前言
前几期讲过类,构造函数,运算符重载,这一期就利用这三块的知识做一期简单的应用,用C++封装顺序表。
一、顺序表是什么?
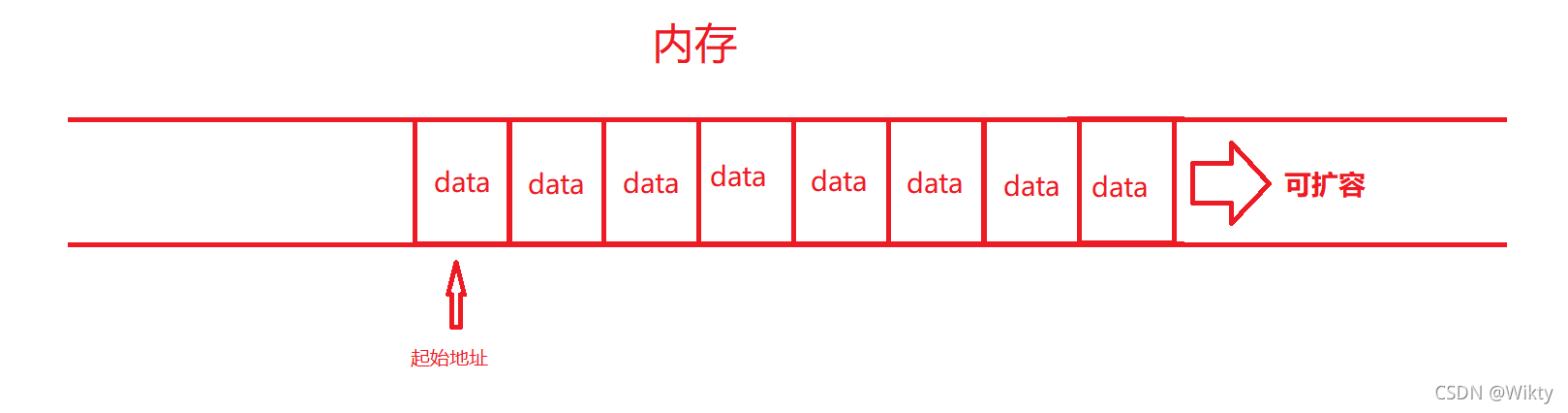 如图所示,在内存中的一块连续的空间 这种内存布局非常像数组,因此也被成为动态数组
在C++标椎模板库中有一个顺序表vector容器,我们要做的就是封装一个丐版的vector容器
二、需求分析
1.定义Myvector类,和内部接口
2.初始化、销毁、深拷贝
3.自动扩容
4.增删查改
5.获取大小和容量
6.用 [ 索引 ] 访问数据
三、具体实现
1.定义Myvector类,和内部接口
template<typename Type>
class Myvector
{
protected:
Type* data;
int _size;
int _capacity;
public:
Myvector();
Myvector(Myvector& vec);
~Myvector();
void EnlargedCapacity();
Myvector& pushback(Type data);
Myvector& pushfront(Type data);
Myvector& popback();
Myvector& popfront();
int size();
int capacity();
Type operator[](Type indexes);
Myvector& operator=(Myvector& vec);
};
2.初始化、销毁、深拷贝
template<typename Type>
Myvector<Type>::Myvector()
{
_size = 0;
_capacity = 4;
data = new Type[_capacity];
}
template<typename Type>
Myvector<Type>::Myvector(Myvector<Type>& vec)
{
this->data = new Type[vec._size];
memcpy(this->data, vec.data, _size * sizeof(Type));
this->_capacity = vec._capacity;
this->_size = vec._size;
}
template<typename Type>
Myvector<Type>& Myvector<Type>::operator=(Myvector& vec)
{
this->data = new Type[vec._size];
memcpy(this->data, vec.data, _size * sizeof(Type));
this->_capacity = vec._capacity;
this->_size = vec._size;
return *this;
}
template<typename Type>
Myvector<Type>::~Myvector()
{
delete[] data;
data = NULL;
_size = 0;
_capacity = 0;
}
3.自动扩容
template<typename Type>
void Myvector<Type>::EnlargedCapacity()
{
if (_size >= _capacity)
{
_capacity *= 2;
int* p = new Type[_capacity];
memcpy(p, data, _size * sizeof(Type));
delete[] data;
data = p;
p = nullptr;
}
}
4.增删查改
template<typename Type>
Myvector<Type>& Myvector<Type>::pushback(Type data)
{
EnlargedCapacity();
this->data[_size] = data;
_size++;
return *this;
}
template<typename Type>
Myvector<Type>& Myvector<Type>::popback()
{
_size--;
return *this;
}
template<typename Type>
Myvector<Type>& Myvector<Type>::pushfront(Type data)
{
EnlargedCapacity();
for (int i = 0; i < _size; i++)
this->data[_size - i] = this->data[_size - i - 1];
this->data[0] = data;
return *this;
}
template<typename Type>
Myvector<Type>& Myvector<Type>::popfront()
{
_size--;
for (int i = 0; i < _size; i++)
this->data[i] = this->data[i + 1];
return *this;
}
5.获取大小和容量
template<typename Type>
int Myvector<Type>::size()
{
return this->_size;
}
template<typename Type>
