一、集合与数组存储数据概述
集合、数组都是对多个数据进行存储操作的结构,简称java容器 说明:此时的存储,主要指的是内存层面的存储,不涉及到持久化的存储(.txt,.jpg,.avi,数据库中)
数组存储的特点
>一旦初始化以后,其长度就确定了。 >数组一旦定义好,其元素的类型也就确定了,我们也就只能操作指定类型的数据了。 ? ?比如:String[] arr;int[] arr1;Object[] arr2;
数组存储的弊端:
>一旦初始化后,其长度就不可修改 >数组中提供的方法非常有限,对于添加、删除、插入数据等操作,非常不便,同时效率不高。 >获取数组中实际元素的个数的需求,数组没有现成的属性或方法可用 >数组存储数据的特点:有序、可重复。对于无序、不可重复的需求,不能满足。
集合存储的优点:
解决数组存储数据方面的弊端。
二、集合框架
单列集合框架结构
|----Collection接口:单列集合,用来存储一个一个的对象 ? ? ? ? ? ? |----List接口:存储有序的、可重复的数据。? ---->"动态"数组 ? ? ? ? ? ? ? ? ? ?|ArrayList、LinkedList、Vector ? ? ? ? ? ? |----Set接口:存储无序的、不可重复的数据。 ---->高中讲的"集合" ? ? ? ? ? ? ? ? ? ?|HashSet、LinkedHashSet、TreeSet
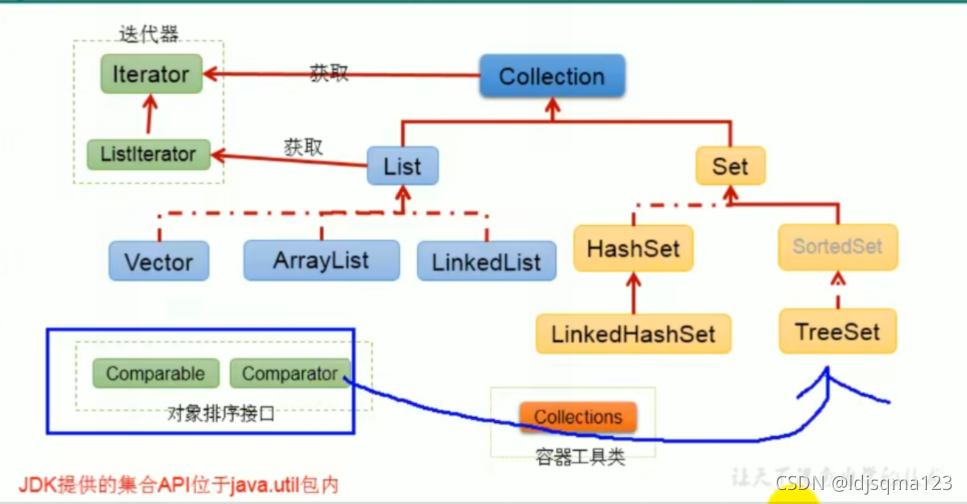
Collection接口常用方法:
- add(Object e):将元素e添加到集合coll中
- size():获取添加的元素的个数
- addAll(Collection coll1):将coll1集合中的元素添加到当前的集合中
- clear():清空集合元素
- isEmpty():判断当前集合是否为空
- contains():判断当前集合中是否包含obj
- containsAll(Collection coll2):判断形参coll2中的所有元素是否都存在于当前集合中
- remove(Obj ect obj):从当前集合中移除obj元素
- removeAll(Collection coll1):差集:从当前集合中移除coll1中所有的元素
- retainAll(Collection coll1):交集:获取当前集合和coll1集合的交集,并返回给当前集合
- equals(Object obj):要想返回true,需要当前集合和形参集合的元素都相同
- hashCode():返回当前对象的哈希值
- 集合 ---->数组:toArray()? ? ? ? ? 拓展:数组---->集合:调用Arrays类的静态方法asList()
- iterator():返回Iterator接口的实例,用于遍历集合元素。放在IteratorTest.java中测试
public class CollectingTest {
@Test
public void test1(){
Collection coll=new ArrayList();
//1.add(Object e):将元素e添加到集合coll中
coll.add("aa");
coll.add("bb");
coll.add(123);//自动装箱
coll.add(456);
coll.add(new Person("哈兰德",21));
coll.add(new String("姆巴佩"));
coll.add(false);
coll.add(new Date());
//2.size():获取添加的元素的个数
System.out.println(coll.size());//4
//3.addAll(Collection coll1):将coll1集合中的元素添加到当前的集合中
Collection coll1=new ArrayList();
coll1.add(4566);
coll1.add("cc");
coll.addAll(coll1);
System.out.println(coll.size());
System.out.println(coll);
//4.clear():清空集合元素
//coll.clear();
//5.isEmpty():判断当前集合是否为空
System.out.println(coll.isEmpty());
//6.contains():判断当前集合中是否包含obj
//我们在判断时会调用obj对象所在的类的equals()
