前言
WebSocket,它是一种全双工的协议,在http协议存在的基础上,它有具有什么独特点,能让其在许多地方被广泛使用呢? 我们可以从RFC标准协议中,看到原因 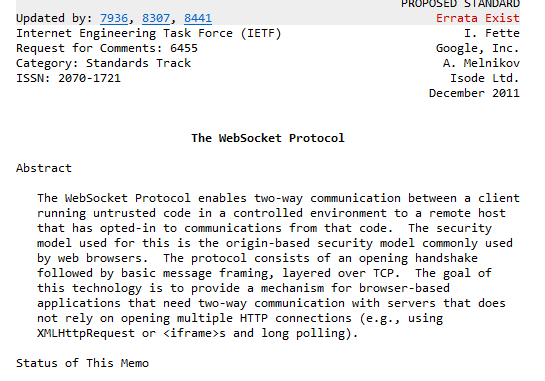 可以看到,http不断轮询,是一种经常被使用的方案,用来在用户不做任何操作的情况下,网页能收到消息并发生变更, 微信验证码就是这样一种方案,每次扫完码后的延迟,可能就包含了一次轮询的间歇周期 但大量轮询,必然会带来性能消耗,而且扫码这种操作,数据量也比较小, 在客户端与服务器都需要收发大量信息的时候,webscoket就比较合适了,它基于tcp,支持服务器主动向客户端发信息
实操
首先我们做一个简单的案例 这里使用springboot来做,首先创建一个空的springboot项目,如图为项目目录 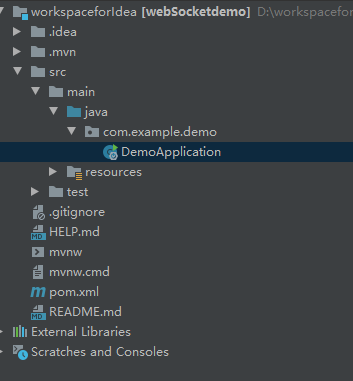
引入依赖
首先我们引入websocket的依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
添加配置类
这里添加一个websocket的配置类
@Configuration
public class WebSocketConfig {
@Bean
public ServerEndpointExporter serverEndpointExporter() {
return new ServerEndpointExporter();
}
}
创建服务器端
可以看到springboot集成的websocket有这些注解,在下面都用得到 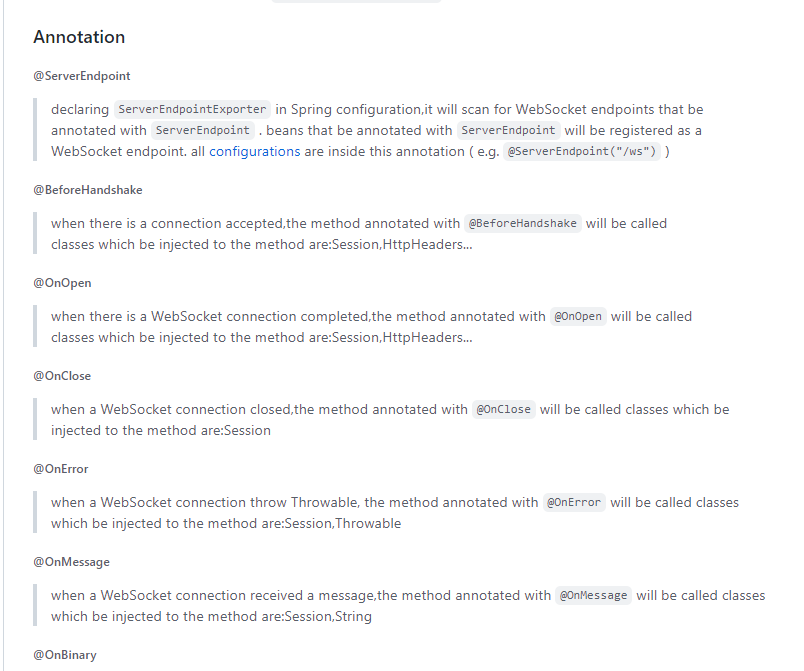
@Component
@Slf4j
@Service
@ServerEndpoint("/api/websocket/{sid}")
public class WebSocketServer {
private static int onlineCount = 0;
private static CopyOnWriteArraySet<WebSocketServer> webSocketSet = new CopyOnWriteArraySet<WebSocketServer>();
private Session session;
private String sid = "";
@OnOpen
public void onOpen(Session session, @PathParam("sid") String sid) {
this.session = session;
webSocketSet.add(this);
this.sid = sid;
addOnlineCount();
try {
sendMessage("conn_success");
log.info("有新窗口开始监听:" + sid + ",当前在线人数为:" + getOnlineCount());
} catch (IOException e) {
log.error("websocket IO Exception");
}
}
@OnClose
public void onClose() {
webSocketSet.remove(this);
subOnlineCount();
log.info("释放的sid为:"+sid);
log.info("有一连接关闭!当前在线人数为" + getOnlineCount());
}
@OnMessage
public void onMessage(String message, Session session) {
log.info("收到来自窗口" + sid + "的信息:" + message);
for (WebSocketServer item : webSocketSet) {
try {
item.sendMessage(message);
} catch (IOException e) {
e.printStackTrace();
}
}
}
@OnError
public void onError(Session session, Throwable error) {
log.error("发生错误");
error.printStackTrace();
}
public void sendMessage(String message) throws IOException {
this.session.getBasicRemote().sendText(message);
}
public static void sendInfo(String message, @PathParam("sid") String sid) throws IOException {
log.info("推送消息到窗口" + sid + ",推送内容:" + message);
for (WebSocketServer item : webSocketSet) {
try {
if (sid == null) {
} else if (item.sid.equals(sid)) {
item.sendMessage(message);
}
} catch (IOException e) {
continue;
}
}
}
public static synchronized int getOnlineCount() {
return onlineCount;
}
public static synchronized void addOnlineCount() {
WebSocketServer.onlineCount++;
}
