一、多选框基础?
多选框(Checkbox)由?选择点?和 提示文字?两部分组成??
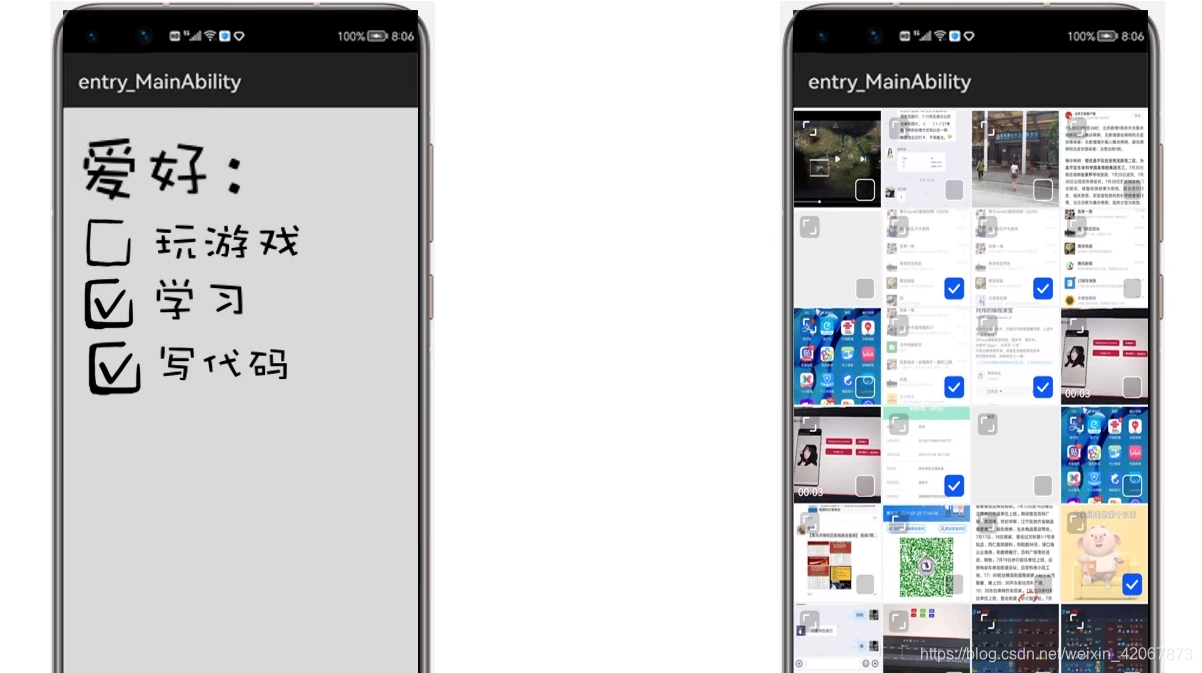
XML中定义组件:
- ohos:marked:true表示选中,false表示未被选中
- ohos:check_element:传数据是图片,表示设置多选框的样式
<?xml version="1.0" encoding="utf-8"?>
<DirectionalLayout
xmlns:ohos="http://schemas.huawei.com/res/ohos"
ohos:height="match_parent"
ohos:width="match_parent"
ohos:alignment="center"
ohos:orientation="vertical">
<Checkbox
ohos:id="$+id:cb"
ohos:height="match_content"
ohos:width="match_content"
ohos:text="多选框"
ohos:background_element="#21a8fd"
ohos:text_size="30fp"
ohos:marked="false"
/>
</DirectionalLayout>
Java中定义业务逻辑:
- isChecked():判断多选框是否被选中,返回值为boolean类型,true表示选中,false表示未选中
- setCheckedStateChangedListener(context):设置多选框监听事件
public class MainAbilitySlice extends AbilitySlice implements AbsButton.CheckedStateChangedListener {
@Override
public void onStart(Intent intent) {
super.onStart(intent);
super.setUIContent(ResourceTable.Layout_ability_main);
//1、找到组件
Checkbox cb = (Checkbox) findComponentById(ResourceTable.Id_cb);
//2、设置多选框组件
//true:表示选中, false表示未选中
//cb.setChecked(false);
//判断当前的多选框是否被选中
// boolean checked = cb.isChecked();
// if(checked) {
// MyToast.showDialog(this, "被选中了");
// } else {
// MyToast.showDialog(this, "没有选中");
// }
//给多选框添加状态监听事件
cb.setCheckedStateChangedListener(this);
}
@Override
public void onActive() {
super.onActive();
}
@Override
public void onForeground(Intent intent) {
super.onForeground(intent);
}
//当多选框的状态改变后就自动被调用
//参数1:AbsButton:继承于Button,其实就代表状态被改变的多选框
//参数2:表示当前多选框的状态,true:选中,false:未选中
@Override
public void onCheckedChanged(AbsButton absButton, boolean b) {
if(b) {
MyToast.showDialog(this, "被选中了");
} else {
MyToast.showDialog(this, "未被选中");
}
}
}
?二、案例分析
做一个时间格式调整器,通过多选框选择是否去显示年、月、日、时、分、秒等
