package collection;
public class Collection01 {
public static void main(String[] args) {
集合的框架体系: Collection接口(单列集合) 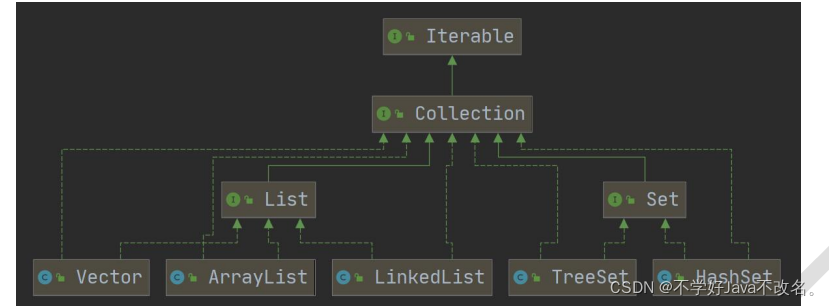
Map接口(双列接口):
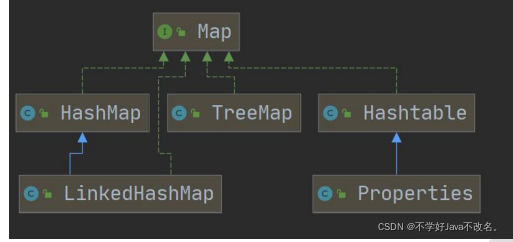
package collection;
import java.util.ArrayList;
import java.util.List;
public class Collection02 {
public static void main(String[] args) {
@SuppressWarnings({"all"})
List l1=new ArrayList();
l1.add("西游记");
l1.add(10);
l1.add(true);
System.out.println("l1:"+l1);
l1.remove(0);
l1.remove(true);
System.out.println("l1:"+l1);
System.out.println(l1.contains(true));
System.out.println(l1.size());
System.out.println(l1.isEmpty());
l1.clear();
System.out.println("l1:"+l1);
List l2=new ArrayList();
l2.add("三国演义");
l2.add("水浒传");
System.out.println("l2:"+l2);
l1.addAll(l2);
System.out.println("l1:"+l1);
l1.removeAll(l2);
System.out.println("l1:"+l1);
System.out.println(l1.containsAll(l2));
}
}
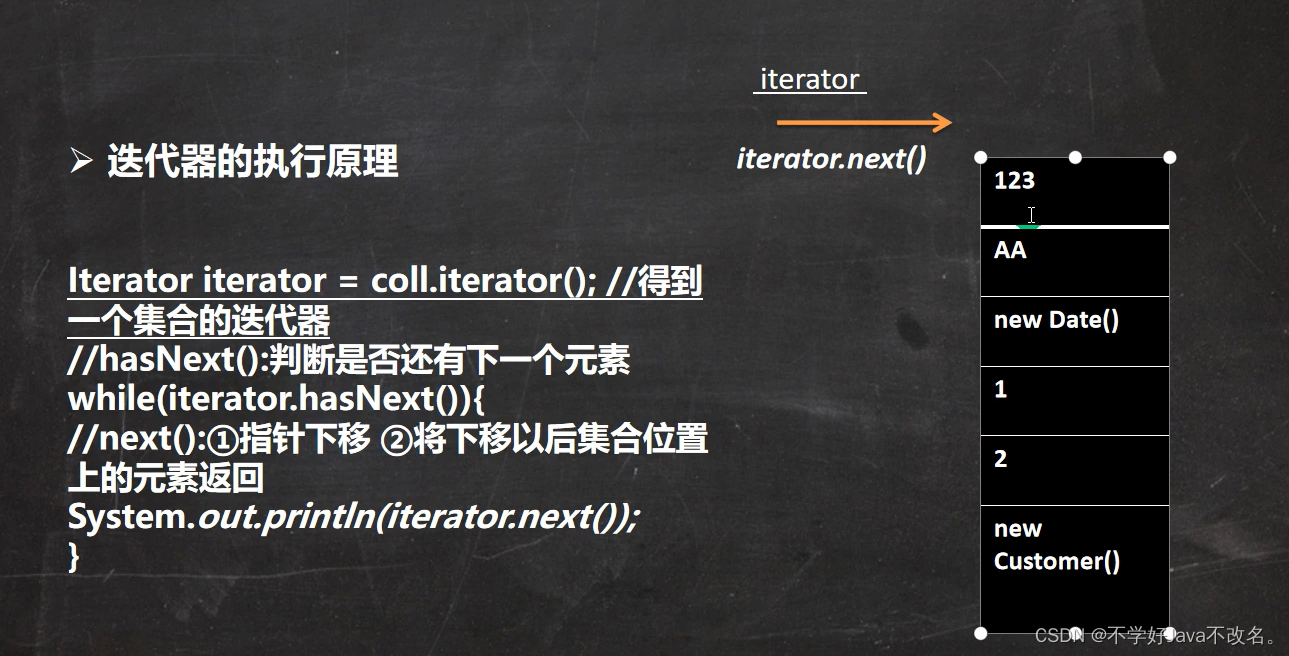 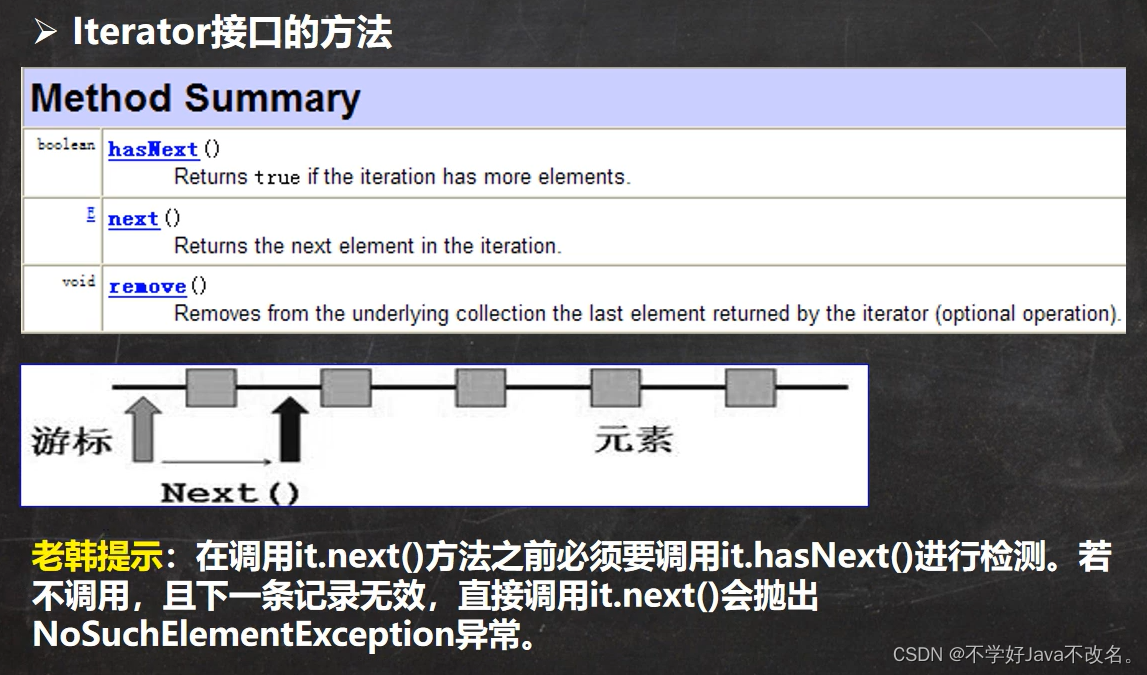
package collection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
public class Collection12 {
public static void main(String[] args) {
Collection col=new ArrayList();
col.add(new Book("西游记","吴承恩",100));
col.add(new Book("三国演义","吴贯中",98));
col.add(new Book("红楼梦","曹雪芹",93));
System.out.println("col:"+col);
Iterator iterator=col.iterator();
while(iterator.hasNext()){
Object obj=iterator.next();
System.out.println("obj:"+obj);
}
iterator=col.iterator();
while(iterator.hasNext()){
Object obj=iterator.next();
System.out.println("obj:"+obj);
}
}
}
class Book{
private String name;
private String author;
private double price;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public Book(String name, String author, double price) {
this.name = name;
this.author = author;
