1.list容器基本概念
(1)list容器实际上就是一个链表,不同于vector容器,list容器是通过指针将数据链式储存起来;
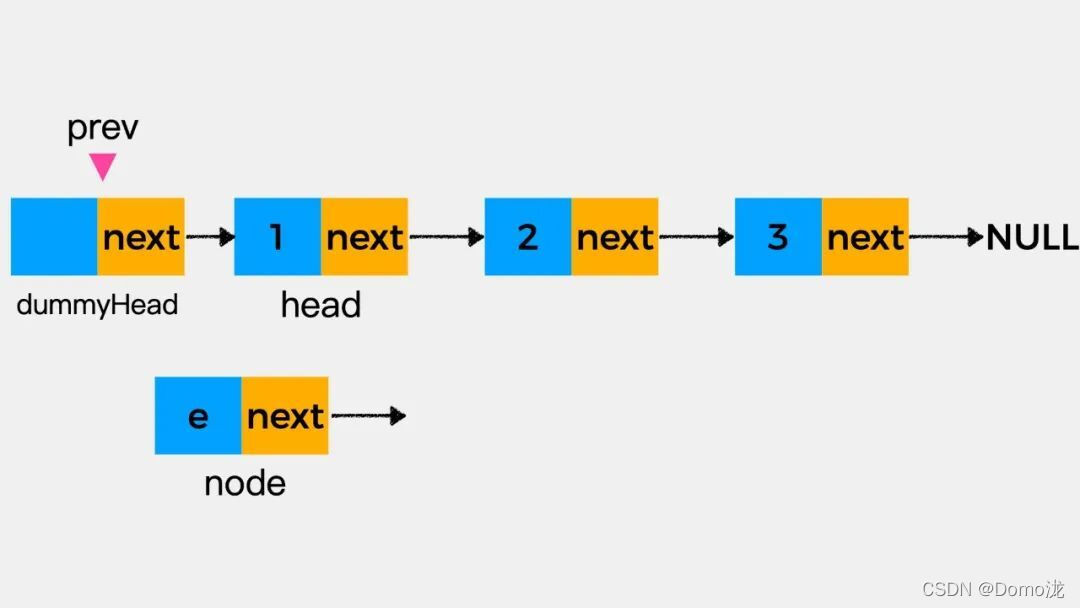
(2)链表是由一系列结点组成的,每一个结点由储存数据的数据域和储存下一个数据的地址的指针域组成;
链表的知识在之前的文章中**线性表的链式存储结构 ( 链表 ).**
list容器中的链表是一个双向循环链表,如下图所示;
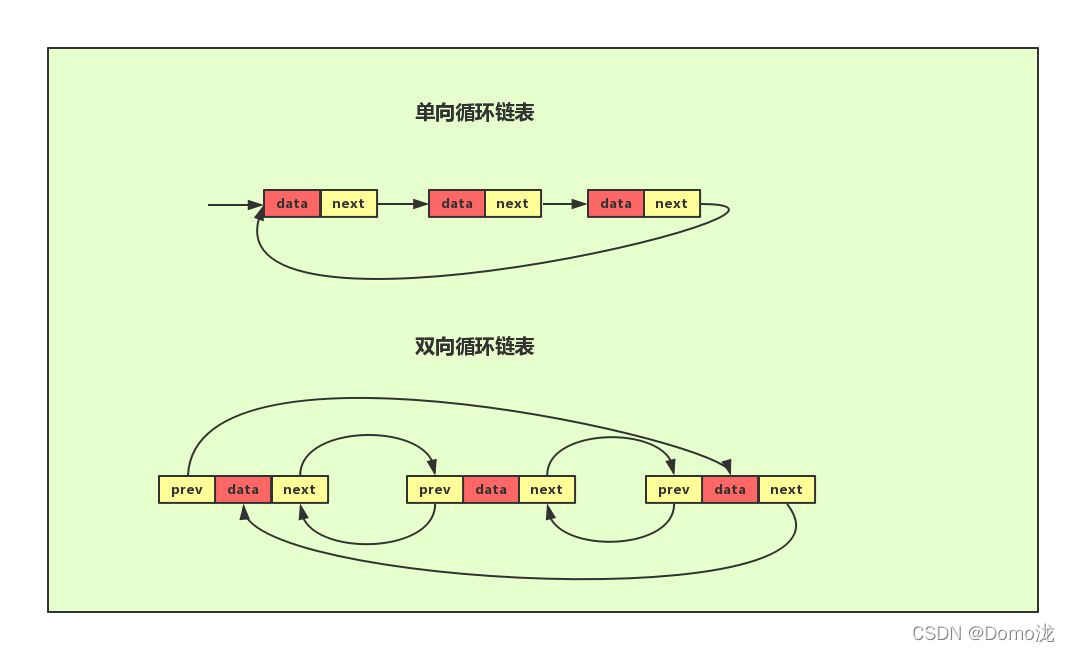
(1)list的优缺点
优
1 . 采用动态存储分配,不会造成内存浪费和溢出; 2 . 链表执行插入和删除操作十分方便,修改指针即可,不需要移动大量元素。
缺
1 .list链表的访问不支持随机访问,只能使用迭代器访问,操作较复杂 2 . 链表灵活,但是空间(指针域)和时间(遍历)额外耗费较大,遍历速度没有数组快,且占用空间比数组大。 3 . list有一个重要的性质,插入操作和删除操作都不会造成原有list迭代器的失效,这在vector是不成立的。
好了,理论知识就到这里,我们主要靠实战举例理解
2.创建list容器
构造函数原型:(和vector基本相同)
list<T> lst;
list(begin,end);
list(n,e);
list(const list &lst);
示例
#include<iostream>
using namespace std;
#include <list>
void showList01(const list<int>& L)
{
for (list<int>::const_iterator p = L.begin(); p != L.end(); p++) {
cout << *p << " ";
}
cout << endl;
}
void testit()
{
list<int>L1;
for (int i = 3; i < 50; i += 10)
{
L1.push_back(i);
}
showList01(L1);
list<int>L2(L1.begin(), L1.end());
showList01(L2);
list<int>L3(L2);
showList01(L3);
list<int>L4(10, 666);
showList01(L4);
}
int main() {
testit();
system("pause");
return 0;
}
运行结果 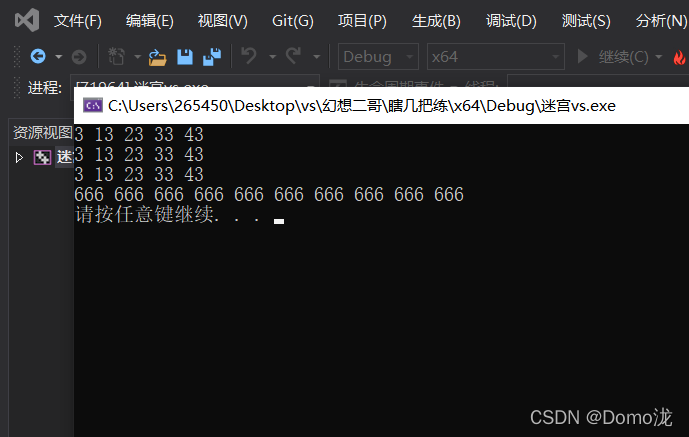
3.list容器赋值和交换
接口函数原型(和vector基本相同)
assign(begin, end);
assign(n, t);
list& operator=(const list &lst);
swap(lst);
示例
#include<iostream>
