此为牛客网Linux C++课程1.20课程笔记。
1.open函数
open函数有两种,分别是打开一个已经存在的文件和创建并打开一个不存在的文件。
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int open(const char *pathname, int flags);
int open(const char *pathname, int flags, mode_t mode);
参数:
- pathname:要打开的文件路径
- flags:对文件的操作权限设置还有其他的设置
- mode:八进制的数,表示创建出的新的文件的操作权限,比如:0775
flags有三个必选项:O_RDONLY, O_WRONLY, O_RDWR 。 分别是只读、只写和读写,这三个设置是互斥的。
flags还有一些非必选项: O_CREAT 如果文件不存在则创建该文件 O_EXCL 如果使用O_CREAT选项且文件存在,则返回错误消息 O_NOCTTY 如果文件为终端,那么终端不可以调用open系统调用的那个进程的控制终端 O_TRUNC 如果文件已经存在则删除文件中原有数据 O_APPEND 以追加的方式打开
必选项和非必选项可以组合使用,中间用 | 隔开,如O_RDWR | O_CREAT | O_TRUNC。
mode参数用一个八进制数与取反后的当前进程的umask掩码进行按位与,得到新文件的操作权限。
返回值:返回一个新的文件描述符,如果调用失败,返回-1。
2. 关于errno
errno是Linux系统函数里面的一个全局变量,记录的是最近一次错误的错误号。 在/usr/include/asm-generic/errno.h和/usr/include/asm-generic/errno-base.h中记录了定义了这些错误号,如图: 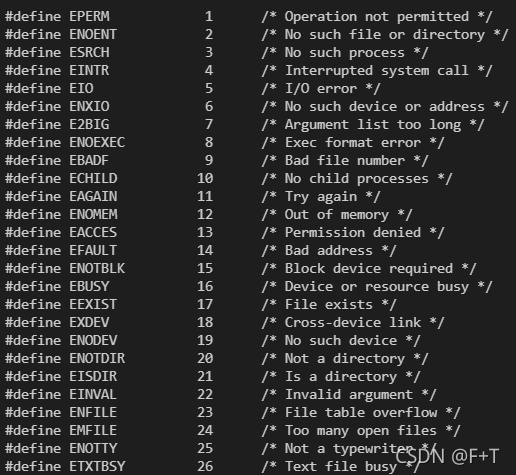 一个错误号对应一个错误信息。
我们可以用perror函数来打印errno对应的错误信息
#include <stdio.h>
void perror(const char *s);
s参数:用户描述,比如hello,最终输出的内容是 hello:xxx(实际的错误描述)。
如以下示例程序,打开一个不存在的文件a.txt
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
int main() {
int fd = open("a.txt", O_RDONLY);
if(fd == -1) {
perror("open file");
}
close(fd);
return 0;
}
输出的结果是:  是s参数+实际错误描述的组合。
3. read和write函数
read函数:
#include <unistd.h>
ssize_t read(int fd, void *buf, size_t count);
参数:
- fd:文件描述符,open得到的,通过这个文件描述符操作某个文件
- buf:需要读取数据存放的地方,数组的地址(传出参数)
- count:指定的数组的大小
返回值:一个整数,有以下情况:
- 成功:
>0: 返回实际的读取到的字节数 =0:文件已经读取完了 - 失败:-1 ,并且设置errno。
write函数:
#include <unistd.h>
ssize_t write(int fd, const void *buf, size_t count);
参数:
- fd:文件描述符,open得到的,通过这个文件描述符操作某个文件
- buf:要往磁盘写入的数据,数据
- count:要写的数据的实际的大小
返回值:
- 成功:实际写入的字节数
- 失败:返回-1,并设置errno
示例程序如下,该程序能够读入一个文件中的全部内容,复制到一个新创建的文件中:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
int main()
{
int fdread = open("english.txt", O_RDONLY);
if(fdread == -1) {
perror("open english.txt");
return -1;
}
int fdwrite = open("copy.txt", O_WRONLY | O_CREAT, 0664);
if(fdwrite == -1) {
perror("create and open aftercopy.txt");
return -1;
}
char buffer[1024] = {0};
int len = 0;
while((len = read(fdread, buffer, sizeof(buffer))) > 0) {
write(fdwrite, buffer, len);
}
close(fdwrite);
close(fdread);
return 0;
}
4. lseek函数
#include <sys/types.h>
include <unistd.h>
off_t lseek(int fd, off_t offset, int whence);
参数:
- fd:文件描述符,通过open得到的,通过这个fd操作某个文件
- offset:偏移量
- whence:以下三选一
SEEK_SET 设置文件指针的偏移量 SEEK_CUR 设置偏移量:当前位置 + 第二个参数offset的值 SEEK_END 设置偏移量:文件大小 + 第二个参数offset的值
返回值:返回文件指针的位置
该函数主要有以下作用:
- 移动文件指针到文件头:lseek(fd, 0, SEEK_SET);
- 获取当前文件指针的位置:lseek(fd, 0, SEEK_CUR);
- 获取文件长度:lseek(fd, 0, SEEK_END);
- 拓展文件的长度,当前文件10b, 110b, 增加了100个字节:lseek(fd, 100, SEEK_END), 注意:需要写一次数据。
扩展文件长度的示例程序如下:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <stdio.h>
int main() {
int fd = open("hello.txt", O_RDWR);
if(fd == -1) {
perror("open");
return -1;
}
int ret = lseek(fd, 100, SEEK_END);
if(ret == -1) {
perror("lseek");
return -1;
}
write(fd, " ", 1);
close(fd);
return 0;
}
|