public static synchronized void subOnlineCount() {
WebSocketServer.onlineCount--;
}
public static CopyOnWriteArraySet<WebSocketServer> getWebSocketSet() {
return webSocketSet;
}
}
controller配置
配置前端页面访问地址和websocket连接地址
@Controller("web_Scoket_system")
@RequestMapping("/api/socket")
public class SystemController {
@GetMapping("/index/{userId}")
public ModelAndView socket(@PathVariable String userId) {
ModelAndView mav = new ModelAndView("/socket1");
mav.addObject("userId", userId);
return mav;
}
@ResponseBody
@RequestMapping("/socket/push/{cid}")
public Map pushToWeb(@PathVariable String cid, String message) {
Map<String,Object> result = new HashMap<>();
try {
WebSocketServer.sendInfo(message, cid);
result.put("code", cid);
result.put("msg", message);
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
}
前端页面
注意这里的websocket后的地址改成自己的ip地址,https则要用前缀wss
<html >
<head>
<meta charset="utf-8">
<title>springboot整合websocket</title>
<script type="text/javascript" src="js/jquery.min.js"></script>
</head>
<body>
<div id="main" style="width: 1200px;height:800px;"></div>
Welcome<br/><input id="text" type="text" />
<button onclick="send()">发送消息</button>
<hr/>
<button onclick="closeWebSocket()">关闭WebSocket连接</button>
<hr/>
<div id="message"></div>
</body>
<script type="text/javascript">
var websocket = null;
if('WebSocket' in window) {
websocket = new WebSocket("ws://192.168.152.1:8089/api/websocket/100");
} else {
alert('当前浏览器 Not support websocket')
}
websocket.onerror = function() {
setMessageInnerHTML("WebSocket连接发生错误");
};
websocket.onopen = function() {
setMessageInnerHTML("WebSocket连接成功");
}
var U01data, Uidata, Usdata
websocket.onmessage = function(event) {
console.log(event);
setMessageInnerHTML(event);
setechart()
}
websocket.onclose = function() {
setMessageInnerHTML("WebSocket连接关闭");
}
window.onbeforeunload = function() {
closeWebSocket();
}
function setMessageInnerHTML(innerHTML) {
document.getElementById('message').innerHTML += innerHTML + '<br/>';
}
function closeWebSocket() {
websocket.close();
}
function send() {
var message = document.getElementById('text').value;
websocket.send('{"msg":"' + message + '"}');
setMessageInnerHTML(message + " ");
}
</script>
</html>
运行结果
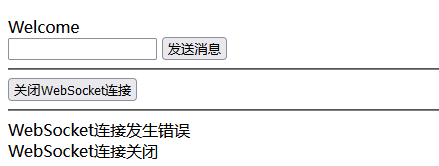  这里看到连接出现错误,一段时间的查找后,发现缺少一个配置类
添加配置类
@Configuration
public class WebSocketConfig {
@Bean
public ServerEndpointExporter serverEndpointExporter() {
return new ServerEndpointExporter();
}
}
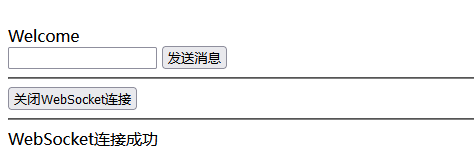
|