using namespace std;
#include <list>
void showList01(const list<int>& L)
{
for (list<int>::const_iterator p = L.begin(); p != L.end(); p++) {
cout << *p << " ";
}
cout << endl;
}
void testit01()
{
list<int>L1;
for (int i = 3; i < 50; i += 10)
{
L1.push_back(i);
}
showList01(L1);
list<int>L2;
L2 = L1;
showList01(L2);
list<int>L3;
L3.assign(L2.begin(), L2.end());
showList01(L3);
list<int>L4;
L4.assign(10, 33);
showList01(L4);
}
void testit02()
{
list<int>L1;
for (int i = 3; i < 50; i += 10)
{
L1.push_back(i);
}
list<int>L2;
L2.assign(10, 66);
cout << "交换前: " << endl;
showList01(L1);
showList01(L2);
cout << endl;
L1.swap(L2);
cout << "交换后: " << endl;
showList01(L1);
showList01(L2);
}
int main() {
testit01();
cout << "***********下一个测试案例**********" << endl;
testit02();
system("pause");
return 0;
}
运行结果 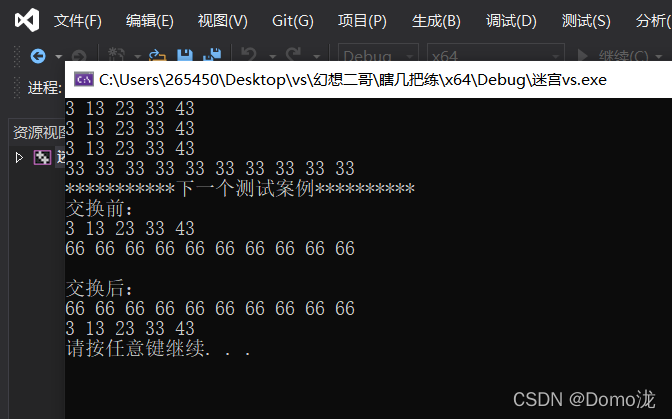
4.list大小操作
接口函数原型(和vector基本相同)
size();
empty();
resize(num);
?
resize(num, elem);
示例
#include<iostream>
using namespace std;
#include <list>
void showList01(const list<int>& L)
{
for (list<int>::const_iterator p = L.begin(); p != L.end(); p++) {
cout << *p << " ";
}
cout << endl;
}
void testit01()
{
list<int>L1;
for (int i = 3; i < 50; i += 10)
{
L1.push_back(i);
}
showList01(L1);
if (L1.empty())
{
