信号编程
信号低级编程实战
通过信号来kill进程
代码:
#include<stdio.h>
#include<signal.h>
void handler(int signum)
{
printf("get signum=%d\n",signum);
switch(signum){
case 2:
printf("SIGINT\n");
break;
case 9:
printf("SIGKILL\n");
break;
case 10:
printf("SIGUSR1\n");
break;
}
printf("never quit\n");
}
int main()
{
signal(SIGINT,handler);
signal(SIGKILL,handler);
signal(SIGUSR1,handler);
while(1);
return 0;
}
通过ctrl + C 是结束不了该进程的
通过ps -aux | grep Pro 来查询当前进程的pid
然后运行以下代码:
#include<stdio.h>
#include<stdlib.h>
#include<sys/types.h>
#include<signal.h>
int main(int argc,char **argv)
{
int signum;
int pid;
char cmd[128] = {0};
signum = atoi(argv[1]);
pid = atoi(argv[2]);
printf("num = %d,pid =%d\n",signum,pid);
sprintf(cmd,"kill -%d %d",signum,pid);
system(cmd);
return 0;
}
执行上方代码,命令参数为:./a.out 9 (进程pid)
然后就kill掉该进程了
执行结果如下:
执行第一个代码后
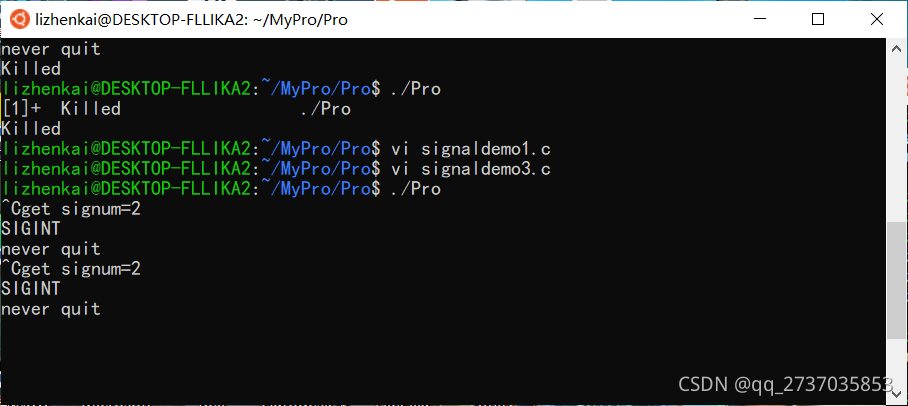
阻塞在这里
然后开启另外一个终端,查询当前pid值,我的程序命名为Pro
ps -aux | grep Pro
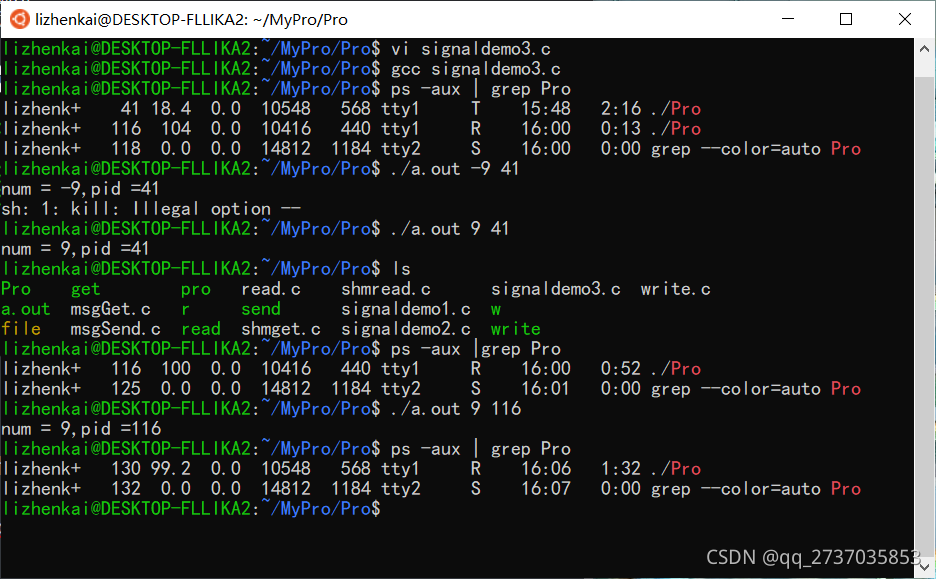
然后运行第二个代码
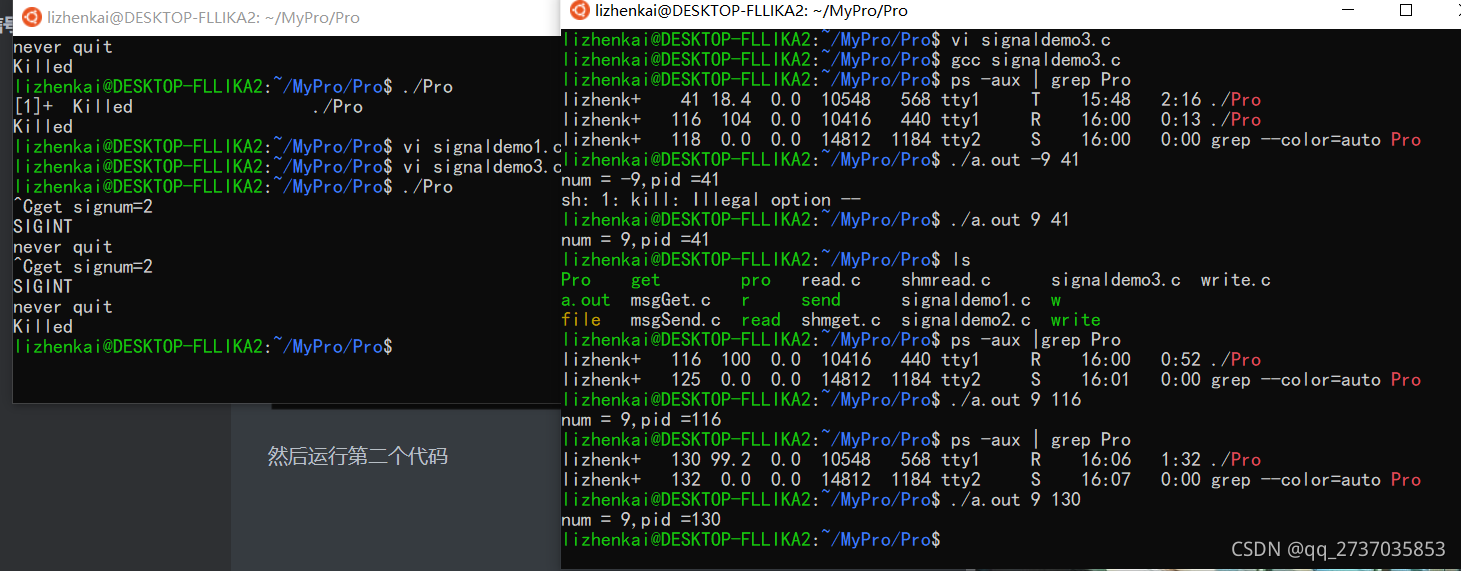
就kill掉该进程了。
信号携带消息高级编程实战
函数原型
NAME sigaction, rt_sigaction - examine and change a signal action
SYNOPSIS #include <signal.h>
int sigaction(int signum, const struct sigaction *act,
struct sigaction *oldact);
Feature Test Macro Requirements for glibc (see feature_test_macros(7)):
sigaction(): _POSIX_C_SOURCE
siginfo_t: _POSIX_C_SOURCE >= 199309L
DESCRIPTION The sigaction() system call is used to change the action taken by a process on receipt of a specific signal. (See signal(7) for an overview of signals.)
signum specifies the signal and can be any valid signal except SIGKILL and SIGSTOP.
If act is non-NULL, the new action for signal signum is installed from act. If oldact is non-NULL, the previous action is saved in oldact.
