makefile的编写
规则
命名:makefile / Makefile
规则中的三要素:目标,依赖,命令(TAB键起始)
子目标和终极目标的关系: 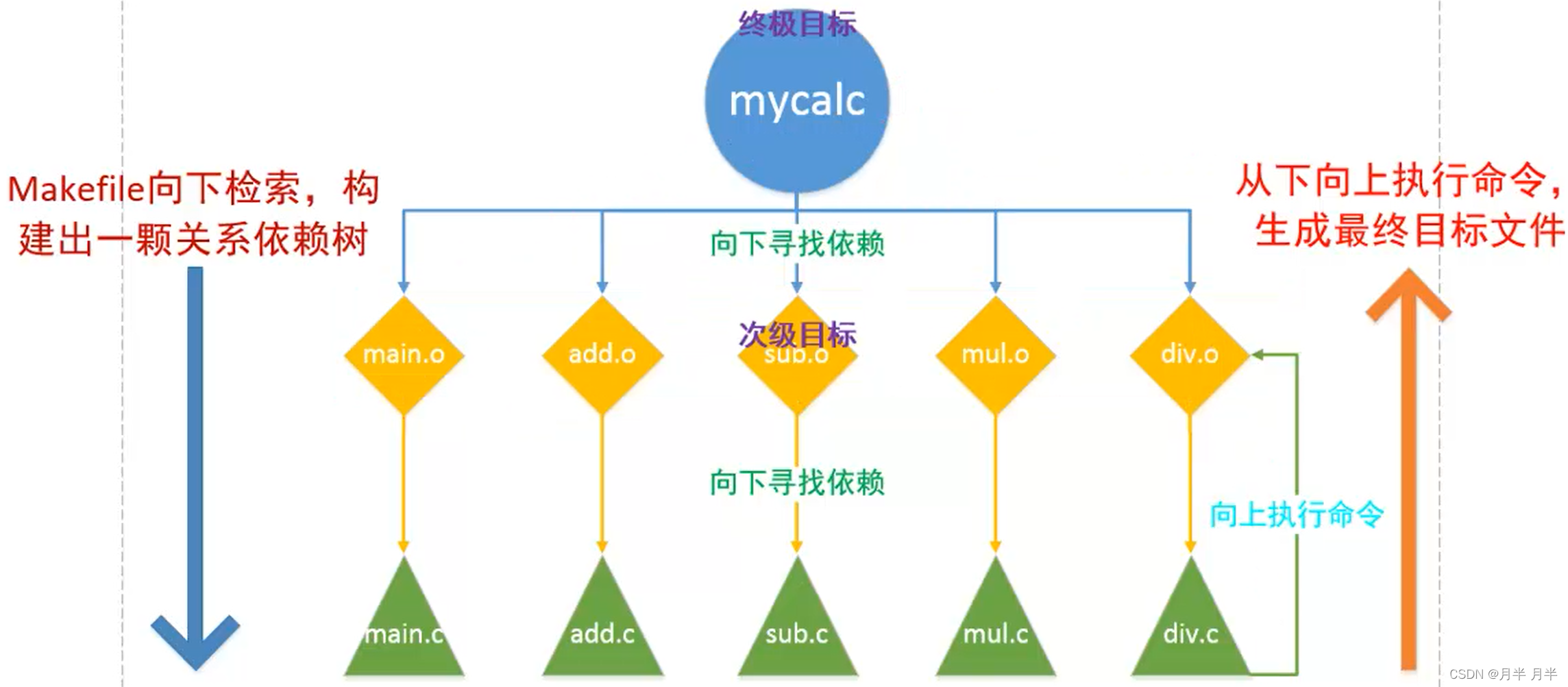 生成目标的流程及更新目标的原则: 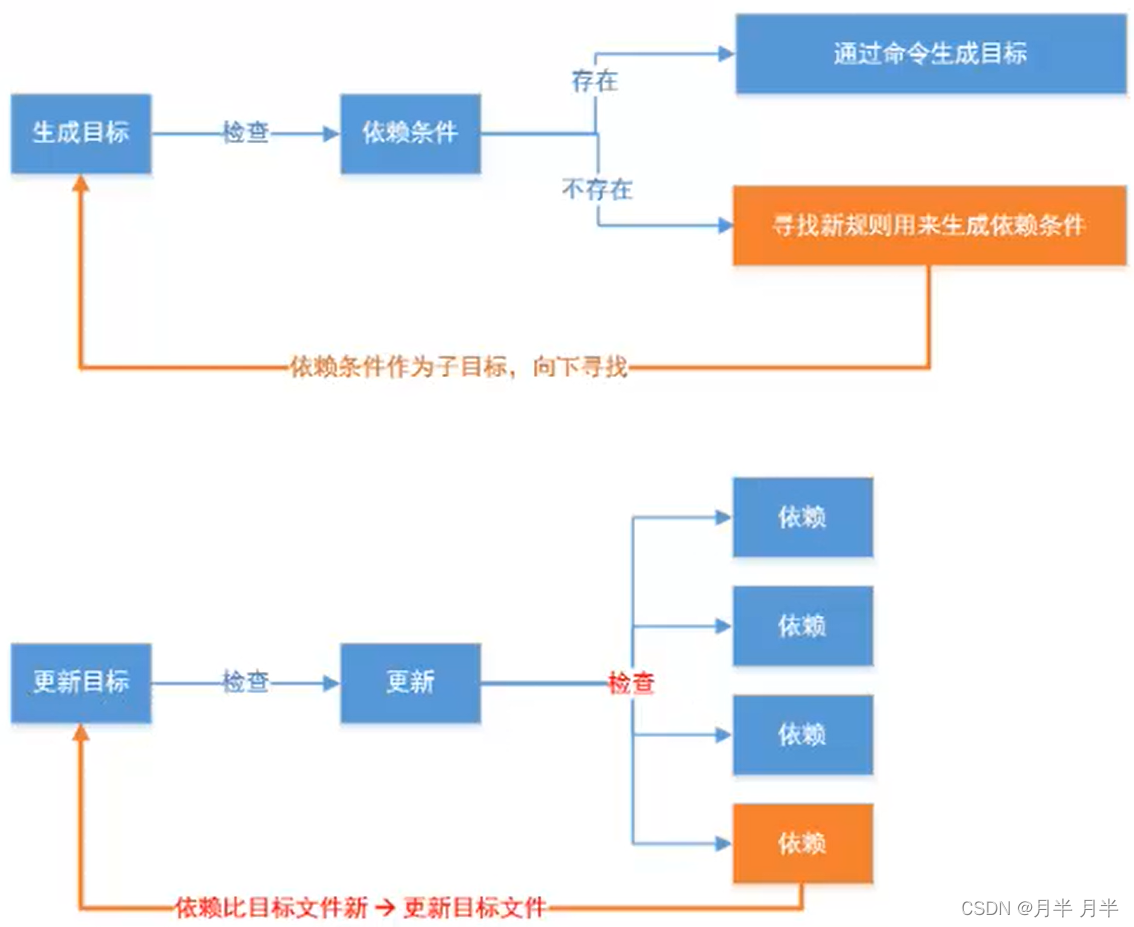
两个函数
三个自动变量
- $< 第一个依赖条件
- $^ 所有的依赖条件
- $@ 规则的目标
模式规则
%.o:%.c
gcc -c $< -o $@
系统IO函数
基本概念
- 文件描述符:
- int类型
- 一个进程最多可打开多少文件:1024-3(标准输入、标准输出、标准错误被默认打开)
- pcb
- 进程控制块
- 在其中有一个文件描述符表 – 数组[1024],从数组下标中选择未占用的最小的数
- 虚拟地址空间
- 用户区(0-3G)、内核区(3G-4G)
- 4G虚拟地址空间,从上往下依次为:
内核区 环境变量 栈(从上往下寻址) 共享库 堆(从下往上寻址) 未被初始化的全局变量 已初始化的全局变量 代码段
open和close
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int open(const char *pathname, int flags);
int open(const char *pathname, int flags, mode_t mode);
int creat(const char *pathname, mode_t mode);
#include <unistd.h>
int close(int fd);
打开方式:
- 必选项
- O_RDONLY 只读
- O_WRONLY 只写
- O_RDWR 可读写
- 可选项
- O_CREAT
- 文件权限,本地有一个掩码,文件的实际权限为给定的参数与本地掩码取反后按位与的结果,掩码可以临时更改
umask 0002 - O_TRUNC
- O_EXCL
- O_APPEND
int fd;
fd = open("hello.c", O_RDWR);
fd = open("myhello", O_RDWR | O_CREAT, 0777);
fd = open("myhello", O_RDWR | O_CREAT | O_EXCL, 0777);
fd = open("myhello", O_RDWR | O_TRUNC);
if (fd == -1)
{
perror("Open failed");
exit(1);
}
