简介
使用WebSocket实现的一个简单的聊天功能业务
使用了SpringBoot的ApplicationEvent事件监听用来与业务解耦
需要注意的是websocket的长连接默认会在1分钟后自动关闭,状态码为:1001,正常关闭状态码为:1000
因此客户端要定义一个定时器反复向服务端发送心跳,保持处于活跃状态(本文并未去实现)
一、Maven的引入
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
<version>2.4.0</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
</dependencies>
二、后端代码结构图
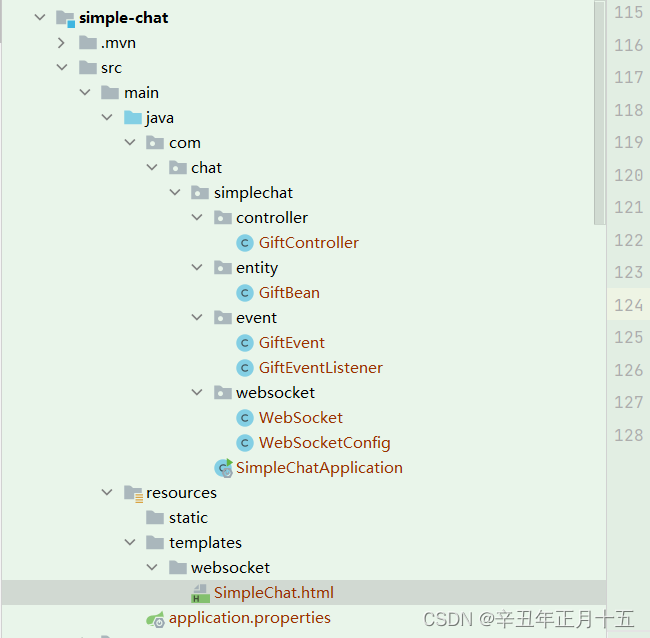
二(1)ApplicationEvent及监听
package com.chat.simplechat.event;
import com.chat.simplechat.entity.GiftBean;
import lombok.Getter;
import org.springframework.context.ApplicationEvent;
@Getter
public class GiftEvent extends ApplicationEvent {
private GiftBean giftBean;
public GiftEvent(Object source,GiftBean giftBean) {
super(source);
this.giftBean = giftBean;
}
}
package com.chat.simplechat.event;
import com.alibaba.fastjson.JSONObject;
import com.chat.simplechat.entity.GiftBean;
import com.chat.simplechat.websocket.WebSocket;
import lombok.extern.slf4j.Slf4j;
import org.springframework.context.event.EventListener;
import org.springframework.stereotype.Component;
import javax.annotation.Resource;
import java.util.Random;
@Slf4j
@Component
public class GiftEventListener {
@Resource
private WebSocket webSocket;
@EventListener
public void givingGifts(GiftEvent giftEvent){
GiftBean giftBean = giftEvent.getGiftBean();
Random random = new Random();
String[] str = new String[]{"烟花","跑车","皇冠","凤冠","穿云箭"};
int i = random.nextInt(str.length);
JSONObject jsonObject = new JSONObject();
jsonObject.put("fromUserId",giftBean.getFromUserId());
jsonObject.put("toUserId",giftBean.getToUserId());
jsonObject.put("contentText",str[i]);
webSocket.sendOneMessage(giftBean.getToUserId(),jsonObject.toJSONString());
}
}
二(2)WebSocket及配置
package com.chat.simplechat.websocket;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import lombok.extern.slf4j.Slf4j;
import org.springframework.stereotype.Component;
import org.springframework.util.StringUtils;
import javax.websocket.*;
import javax.websocket.server.PathParam;
import javax.websocket.server.ServerEndpoint;
import java.util.Iterator;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.CopyOnWriteArraySet;
@Slf4j
@Component
@ServerEndpoint("/websocket/{userId}")
public class WebSocket {
private Session session;
private String userId;
private static int onlineCount = 0;
private static CopyOnWriteArraySet<WebSocket> webSockets = new CopyOnWriteArraySet<>();
private static ConcurrentHashMap<String,Session> sessionPool = new ConcurrentHashMap<>();
@OnOpen
public void onOpen(Session session, @PathParam("userId") String userId){
this.session = session;
this.userId = userId;
boolean exists = false;
Iterator<WebSocket> iterator = webSockets.iterator();
log.info("iterator:{}",iterator.hasNext());
while (iterator.hasNext()){
WebSocket webSocket = iterator.next();
if(webSocket.userId.equals(userId)){
exists = true;
break;
}
}
if(exists){
this.remove();
}
webSockets.add(this);
sessionPool.put(userId,session);
log.info("【WebSocket】用户["+this.userId+"]已上线,当前在线用户数量:"+webSockets.size());
this.addOnlineCount();
}
@OnClose