The sigaction structure is defined as something like:
struct sigaction {
void (*sa_handler)(int);
void (*sa_sigaction)(int, siginfo_t *, void *);
sigset_t sa_mask;
int sa_flags;
void (*sa_restorer)(void);
};
On some architectures a union is involved: do not assign to both sa_handler and sa_sigaction.
代码如下:
send.c
#include<stdio.h>
#include<signal.h>
#include<stdlib.h>
#include<unistd.h>
#include<sys/types.h>
int main(int argc,char **argv)
{
int signum;
int pid;
signum = atoi(argv[1]);
pid = atoi(argv[2]);
union sigval value;
value.sival_int = 100;
sigqueue(pid,signum,value);
printf("%d,done\n",getpid());
return 0;
}
signal.c
#include<stdio.h>
#include<stdlib.h>
#include<signal.h>
#include<unistd.h>
#include<sys/types.h>
void handler(int signum,siginfo_t *info ,void *context)
{
printf("get signum = %d\n",signum);
if(context != NULL){
printf("get data = %d\n",info->si_int);
printf("get data =%d\n",info->si_value.sival_int);
printf("from %d\n",info->si_pid);
}
}
int main()
{
struct sigaction act;
printf("pid = %d\n",getpid());
act.sa_sigaction = handler;
act.sa_flags = SA_SIGINFO;
sigaction(SIGUSR1,&act,NULL);
while(1);
return 0;
}
发送信号函数原型
NAME sigqueue - queue a signal and data to a process
SYNOPSIS #include <signal.h>
int sigqueue(pid_t pid, int sig, const union sigval value);
Feature Test Macro Requirements for glibc (see feature_test_macros(7)):
sigqueue(): _POSIX_C_SOURCE >= 199309L
DESCRIPTION sigqueue() sends the signal specified in sig to the process whose PID is given in pid. The permissions required to send a signal are the same as for kill(2). As with kill(2), the null signal (0) can be used to check if a process with a given PID exists.