int Myvector<Type>::capacity()
{
return this->_capacity;
}
6.用 [ 索引 ] 访问数据
template<typename Type>
Type Myvector<Type>::operator[](Type indexes)
{
return this->data[indexes];
}
全部代码:
#include<iostream>
template<typename Type>
class Myvector
{
protected:
Type* data;
int _size;
int _capacity;
public:
Myvector();
Myvector(Myvector& vec);
~Myvector();
void EnlargedCapacity();
Myvector& pushback(Type data);
Myvector& pushfront(Type data);
Myvector& popback();
Myvector& popfront();
int size();
int capacity();
Type operator[](Type indexes);
Myvector& operator=(Myvector& vec);
};
template<typename Type>
Myvector<Type>::Myvector()
{
_size = 0;
_capacity = 4;
data = new Type[_capacity];
}
template<typename Type>
Myvector<Type>::Myvector(Myvector<Type>& vec)
{
this->data = new Type[vec._size];
memcpy(this->data, vec.data, _size * sizeof(Type));
this->_capacity = vec._capacity;
this->_size = vec._size;
}
template<typename Type>
Myvector<Type>& Myvector<Type>::operator=(Myvector& vec)
{
this->data = new Type[vec._size];
memcpy(this->data, vec.data, _size * sizeof(Type));
this->_capacity = vec._capacity;
this->_size = vec._size;
return *this;
}
template<typename Type>
Myvector<Type>::~Myvector()
{
delete[] data;
data = NULL;
_size = 0;
_capacity = 0;
}
template<typename Type>
void Myvector<Type>::EnlargedCapacity()
{
if (_size >= _capacity)
{
_capacity *= 2;
int* p = new Type[_capacity];
memcpy(p, data, _size * sizeof(Type));
delete[] data;
data = p;
p = nullptr;
}
}
template<typename Type>
Myvector<Type>& Myvector<Type>::pushback(Type data)
{
EnlargedCapacity();
this->data[_size] = data;
_size++;
return *this;
}
template<typename Type>
Myvector<Type>& Myvector<Type>::popback()
{
_size--;
return *this;
}
template<typename Type>
Myvector<Type>& Myvector<Type>::pushfront(Type data)
{
EnlargedCapacity();
for (int i = 0; i < _size; i++)
this->data[_size - i] = this->data[_size - i - 1];
this->data[0] = data;
return *this;
}
template<typename Type>
Myvector<Type>& Myvector<Type>::popfront()
{
_size--;
for (int i = 0; i < _size; i++)
this->data[i] = this->data[i + 1];
return *this;
}
template<typename Type>
int Myvector<Type>::size()
{
return this->_size;
}
template<typename Type>
int Myvector<Type>::capacity()
{
return this->_capacity;
}
template<typename Type>
Type Myvector<Type>::operator[](Type indexes)
{
return this->data[indexes];
}
|