this.price = price;
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", author='" + author + '\'' +
", price=" + price +
'}';
}
}
package collection;
import java.util.ArrayList;
import java.util.Collection;
public class Collection03 {
public static void main(String[] args) {
Collection col=new ArrayList();
col.add(new Book("西游记","吴承恩",100));
col.add(new Book("三国演义","吴贯中",98));
col.add(new Book("红楼梦","曹雪芹",93));
for(Object o:col ){
System.out.println("o:"+o);
}
int[] nums={2,3,4};
for(int a:nums){
System.out.println(a);
}
}
}
package collection;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class Collection04 {
public static void main(String[] args) {
List l=new ArrayList();
l.add(new Dog("小黄",19));
l.add(new Dog("小黑",20));
l.add(new Dog("小蓝",21));
Iterator iterator=l.iterator();
while(iterator.hasNext()){
Object o=iterator.next();
System.out.println("o:"+o);
}
for(Object o:l){
System.out.println("o:"+o);
}
}
}
class Dog{
private String name;
private int age;
public Dog(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Dog{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
package collection;
import java.util.ArrayList;
import java.util.List;
public class Collection05 {
public static void main(String[] args) {
List l=new ArrayList();
l.add("哈哈");
l.add("呵呵");
l.add("呵呵");
System.out.println("l:"+l);
l.add("呵呵");
System.out.println("l:"+l);
System.out.println(l.get(3));
l.add(0,"你好");
List l2=new ArrayList();
l.addAll(1,l2);
System.out.println(l.get(0));
System.out.println(l.indexOf("呵呵"));
System.out.println(l.indexOf("呵呵"));
l.remove(0);
l.set(0,"你好");
List returnList=l.subList(2,3);
System.out.println("returnList:"+returnList);
}
}
package collection;
import java.util.ArrayList;
import java.util.List;