The value argument is used to specify an accompanying item of data (either an integer or a pointer value) to be sent with the signal, and has the following
type:
union sigval { int sival_int; void *sival_ptr; };
If the receiving process has installed a handler for this signal using the
SA_SIGINFO flag to sigaction(2), then it can obtain this data via the si_value
field of the siginfo_t structure passed as the second argument to the handler.
Furthermore, the si_code field of that structure will be set to SI_QUEUE.
RETURN VALUE On success, sigqueue() returns 0, indicating that the signal was successfully queued to the receiving process. Otherwise, -1 is returned and errno is set to indicate the error.
ERRORS EAGAIN The limit of signals which may be queued has been reached. (See signal(7) for further information.)
执行结果如图:
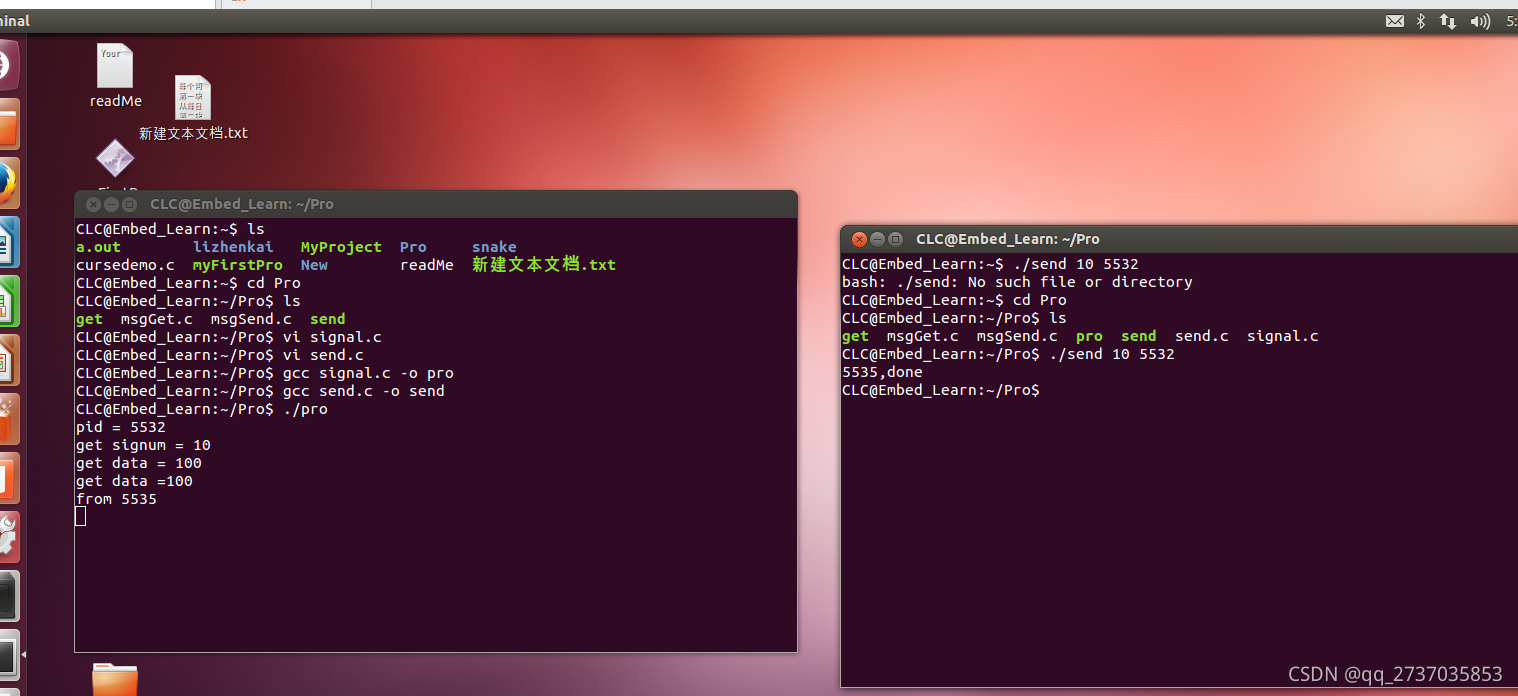
|