int ret = close(fd);
if(ret == -1)
{
perror("Close failed");
exit(1);
}
read和write
#include <unistd.h>
ssize_t read(int fd, void *buf, size_t count);
返回值:-1 读文件失败
0 文件读完了
>0 读取的字节数
ssize_t write(int fd, void *buf, size_t count);
读写文件示例
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
int main()
{
int fd = open("english.txt", O_RDONLY);
if (fd == -1)
{
perror("Open failed");
exit(1);
}
int fd1 = open("newfile", O_CREAT | O_WRONLY, 0664);
if (fd1 == -1)
{
perror("Open failed");
exit(1);
}
char buf[2048] = {0};
int count = read(fd, buf, sizeof(buf));
if(count == -1)
{
perror("Read failed");
exit(1);
}
while(count)
{
int ret = write(fd1, buf, count);
printf("write %d bytes\n", ret);
count = read(fd, buf, sizeof(buf));
}
close(fd);
close(fd1);
return 0;
}
lseek
#include <sys/types.h>
#include <unistd.h>
off_t lseek(int fd, off_t offset, int whence);
文件扩展示例:
int main()
{
int fd = open("english.txt", O_RDONLY);
if (fd == -1)
{
perror("Open failed");
exit(1);
}
int ret = lseek(fd, 0, SEEK_END);
printf("file length = %d\n", ret);
ret = lseek(fd, 2000, SEEK_END);
printf("file length = %d\n", ret);
write(fd, "a", 1);
close(fd);
return 0;
}
|