cout << "L1为空" << endl;
}
else
{
cout << "L1不为空" << endl;
cout << "L1的大小为: " << L1.size() << endl;
}
L1.resize(10);
showList01(L1);
L1.resize(2);
showList01(L1);
}
int main() {
testit01();
system("pause");
return 0;
}
运行结果 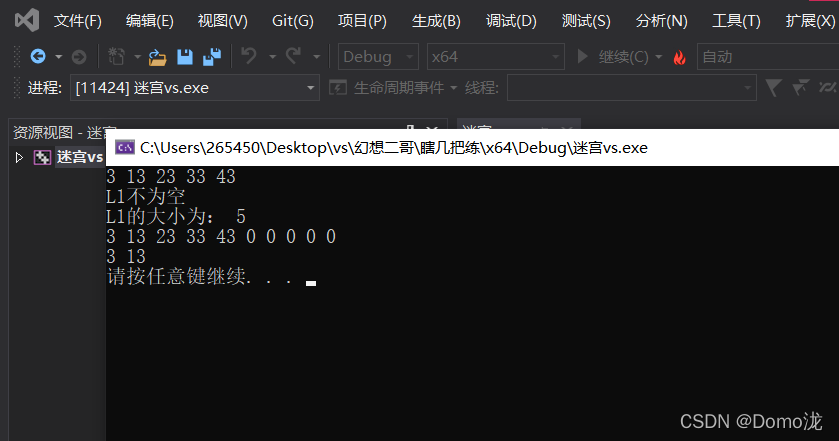
5.list插入和删除
接口函数原型.(和vector有区别点)
push_back(elem);
pop_back();
push_front(elem);
pop_front();
insert(pos,elem);
insert(pos,n,elem);
insert(pos,beg,end);
clear();
erase(beg,end);
erase(pos);
remove(elem);
示例
#include<iostream>
using namespace std;
#include <list>
void showList01(const list<int>& L)
{
for (list<int>::const_iterator p = L.begin(); p != L.end(); p++) {
cout << *p << " ";
}
cout << endl;
}
void testit01()
{
list<int> L;
L.push_back(11);
L.push_back(22);
L.push_back(33);
L.push_front(150);
L.push_front(250);
L.push_front(350);
showList01(L);
L.pop_back();
showList01(L);
L.pop_front();
showList01(L);
list<int>::iterator p = L.begin();
L.insert(++p, 888);
showList01(L);
p = L.begin();
L.erase(++p);
showList01(L);
L.push_back(9999);
L.push_back(9999);
L.push_back(999);
showList01(L);
L.remove(9999);
showList01(L);
L.clear();
showList01(L);
}
int main() {
testit01();
system("pause");
return 0;
}
运行结果 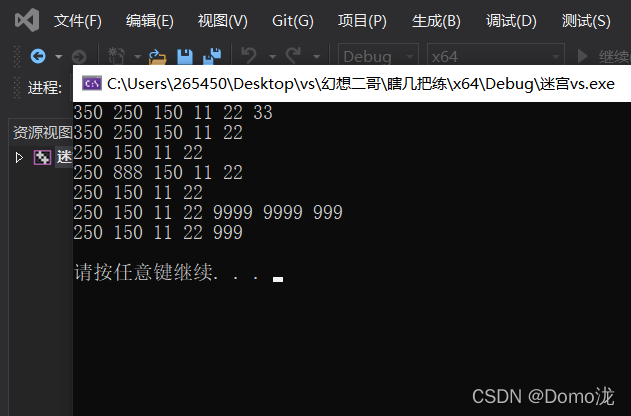
6.list数据存取
接口函数原型:
front()
back()
示例
