Ò»¡¢ÏîÄ¿´´½¨ÒÔ¼°Ò³ÃæÉèÖÃ
1.´´½¨ÏîÄ¿ 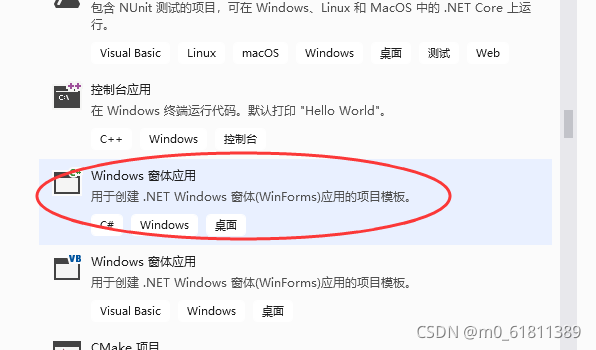 2.´°¿Ú´´½¨

¶þ¡¢´úÂ벿·Ö
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Net.Sockets;
using System.Windows.Forms;
using System.Net;
using System.Media;
using System.Threading;
namespace game1
{
public partial class Form1 : Form
{
private NetworkStream stream;
private TcpClient tcpClient;
SoundPlayer player = new SoundPlayer("E:/Win2/game1/G/y.wav");
Socket socket_send;
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
tcpClient = new TcpClient();
socket_send = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
IPEndPoint point = new IPEndPoint(IPAddress.Parse("10.1.230.74"), 3900);
socket_send.Connect(point);
try
{
tcpClient.Connect("10.1.230.74", 3900);
listBox1.Items.Add("Á¬½Ó³É¹¦");
stream = tcpClient.GetStream();
receive_stream();
}
catch
{
listBox1.Items.Add("·þÎñÆ÷δÆô¶¯");
}
}
void receive_stream()
{
byte[] receive_data = new byte[1024];
System.Text.Encoding.RegisterProvider(System.Text.CodePagesEncodingProvider.Instance);
System.Text.Encoding GBK = System.Text.Encoding.GetEncoding("GBK");
if (stream.CanRead)
{
int len = stream.Read(receive_data, 0, receive_data.Length);
string msg = GBK.GetString(receive_data, 0, receive_data.Length);
string str = "\r\n";
char[] str1 = str.ToCharArray();
string[] messy_code = { "ÿ?ÿ?[2J ", "[5m", "[44m", "[37;0m", "[1;33m", "[1;32m", "[1;31m" };
string[] msg1 = msg.Split(str1);
for (int j = 0; j < msg1.Length; j++)
{
msg1[j] = msg1[j].Replace(messy_code[0], " ");
msg1[j] = msg1[j].Replace(messy_code[1], " ");
msg1[j] = msg1[j].Replace(messy_code[2], " ");
msg1[j] = msg1[j].Replace(messy_code[3], " ");
msg1[j] = msg1[j].Replace(messy_code[4], " ");
msg1[j] = msg1[j].Replace(messy_code[5], " ");
msg1[j] = msg1[j].Replace(messy_code[6], " ");
listBox1.Items.Add(msg1[j]);
}
}
}
void send_stream(string str)
{
System.Text.Encoding.RegisterProvider(System.Text.CodePagesEncodingProvider.Instance);
System.Text.Encoding GBK = System.Text.Encoding.GetEncoding("GBK");
byte[] buffer = GBK.GetBytes(str + "\n");
stream.Write(buffer, 0, buffer.Length);
}
private void button2_Click(object sender, EventArgs e)
{
if (stream != null)
{
stream.Close();
tcpClient.Close();
socket_send.Close();
}
listBox1.Items.Add("ÒѾÍ˳öÓÎÏ·");
}
private void button5_Click(object sender, EventArgs e)
{
if (tcpClient.Connected)
{
string action = textBox1.Text.ToString();
listBox1.Items.Add("ÊäÈëµÄÐÅϢΪ:" + action);
send_stream(action);
receive_stream();
}
else
{
listBox1.Items.Add("Á¬½ÓÍ˳ö");
}
}
private void button3_Click(object sender, EventArgs e)
{
player.Load();
player.Play();
}
private void button4_Click(object sender, EventArgs e)
{
player.Stop();
}
int flag = 0;
private void timer1_Tick(object sender, EventArgs e)
{
Thread th = new Thread(play_pic);
th.IsBackground = true;
th.Start();
}
void play_pic()
{
flag++;
string picturePath = @"E:/Win2/game1/G" + flag + ".jpg";
pictureBox1.Image = Image.FromFile(picturePath);
if (flag == 5)
{
flag = 0;
}
}
}
}
½á¹ûͼ 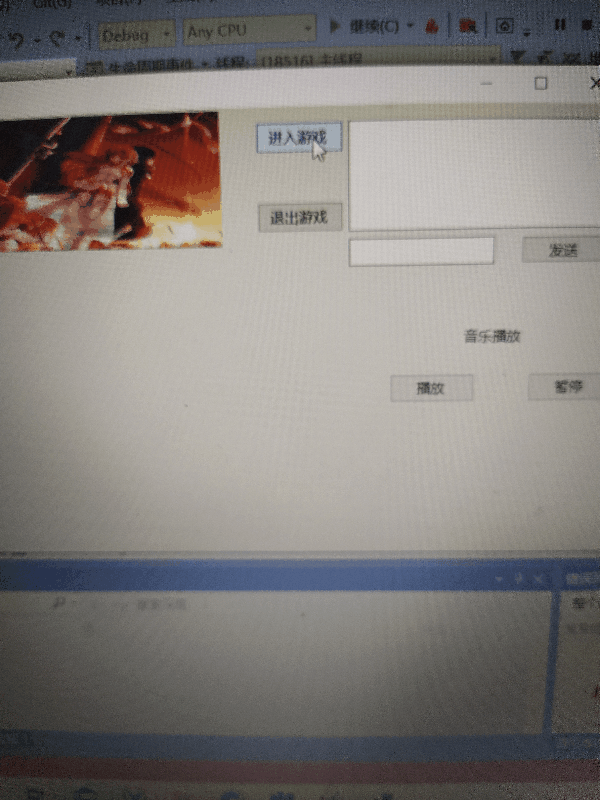
Èý¡¢×ܽá
ͨ¹ýÕâ´ÎµÄʵÑé,ÎÒ¶ÔC#¿ÉÊÓ»¯½çÃæÓÐÒ»¶¨µÄÁ˽â,¿ÉÒÔÓÃwavÎļþÀ´²¥·ÅÒôÀÖ,µ«ÊÇÔÚ×öµÄ¹ý³ÌÖÐÒ²³öÏÖһЩÎÊÌâ,¾ÍÊÇͼƬÎÞ·¨¸ü»»¡£
ËÄ¡¢²Î¿¼ÎÄÏ×
https://blog.csdn.net/qq_43279579/article/details/109693257?ops_request_misc=%257B%2522request%255Fid%2522%253A%2522163758681616780261990203%2522%252C%2522scm%2522%253A%252220140713.130102334.pc%255Fblog.%2522%257D&request_id=163758681616780261990203&biz_id=0&utm_medium=distribute.pc_search_result.none-task-blog-2blogfirst_rank_v2~rank_v29-1-109693257.pc_v2_rank_blog_default&utm_term=%E6%B8%B8%E6%88%8F&spm=1018.2226.3001.4450
https://blog.csdn.net/weixin_46628481/article/details/121445716?spm=1001.2014.3001.5501
|