public class Collection06 {
public static void main(String[] args) {
List l=new ArrayList();
l.add("你");
l.add("是");
l.add("最棒");
l.add("的");
l.add("请相信自己");
l.add("加油");
l.add("相信自己");
l.add("奥里给");
l.add("奥里给");
l.add("奥里给");
l.add("奥里给");
l.add(2,"韩顺平教育");
l.get(4);
l.remove(5);
for(Object o:l){
System.out.println(o);
}
}
}
package collection;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class Collection07 {
public static void main(String[] args) {
List l=new ArrayList();
l.add("jack");
l.add("helo");
l.add("sdf");
Iterator iterator=l.iterator();
while (iterator.hasNext()) {
Object next = iterator.next();
System.out.println("next:"+next);
}
for(Object o:l){
System.out.println("o:"+o);
}
for(int i=0;i<l.size();i++){
System.out.println("对象:"+l.get(i));
}
}
}
package collection;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class Collection08 {
public static void main(String[] args) {
List l=new ArrayList();
l.add(new Book1("三国演义",200,"罗贯中"));
l.add(new Book1("水浒传",190,"施耐庵"));
l.add(new Book1("西游记",210,"吴承恩"));
sort(l);
Iterator iterator=l.iterator();
while (iterator.hasNext()) {
Object next = iterator.next();
System.out.println(next);
}
}
public static void sort(List l){
int listSize=l.size();
for(int i=0;i<listSize-1;i++){
for(int j=0;j<listSize-i-1;j++){
Book1 book1=(Book1)l.get(j);
Book1 book2=(Book1)l.get(j+1);
if(book1.getPrice()>book2.getPrice()){
l.set(j,book2);
l.set(j+1,book1);
}
}
}
}
}
class Book1{
private String name;
private double price;
private String author;
public Book1(String name, double price, String author) {
this.name = name;
this.price = price;
this.author = author;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
@Override
public String toString() {
return "名称:"+name+"\t"+"价格:"+price+"\t"+author;
}
}
|