目录
需求
请求端
1,添加依赖
2,请求对象
3,请求工具类
4,请求测试(事先开启接收端的服务)
接收端
数据请求模拟
需求
本项目需要通过向对端第三方项目发送一个http的post类型的请求,并且指定了一些请求字段,数据传输采用了json,对请求头没有其他特殊要求,所以这里写了一个demo作为参考
请求端
1,添加依赖
这里我在对json进行发送和解析的时候,我采用了fastjson工具。
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
2,请求对象
这个对象主要的作用就是将需要发送的参数作为对象的属性然后可以将对象直接转化为json,这种请求在请求参数的类型不一致的时候可以使用(Long,String,DateTIme等等)
public class RequestChange {
private Long accNum;
private String servPwdResetChnl;
private String servPwdResetTime;
private String system;
//set和get方法 省略.....
}
3,请求工具类
这里多注意代码中这个语句,之前一直没有添加 "UTF-8"这个参数,导致请求的中文参数全是???。如图所示
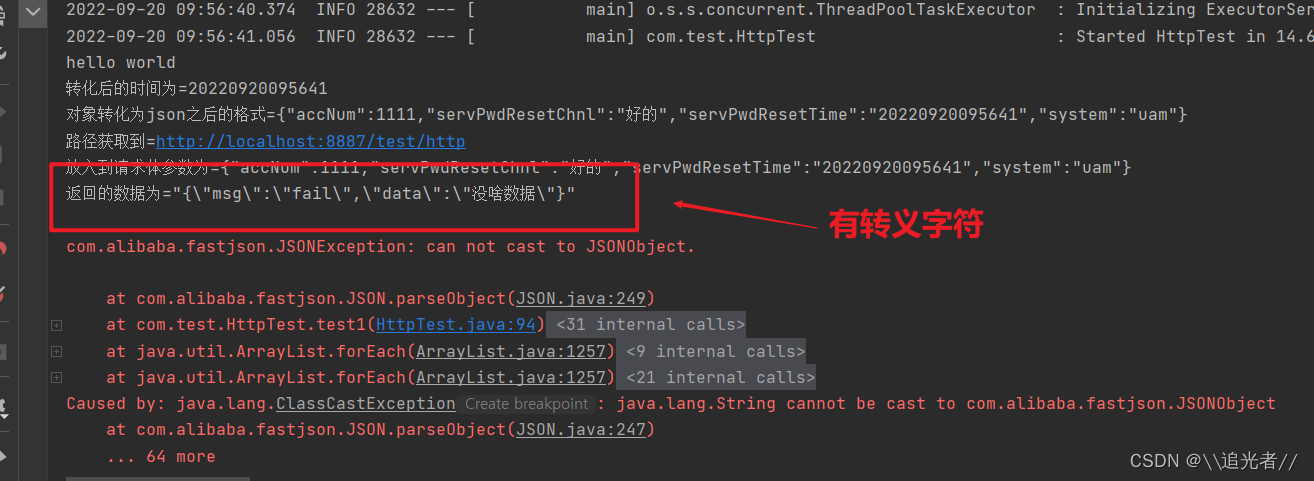
// 设置请求参数
StringEntity se = new StringEntity(jsonstr,"UTF-8");
import org.apache.http.*;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import org.springframework.stereotype.Component;
import java.io.IOException;
import java.net.URLEncoder;
@Component
public class HttpUtil {
/**
* httpClient get请求
*
* @param url 请求url
* @param jsonstr 请求实体 json/xml提交适用
* @return 可能为空 需要处理
* @throws Exception
*
*/
public static String sendHttpGet(String url, String jsonstr)
throws Exception {
String result = "";
CloseableHttpClient httpClient = null;
try {
httpClient = HttpClients.createDefault();
if(null != jsonstr && !"".equals(jsonstr)) {
jsonstr = URLEncoder.encode(jsonstr, "UTF-8");
url = url + "?req=" + jsonstr;
}
System.out.println("url------------" + url);
HttpGet httpGet = new HttpGet(url);
// 设置头信息
httpGet.addHeader("Content-Type", "application/json;charset=utf-8");
httpGet.addHeader("Accept", "application/json;charset=utf-8");
HttpResponse httpResponse = httpClient.execute(httpGet);
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode == HttpStatus.SC_OK) {
HttpEntity resEntity = httpResponse.getEntity();
result = EntityUtils.toString(resEntity);
} else {
readHttpResponse(httpResponse);
}
} catch (Exception e) {
throw e;
} finally {
if (httpClient != null) {
httpClient.close();
}
}
return result;
}
/**
* httpClient post请求
*
* @param url 请求url
* @param jsonstr 请求实体 json/xml提交适用
* @return 可能为空 需要处理
* @throws Exception
*
*/
public static String sendHttpPost(String url, String jsonstr)
throws Exception {
String result = "";
CloseableHttpClient httpClient = null;