boolean contains=coll.contains(123);
System.out.println(contains);
System.out.println(coll.contains(new String("姆巴佩")));
System.out.println(coll.contains(new Person("哈兰德",21)));
//7.containsAll(Collection coll2):判断形参coll2中的所有元素是否都存在于当前集合中
Collection coll2= Arrays.asList(123,456);
System.out.println(coll.containsAll(coll2));
}
@Test
public void test2(){
//8.remove(Obj ect obj):从当前集合中移除obj元素
Collection coll=new ArrayList();
coll.add("aa");
coll.add("bb");
coll.add(123);
coll.add(456);
coll.add(new Person("哈兰德",21));
coll.add(new String("姆巴佩"));
coll.add(false);
coll.remove(123);
System.out.println(coll);
coll.remove(new Person("哈兰德",21));
//9.removeAll(Collection coll1):差集:从当前集合中移除coll1中所有的元素
Collection coll1=Arrays.asList(123,4567);
coll.remove(coll1);
System.out.println(coll);
}
@Test
public void test3() {
Collection coll = new ArrayList();
coll.add("aa");
coll.add("bb");
coll.add(123);
coll.add(456);
coll.add(new Person("哈兰德", 21));
coll.add(new String("姆巴佩"));
coll.add(false);
/* //10.retainAll(Collection coll1):交集:获取当前集合和coll1集合的交集,并返回给当前集合
Collection coll1=Arrays.asList(123,4567,789);
coll.retainAll(coll1); //求交集
System.out.println(coll);*/
//11.equals(Object obj):要想返回true,需要当前集合和形参集合的元素都相同
Collection coll1 = new ArrayList(); //ArrayList()有序,里面元素不能换位
coll1.add("aa");
coll1.add("bb");
coll1.add(456);
coll1.add(123);
coll1.add(new Person("哈兰德", 21));
coll1.add(new String("姆巴佩"));
coll1.add(false);
System.out.println(coll.equals(coll1));
}
@Test
public void Test4(){
Collection coll1 = new ArrayList();
coll1.add("aa");
coll1.add(456);
coll1.add(123);
coll1.add(new Person("哈兰德", 21));
coll1.add(new String("姆巴佩"));
coll1.add(false);
//12.hashCode():返回当前对象的哈希值
System.out.println(coll1.hashCode());
/*//13.集合 ---->数组:toArray()
Object[] arr = coll1.toArray();
for(int i=0;i<=arr.length;i++){
System.out.println(arr[i]);
}*/
//拓展:数组---->集合:调用Arrays类的静态方法asList()
List<String> list = Arrays.asList(new String[]{"aa", "bb", "cc"});
System.out.println(list);