#include<iostream>
using namespace std;
#include <list>
void testit01()
{
list<int>L1;
for (int i = 3; i < 50; i += 10)
{
L1.push_back(i);
}
cout << "第一个元素为: " << L1.front() << endl;
cout << "最后一个元素为: " << L1.back() << endl;
list<int>::iterator it = L1.begin();
it++;
cout << "第二个元素为: " << *it << endl;
it++;
cout << "第三个元素为: " << *it << endl;
}
int main() {
testit01();
system("pause");
return 0;
}
7.list反转和排序
接口函数原型
reverse();
sort();
示例
#include<iostream>
using namespace std;
#include <list>
void showList01(const list<int>& L)
{
for (list<int>::const_iterator p = L.begin(); p != L.end(); p++) {
cout << *p << " ";
}
cout << endl;
}
bool My_sortway(int a, int b)
{
return a > b;
}
void test01()
{
list<int> L;
L.push_back(99);
L.push_back(33);
L.push_back(22);
L.push_back(77);
showList01(L);
L.reverse();
cout << "反转后 :";
showList01(L);
L.sort();
cout << "从小到大排序后 :";
showList01(L);
L.sort(My_sortway);
cout << "从大到小排序后 :";
showList01(L);
}
int main() {
test01();
system("pause");
return 0;
}
运行结果 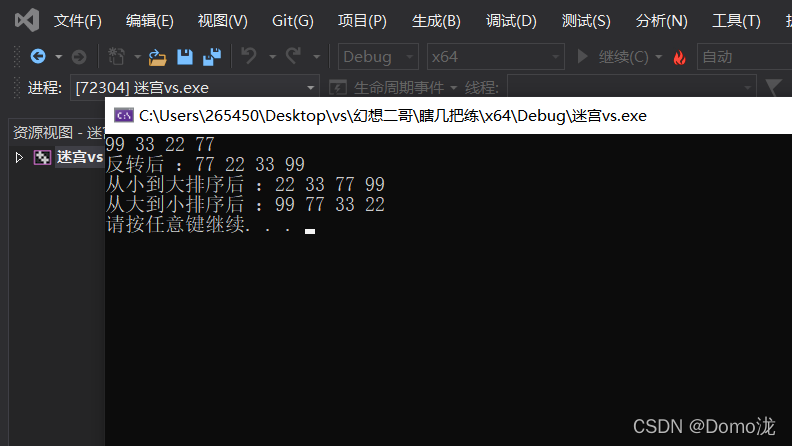
list实战(学生成绩管理系统)
题目要求: 1 .制作一个学生成绩单管理系统 2 .将student自定义数据类型进行排序,student中属性有姓名、年龄、语文成绩,数学成绩,英语成绩 排序规则:按照总成绩sum进行降序,如果总成绩sum相同按照语文成绩进行降序
源代码
#include<iostream>
using namespace std;
#include <list>
#include <string>