try {
httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
// 设置头信息
httpPost.addHeader("Content-Type", "application/json;charset=utf-8");
httpPost.addHeader("Accept", "application/json;charset=utf-8");
System.out.println("放入到请求体参数为="+jsonstr);
// 设置请求参数
StringEntity se = new StringEntity(jsonstr,"UTF-8");
httpPost.setEntity(se);
HttpResponse httpResponse = httpClient.execute(httpPost);
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode == HttpStatus.SC_OK) {
HttpEntity resEntity = httpResponse.getEntity();
result = EntityUtils.toString(resEntity);
} else {
readHttpResponse(httpResponse);
}
} catch (Exception e) {
throw e;
} finally {
if (httpClient != null) {
httpClient.close();
}
}
return result;
}
public static String readHttpResponse(HttpResponse httpResponse)
throws ParseException, IOException {
StringBuilder builder = new StringBuilder();
// 获取响应消息实体
HttpEntity entity = httpResponse.getEntity();
// 响应状态
builder.append("status:" + httpResponse.getStatusLine());
builder.append("headers:");
HeaderIterator iterator = httpResponse.headerIterator();
while (iterator.hasNext()) {
builder.append("\t" + iterator.next());
}
// 判断响应实体是否为空
if (entity != null) {
String responseString = EntityUtils.toString(entity);
builder.append("response length:" + responseString.length());
builder.append("response content:"
+ responseString.replace("\r\n", ""));
}
return builder.toString();
}
public static void main(String[] args) throws Exception {
System.out.println(sendHttpGet("http://127.0.0.1:8080//AccountSynchro", "{\"login\" : \"\", \"alias\" : \"153057123231021\", \"passwd\" : \"122323456\"}"));
}
}
4,请求测试(事先开启接收端的服务)
主要就是将请求对象进行赋值,然后通过fastJson将对象转化为将json数据然后进行请求,
这里需要注意的是接受请求的时候 ,这里我进行了一个格式话的接受json数据的解析,如果不能
//解析返回的json字符串 返回的数据为="{\"msg\":\"fail\",\"data\":\"没啥数据\"}" 带有转义字符需要进行重新编译
Object parse = JSON.parse(resultJson);
String s2 = parse.toString();
RespChange respChange = JSON.parseObject(s2, RespChange.class);
System.out.println(respChange.getMsg());
全部请求和解析json代码
@SpringBootTest(classes = UagApplication.class)
public class HttpTest {
//获取到传送工具类
// @Autowired
// private HttpUtil httpUtil;
//获取到请求路径
@Value("${Changes.remind.path}")
private String remindPath="";