List arr1 = Arrays.asList(new int[]{123,456});
System.out.println(arr1);//1
List arr2 = Arrays.asList(new Integer[]{123,456});
System.out.println(arr2.size());//2
//14.iterator():返回Iterator接口的实例,用于遍历集合元素。放在IteratorTest.java中测试
}
}
Collection集合与数组间的转换
//13.集合 ---->数组:toArray()
Object[] arr = coll1.toArray();
for(int i=0;i<=arr.length;i++){
System.out.println(arr[i]);
}
//拓展:数组---->集合:调用Arrays类的静态方法asList()
List<String> list = Arrays.asList(new String[]{"aa", "bb", "cc"});
System.out.println(list);
List arr1 = Arrays.asList(new int[]{123,456});
System.out.println(arr1);//1
List arr2 = Arrays.asList(new Integer[]{123,456});
System.out.println(arr2.size());//2
使用Collection集合存储对象,要求对象所属的类满足:
向Collection接口的实现类的对象中添加数据obj时,要求obj所在类要重写equals()
遍历Collection的两种方式
- 使用迭代器Iterator
- foreach循环(或增强for循环)
Iterator
遍历集合Collection元素
1.内部的方法:hasNest()和next() 2.集合对象每次调用iterator()方法都得到一个全新的迭代器对象, ? ?默认游标都在集合的第一个元素之前。 3.内部定义了remove(),可以在遍历的时候,删除集合中的元素,此方法不同于集合直接调用remove()
hasNest()和next()固定模式:
Iterator iterator = coll.iterator();
//hasNext():判断是否还有下一个元素
while(iterator.hasNext()){
//next():1.指针下移 2.将下移以后集合位置上的元素返回
System.out.println(iterator.next());
}
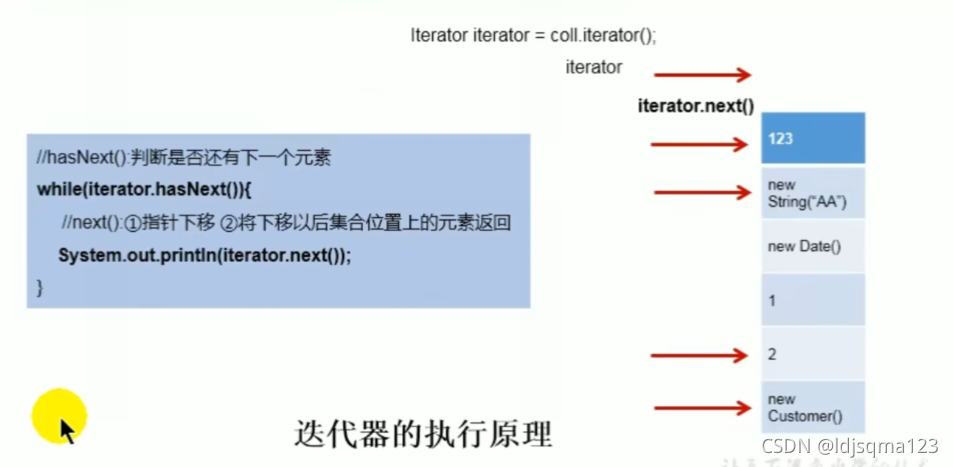
?remove()的使用
//测试Iterator中的remove()
@Test
public void test3(){
Collection coll=new ArrayList();
coll.add("aa");
coll.add("bb");
coll.add(123);
coll.add(456);
coll.add(new Person("哈兰德",21));
coll.add(new String("姆巴佩"));
coll.add(false);
//删除集合中的"bb"
Iterator iterator = coll.iterator();
while(iterator.hasNext()){
Object obj = iterator.next();
if("bb".equals(obj)){
iterator.remove();
}
}
//遍历集合
iterator = coll.iterator();
while(iterator.hasNext()){
System.out.println(iterator.next());
}
}
foreach循环
说明:内部仍然调用了迭代器
/*
* jdk5.0 新增了foreach循环,用于遍历集合、数组
*
* */
public class ForTest {
@Test
public void test1(){
Collection coll=new ArrayList();
coll.add("aa");
coll.add("bb");
coll.add(123);
coll.add(456);
coll.add(new Person("哈兰德",21));
//coll.add(new String("姆巴佩"));
coll.add(false);
//for(结婚元素的类型 局部变量 : 集合对象)