public void onClose(){
try {
this.remove();
log.info("【WebSocket】用户["+this.userId+"]已下线,当前在线用户数量:"+webSockets.size());
} catch (Exception e) {
throw new RuntimeException(e);
}
}
@OnMessage
public void onMessage(String message){
log.info("【WebSocket】收到客户端消息:"+message);
if(StringUtils.hasText(message)){
JSONObject jsonObject = JSON.parseObject(message);
jsonObject.put("fromUserId",this.userId);
String toUserId = jsonObject.getString("toUserId");
Session session = sessionPool.get(toUserId);
if(StringUtils.hasText(toUserId) && null != session){
session.getAsyncRemote().sendText(jsonObject.toJSONString());
}else{
log.error("请求的userid:{}不在该服务器上",toUserId);
}
}
}
@OnError
public void onError(Session session,Throwable throwable){
log.error("消息发送错误,原因:{}",throwable.getMessage());
}
public void sendOneMessage(String userId,String message){
try {
Session session = sessionPool.get(userId);
if(null != session && session.isOpen()){
session.getAsyncRemote().sendText(message);
log.info("消息发送成功!");
}
} catch (Exception e) {
throw new RuntimeException("消息发送失败:",e);
}
}
public void sendMoreMessage(String[] userIds,String message){
for (String userId : userIds) {
try {
Session session = sessionPool.get(userId);
if(null != session && session.isOpen()){
session.getAsyncRemote().sendText(message);
}
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
public void radioBroadcast(String message){
for (WebSocket webSocket : webSockets) {
try {
if(webSocket.session.isOpen()){
webSocket.session.getAsyncRemote().sendText(message);
}
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
public void sendMessage(String message){
this.session.getAsyncRemote().sendText(message);
}
private void remove() {
webSockets.remove(this);
sessionPool.remove(userId);
this.subOnlineCount();
}
public static synchronized int getOnlineCount(){
return onlineCount;
}
public static synchronized void addOnlineCount(){
WebSocket.onlineCount++;
}
public static synchronized void subOnlineCount(){
WebSocket.onlineCount--;
}
}
package com.chat.simplechat.websocket;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.socket.server.standard.ServerEndpointExporter;
@Configuration
public class WebSocketConfig {
@Bean
public ServerEndpointExporter serverEndpointExporter(){
return new ServerEndpointExporter();
}
}
二(3)控制器及实体类
package com.chat.simplechat.controller;
import com.chat.simplechat.entity.GiftBean;
import com.chat.simplechat.event.GiftEvent;
import org.springframework.context.ApplicationContext;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import javax.annotation.Resource;
@Controller
@RequestMapping("/gift")
public class GiftController {
@Resource
private ApplicationContext applicationContext;
@ResponseBody
@GetMapping("/randomGift")
public String randomGift(GiftBean giftBean){
applicationContext.publishEvent(new GiftEvent(this,giftBean));
return "成功";
}
@GetMapping("/page")
public String page(){
return "websocket/SimpleChat";
}
}
package com.chat.simplechat.entity;
import lombok.Data;
@Data
public class GiftBean {
private String fromUserId;
private String toUserId;