@Autowired
private ProvincialEvents provincialEvents;
@Autowired
private ITransactionIdManager transIdManager = null;
@Test
public void test1() throws Exception {
System.out.println("hello world");
//测试发送 json数据 多个参数不同类型
long accNum=1111;
String system="uam";
//将参数字段转化为字符串 创建一个对象
RequestChange requestChange = new RequestChange();
requestChange.setAccNum(accNum);
//requestChange.setServPwdResetChnl(servPwdResetChnl);
requestChange.setServPwdResetChnl("好的");
//创建当前时间
Date date = Calendar.getInstance().getTime();
DateFormat dateFormat = new SimpleDateFormat("YYYYMMddHHmmss");
String format = dateFormat.format(date);
requestChange.setServPwdResetTime(format);
requestChange.setSystem(system);
System.out.println("转化后的时间为="+requestChange.getServPwdResetTime());
//将对象转化为json
String s = JSON.toJSONString(requestChange);
System.out.println("对象转化为json之后的格式="+s);
if (StringUtil.isEmptyString(remindPath)){
System.out.println("路径没有获取到"+remindPath);
}
System.out.println("路径获取到="+remindPath);
String resultJson = HttpUtil.sendHttpPost(remindPath, s);
System.out.println("返回的数据为="+resultJson);
//解析返回的json字符串 返回的数据为="{\"msg\":\"fail\",\"data\":\"没啥数据\"}" 带有转义字符需要进行重新编译
Object parse = JSON.parse(resultJson);
String s2 = parse.toString();
RespChange respChange = JSON.parseObject(s2, RespChange.class);
System.out.println(respChange.getMsg());
}
}
接收端
接收请求发过来的json数据,并且可以解析成自己需要格式(String,Map,对象)
@RestController
@RequestMapping(value ="/test")
public class TestHttpController {
@RequestMapping(value = "/http",method=RequestMethod.POST)
public String testHttp(@RequestBody JSONObject json){
//解析json数据,获取到每一个属性对应的值
System.out.println(json.getString("accNum"));
System.out.println(json.getString("servPwdResetChnl"));
System.out.println(json.getString("servPwdResetTime"));
System.out.println(json.getString("system"));
//返回响应json字符串
HashMap<String, String> map = new HashMap<>();
map.put("msg","success");
map.put("data","没啥数据");
String s = JSON.toJSONString(map);
return s;
}
}
数据请求模拟
1,开始接收端服务
2,开启请求端服务发送请求
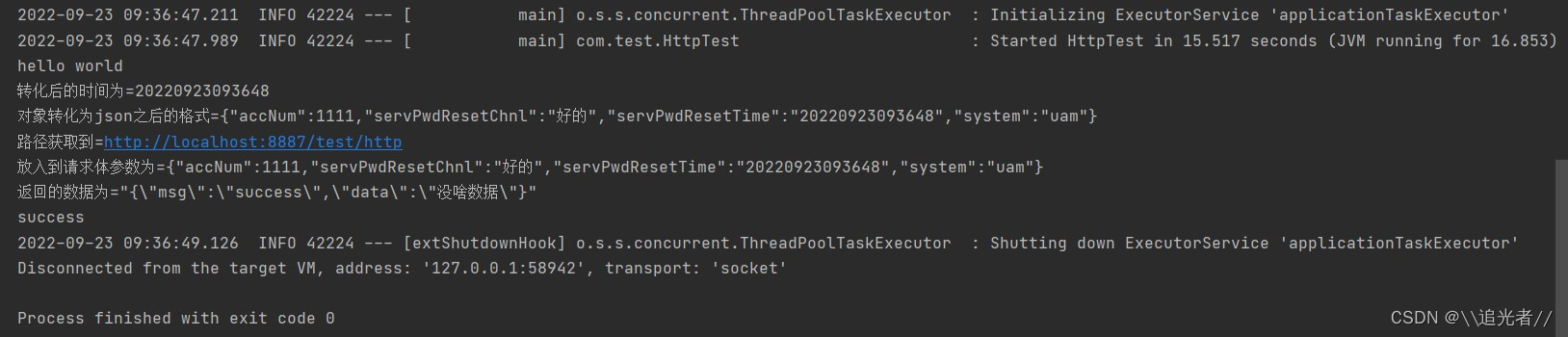
?
?
参考文章:
json如何传输数据?
(31条消息) Java中使用JSON数据传递_杀神lwz的博客-CSDN博客_java传递json格式数据
接受方如何解析
(31条消息) Java接收json参数_詹Sir(开源字节)的博客-CSDN博客_java接收json对象
|