//内部仍然调用了迭代器
for(Object obj:coll){
System.out.println(obj);
}
}
@Test
public void test2(){
int[] arr=new int[]{1,2,3,4,5,6};
//for(结婚元素的类型 局部变量 : 数组对象)
for (int i:arr) {
System.out.println(i);
}
}
//面试题
@Test
public void test3(){
String[] arr=new String[]{"莱万","吉鲁","德佩"};
// //方式一:普通for赋值
// for (int i=0;i<arr.length;i++){
// arr[i]="金球奖";
// }
//方式二:增强for循环
for (String s:arr){
s="金球奖";
}
for (int i=0;i<arr.length;i++){
System.out.println(arr[i]);
}
}
}
List接口
常用实现类
|----Collection接口:单列集合,用来存储一个一个的对象 ?????? |----List接口:存储有序的、可重复的数据。?? ---->"动态"数组,替换原有的数组 ? ? ? ? ? ? ? ?|----ArrayList:作为List接口的主要实现类;线程不安全的,效率高;底层使用Object[] elementDate存储 ? ? ? ? ? ? ? ?|----LinkedList:对于频繁的插入、删除操作,使用此类效率比ArrayList高;底层使用双向链表存储 ? ? ? ? ? ? ? ?|----Vector:作为List接口的古老实现类;线程安全的,效率低;底层使用Object[] elementDate存储
存储的数据特点
存储有序的、可重复的数据。?? ---->"动态"数组,替换原有的数组
所有方法
void add(int index,object ele):在index位置插入ele元素 boolean addAll(int index,collection eles):从index位置开始将eLes中的所有元素添加进来 0bject get(int index)∶获取指定index位置的元素 int indexof(object obj):返回obj在集合中首次出现的位置 int lastIndex0f(object obj):返回obj在当前集合中末次出现的位置 0bject remove(int index):移除指定index位置的元素,并返回此元素 0bject set(int index,object ele):设置指定index位置的元素为ele list sublist(int fromIndex, int toIndex):返回从fromIndex到toIndex位置的子集合
常用方法
增:add(Object obj) 删:remove(int index) / remoove(Object obj) 改:set(int index,Object ele) 查:get(int index) 插:add(int index,Object ele) 长度:size() 遍历:1、Iterator迭代器方式 ? ? ? ? ? ?2、增强for循环 ? ? ? ? ? ?3、普通的循环
源码分析(巨难无比)
ArrayList的源码分析
2.1jdk 情况下7
* ArrayList List=new ArrayList();//底层创建了长度是10的Object[]数组elementDate
* List.add(123);// elementDate[0]=new Integer(123);
* ...
* List.add(11);//如果此次的添加导致底层elementDate数组容量不够,则扩容。
* 默认情况下,扩容为原来的容量的1.5倍,同时需要将原有数组中的数据复制到新的数组中。
*
* 结论:建议开发中使用带参的构造器:ArrayList List=new ArrayList(int capacity);
*
* 2.2jdk 8中ArrayList的变化
* ArrayList List=new ArrayList();//底层Object[] elementDate初始化为{},并没有创建长度为10的数组
* List.add(123);//第一次调用add()时,底层才创建了长度10的数组,并将数据123添加到elementDate[0]
* ...