XML中定义组件:
<?xml version="1.0" encoding="utf-8"?>
<DirectionalLayout
xmlns:ohos="http://schemas.huawei.com/res/ohos"
ohos:height="match_parent"
ohos:width="match_parent"
ohos:orientation="vertical">
<Clock
ohos:id="$+id:clock"
ohos:height="match_content"
ohos:width="match_content"
ohos:mode_24_hour="yyyy年MM月dd日 HH:mm:ss"
ohos:background_element="#21a8fd"
ohos:text_color="#FFFFFF"
ohos:text_size="30fp"
/>
<Checkbox
ohos:id="$+id:year"
ohos:height="match_content"
ohos:width="match_content"
ohos:text="年"
ohos:background_element="#21a9fd"
ohos:text_size="30fp"
ohos:top_margin="10vp"
ohos:left_margin="30vp"
/>
<Checkbox
ohos:id="$+id:mouth"
ohos:height="match_content"
ohos:width="match_content"
ohos:text="月"
ohos:background_element="#21a9fd"
ohos:text_size="30fp"
ohos:top_margin="10vp"
ohos:left_margin="30vp"
/>
<Checkbox
ohos:id="$+id:day"
ohos:height="match_content"
ohos:width="match_content"
ohos:text="日"
ohos:background_element="#21a9fd"
ohos:text_size="30fp"
ohos:top_margin="10vp"
ohos:left_margin="30vp"
/>
<Checkbox
ohos:id="$+id:hour"
ohos:height="match_content"
ohos:width="match_content"
ohos:text="时"
ohos:background_element="#21a9fd"
ohos:text_size="30fp"
ohos:top_margin="10vp"
ohos:left_margin="30vp"
/>
<Checkbox
ohos:id="$+id:minutes"
ohos:height="match_content"
ohos:width="match_content"
ohos:text="分"
ohos:background_element="#21a9fd"
ohos:text_size="30fp"
ohos:top_margin="10vp"
ohos:left_margin="30vp"
/>
<Checkbox
ohos:id="$+id:second"
ohos:height="match_content"
ohos:width="match_content"
ohos:text="秒"
ohos:background_element="#21a9fd"
ohos:text_size="30fp"
ohos:top_margin="10vp"
ohos:left_margin="30vp"
/>
<Button
ohos:id="$+id:submit"
ohos:height="match_content"
ohos:width="match_content"
