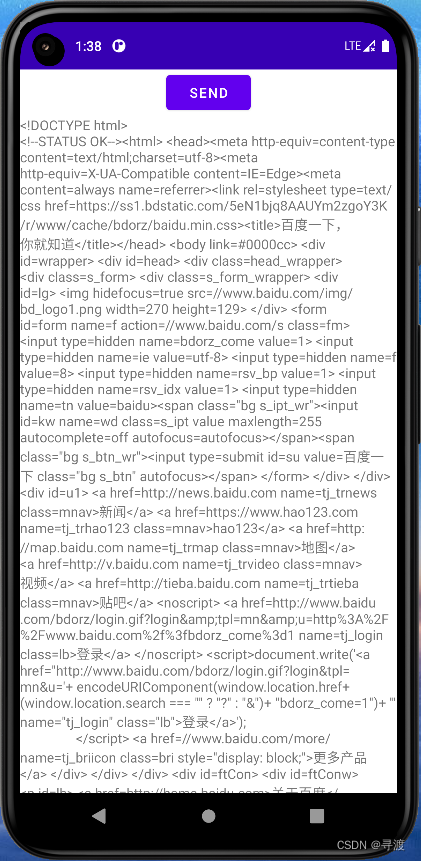
|