}
三、建立HTML
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="utf-8">
<title>简单聊天室</title>
</head>
<script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script>
<script>
$(function(){
$("#div").hide();
$(".on-line").hide();
})
var socket;
function openSocket() {
if(typeof(WebSocket) == "undefined") {
console.log("您的浏览器不支持WebSocket");
}else{
console.log("您的浏览器支持WebSocket");
var socketUrl="http://localhost:7523/websocket/"+$("#userId").val();
socketUrl=socketUrl.replace("https","ws").replace("http","ws");
console.log(socketUrl);
if(socket!=null){
socket.close();
socket=null;
}
socket = new WebSocket(socketUrl);
socket.onopen = function() {
console.log("websocket已打开");
$("#div").show();
$(".on-line").show();
$("#login").hide();
};
socket.onmessage = function(msg) {
console.log(msg);
if(msg.data == "连接成功"){
$("#sendMessage").css("color","green");
}else{
var user = JSON.parse(msg.data);
console.log(user);
$("#user").text("【"+user.fromUserId+"】的消息:");
$("#msg").empty();
$("#msg").text(user.contentText);
}
console.log(msg.data);
};
socket.onclose = function() {
console.log("websocket已关闭");
};
socket.onerror = function(e) {
console.log(e);
console.log("websocket发生了错误");
}
}
}
function sendMessage() {
var msg = $("#contentText").val();
$("#contentText").val("");
console.log(msg);
if(typeof(WebSocket) == "undefined") {
console.log("您的浏览器不支持WebSocket");
$("#msg").val("您的浏览器不支持WebSocket");
}else {
console.log("您的浏览器支持WebSocket");
console.log('{"toUserId":"'+$("#toUserId").val()+'","contentText":"'+msg+'"}');
socket.send('{"toUserId":"'+$("#toUserId").val()+'","contentText":"'+msg+'"}');
}
}
function givingGifts(){
$.ajax({
url:'/gift/randomGift',
post:'get',
data:{"fromUserId":$("#userId").val(),"toUserId":$("#toUserId").val()},
success:function(data){
console.log(data);
},error:function(e){
console.log(e);
}
})
}
</script>
<body style="margin: 0;width: 100%;margin: auto">
<div style="margin: 10% 50% 10% 25%;border: 1px solid #cefff4;padding:1% 2% 1% 2%;background-color: #cefff4">
<div>
<p><h1>无痕聊天室😳</h1></p>
<p style="padding-left: 70%" class="on-line">
<span style="color: purple">在线</span>|
<span style="color: crimson;cursor: pointer" onclick="givingGifts()">送礼物</span>
</p>
<p><span id="gift"></span></p>
</div>
<div>
<p>【😀】: 
<select id="userId">
<option value="S">S</option>
<option value="N">N</option>
</select></p>
<p>【😄】: 
<select id="toUserId">
<option value="N">N</option>
<option value="S">S</option>
</select>
</p>
<p><span id="user"></span><span id="msg"></span></p>
<p>
<input id="contentText" name="contentText">
</p>
<p><div id="div" style="margin-left: 70%"><button id="sendMessage" onclick="sendMessage()" style="cursor: pointer">发送消息</button></div>
<p style="padding-left: 70%"><button id="login" onclick="openSocket()" style="cursor: pointer">上线</button></p>
</div>
</div>
</body>
</html>
四、成功截图
测试的时候只需要在网页中打开两个链接选择不同角色即可
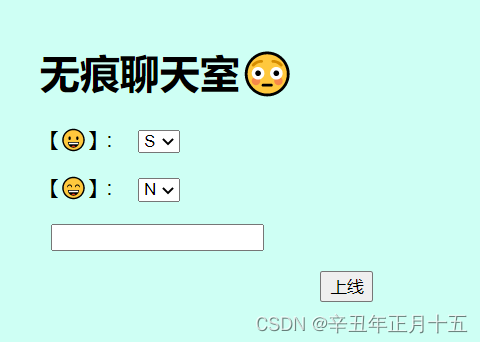
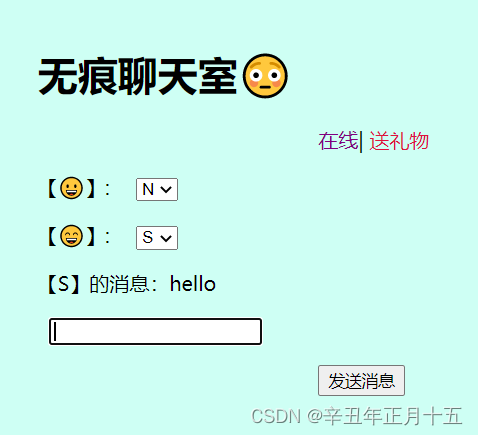 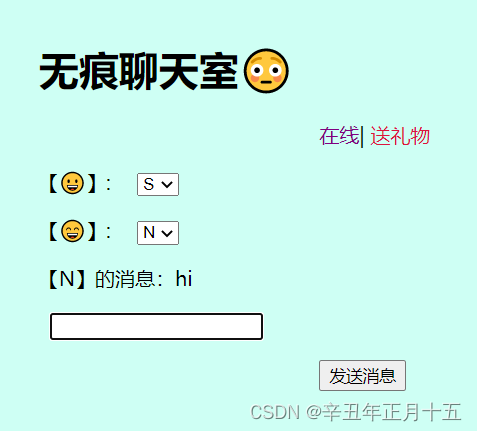 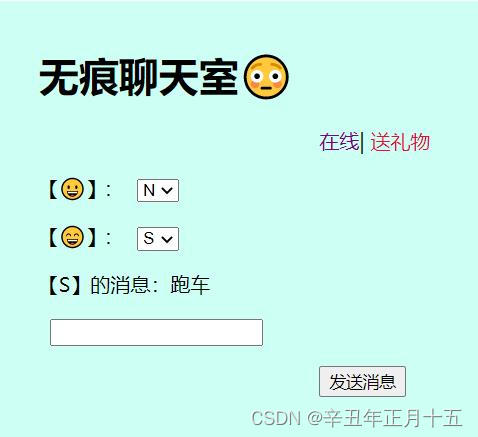
|