ohos:background_element="#FF0000"
ohos:text="确定"
ohos:text_size="30fp"
ohos:text_color="#FFFFFF"
ohos:top_margin="20vp"
ohos:left_margin="30vp"
/>
</DirectionalLayout>
Java中撰写逻辑代码:
本案例设计多个组件,其中有6个多选框,如果一个一个判断多选框的状态太麻烦,现在通过一个撰写一个检测方法来判断是否被选中
public class MainAbilitySlice extends AbilitySlice implements Component.ClickedListener {
Clock clock = null; //时钟组件
Button but = null; //确定按钮
@Override
public void onStart(Intent intent) {
super.onStart(intent);
super.setUIContent(ResourceTable.Layout_ability_main);
//1、找到组件
clock = (Clock) findComponentById(ResourceTable.Id_clock);
but = (Button) findComponentById(ResourceTable.Id_submit);
//2、按钮绑定点击事件
but.setClickedListener(this);
}
@Override
public void onActive() {
super.onActive();
}
@Override
public void onForeground(Intent intent) {
super.onForeground(intent);
}
@Override
public void onClick(Component component) {
//判断6个多选框的状态
String year = check(ResourceTable.Id_year, "yyyy年");
String mouth = check(ResourceTable.Id_mouth, "MM月");
String day = check(ResourceTable.Id_day, "dd日");
String hour = check(ResourceTable.Id_hour, "HH:");
String minute = check(ResourceTable.Id_minutes, "mm:");
String second = check(ResourceTable.Id_second, "ss");
//拼接时间显示格式
String result = year + mouth + day + " " + hour + minute + second;
//格式判断, 也即 result == " "
if(result.length() == 1) {
MyToast.showDialog(this, "不能一个都不选");
return;
}
//设置时间格式
clock.setFormatIn24HourMode(result);
}
//定义一个方法
//作用:判断多选框有没有被选中
//如果选中了,就返回字符串
//如果没有被选中,则返回一个长度为0的字符串
public String check(int id, String result) {
//根据传递过来的id判断多选框选中与否
Checkbox cb = (Checkbox) findComponentById(id);
//判断当前的框是否被选中
if(cb.isChecked()) {
return result;
} else {
return "";
}
}
}
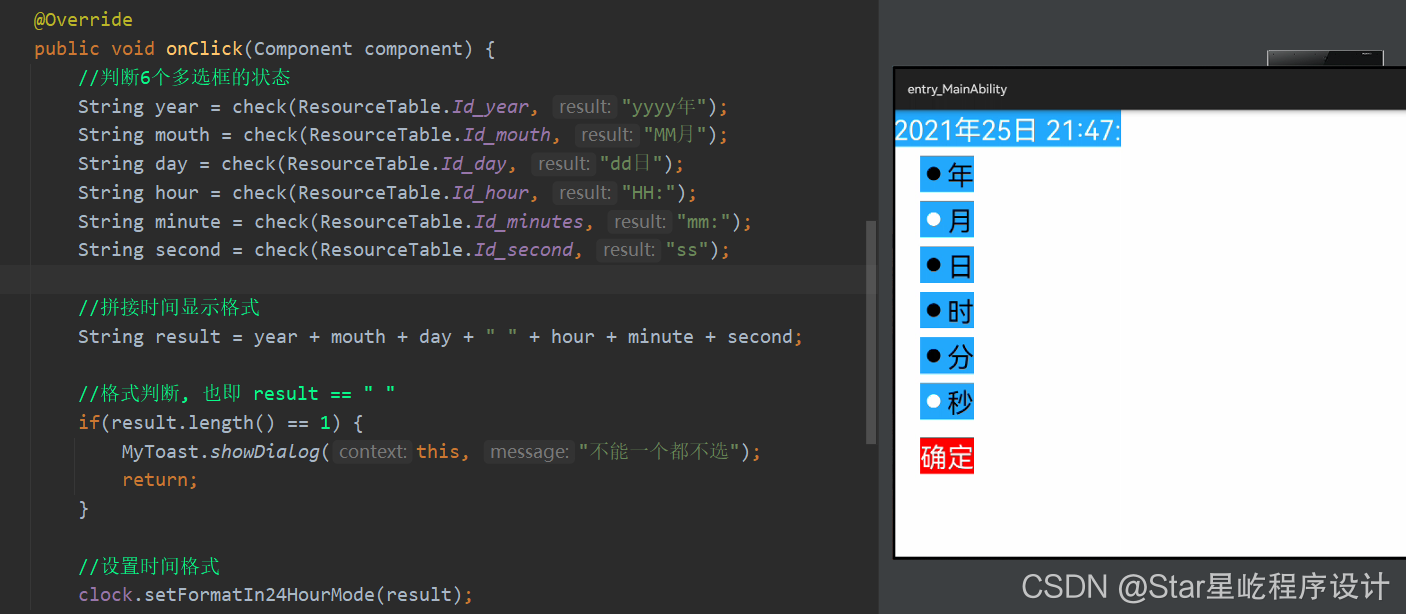
|