class Student {
public:
Student(string name, int ch, int ma,int e) {
m_Name = name;
chinese = ch;
math = ma;
English = e;
sum = ch + ma + e;
}
public:
string m_Name;
int chinese;
int math;
int English;
int sum;
};
bool ComparePerson(Student& p1, Student& p2)
{
if (p1.sum == p2.sum) {
return p1.sum < p2.sum;
}
else
{
return p1.chinese < p2.chinese;
}
}
void test() {
list<Student> k;
Student p1("杜雯菲", 88,77,95);
Student p2("杜蚊分", 67,58,26);
Student p3("李八八", 95,77,88);
Student p4("赵二蛋",86,75,68);
Student p5("王小牛", 86,46,86);
Student p6("张小哈",89,57,68);
k.push_back(p1);
k.push_back(p2);
k.push_back(p3);
k.push_back(p4);
k.push_back(p5);
k.push_back(p6);
for (list<Student>::iterator it = k.begin(); it != k.end(); it++) {
cout << "姓名: " << it->m_Name << " 语文: " << it->chinese
<< " 数学: " << it->math << " 英语: " << it->English<< " 总成绩: " << it->sum<< endl;
}
cout << "---------------------------------" << endl;
k.sort(ComparePerson);
cout << "排序后" << endl;
for (list<Student>::iterator it = k.begin(); it != k.end(); it++) {
cout << "姓名: " << it->m_Name << " 语文: " << it->chinese
<< " 数学: " << it->math << " 英语: " << it->English << " 总成绩: " << it->sum << endl;
}
}
int main() {
test();
system("pause");
return 0;
}
运行结果 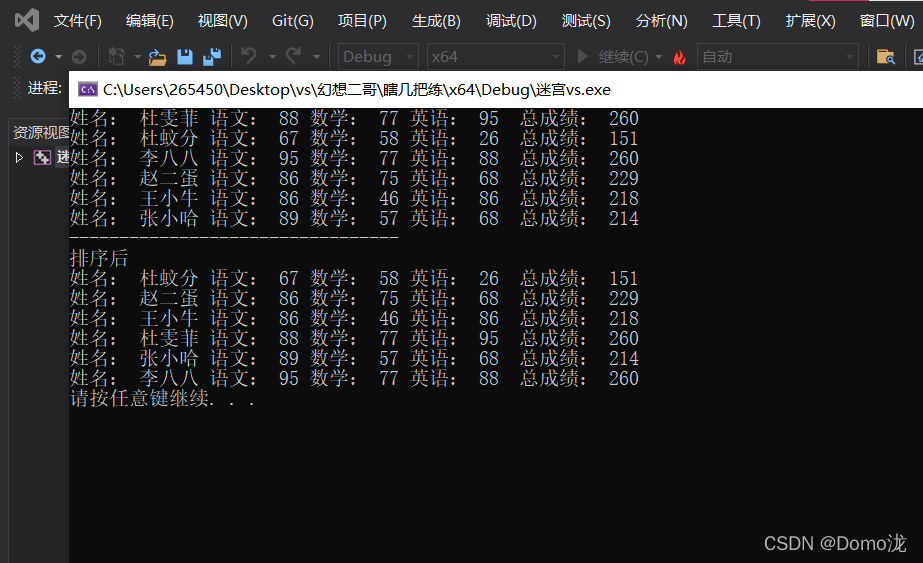
|