* 后续的添加和扩容操作与jdk 7无异
* 2.3小结:jdk7中的ArrayList的对象的创建类似于单例的饿汉式,而jdk8中的ArrayList的对象的创建类似于
* 单例的懒汉式,延迟了数组的创建,节省内存。
LinkedList的源码分析
LinkedList list=new LinkedList();//内部声明了Node类型的first和last属性,默认值为null
* list.add(123);//将123封装到Node中创建了Node对象。
* //Node定义体现了LinkedList的双向链表的说法
存储的元素的要求
添加的对象,所在的类要重写equals()方法(一定必须)
Set接口
常用实现类
|----Collection接口:单列集合,用来存储一个一个的对象 ??????? |----Set接口:存储无序的、不可重复的数据。 ---->高中讲的"集合" ????????????? |----HashSet:作为Set接口的主要实现类;线程不安全的;可以存储null值 ?????????????????? |----LinkedHashSet:作为HashSet的子类;遍历其内部数据时,可以按照添加的顺序? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?遍历;在添加数据的同时,每个数据还维护了两个引用,记录此? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?数据前一个数据和后一个数据;对于频繁的遍历操作,? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?LinkedHashSet效率高于HashSet ????????????? |----TreeSet:可以按照添加对象的指定属性,进行排序。
存储的数据特点
以HashSet为例说明: ???? 1.无序性:不等于随机性。存储的数据在底层数组中并非按照数组索引的顺序添加,而是根据数据的哈希值决定的。 ???? 2.不可重复性:保证添加的元素按照equals()判断时,不能反悔true,即:相同的元素只能添加一个。
元素的添加过程:(以HashSet为例)(又是恶心的底层)
我们向HashSet中添加元素a,首先调用元素a所在类的hashCode()方法,计算元素a的哈希值,
此哈希值接着通过某种算法计算出在HashSet底层数组中的存放位置(即为:索引位置),判断
数组此位置上是否已经有元素:
如果此位之上没有其他元素,则元素a添加成功 ---->情况1
如果此位之上有其他元素b(或以链表形式存在的多个元素),则比较元素a与元素b的hash值:
如果hash值不相同,则元素a添加成功。 ---->情况2
如果hash值相同,进而需要调用元素a所在类的equals()方法:
equals()返回true,元素a添加失败
equals()返回false,则元素a添加成功。 ---->情况3
对于添加成功的情况2和情况3而言:元素a与已经存在指定索引位置上数据以链表的方式存储。
jdk 7:元素a放到数组中,指向原来的元素
jdk 8:原来的元素在数组中,指向元素a
总结:七上八下
HashSet底层:数据+链表的结构。
常用方法
Set接口中没有额外定义新的方法,使用的都是Collection中声明过的方法。
存储对象所在类的要求
要求:向Set(主要指:HashSet、LinkedHashSet)中添加的数据,其所在的类一定要重写hashCode()和equals() 要求:重写的hashCode()和equals()尽可能保持一致性:相等的对象必须具有相等的散列码
TreeSet
1.向TreeSet中添加的数据,要求是相同类的对象 2.两种排序方式:自然排序(实现Comparable接口) 和 定制排序(Comparator) ? ?自然排序中,比较两个对象是否相同的标准为:compareTo()返回0,不再是equals(). ? ?定制排序中,比较两个对象是否相同的标准为:compare()返回0,不再是equals().
常用的排序方式
自然排序:
@Test
public void test1(){
TreeSet set = new TreeSet();
//失败:不能添加不同类的对象
// set.add(123);
// set.add(456);
// set.add("登贝莱");
// set.add(new User("Tom", 12));
//举例一:
// set.add(34);
// set.add(-65);
// set.add(43);
// set.add(11);
// set.add(8);
//举例二:
set.add(new User("Tom", 12));
set.add(new User("Som", 15));
set.add(new User("Com", 62));
set.add(new User("Zom", 18));
Iterator iterator = set.iterator();
while (iterator.hasNext()){
System.out.println(iterator.next());
}
}
定制排序:
@Test
public void test2(){
Comparator com=new Comparator() {
//按照年龄从小到大排列
@Override
public int compare(Object o1, Object o2) {
return 0;
}
};
TreeSet set = new TreeSet();
set.add(new User("Tom", 12));
set.add(new User("Som", 15));
set.add(new User("Com", 62));
set.add(new User("Zom", 18));
Iterator iterator = set.iterator();
while (iterator.hasNext()){
System.out.println(iterator.next());
}
}
|